Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / InputLanguageEventArgs.cs / 1305600 / InputLanguageEventArgs.cs
using System; using System.Collections; using System.Windows.Threading; using System.Windows; using System.Globalization; namespace System.Windows.Input { ////// The InputLanguageEventArgs class represents a type of /// RoutedEventArgs that are relevant to events raised to indicate /// changes. /// public abstract class InputLanguageEventArgs : EventArgs { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Constructs an instance of the InputLanguageEventArgs class. /// /// /// The new language id. /// /// /// The previous language id. /// protected InputLanguageEventArgs(CultureInfo newLanguageId, CultureInfo previousLanguageId) { _newLanguageId = newLanguageId; _previousLanguageId = previousLanguageId; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// New Language Id. /// public virtual CultureInfo NewLanguage { get { return _newLanguageId; } } ////// Previous Language Id. /// public virtual CultureInfo PreviousLanguage { get { return _previousLanguageId; } } //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // the new input language. private CultureInfo _newLanguageId; // the previous input language. private CultureInfo _previousLanguageId; #endregion Private Fields } ////// The InputLanguageEventArgs class represents a type of /// RoutedEventArgs that are relevant to events raised to indicate /// changes. /// public class InputLanguageChangedEventArgs : InputLanguageEventArgs { //------------------------------------------------------ // // Constructors // //------------------------------------------------------ ////// Constructs an instance of the InputLanguageEventArgs class. /// /// /// The new language id. /// /// /// The new language id. /// public InputLanguageChangedEventArgs(CultureInfo newLanguageId, CultureInfo previousLanguageId) : base(newLanguageId, previousLanguageId) { } } ////// The InputLanguageEventArgs class represents a type of /// RoutedEventArgs that are relevant to events raised to indicate /// changes. /// ///public class InputLanguageChangingEventArgs : InputLanguageEventArgs { //----------------------------------------------------- // // Constructors // //------------------------------------------------------ /// /// Constructs an instance of the InputLanguageEventArgs class. /// /// /// The new language id. /// /// /// The previous language id. /// public InputLanguageChangingEventArgs(CultureInfo newLanguageId, CultureInfo previousLanguageId) : base(newLanguageId, previousLanguageId) { _rejected = false; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// This is a value to reject the input language change. /// public bool Rejected { get { return _rejected; } set { _rejected = value; } } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // bool to reject the input language change. private bool _rejected; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Windows.Threading; using System.Windows; using System.Globalization; namespace System.Windows.Input { ////// The InputLanguageEventArgs class represents a type of /// RoutedEventArgs that are relevant to events raised to indicate /// changes. /// public abstract class InputLanguageEventArgs : EventArgs { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Constructs an instance of the InputLanguageEventArgs class. /// /// /// The new language id. /// /// /// The previous language id. /// protected InputLanguageEventArgs(CultureInfo newLanguageId, CultureInfo previousLanguageId) { _newLanguageId = newLanguageId; _previousLanguageId = previousLanguageId; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// New Language Id. /// public virtual CultureInfo NewLanguage { get { return _newLanguageId; } } ////// Previous Language Id. /// public virtual CultureInfo PreviousLanguage { get { return _previousLanguageId; } } //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // the new input language. private CultureInfo _newLanguageId; // the previous input language. private CultureInfo _previousLanguageId; #endregion Private Fields } ////// The InputLanguageEventArgs class represents a type of /// RoutedEventArgs that are relevant to events raised to indicate /// changes. /// public class InputLanguageChangedEventArgs : InputLanguageEventArgs { //------------------------------------------------------ // // Constructors // //------------------------------------------------------ ////// Constructs an instance of the InputLanguageEventArgs class. /// /// /// The new language id. /// /// /// The new language id. /// public InputLanguageChangedEventArgs(CultureInfo newLanguageId, CultureInfo previousLanguageId) : base(newLanguageId, previousLanguageId) { } } ////// The InputLanguageEventArgs class represents a type of /// RoutedEventArgs that are relevant to events raised to indicate /// changes. /// ///public class InputLanguageChangingEventArgs : InputLanguageEventArgs { //----------------------------------------------------- // // Constructors // //------------------------------------------------------ /// /// Constructs an instance of the InputLanguageEventArgs class. /// /// /// The new language id. /// /// /// The previous language id. /// public InputLanguageChangingEventArgs(CultureInfo newLanguageId, CultureInfo previousLanguageId) : base(newLanguageId, previousLanguageId) { _rejected = false; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// This is a value to reject the input language change. /// public bool Rejected { get { return _rejected; } set { _rejected = value; } } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // bool to reject the input language change. private bool _rejected; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
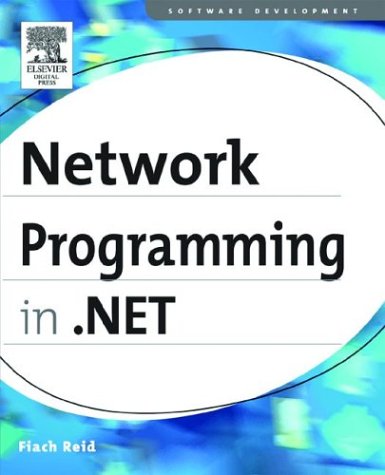
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LingerOption.cs
- LogicalTreeHelper.cs
- BindUriHelper.cs
- AccessViolationException.cs
- SqlCommandBuilder.cs
- OdbcCommandBuilder.cs
- CallSiteHelpers.cs
- PostBackOptions.cs
- UnsafeNativeMethods.cs
- InputLanguage.cs
- TypeToken.cs
- XPathAncestorQuery.cs
- IdentityManager.cs
- Compiler.cs
- CollectionViewGroupInternal.cs
- QueryTreeBuilder.cs
- HttpProfileBase.cs
- RecognizerBase.cs
- PathFigure.cs
- WebPartCollection.cs
- SecurityCriticalDataForSet.cs
- CodeAccessSecurityEngine.cs
- ScrollViewerAutomationPeer.cs
- NetCodeGroup.cs
- TextBoxView.cs
- _IPv4Address.cs
- TextTreeTextNode.cs
- FlowNode.cs
- DataGridCellClipboardEventArgs.cs
- BindableAttribute.cs
- UriScheme.cs
- PropertySourceInfo.cs
- EndSelectCardRequest.cs
- ReadOnlyCollectionBase.cs
- SerializationSectionGroup.cs
- ControlBuilderAttribute.cs
- AuthenticationModulesSection.cs
- CodeDirectoryCompiler.cs
- HtmlShim.cs
- DataObjectEventArgs.cs
- StyleReferenceConverter.cs
- Link.cs
- PolyBezierSegment.cs
- OdbcError.cs
- TransactionBridge.cs
- ProfileServiceManager.cs
- XmlNullResolver.cs
- PagesSection.cs
- CodeTypeDelegate.cs
- GeometryCombineModeValidation.cs
- RuntimeArgumentHandle.cs
- Trigger.cs
- IconBitmapDecoder.cs
- X509Certificate2Collection.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ProcessModelSection.cs
- ObjectDataSourceDisposingEventArgs.cs
- WebBrowserHelper.cs
- LoginCancelEventArgs.cs
- SmtpTransport.cs
- DrawingContextDrawingContextWalker.cs
- ExpressionNode.cs
- EntityKeyElement.cs
- ICspAsymmetricAlgorithm.cs
- AsyncResult.cs
- XPathNavigator.cs
- WebBrowser.cs
- XmlElementList.cs
- ClientUtils.cs
- XmlILConstructAnalyzer.cs
- ToolStripGripRenderEventArgs.cs
- CheckBoxStandardAdapter.cs
- TransactionProtocol.cs
- HatchBrush.cs
- XmlResolver.cs
- CompiledQueryCacheKey.cs
- FixedHyperLink.cs
- CredentialCache.cs
- TransformedBitmap.cs
- CompilerCollection.cs
- Transform.cs
- MethodImplAttribute.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- OneOfConst.cs
- TextEditorParagraphs.cs
- ComponentEditorForm.cs
- StylusTip.cs
- UnlockInstanceCommand.cs
- CancellationState.cs
- SubqueryRules.cs
- propertytag.cs
- CompilerCollection.cs
- ProviderConnectionPoint.cs
- DataGridViewDataConnection.cs
- DuplicateWaitObjectException.cs
- BufferedGraphicsManager.cs
- TranslateTransform.cs
- SaveFileDialog.cs
- Brushes.cs
- AmbientEnvironment.cs