Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Data / CollectionViewGroupInternal.cs / 1458001 / CollectionViewGroupInternal.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: A CollectionViewGroupInternal, as created by a CollectionView according to a GroupDescription. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.ComponentModel; // PropertyChangedEventArgs, GroupDescription using System.Windows; // DependencyProperty.UnsetValue using System.Windows.Data; // CollectionViewGroup namespace MS.Internal.Data { internal class CollectionViewGroupInternal : CollectionViewGroup { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- internal CollectionViewGroupInternal(object name, CollectionViewGroupInternal parent) : base(name) { _parentGroup = parent; } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// Is this group at the bottom level (not further subgrouped). /// public override bool IsBottomLevel { get { return (_groupBy == null); } } #endregion Public Properties #region Internal Properties //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ // how this group divides into subgroups internal GroupDescription GroupBy { get { return _groupBy; } set { bool oldIsBottomLevel = IsBottomLevel; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged -= new PropertyChangedEventHandler(OnGroupByChanged); _groupBy = value; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged += new PropertyChangedEventHandler(OnGroupByChanged); if (oldIsBottomLevel != IsBottomLevel) { OnPropertyChanged(new PropertyChangedEventArgs("IsBottomLevel")); } } } // the number of items and groups in the subtree under this group internal int FullCount { get { return _fullCount; } set { _fullCount = value; } } // the most recent index where actvity took place internal int LastIndex { get { return _lastIndex; } set { _lastIndex = value; } } // the first item (leaf) added to this group. If this can't be determined, // DependencyProperty.UnsetValue. internal object SeedItem { get { if (ItemCount > 0 && (GroupBy == null || GroupBy.GroupNames.Count == 0)) { // look for first item, child by child for (int k=0, n=Items.Count; k0) { // child is a nonempty subgroup - ask it return subgroup.SeedItem; } // otherwise child is an empty subgroup - go to next child } // we shouldn't get here, but just in case... return DependencyProperty.UnsetValue; } else { // the group is empty, or it has explicit subgroups. // In either case, we cannot determine the first item - // it could have gone into any of the subgroups. return DependencyProperty.UnsetValue; } } } #endregion Properties #region Internal methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ internal void Add(object item) { ChangeCounts(item, +1); ProtectedItems.Add(item); } internal int Remove(object item, bool returnLeafIndex) { int index = -1; int localIndex = ProtectedItems.IndexOf(item); if (localIndex >= 0) { if (returnLeafIndex) { index = LeafIndexFromItem(null, localIndex); } ChangeCounts(item, -1); ProtectedItems.RemoveAt(localIndex); } return index; } internal void Clear() { FullCount = 1; ProtectedItemCount = 0; ProtectedItems.Clear(); } // return the index of the given item within the list of leaves governed // by this group internal int LeafIndexOf(object item) { int leaves = 0; // number of leaves we've passed over so far for (int k=0, n=Items.Count; k y } } // if we don't see either x or y, declare x > y. // This has the effect of putting x at the end of the list. return + 1; } int _index; IList _list; } #endregion Internal Types #region Private Properties //----------------------------------------------------- // // Private Properties // //----------------------------------------------------- private CollectionViewGroupInternal Parent { get { return _parentGroup; } } #endregion Private Properties #region Private methods //----------------------------------------------------- // // Private methods // //------------------------------------------------------ protected void ChangeCounts(object item, int delta) { bool changeLeafCount = !