Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / Logging.cs / 1 / Logging.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.IO; using System.Threading; using System.Diagnostics; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Globalization; using Microsoft.Win32; internal class Logging { private static bool s_LoggingEnabled = true; private static bool s_LoggingInitialized; private static bool s_AppDomainShutdown; private const int DefaultMaxDumpSize = 1024; private const bool DefaultUseProtocolTextOnly = false; private const string AttributeNameMaxSize = "maxdatasize"; private const string AttributeNameTraceMode = "tracemode"; private static readonly string[] SupportedAttributes = new string[] { AttributeNameMaxSize, AttributeNameTraceMode }; private const string AttributeValueProtocolOnly = "protocolonly"; //private const string AttributeValueIncludeHex = "includehex"; private const string TraceSourceWebName = "System.Net"; private const string TraceSourceHttpListenerName = "System.Net.HttpListener"; private const string TraceSourceSocketsName = "System.Net.Sockets"; private const string TraceSourceCacheName = "System.Net.Cache"; private static TraceSource s_WebTraceSource; private static TraceSource s_HttpListenerTraceSource; private static TraceSource s_SocketsTraceSource; private static TraceSource s_CacheTraceSource; private Logging() { } private static object s_InternalSyncObject; private static object InternalSyncObject { get { if (s_InternalSyncObject == null) { object o = new Object(); Interlocked.CompareExchange(ref s_InternalSyncObject, o, null); } return s_InternalSyncObject; } } internal static bool On { get { if (!s_LoggingInitialized) { InitializeLogging(); } return s_LoggingEnabled; } } internal static bool IsVerbose(TraceSource traceSource) { return ValidateSettings(traceSource, TraceEventType.Verbose); } internal static TraceSource Web { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_WebTraceSource; } } internal static TraceSource HttpListener { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_HttpListenerTraceSource; } } internal static TraceSource Sockets { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_SocketsTraceSource; } } internal static TraceSource RequestCache { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_CacheTraceSource; } } private static bool GetUseProtocolTextSetting(TraceSource traceSource) { bool useProtocolTextOnly = DefaultUseProtocolTextOnly; if (traceSource.Attributes[AttributeNameTraceMode] == AttributeValueProtocolOnly) { useProtocolTextOnly = true; } return useProtocolTextOnly; } private static int GetMaxDumpSizeSetting(TraceSource traceSource) { int maxDumpSize = DefaultMaxDumpSize; if (traceSource.Attributes.ContainsKey(AttributeNameMaxSize)) { try { maxDumpSize = Int32.Parse(traceSource.Attributes[AttributeNameMaxSize], NumberFormatInfo.InvariantInfo); } catch (Exception exception) { if (exception is ThreadAbortException || exception is StackOverflowException || exception is OutOfMemoryException) { throw; } traceSource.Attributes[AttributeNameMaxSize] = maxDumpSize.ToString(NumberFormatInfo.InvariantInfo); } } return maxDumpSize; } ////// private static void InitializeLogging() { lock(InternalSyncObject) { if (!s_LoggingInitialized) { bool loggingEnabled = false; s_WebTraceSource = new NclTraceSource(TraceSourceWebName); s_HttpListenerTraceSource = new NclTraceSource(TraceSourceHttpListenerName); s_SocketsTraceSource = new NclTraceSource(TraceSourceSocketsName); s_CacheTraceSource = new NclTraceSource(TraceSourceCacheName); GlobalLog.Print("Initalizating tracing"); if (s_WebTraceSource.Switch.ShouldTrace(TraceEventType.Critical) || s_HttpListenerTraceSource.Switch.ShouldTrace(TraceEventType.Critical) || s_SocketsTraceSource.Switch.ShouldTrace(TraceEventType.Critical) || s_CacheTraceSource.Switch.ShouldTrace(TraceEventType.Critical)) { // if using Logging, then grab other logging switches loggingEnabled = true; AppDomain currentDomain = AppDomain.CurrentDomain; currentDomain.UnhandledException += new UnhandledExceptionEventHandler(UnhandledExceptionHandler); currentDomain.DomainUnload += new EventHandler(AppDomainUnloadEvent); currentDomain.ProcessExit += new EventHandler(ProcessExitEvent); } s_LoggingEnabled = loggingEnabled; s_LoggingInitialized = true; } } } private static void Close() { if (s_WebTraceSource != null) s_WebTraceSource.Close(); if (s_HttpListenerTraceSource != null) s_HttpListenerTraceSource.Close(); if (s_SocketsTraceSource != null) s_SocketsTraceSource.Close(); if (s_CacheTraceSource != null) s_CacheTraceSource.Close(); } ///Sets up internal config settings for logging. (MUST be called under critsec) ////// private static void UnhandledExceptionHandler(object sender, UnhandledExceptionEventArgs args) { Exception e = (Exception) args.ExceptionObject; Exception(Web, sender, "UnhandledExceptionHandler", e); } private static void ProcessExitEvent(object sender, EventArgs e) { Close(); s_AppDomainShutdown = true; } ///Logs any unhandled exception through this event handler ////// private static void AppDomainUnloadEvent(object sender, EventArgs e) { Close(); s_AppDomainShutdown = true; } ///Called when the system is shutting down, used to prevent additional logging post-shutdown ////// private static bool ValidateSettings(TraceSource traceSource, TraceEventType traceLevel) { if (!s_LoggingEnabled) { return false; } if (!s_LoggingInitialized) { InitializeLogging(); } if (traceSource == null || !traceSource.Switch.ShouldTrace(traceLevel)) { return false; } if (s_AppDomainShutdown) { return false; } return true; } ///Confirms logging is enabled, given current logging settings ////// private static string GetObjectName(object obj) { string objName = obj.ToString(); try { if (!(obj is Uri)) { int index = objName.LastIndexOf('.')+1; return objName.Substring(index, objName.Length-index); } else { return objName; } } catch (Exception exception) { if (exception is ThreadAbortException || exception is StackOverflowException || exception is OutOfMemoryException) { throw; } return objName; } } internal static uint GetThreadId() { uint threadId = UnsafeNclNativeMethods.GetCurrentThreadId(); if (threadId == 0) { threadId = (uint)Thread.CurrentThread.GetHashCode(); } return threadId; } internal static void PrintLine(TraceSource traceSource, TraceEventType eventType, int id, string msg) { string logHeader = "["+GetThreadId().ToString("d4", CultureInfo.InvariantCulture)+"] " ; traceSource.TraceEvent(eventType, id, logHeader + msg); } ///Converts an object to a normalized string that can be printed /// takes System.Net.ObjectNamedFoo and coverts to ObjectNamedFoo ////// internal static void Associate(TraceSource traceSource, object objA, object objB) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string lineA = GetObjectName(objA) + "#" + ValidationHelper.HashString(objA); string lineB = GetObjectName(objB) + "#" + ValidationHelper.HashString(objB); PrintLine(traceSource, TraceEventType.Information, 0, "Associating " + lineA + " with " + lineB); } ///Indicates that two objects are getting used with one another ////// internal static void Enter(TraceSource traceSource, object obj, string method, string param) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj), method, param); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, object obj, string method, object paramObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj), method, paramObject); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, string obj, string method, string param) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, obj +"::"+method+"("+param+")"); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, string obj, string method, object paramObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string paramObjectValue = ""; if (paramObject != null) { paramObjectValue = GetObjectName(paramObject) + "#" + ValidationHelper.HashString(paramObject); } Enter(traceSource, obj +"::"+method+"("+paramObjectValue+")"); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, string method, string parameters) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, method+"("+parameters+")"); } ///Logs