Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2IProvidePropertyBuilderHandler.cs / 1305376 / COM2IProvidePropertyBuilderHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using Microsoft.Win32; using System.Drawing.Design; [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal class Com2IProvidePropertyBuilderHandler : Com2ExtendedBrowsingHandler { public override Type Interface { get { return typeof(NativeMethods.IProvidePropertyBuilder); } } private bool GetBuilderGuidString(NativeMethods.IProvidePropertyBuilder target, int dispid, ref string strGuidBldr, int[] bldrType) { bool valid = false; string[] pGuidBldr = new string[1]; if (NativeMethods.Failed(target.MapPropertyToBuilder(dispid, bldrType, pGuidBldr, ref valid))) { valid = false; } if (valid && (bldrType[0] & _CTLBLDTYPE.CTLBLDTYPE_FINTERNALBUILDER) == 0) { valid = false; Debug.Fail("Property Browser doesn't support standard builders -- NYI"); } if (!valid) { return false; } if (pGuidBldr[0] == null) { strGuidBldr = Guid.Empty.ToString(); } else { strGuidBldr = pGuidBldr[0]; } return true; } public override void SetupPropertyHandlers(Com2PropertyDescriptor[] propDesc) { if (propDesc == null) { return; } for (int i = 0; i < propDesc.Length; i++) { propDesc[i].QueryGetBaseAttributes += new GetAttributesEventHandler(this.OnGetBaseAttributes); propDesc[i].QueryGetTypeConverterAndTypeEditor += new GetTypeConverterAndTypeEditorEventHandler(this.OnGetTypeConverterAndTypeEditor); } } ////// /// Here is where we handle IVsPerPropertyBrowsing.GetLocalizedPropertyInfo and IVsPerPropertyBrowsing. HideProperty /// such as IPerPropertyBrowsing, IProvidePropertyBuilder, etc. /// private void OnGetBaseAttributes(Com2PropertyDescriptor sender, GetAttributesEvent attrEvent) { NativeMethods.IProvidePropertyBuilder target = sender.TargetObject as NativeMethods.IProvidePropertyBuilder; if (target != null ) { string s = null; bool builderValid = GetBuilderGuidString(target, sender.DISPID, ref s, new int[1]); // we hide IDispatch props by default, we we need to force showing them here if (sender.CanShow && builderValid) { if (typeof(UnsafeNativeMethods.IDispatch).IsAssignableFrom(sender.PropertyType)) { attrEvent.Add(BrowsableAttribute.Yes); } } } } private void OnGetTypeConverterAndTypeEditor(Com2PropertyDescriptor sender, GetTypeConverterAndTypeEditorEvent gveevent) { object target = sender.TargetObject; if (target is NativeMethods.IProvidePropertyBuilder) { NativeMethods.IProvidePropertyBuilder propBuilder = (NativeMethods.IProvidePropertyBuilder)target; int[] pctlBldType = new int[1]; string guidString = null; if (GetBuilderGuidString(propBuilder, sender.DISPID, ref guidString, pctlBldType)) { gveevent.TypeEditor = new Com2PropertyBuilderUITypeEditor(sender, guidString, pctlBldType[0], (UITypeEditor)gveevent.TypeEditor); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using Microsoft.Win32; using System.Drawing.Design; [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal class Com2IProvidePropertyBuilderHandler : Com2ExtendedBrowsingHandler { public override Type Interface { get { return typeof(NativeMethods.IProvidePropertyBuilder); } } private bool GetBuilderGuidString(NativeMethods.IProvidePropertyBuilder target, int dispid, ref string strGuidBldr, int[] bldrType) { bool valid = false; string[] pGuidBldr = new string[1]; if (NativeMethods.Failed(target.MapPropertyToBuilder(dispid, bldrType, pGuidBldr, ref valid))) { valid = false; } if (valid && (bldrType[0] & _CTLBLDTYPE.CTLBLDTYPE_FINTERNALBUILDER) == 0) { valid = false; Debug.Fail("Property Browser doesn't support standard builders -- NYI"); } if (!valid) { return false; } if (pGuidBldr[0] == null) { strGuidBldr = Guid.Empty.ToString(); } else { strGuidBldr = pGuidBldr[0]; } return true; } public override void SetupPropertyHandlers(Com2PropertyDescriptor[] propDesc) { if (propDesc == null) { return; } for (int i = 0; i < propDesc.Length; i++) { propDesc[i].QueryGetBaseAttributes += new GetAttributesEventHandler(this.OnGetBaseAttributes); propDesc[i].QueryGetTypeConverterAndTypeEditor += new GetTypeConverterAndTypeEditorEventHandler(this.OnGetTypeConverterAndTypeEditor); } } ////// /// Here is where we handle IVsPerPropertyBrowsing.GetLocalizedPropertyInfo and IVsPerPropertyBrowsing. HideProperty /// such as IPerPropertyBrowsing, IProvidePropertyBuilder, etc. /// private void OnGetBaseAttributes(Com2PropertyDescriptor sender, GetAttributesEvent attrEvent) { NativeMethods.IProvidePropertyBuilder target = sender.TargetObject as NativeMethods.IProvidePropertyBuilder; if (target != null ) { string s = null; bool builderValid = GetBuilderGuidString(target, sender.DISPID, ref s, new int[1]); // we hide IDispatch props by default, we we need to force showing them here if (sender.CanShow && builderValid) { if (typeof(UnsafeNativeMethods.IDispatch).IsAssignableFrom(sender.PropertyType)) { attrEvent.Add(BrowsableAttribute.Yes); } } } } private void OnGetTypeConverterAndTypeEditor(Com2PropertyDescriptor sender, GetTypeConverterAndTypeEditorEvent gveevent) { object target = sender.TargetObject; if (target is NativeMethods.IProvidePropertyBuilder) { NativeMethods.IProvidePropertyBuilder propBuilder = (NativeMethods.IProvidePropertyBuilder)target; int[] pctlBldType = new int[1]; string guidString = null; if (GetBuilderGuidString(propBuilder, sender.DISPID, ref guidString, pctlBldType)) { gveevent.TypeEditor = new Com2PropertyBuilderUITypeEditor(sender, guidString, pctlBldType[0], (UITypeEditor)gveevent.TypeEditor); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
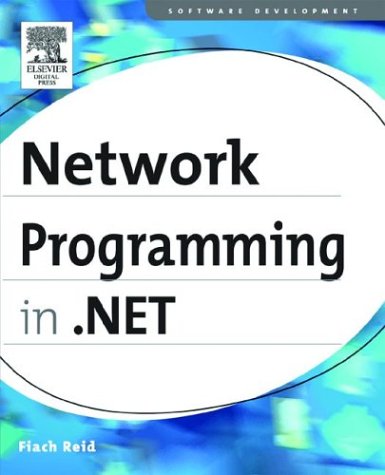
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RightNameExpirationInfoPair.cs
- AddInController.cs
- ProfileSettings.cs
- TableLayoutPanelDesigner.cs
- FrameworkElement.cs
- Sql8ConformanceChecker.cs
- MexServiceChannelBuilder.cs
- DriveInfo.cs
- BindingExpressionUncommonField.cs
- ObjectContextServiceProvider.cs
- Socket.cs
- LayoutEvent.cs
- SchemaImporter.cs
- HtmlProps.cs
- SimpleTypesSurrogate.cs
- DataSetUtil.cs
- DragDropHelper.cs
- ConstantExpression.cs
- SizeFConverter.cs
- HTMLTextWriter.cs
- ConnectionString.cs
- SwitchElementsCollection.cs
- StyleHelper.cs
- ScrollBar.cs
- SchemaElementDecl.cs
- ObjectSet.cs
- WebProxyScriptElement.cs
- DbMetaDataCollectionNames.cs
- StackBuilderSink.cs
- XmlSerializerNamespaces.cs
- updatecommandorderer.cs
- HtmlTableCellCollection.cs
- SystemColorTracker.cs
- ServiceBehaviorAttribute.cs
- WinCategoryAttribute.cs
- ObjectSet.cs
- GenericEnumerator.cs
- XmlDocument.cs
- IteratorFilter.cs
- METAHEADER.cs
- CollectionViewGroupRoot.cs
- ToolBarTray.cs
- AdapterUtil.cs
- BitStack.cs
- NativeCompoundFileAPIs.cs
- ChangePassword.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- AmbiguousMatchException.cs
- ToolStripDropDownClosedEventArgs.cs
- InheritanceRules.cs
- AnnotationAuthorChangedEventArgs.cs
- _HeaderInfo.cs
- DataBindingCollection.cs
- TemplateKeyConverter.cs
- InputLanguageEventArgs.cs
- ContextCorrelationInitializer.cs
- GraphicsContainer.cs
- CacheDependency.cs
- ADConnectionHelper.cs
- PageBreakRecord.cs
- SQLUtility.cs
- AutomationElement.cs
- FixedDocumentSequencePaginator.cs
- ResourceManagerWrapper.cs
- SqlConnectionFactory.cs
- MdiWindowListItemConverter.cs
- TextParentUndoUnit.cs
- RefreshPropertiesAttribute.cs
- ExtensibleClassFactory.cs
- URI.cs
- WebScriptMetadataMessageEncoderFactory.cs
- RectAnimation.cs
- IndicFontClient.cs
- TextSpan.cs
- InternalConfigHost.cs
- RolePrincipal.cs
- HtmlHead.cs
- PropertyValueUIItem.cs
- DataGridToolTip.cs
- DurationConverter.cs
- DirectionalLight.cs
- StatusBarAutomationPeer.cs
- TextLine.cs
- GenericQueueSurrogate.cs
- pingexception.cs
- IndexedEnumerable.cs
- SingleSelectRootGridEntry.cs
- ExcCanonicalXml.cs
- ExpressionBuilder.cs
- EncoderFallback.cs
- BadImageFormatException.cs
- EllipseGeometry.cs
- ScriptingProfileServiceSection.cs
- StaticFileHandler.cs
- BamlRecords.cs
- AnonymousIdentificationSection.cs
- BamlTreeUpdater.cs
- ErrorRuntimeConfig.cs
- RefreshEventArgs.cs
- ScrollBar.cs