Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / MoveSizeWinEventHandler.cs / 1305600 / MoveSizeWinEventHandler.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: MoveSizeWinEventHandler implementation. // // History: // 02/04/2005 : yutakas - created. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; namespace System.Windows.Documents { internal class MoveSizeWinEventHandler : WinEventHandler { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // ctor that takes a range of events internal MoveSizeWinEventHandler() : base(NativeMethods.EVENT_SYSTEM_MOVESIZEEND, NativeMethods.EVENT_SYSTEM_MOVESIZEEND) { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Register text store that will receive move/sice event. internal void RegisterTextStore(TextStore textstore) { if (_arTextStore == null) { _arTextStore = new ArrayList(1); } _arTextStore.Add(textstore); } // Unregister text store. internal void UnregisterTextStore(TextStore textstore) { _arTextStore.Remove(textstore); } // The callback from WinEvent. ////// Critical - as this invokes Critical method CriticalSourceHwnd /// TreatAsSafe - as this doesn't expose this information but just calls OnLayoutUpdated on the TextStore. /// [SecurityCritical, SecurityTreatAsSafe] internal override void WinEventProc(int eventId, IntPtr hwnd) { Invariant.Assert(eventId == NativeMethods.EVENT_SYSTEM_MOVESIZEEND); if (_arTextStore != null) { for (int i = 0; i < _arTextStore.Count; i++) { bool notified = false; TextStore textstore = (TextStore)_arTextStore[i]; IntPtr hwndTemp = textstore.CriticalSourceWnd; while (hwndTemp != IntPtr.Zero) { if (hwnd == hwndTemp) { // Only when the parent window of the source of this TextStore is // moved or resized, we notfiy to Cicero. textstore.OnLayoutUpdated(); notified = true; break; } hwndTemp = UnsafeNativeMethods.GetParent(new HandleRef(this, hwndTemp)); } if (!notified) textstore.MakeLayoutChangeOnGotFocus(); } } } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties // Number of TextStores listening to this event. internal int TextStoreCount { get { return _arTextStore.Count; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // list of the registered TextStores. private ArrayList _arTextStore; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: MoveSizeWinEventHandler implementation. // // History: // 02/04/2005 : yutakas - created. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; namespace System.Windows.Documents { internal class MoveSizeWinEventHandler : WinEventHandler { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // ctor that takes a range of events internal MoveSizeWinEventHandler() : base(NativeMethods.EVENT_SYSTEM_MOVESIZEEND, NativeMethods.EVENT_SYSTEM_MOVESIZEEND) { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Register text store that will receive move/sice event. internal void RegisterTextStore(TextStore textstore) { if (_arTextStore == null) { _arTextStore = new ArrayList(1); } _arTextStore.Add(textstore); } // Unregister text store. internal void UnregisterTextStore(TextStore textstore) { _arTextStore.Remove(textstore); } // The callback from WinEvent. ////// Critical - as this invokes Critical method CriticalSourceHwnd /// TreatAsSafe - as this doesn't expose this information but just calls OnLayoutUpdated on the TextStore. /// [SecurityCritical, SecurityTreatAsSafe] internal override void WinEventProc(int