entrance to a function, indents and points that out ////// internal static void Enter(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } // Trace.CorrelationManager.StartLogicalOperation(); PrintLine(traceSource, TraceEventType.Verbose, 0, msg); } ///Logs entrance to a function, indents and points that out ////// internal static void Exit(TraceSource traceSource, object obj, string method, object retObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string retValue = ""; if (retObject != null) { retValue = GetObjectName(retObject)+ "#" + ValidationHelper.HashString(retObject); } Exit(traceSource, obj, method, retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string obj, string method, object retObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string retValue = ""; if (retObject != null) { retValue = GetObjectName(retObject) + "#" + ValidationHelper.HashString(retObject); } Exit(traceSource, obj, method, retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, object obj, string method, string retValue) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Exit(traceSource, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj), method, retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string obj, string method, string retValue) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } if (!ValidationHelper.IsBlankString(retValue)) { retValue = "\t-> " + retValue; } Exit(traceSource, obj+"::"+method+"() "+retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string method, string parameters) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Exit(traceSource, method+"() "+parameters); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Verbose, 0, "Exiting "+msg); // Trace.CorrelationManager.StopLogicalOperation(); } ///Logs exit from a function ////// internal static void Exception(TraceSource traceSource, object obj, string method, Exception e) { if (!ValidateSettings(traceSource, TraceEventType.Error)) { return; } string infoLine = "Exception in the "+GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+" - "; //Trace.IndentLevel = 0; PrintLine(traceSource, TraceEventType.Error, 0, infoLine + e.Message); if (!ValidationHelper.IsBlankString(e.StackTrace)) { PrintLine(traceSource, TraceEventType.Error, 0, e.StackTrace); } /* if (e.InnerException != null) { PrintLine(" ---- inner exception --- "); PrintLine(traceSource, TraceEventType.Error, 0, infoLine + e.InnerException.Message); PrintLine(traceSource, TraceEventType.Error, 0, infoLine + e.InnerException.Message); if (!ValidationHelper.IsBlankString(e.InnerException.StackTrace)) { PrintLine(traceSource, TraceEventType.Error, 0, e.InnerException.StackTrace); } PrintLine(" ---- End of inner exception --- "); } */ } ///Logs Exception, restores indenting ////// internal static void PrintInfo(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Information, 0, msg); } ///Logs an Info line ////// internal static void PrintInfo(TraceSource traceSource, object obj, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Information, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +" - "+msg); } ///Logs an Info line ////// internal static void PrintInfo(TraceSource traceSource, object obj, string method, string param) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Information, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+"("+param+")"); } ///Logs an Info line ////// internal static void PrintWarning(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Warning)) { return; } PrintLine(traceSource, TraceEventType.Warning, 0, msg); } ///Logs a Warning line ////// internal static void PrintWarning(TraceSource traceSource, object obj, string method, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Warning)) { return; } PrintLine(traceSource, TraceEventType.Warning, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+"() - "+msg); } ///Logs a Warning line ////// internal static void PrintError(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Error)) { return; } PrintLine(traceSource, TraceEventType.Error, 0, msg); } ///Logs an Error line ////// internal static void PrintError(TraceSource traceSource, object obj, string method, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Error)) { return; } PrintLine(traceSource, TraceEventType.Error, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+"() - "+msg); } ///Logs an Error line ////// internal static void Dump(TraceSource traceSource, object obj, string method, IntPtr bufferPtr, int length) { if (!ValidateSettings(traceSource, TraceEventType.Verbose) || bufferPtr == IntPtr.Zero || length < 0) { return; } byte [] buffer = new byte[length]; Marshal.Copy(bufferPtr, buffer, 0, length); Dump(traceSource, obj, method, buffer, 0, length); } ///Marhsalls a buffer ptr to an array and then dumps the byte array to the log ////// internal static void Dump(TraceSource traceSource, object obj, string method, byte[] buffer, int offset, int length) { if (!ValidateSettings(traceSource, TraceEventType.Verbose)) { return; } if (buffer == null) { PrintLine(traceSource, TraceEventType.Verbose, 0, "(null)"); return; } if (offset > buffer.Length) { PrintLine(traceSource, TraceEventType.Verbose, 0, "(offset out of range)"); return; } PrintLine(traceSource, TraceEventType.Verbose, 0, "Data from "+GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method); int maxDumpSize = GetMaxDumpSizeSetting(traceSource); if (length > maxDumpSize) { PrintLine(traceSource, TraceEventType.Verbose, 0, "(printing " + maxDumpSize.ToString(NumberFormatInfo.InvariantInfo) + " out of " + length.ToString(NumberFormatInfo.InvariantInfo) + ")"); length = maxDumpSize; } if ((length < 0) || (length > buffer.Length - offset)) { length = buffer.Length - offset; } if (GetUseProtocolTextSetting(traceSource)) { string output = "<<" + WebHeaderCollection.HeaderEncoding.GetString(buffer, offset, length) + ">>"; PrintLine(traceSource, TraceEventType.Verbose, 0, output); return; } do { int n = Math.Min(length, 16); string disp = String.Format(CultureInfo.CurrentCulture, "{0:X8} : ", offset); for (int i = 0; i < n; ++i) { disp += String.Format(CultureInfo.CurrentCulture, "{0:X2}", buffer[offset + i]) + ((i == 7) ? '-' : ' '); } for (int i = n; i < 16; ++i) { disp += " "; } disp += ": "; for (int i = 0; i < n; ++i) { disp += ((buffer[offset + i] < 0x20) || (buffer[offset + i] > 0x7e)) ? '.' : (char)(buffer[offset + i]); } PrintLine(traceSource, TraceEventType.Verbose, 0, disp); offset += n; length -= n; } while (length > 0); } private class NclTraceSource : TraceSource { internal NclTraceSource(string name) : base(name) { } protected internal override string[] GetSupportedAttributes() { return Logging.SupportedAttributes; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Dumps a byte array to the log ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.IO; using System.Threading; using System.Diagnostics; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Globalization; using Microsoft.Win32; internal class Logging { private static bool s_LoggingEnabled = true; private static bool s_LoggingInitialized; private static bool s_AppDomainShutdown; private const int DefaultMaxDumpSize = 1024; private const bool DefaultUseProtocolTextOnly = false; private const string AttributeNameMaxSize = "maxdatasize"; private const string AttributeNameTraceMode = "tracemode"; private static readonly string[] SupportedAttributes = new string[] { AttributeNameMaxSize, AttributeNameTraceMode }; private const string AttributeValueProtocolOnly = "protocolonly"; //private const string AttributeValueIncludeHex = "includehex"; private const string TraceSourceWebName = "System.Net"; private const string TraceSourceHttpListenerName = "System.Net.HttpListener"; private const string TraceSourceSocketsName = "System.Net.Sockets"; private const string TraceSourceCacheName = "System.Net.Cache"; private static TraceSource s_WebTraceSource; private static TraceSource s_HttpListenerTraceSource; private static TraceSource s_SocketsTraceSource; private static TraceSource s_CacheTraceSource; private Logging() { } private static object s_InternalSyncObject; private static object InternalSyncObject { get { if (s_InternalSyncObject == null) { object o = new Object(); Interlocked.CompareExchange(ref s_InternalSyncObject, o, null); } return s_InternalSyncObject; } } internal static bool On { get { if (!s_LoggingInitialized) { InitializeLogging(); } return s_LoggingEnabled; } } internal static bool IsVerbose(TraceSource traceSource) { return ValidateSettings(traceSource, TraceEventType.Verbose); } internal static TraceSource Web { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_WebTraceSource; } } internal static TraceSource HttpListener { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_HttpListenerTraceSource; } } internal static TraceSource Sockets { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_SocketsTraceSource; } } internal static TraceSource RequestCache { get { if (!s_LoggingInitialized) { InitializeLogging(); } if (!s_LoggingEnabled) { return null; } return s_CacheTraceSource; } } private static bool GetUseProtocolTextSetting(TraceSource traceSource) { bool useProtocolTextOnly = DefaultUseProtocolTextOnly; if (traceSource.Attributes[AttributeNameTraceMode] == AttributeValueProtocolOnly) { useProtocolTextOnly = true; } return useProtocolTextOnly; } private static int GetMaxDumpSizeSetting(TraceSource traceSource) { int maxDumpSize = DefaultMaxDumpSize; if (traceSource.Attributes.ContainsKey(AttributeNameMaxSize)) { try { maxDumpSize = Int32.Parse(traceSource.Attributes[AttributeNameMaxSize], NumberFormatInfo.InvariantInfo); } catch (Exception exception) { if (exception is ThreadAbortException || exception is StackOverflowException || exception is OutOfMemoryException) { throw; } traceSource.Attributes[AttributeNameMaxSize] = maxDumpSize.ToString(NumberFormatInfo.InvariantInfo); } } return maxDumpSize; } ////// private static void InitializeLogging() { lock(InternalSyncObject) { if (!s_LoggingInitialized) { bool loggingEnabled = false; s_WebTraceSource = new NclTraceSource(TraceSourceWebName); s_HttpListenerTraceSource = new NclTraceSource(TraceSourceHttpListenerName); s_SocketsTraceSource = new NclTraceSource(TraceSourceSocketsName); s_CacheTraceSource = new NclTraceSource(TraceSourceCacheName); GlobalLog.Print("Initalizating tracing"); if (s_WebTraceSource.Switch.ShouldTrace(TraceEventType.Critical) || s_HttpListenerTraceSource.Switch.ShouldTrace(TraceEventType.Critical) || s_SocketsTraceSource.Switch.ShouldTrace(TraceEventType.Critical) || s_CacheTraceSource.Switch.ShouldTrace(TraceEventType.Critical)) { // if using Logging, then grab other logging switches loggingEnabled = true; AppDomain currentDomain = AppDomain.CurrentDomain; currentDomain.UnhandledException += new UnhandledExceptionEventHandler(UnhandledExceptionHandler); currentDomain.DomainUnload += new EventHandler(AppDomainUnloadEvent); currentDomain.ProcessExit += new EventHandler(ProcessExitEvent); } s_LoggingEnabled = loggingEnabled; s_LoggingInitialized = true; } } } private static void Close() { if (s_WebTraceSource != null) s_WebTraceSource.Close(); if (s_HttpListenerTraceSource != null) s_HttpListenerTraceSource.Close(); if (s_SocketsTraceSource != null) s_SocketsTraceSource.Close(); if (s_CacheTraceSource != null) s_CacheTraceSource.Close(); } ///Sets up internal config settings for logging. (MUST be called under critsec) ////// private static void UnhandledExceptionHandler(object sender, UnhandledExceptionEventArgs args) { Exception e = (Exception) args.ExceptionObject; Exception(Web, sender, "UnhandledExceptionHandler", e); } private static void ProcessExitEvent(object sender, EventArgs e) { Close(); s_AppDomainShutdown = true; } ///Logs any unhandled exception through this event handler ////// private static void AppDomainUnloadEvent(object sender, EventArgs e) { Close(); s_AppDomainShutdown = true; } ///Called when the system is shutting down, used to prevent additional logging post-shutdown ////// private static bool ValidateSettings(TraceSource traceSource, TraceEventType traceLevel) { if (!s_LoggingEnabled) { return false; } if (!s_LoggingInitialized) { InitializeLogging(); } if (traceSource == null || !traceSource.Switch.ShouldTrace(traceLevel)) { return false; } if (s_AppDomainShutdown) { return false; } return true; } ///Confirms logging is enabled, given current logging settings ////// private static string GetObjectName(object obj) { string objName = obj.ToString(); try { if (!