(item is CollectionViewGroup); for ( CollectionViewGroupInternal group = this; group != null; group = group._parentGroup ) { group.FullCount += delta; if (changeLeafCount) { group.ProtectedItemCount += delta; if (group.ProtectedItemCount == 0) { RemoveEmptyGroup(group); } } } unchecked {++_version;} // this invalidates enumerators } void RemoveEmptyGroup(CollectionViewGroupInternal group) { CollectionViewGroupInternal parent = group.Parent; if (parent != null) { GroupDescription groupBy = parent.GroupBy; int index = parent.ProtectedItems.IndexOf(group); // remove the subgroup unless it is one of the explicit groups if (index >= groupBy.GroupNames.Count) { parent.Remove(group, false); } } } void OnGroupByChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e) { OnGroupByChanged(); } #endregion Private methods #region Private fields //----------------------------------------------------- // // Private fields // //------------------------------------------------------ GroupDescription _groupBy; CollectionViewGroupInternal _parentGroup; int _fullCount = 1; int _lastIndex; int _version; // for detecting stale enumerators #endregion Private fields #region Private classes //------------------------------------------------------ // // Private classes // //----------------------------------------------------- private class LeafEnumerator : IEnumerator { public LeafEnumerator(CollectionViewGroupInternal group) { _group = group; DoReset(); // don't call virtual Reset in ctor } void IEnumerator.Reset() { DoReset(); } void DoReset() { _version = _group._version; _index = -1; _subEnum = null; } bool IEnumerator.MoveNext() { // check for invalidated enumerator if (_group._version != _version) throw new InvalidOperationException(); // move forward to the next leaf while (_subEnum == null || !_subEnum.MoveNext()) { // done with the current top-level item. Move to the next one. ++ _index; if (_index >= _group.Items.Count) return false; CollectionViewGroupInternal subgroup = _group.Items[_index] as CollectionViewGroupInternal; if (subgroup == null) { // current item is a leaf - it's the new Current _current = _group.Items[_index]; _subEnum = null; return true; } else { // current item is a subgroup - get its enumerator _subEnum = subgroup.GetLeafEnumerator(); } } // the loop terminates only when we have a subgroup enumerator // positioned at the new Current item _current = _subEnum.Current; return true; } object IEnumerator.Current { get { if (_index < 0 || _index >= _group.Items.Count) throw new InvalidOperationException(); return _current; } } CollectionViewGroupInternal _group; // parent group int _version; // parent group's version at ctor int _index; // current index into Items IEnumerator _subEnum; // enumerator over current subgroup object _current; // current item } #endregion Private classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: A CollectionViewGroupInternal, as created by a CollectionView according to a GroupDescription. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.ComponentModel; // PropertyChangedEventArgs, GroupDescription using System.Windows; // DependencyProperty.UnsetValue using System.Windows.Data; // CollectionViewGroup namespace MS.Internal.Data { internal class CollectionViewGroupInternal : CollectionViewGroup { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- internal CollectionViewGroupInternal(object name, CollectionViewGroupInternal parent) : base(name) { _parentGroup = parent; } #endregion Constructors #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// Is this group at the bottom level (not further subgrouped). /// public override bool IsBottomLevel { get { return (_groupBy == null); } } #endregion Public Properties #region Internal Properties //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ // how this group divides into subgroups internal GroupDescription GroupBy { get { return _groupBy; } set { bool oldIsBottomLevel = IsBottomLevel; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged -= new PropertyChangedEventHandler(OnGroupByChanged); _groupBy = value; if (_groupBy != null) ((INotifyPropertyChanged)_groupBy).PropertyChanged += new PropertyChangedEventHandler(OnGroupByChanged); if (oldIsBottomLevel != IsBottomLevel) { OnPropertyChanged(new PropertyChangedEventArgs("IsBottomLevel")); } } } // the number of items and groups in the subtree under this group internal int FullCount { get { return _fullCount; } set { _fullCount = value; } } // the most recent index where actvity took place internal int LastIndex { get { return _lastIndex; } set { _lastIndex = value; } } // the first item (leaf) added to this group. If this can't be determined, // DependencyProperty.UnsetValue. internal object SeedItem { get { if (ItemCount > 0 && (GroupBy == null || GroupBy.GroupNames.Count == 0)) { // look for first item, child by child for (int k=0, n=Items.Count; k0) { // child is a nonempty subgroup - ask it return subgroup.SeedItem; } // otherwise child is an empty subgroup - go to next child } // we shouldn't get here, but just in case... return DependencyProperty.UnsetValue; } else { // the group is empty, or it has explicit subgroups. // In either case, we cannot determine the first item - // it could have gone into any of the subgroups. return DependencyProperty.UnsetValue; } } } #endregion Properties #region Internal methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ internal void Add(object item) { ChangeCounts(item, +1); ProtectedItems.Add(item); } internal int Remove(object item, bool returnLeafIndex) { int index = -1; int localIndex = ProtectedItems.IndexOf(item); if (localIndex >= 0) { if (returnLeafIndex) { index = LeafIndexFromItem(null, localIndex); } ChangeCounts(item, -1); ProtectedItems.RemoveAt(localIndex); } return index; } internal void Clear() { FullCount = 1; ProtectedItemCount = 0; ProtectedItems.Clear(); } // return the index of the given item within the list of leaves governed // by this group internal int LeafIndexOf(object item) { int leaves = 0; // number of leaves we've passed over so far for (int k=0, n=Items.Count; k y } } // if we don't see either x or y, declare x > y. // This has the effect of putting x at the end of the list. return + 1; } int _index; IList _list; } #endregion Internal Types #region Private Properties //----------------------------------------------------- // // Private Properties // //----------------------------------------------------- private CollectionViewGroupInternal Parent { get { return _parentGroup; } } #endregion Private Properties #region Private methods //----------------------------------------------------- // // Private methods // //------------------------------------------------------ protected void ChangeCounts(object item, int delta) { bool changeLeafCount = !(item is CollectionViewGroup); for ( CollectionViewGroupInternal group = this; group != null; group = group._parentGroup ) { group.FullCount += delta; if (changeLeafCount) { group.ProtectedItemCount += delta; if (group.ProtectedItemCount == 0) { RemoveEmptyGroup(group); } } } unchecked {++_version;} // this invalidates enumerators } void RemoveEmptyGroup(CollectionViewGroupInternal group) { CollectionViewGroupInternal parent = group.Parent; if (parent != null) { GroupDescription groupBy = parent.GroupBy; int index = parent.ProtectedItems.IndexOf(group); // remove the subgroup unless it is one of the explicit groups if (index >= groupBy.GroupNames.Count) { parent.Remove(group, false); } } } void OnGroupByChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e) { OnGroupByChanged(); } #endregion Private methods #region Private fields //----------------------------------------------------- // // Private fields // //------------------------------------------------------ GroupDescription _groupBy; CollectionViewGroupInternal _parentGroup; int _fullCount = 1; int _lastIndex; int _version; // for detecting stale enumerators #endregion Private fields #region Private classes //------------------------------------------------------ // // Private classes // //----------------------------------------------------- private class LeafEnumerator : IEnumerator { public LeafEnumerator(CollectionViewGroupInternal group) { _group = group; DoReset(); // don't call virtual Reset in ctor } void IEnumerator.Reset() { DoReset(); } void DoReset() { _version = _group._version; _index = -1; _subEnum = null; } bool IEnumerator.MoveNext() { // check for invalidated enumerator if (_group._version != _version) throw new InvalidOperationException(); // move forward to the next leaf while (_subEnum == null || !