eventId, IntPtr hwnd) { Invariant.Assert(eventId == NativeMethods.EVENT_SYSTEM_MOVESIZEEND); if (_arTextStore != null) { for (int i = 0; i < _arTextStore.Count; i++) { bool notified = false; TextStore textstore = (TextStore)_arTextStore[i]; IntPtr hwndTemp = textstore.CriticalSourceWnd; while (hwndTemp != IntPtr.Zero) { if (hwnd == hwndTemp) { // Only when the parent window of the source of this TextStore is // moved or resized, we notfiy to Cicero. textstore.OnLayoutUpdated(); notified = true; break; } hwndTemp = UnsafeNativeMethods.GetParent(new HandleRef(this, hwndTemp)); } if (!notified) textstore.MakeLayoutChangeOnGotFocus(); } } } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties // Number of TextStores listening to this event. internal int TextStoreCount { get { return _arTextStore.Count; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // list of the registered TextStores. private ArrayList _arTextStore; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
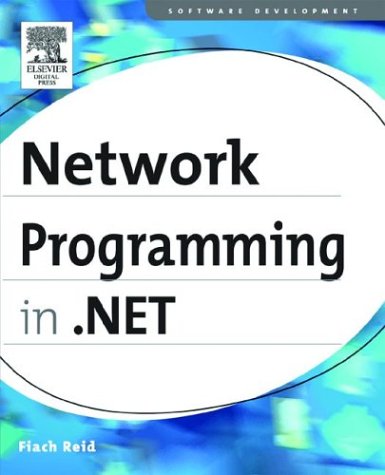
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlAttributes.cs
- PositiveTimeSpanValidator.cs
- HostProtectionPermission.cs
- SecureStringHasher.cs
- Permission.cs
- TraceHwndHost.cs
- DataGridRowClipboardEventArgs.cs
- infer.cs
- MenuItemStyleCollection.cs
- TextFormatterImp.cs
- ActiveDocumentEvent.cs
- OdbcEnvironment.cs
- MatchingStyle.cs
- EUCJPEncoding.cs
- PropertyConverter.cs
- ExtendedPropertyInfo.cs
- autovalidator.cs
- Privilege.cs
- BuildResult.cs
- SortAction.cs
- ActivityTypeDesigner.xaml.cs
- PersonalizationDictionary.cs
- FirstQueryOperator.cs
- SecurityContextTokenValidationException.cs
- PieceNameHelper.cs
- ClassGenerator.cs
- NonSerializedAttribute.cs
- HttpVersion.cs
- OletxCommittableTransaction.cs
- SrgsText.cs
- ObfuscationAttribute.cs
- EpmSourcePathSegment.cs
- ScrollData.cs
- Wildcard.cs
- BCLDebug.cs
- SafeNativeMethods.cs
- CodeCastExpression.cs
- Grid.cs
- ButtonColumn.cs
- InfoCardBaseException.cs
- WhitespaceRule.cs
- StylusPlugin.cs
- ExceptionUtil.cs
- JavaScriptObjectDeserializer.cs
- IRCollection.cs
- FixedFindEngine.cs
- MatcherBuilder.cs
- TrustManagerPromptUI.cs
- Debug.cs
- CalendarSelectionChangedEventArgs.cs
- webbrowsersite.cs
- Attributes.cs
- DBBindings.cs
- HttpServerUtilityWrapper.cs
- ColorKeyFrameCollection.cs
- SchemaName.cs
- CompilerGeneratedAttribute.cs
- TextParaClient.cs
- WebRequestModuleElement.cs
- FontUnitConverter.cs
- SharedPerformanceCounter.cs
- CallSite.cs
- HighlightVisual.cs
- CreateUserWizardStep.cs
- Logging.cs
- SmtpFailedRecipientsException.cs
- RegexGroup.cs
- ConnectionManagementSection.cs
- HttpListenerTimeoutManager.cs
- ExpressionReplacer.cs
- IteratorFilter.cs
- SizeF.cs
- ContextTokenTypeConverter.cs
- ValidatingPropertiesEventArgs.cs
- EmbeddedMailObject.cs
- Exceptions.cs
- PermissionRequestEvidence.cs
- CellQuery.cs
- ContentPlaceHolderDesigner.cs
- MultiView.cs
- FontStretchConverter.cs
- ListViewGroupCollectionEditor.cs
- xmlsaver.cs
- ObjectSet.cs
- DiscoveryEndpointValidator.cs
- DictionaryKeyPropertyAttribute.cs
- RequestBringIntoViewEventArgs.cs
- XmlCustomFormatter.cs
- Utilities.cs
- SqlCharStream.cs
- HtmlFormParameterWriter.cs
- CorrelationManager.cs
- DictionaryEntry.cs
- WebPartManagerInternals.cs
- DoWhile.cs
- ComEventsInfo.cs
- ConstraintStruct.cs
- SqlRowUpdatingEvent.cs
- EventLogTraceListener.cs
- ConfigDefinitionUpdates.cs