Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Objects / ELinq / Visitors / LinqMaximalSubtreeNominator.cs / 3 / LinqMaximalSubtreeNominator.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Linq.Expressions; using System.Collections.Generic; using System.Diagnostics; namespace System.Data.Objects.ELinq { ////// Goes from the bottom to top and nominates nodes where all the nodes /// below the node return true from the shouldBeNominatedDelegate /// internal sealed class LinqMaximalSubtreeNominator : ExpressionVisitor { readonly HashSet_candidates; readonly Func _shouldBeNominatedDelegate; bool _cannotBeNominated = false; // not creatable private LinqMaximalSubtreeNominator(HashSet candidates, Func shouldBeNominatedDelegate) { _candidates = candidates; _shouldBeNominatedDelegate = shouldBeNominatedDelegate; } internal static HashSet Nominate(Expression expression, HashSet candidates, Func shouldBeNominatedDelegate) { Debug.Assert(candidates != null, "Candidates hashset cannot be null"); LinqMaximalSubtreeNominator nominator = new LinqMaximalSubtreeNominator(candidates, shouldBeNominatedDelegate); nominator.Visit(expression); return nominator._candidates; } internal static HashSet FindMaximalSubtrees(Expression expression, Func shouldBeNominatedDelegate) { HashSet nominees = Nominate(expression, new HashSet (), shouldBeNominatedDelegate); return MaximalSubtreeVisitor.FindMaximalSubtrees(nominees, expression); } internal override Expression Visit(Expression exp) { if (exp != null) { bool saveCannotBeNominated = _cannotBeNominated; _cannotBeNominated = false; base.Visit(exp); if (!_cannotBeNominated) { // everyone below me can be nominated, so // see if this one can be also if (_shouldBeNominatedDelegate(exp)) { _candidates.Add(exp); } else { _cannotBeNominated = true; } } _cannotBeNominated |= saveCannotBeNominated; } return exp; } /// /// Visitor that identifies maximal subtrees given a set of nominees. It walks the tree top down /// and when it identifies a nominated node, adds it to the _subtree set and stops walking. /// private sealed class MaximalSubtreeVisitor : ExpressionVisitor { private readonly HashSet_subtrees; private readonly HashSet _nominees; private MaximalSubtreeVisitor(HashSet nominees) { _nominees = nominees; _subtrees = new HashSet (); } internal static HashSet FindMaximalSubtrees(HashSet nominees, Expression query) { MaximalSubtreeVisitor visitor = new MaximalSubtreeVisitor(nominees); visitor.Visit(query); return visitor._subtrees; } internal override Expression Visit(Expression exp) { if (exp != null && _nominees.Contains(exp)) { _subtrees.Add(exp); return exp; } return base.Visit(exp); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Linq.Expressions; using System.Collections.Generic; using System.Diagnostics; namespace System.Data.Objects.ELinq { ////// Goes from the bottom to top and nominates nodes where all the nodes /// below the node return true from the shouldBeNominatedDelegate /// internal sealed class LinqMaximalSubtreeNominator : ExpressionVisitor { readonly HashSet_candidates; readonly Func _shouldBeNominatedDelegate; bool _cannotBeNominated = false; // not creatable private LinqMaximalSubtreeNominator(HashSet candidates, Func shouldBeNominatedDelegate) { _candidates = candidates; _shouldBeNominatedDelegate = shouldBeNominatedDelegate; } internal static HashSet Nominate(Expression expression, HashSet candidates, Func shouldBeNominatedDelegate) { Debug.Assert(candidates != null, "Candidates hashset cannot be null"); LinqMaximalSubtreeNominator nominator = new LinqMaximalSubtreeNominator(candidates, shouldBeNominatedDelegate); nominator.Visit(expression); return nominator._candidates; } internal static HashSet FindMaximalSubtrees(Expression expression, Func shouldBeNominatedDelegate) { HashSet nominees = Nominate(expression, new HashSet (), shouldBeNominatedDelegate); return MaximalSubtreeVisitor.FindMaximalSubtrees(nominees, expression); } internal override Expression Visit(Expression exp) { if (exp != null) { bool saveCannotBeNominated = _cannotBeNominated; _cannotBeNominated = false; base.Visit(exp); if (!