_subEnum.MoveNext()) { // done with the current top-level item. Move to the next one. ++ _index; if (_index >= _group.Items.Count) return false; CollectionViewGroupInternal subgroup = _group.Items[_index] as CollectionViewGroupInternal; if (subgroup == null) { // current item is a leaf - it's the new Current _current = _group.Items[_index]; _subEnum = null; return true; } else { // current item is a subgroup - get its enumerator _subEnum = subgroup.GetLeafEnumerator(); } } // the loop terminates only when we have a subgroup enumerator // positioned at the new Current item _current = _subEnum.Current; return true; } object IEnumerator.Current { get { if (_index < 0 || _index >= _group.Items.Count) throw new InvalidOperationException(); return _current; } } CollectionViewGroupInternal _group; // parent group int _version; // parent group's version at ctor int _index; // current index into Items IEnumerator _subEnum; // enumerator over current subgroup object _current; // current item } #endregion Private classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
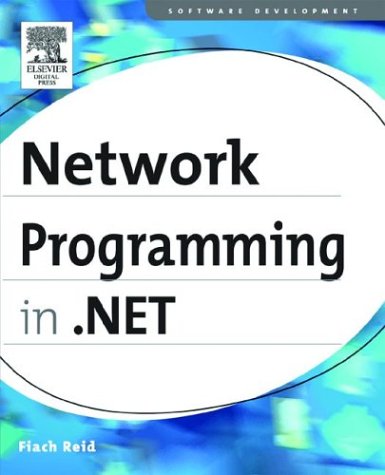
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InternalsVisibleToAttribute.cs
- TransformDescriptor.cs
- SmiMetaData.cs
- ParallelDesigner.cs
- UInt16.cs
- XmlText.cs
- MembershipValidatePasswordEventArgs.cs
- XmlAttributeProperties.cs
- OperatingSystem.cs
- ToolStripSeparatorRenderEventArgs.cs
- ADConnectionHelper.cs
- Screen.cs
- EntityAdapter.cs
- ArgumentDesigner.xaml.cs
- ReadContentAsBinaryHelper.cs
- RouteParametersHelper.cs
- BlockUIContainer.cs
- EntityProxyTypeInfo.cs
- PathTooLongException.cs
- WorkflowDesigner.cs
- XmlAnyElementAttribute.cs
- XmlHierarchicalEnumerable.cs
- SpeechEvent.cs
- PriorityRange.cs
- ErrorFormatterPage.cs
- Calendar.cs
- TextEndOfParagraph.cs
- DataControlField.cs
- ArrayElementGridEntry.cs
- ObjRef.cs
- MaskedTextBoxTextEditor.cs
- UdpDiscoveryEndpoint.cs
- ProxyWebPart.cs
- X509Certificate.cs
- SchemaRegistration.cs
- PrimitiveDataContract.cs
- RecordBuilder.cs
- validationstate.cs
- WebPartZoneCollection.cs
- NonBatchDirectoryCompiler.cs
- DiagnosticSection.cs
- AxHost.cs
- XmlHierarchicalDataSourceView.cs
- UserControl.cs
- FamilyCollection.cs
- XPSSignatureDefinition.cs
- MoveSizeWinEventHandler.cs
- EqualityComparer.cs
- DetailsViewModeEventArgs.cs
- BaseTreeIterator.cs
- LinearGradientBrush.cs
- ProcessHostConfigUtils.cs
- DataGridColumn.cs
- NetworkStream.cs
- MessageSecurityOverMsmqElement.cs
- DataGridViewCellValidatingEventArgs.cs
- GeneralTransform3DTo2DTo3D.cs
- ACE.cs
- BaseCAMarshaler.cs
- ListenerElementsCollection.cs
- DataGridViewColumnConverter.cs
- ChannelListenerBase.cs
- TraversalRequest.cs
- AssemblyBuilder.cs
- TrackingServices.cs
- CodeChecksumPragma.cs
- RoutedEventConverter.cs
- SecurityContextCookieSerializer.cs
- GeneralTransformCollection.cs
- ConfigXmlWhitespace.cs
- SqlClientWrapperSmiStream.cs
- SqlHelper.cs
- SoapException.cs
- TraceListeners.cs
- IssuedTokenClientCredential.cs
- TextEditorThreadLocalStore.cs
- InstanceDataCollection.cs
- WebConvert.cs
- WebConfigurationFileMap.cs
- PeerNameRegistration.cs
- ParseElement.cs
- cookieexception.cs
- newinstructionaction.cs
- TraceHandlerErrorFormatter.cs
- LOSFormatter.cs
- TreeViewEvent.cs
- CreateDataSourceDialog.cs
- CompilerTypeWithParams.cs
- XmlBufferReader.cs
- ListDependantCardsRequest.cs
- Attachment.cs
- DocumentViewerAutomationPeer.cs
- DeflateStream.cs
- DbConnectionStringCommon.cs
- CreateSequenceResponse.cs
- WebPartConnectionsEventArgs.cs
- MsmqHostedTransportConfiguration.cs
- ImageConverter.cs
- RenderingBiasValidation.cs
- Brushes.cs