(obj is Uri)) { int index = objName.LastIndexOf('.')+1; return objName.Substring(index, objName.Length-index); } else { return objName; } } catch (Exception exception) { if (exception is ThreadAbortException || exception is StackOverflowException || exception is OutOfMemoryException) { throw; } return objName; } } internal static uint GetThreadId() { uint threadId = UnsafeNclNativeMethods.GetCurrentThreadId(); if (threadId == 0) { threadId = (uint)Thread.CurrentThread.GetHashCode(); } return threadId; } internal static void PrintLine(TraceSource traceSource, TraceEventType eventType, int id, string msg) { string logHeader = "["+GetThreadId().ToString("d4", CultureInfo.InvariantCulture)+"] " ; traceSource.TraceEvent(eventType, id, logHeader + msg); } ///Converts an object to a normalized string that can be printed /// takes System.Net.ObjectNamedFoo and coverts to ObjectNamedFoo ////// internal static void Associate(TraceSource traceSource, object objA, object objB) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string lineA = GetObjectName(objA) + "#" + ValidationHelper.HashString(objA); string lineB = GetObjectName(objB) + "#" + ValidationHelper.HashString(objB); PrintLine(traceSource, TraceEventType.Information, 0, "Associating " + lineA + " with " + lineB); } ///Indicates that two objects are getting used with one another ////// internal static void Enter(TraceSource traceSource, object obj, string method, string param) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj), method, param); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, object obj, string method, object paramObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj), method, paramObject); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, string obj, string method, string param) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, obj +"::"+method+"("+param+")"); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, string obj, string method, object paramObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string paramObjectValue = ""; if (paramObject != null) { paramObjectValue = GetObjectName(paramObject) + "#" + ValidationHelper.HashString(paramObject); } Enter(traceSource, obj +"::"+method+"("+paramObjectValue+")"); } ///Logs entrance to a function ////// internal static void Enter(TraceSource traceSource, string method, string parameters) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Enter(traceSource, method+"("+parameters+")"); } ///Logs entrance to a function, indents and points that out ////// internal static void Enter(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } // Trace.CorrelationManager.StartLogicalOperation(); PrintLine(traceSource, TraceEventType.Verbose, 0, msg); } ///Logs entrance to a function, indents and points that out ////// internal static void Exit(TraceSource traceSource, object obj, string method, object retObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string retValue = ""; if (retObject != null) { retValue = GetObjectName(retObject)+ "#" + ValidationHelper.HashString(retObject); } Exit(traceSource, obj, method, retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string obj, string method, object retObject) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } string retValue = ""; if (retObject != null) { retValue = GetObjectName(retObject) + "#" + ValidationHelper.HashString(retObject); } Exit(traceSource, obj, method, retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, object obj, string method, string retValue) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Exit(traceSource, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj), method, retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string obj, string method, string retValue) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } if (!ValidationHelper.IsBlankString(retValue)) { retValue = "\t-> " + retValue; } Exit(traceSource, obj+"::"+method+"() "+retValue); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string method, string parameters) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } Exit(traceSource, method+"() "+parameters); } ///Logs exit from a function ////// internal static void Exit(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Verbose, 0, "Exiting "+msg); // Trace.CorrelationManager.StopLogicalOperation(); } ///Logs exit from a function ////// internal static void Exception(TraceSource traceSource, object obj, string method, Exception e) { if (!ValidateSettings(traceSource, TraceEventType.Error)) { return; } string infoLine = "Exception in the "+GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+" - "; //Trace.IndentLevel = 0; PrintLine(traceSource, TraceEventType.Error, 0, infoLine + e.Message); if (!ValidationHelper.IsBlankString(e.StackTrace)) { PrintLine(traceSource, TraceEventType.Error, 0, e.StackTrace); } /* if (e.InnerException != null) { PrintLine(" ---- inner exception --- "); PrintLine(traceSource, TraceEventType.Error, 0, infoLine + e.InnerException.Message); PrintLine(traceSource, TraceEventType.Error, 0, infoLine + e.InnerException.Message); if (!ValidationHelper.IsBlankString(e.InnerException.StackTrace)) { PrintLine(traceSource, TraceEventType.Error, 0, e.InnerException.StackTrace); } PrintLine(" ---- End of inner exception --- "); } */ } ///Logs Exception, restores indenting ////// internal static void PrintInfo(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Information, 0, msg); } ///Logs an Info line ////// internal static void PrintInfo(TraceSource traceSource, object obj, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Information, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +" - "+msg); } ///Logs an Info line ////// internal static void PrintInfo(TraceSource traceSource, object obj, string method, string param) { if (!ValidateSettings(traceSource, TraceEventType.Information)) { return; } PrintLine(traceSource, TraceEventType.Information, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+"("+param+")"); } ///Logs an Info line ////// internal static void PrintWarning(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Warning)) { return; } PrintLine(traceSource, TraceEventType.Warning, 0, msg); } ///Logs a Warning line ////// internal static void PrintWarning(TraceSource traceSource, object obj, string method, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Warning)) { return; } PrintLine(traceSource, TraceEventType.Warning, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+"() - "+msg); } ///Logs a Warning line ////// internal static void PrintError(TraceSource traceSource, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Error)) { return; } PrintLine(traceSource, TraceEventType.Error, 0, msg); } ///Logs an Error line ////// internal static void PrintError(TraceSource traceSource, object obj, string method, string msg) { if (!ValidateSettings(traceSource, TraceEventType.Error)) { return; } PrintLine(traceSource, TraceEventType.Error, 0, GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method+"() - "+msg); } ///Logs an Error line ////// internal static void Dump(TraceSource traceSource, object obj, string method, IntPtr bufferPtr, int length) { if (!ValidateSettings(traceSource, TraceEventType.Verbose) || bufferPtr == IntPtr.Zero || length < 0) { return; } byte [] buffer = new byte[length]; Marshal.Copy(bufferPtr, buffer, 0, length); Dump(traceSource, obj, method, buffer, 0, length); } ///Marhsalls a buffer ptr to an array and then dumps the byte array to the log ////// internal static void Dump(TraceSource traceSource, object obj, string method, byte[] buffer, int offset, int length) { if (!ValidateSettings(traceSource, TraceEventType.Verbose)) { return; } if (buffer == null) { PrintLine(traceSource, TraceEventType.Verbose, 0, "(null)"); return; } if (offset > buffer.Length) { PrintLine(traceSource, TraceEventType.Verbose, 0, "(offset out of range)"); return; } PrintLine(traceSource, TraceEventType.Verbose, 