_cannotBeNominated) { // everyone below me can be nominated, so // see if this one can be also if (_shouldBeNominatedDelegate(exp)) { _candidates.Add(exp); } else { _cannotBeNominated = true; } } _cannotBeNominated |= saveCannotBeNominated; } return exp; } /// /// Visitor that identifies maximal subtrees given a set of nominees. It walks the tree top down /// and when it identifies a nominated node, adds it to the _subtree set and stops walking. /// private sealed class MaximalSubtreeVisitor : ExpressionVisitor { private readonly HashSet_subtrees; private readonly HashSet _nominees; private MaximalSubtreeVisitor(HashSet nominees) { _nominees = nominees; _subtrees = new HashSet (); } internal static HashSet FindMaximalSubtrees(HashSet nominees, Expression query) { MaximalSubtreeVisitor visitor = new MaximalSubtreeVisitor(nominees); visitor.Visit(query); return visitor._subtrees; } internal override Expression Visit(Expression exp) { if (exp != null && _nominees.Contains(exp)) { _subtrees.Add(exp); return exp; } return base.Visit(exp); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
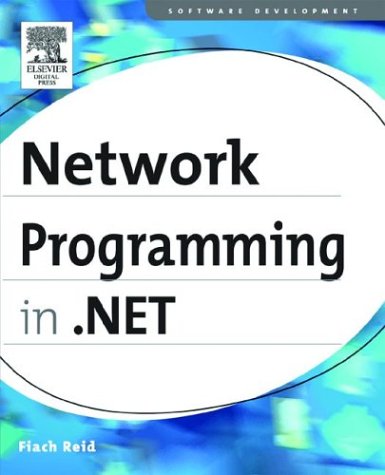
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ModelPerspective.cs
- NavigationPropertyEmitter.cs
- CodeArrayCreateExpression.cs
- ClientConfigurationHost.cs
- SafeFileMapViewHandle.cs
- WebPartConnectionsEventArgs.cs
- OracleBFile.cs
- TextTreeFixupNode.cs
- RequiredFieldValidator.cs
- NativeMethods.cs
- CqlErrorHelper.cs
- typedescriptorpermission.cs
- PropertyValueEditor.cs
- Bits.cs
- StorageInfo.cs
- Suspend.cs
- X509ChainPolicy.cs
- ServiceOperationWrapper.cs
- GifBitmapEncoder.cs
- ImageCodecInfoPrivate.cs
- NodeInfo.cs
- PrimitiveType.cs
- ExeConfigurationFileMap.cs
- FormView.cs
- DurationConverter.cs
- OleDbPropertySetGuid.cs
- assemblycache.cs
- ChildTable.cs
- _SafeNetHandles.cs
- ASCIIEncoding.cs
- _Semaphore.cs
- Pen.cs
- ControlType.cs
- NetCodeGroup.cs
- BCryptSafeHandles.cs
- SchemaSetCompiler.cs
- HashHelpers.cs
- HorizontalAlignConverter.cs
- DebugView.cs
- ListViewHitTestInfo.cs
- BrowserDefinition.cs
- TextCompositionEventArgs.cs
- OdbcEnvironmentHandle.cs
- CheckBoxStandardAdapter.cs
- PriorityChain.cs
- InheritablePropertyChangeInfo.cs
- HandlerBase.cs
- FormsAuthenticationUser.cs
- DynamicMethod.cs
- DetailsViewDeletedEventArgs.cs
- MetadataWorkspace.cs
- TraceHandler.cs
- CharEnumerator.cs
- HttpHandler.cs
- ToolBar.cs
- XmlSignatureProperties.cs
- WindowsTab.cs
- QilFactory.cs
- embossbitmapeffect.cs
- GridViewAutomationPeer.cs
- PropertiesTab.cs
- ListManagerBindingsCollection.cs
- XMLDiffLoader.cs
- namescope.cs
- SoundPlayerAction.cs
- ThreadSafeList.cs
- BaseParaClient.cs
- WebPartDescriptionCollection.cs
- PerformanceCounterManager.cs
- TracePayload.cs
- LinkUtilities.cs
- DecoderExceptionFallback.cs
- LoginStatusDesigner.cs
- MessageEventSubscriptionService.cs
- Utils.cs
- SetterBase.cs
- ServiceRouteHandler.cs
- DefaultEventAttribute.cs
- Effect.cs
- DataGrid.cs
- KeysConverter.cs
- BufferCache.cs
- FrameworkObject.cs
- DependencyPropertyChangedEventArgs.cs
- GroupBoxAutomationPeer.cs
- LongCountAggregationOperator.cs
- HandleCollector.cs
- ConfigDefinitionUpdates.cs
- StorageFunctionMapping.cs
- DataGridViewColumnHeaderCell.cs
- SelectionWordBreaker.cs
- CultureMapper.cs
- ResponseBodyWriter.cs
- SafeHandles.cs
- StateManagedCollection.cs
- DataGridViewColumnHeaderCell.cs
- SafeHandle.cs
- AttachedAnnotation.cs
- _NegoState.cs
- SystemWebExtensionsSectionGroup.cs