0, "Data from "+GetObjectName(obj)+"#"+ValidationHelper.HashString(obj) +"::"+method); int maxDumpSize = GetMaxDumpSizeSetting(traceSource); if (length > maxDumpSize) { PrintLine(traceSource, TraceEventType.Verbose, 0, "(printing " + maxDumpSize.ToString(NumberFormatInfo.InvariantInfo) + " out of " + length.ToString(NumberFormatInfo.InvariantInfo) + ")"); length = maxDumpSize; } if ((length < 0) || (length > buffer.Length - offset)) { length = buffer.Length - offset; } if (GetUseProtocolTextSetting(traceSource)) { string output = "<<" + WebHeaderCollection.HeaderEncoding.GetString(buffer, offset, length) + ">>"; PrintLine(traceSource, TraceEventType.Verbose, 0, output); return; } do { int n = Math.Min(length, 16); string disp = String.Format(CultureInfo.CurrentCulture, "{0:X8} : ", offset); for (int i = 0; i < n; ++i) { disp += String.Format(CultureInfo.CurrentCulture, "{0:X2}", buffer[offset + i]) + ((i == 7) ? '-' : ' '); } for (int i = n; i < 16; ++i) { disp += " "; } disp += ": "; for (int i = 0; i < n; ++i) { disp += ((buffer[offset + i] < 0x20) || (buffer[offset + i] > 0x7e)) ? '.' : (char)(buffer[offset + i]); } PrintLine(traceSource, TraceEventType.Verbose, 0, disp); offset += n; length -= n; } while (length > 0); } private class NclTraceSource : TraceSource { internal NclTraceSource(string name) : base(name) { } protected internal override string[] GetSupportedAttributes() { return Logging.SupportedAttributes; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Dumps a byte array to the log ///
Link Menu
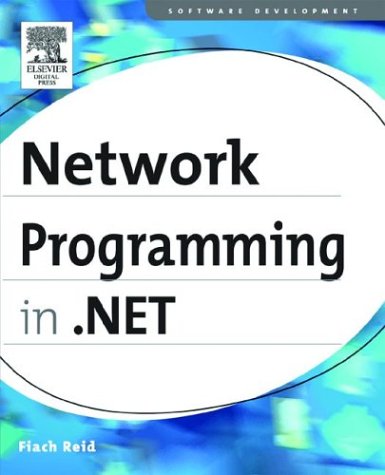
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringExpressionSet.cs
- VideoDrawing.cs
- TimeIntervalCollection.cs
- CustomError.cs
- DesignerRegion.cs
- TextProperties.cs
- ServiceAuthorizationElement.cs
- RoutedUICommand.cs
- PowerModeChangedEventArgs.cs
- HebrewCalendar.cs
- ValueTypeFixupInfo.cs
- MetadataSource.cs
- InvokeWebService.cs
- GcHandle.cs
- SimpleLine.cs
- RadioButtonRenderer.cs
- DbConnectionPool.cs
- XmlSchema.cs
- LocationFactory.cs
- AlphabetConverter.cs
- XmlMembersMapping.cs
- ToolStripContainerDesigner.cs
- EdgeProfileValidation.cs
- DrawListViewSubItemEventArgs.cs
- ModelProperty.cs
- ImageCodecInfoPrivate.cs
- HandlerBase.cs
- RemotingException.cs
- XamlDesignerSerializationManager.cs
- FormsAuthenticationConfiguration.cs
- JapaneseLunisolarCalendar.cs
- ThemeConfigurationDialog.cs
- behaviorssection.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- DbDataReader.cs
- ServiceDebugElement.cs
- MexTcpBindingCollectionElement.cs
- LocatorPart.cs
- ProxyWebPart.cs
- SiteMapHierarchicalDataSourceView.cs
- DataListItemCollection.cs
- InternalConfigRoot.cs
- PathData.cs
- WebPartMinimizeVerb.cs
- MdiWindowListItemConverter.cs
- XmlCountingReader.cs
- TextBoxLine.cs
- HtmlInputCheckBox.cs
- XslVisitor.cs
- XmlEntity.cs
- SystemInformation.cs
- SqlServer2KCompatibilityCheck.cs
- GeneralTransform2DTo3DTo2D.cs
- SqlProviderServices.cs
- safelinkcollection.cs
- TrackBarRenderer.cs
- DeviceContext2.cs
- StyleHelper.cs
- SqlFacetAttribute.cs
- ScriptingJsonSerializationSection.cs
- MessageContractExporter.cs
- Clock.cs
- MetadataArtifactLoaderCompositeResource.cs
- Repeater.cs
- VisualStyleElement.cs
- FileFormatException.cs
- DataGridViewAdvancedBorderStyle.cs
- MetadataCache.cs
- ApplicationProxyInternal.cs
- AlgoModule.cs
- Image.cs
- FormatterServicesNoSerializableCheck.cs
- XmlEncoding.cs
- ParseElement.cs
- KeysConverter.cs
- Win32KeyboardDevice.cs
- DataGridViewTopRowAccessibleObject.cs
- AutomationProperty.cs
- TabControl.cs
- CheckBoxFlatAdapter.cs
- XmlSchemaAnnotation.cs
- RuleSettings.cs
- DbMetaDataCollectionNames.cs
- SymmetricKeyWrap.cs
- DesignerActionUI.cs
- DoubleAnimation.cs
- FilterableAttribute.cs
- XmlSerializationReader.cs
- LazyTextWriterCreator.cs
- TableFieldsEditor.cs
- DefaultAsyncDataDispatcher.cs
- ValueSerializerAttribute.cs
- XPathMultyIterator.cs
- VirtualDirectoryMapping.cs
- MDIControlStrip.cs
- Avt.cs
- DateRangeEvent.cs
- DefaultValidator.cs
- DataControlFieldHeaderCell.cs
- DesignerRegionMouseEventArgs.cs