Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / System.ServiceModel.Activation / System / ServiceModel / Activation / ServiceRouteHandler.cs / 1305376 / ServiceRouteHandler.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Linq; using System.Runtime; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Diagnostics; using System.ServiceModel.Diagnostics.Application; using System.Web; using System.Web.Hosting; using System.Web.Routing; class ServiceRouteHandler : IRouteHandler { string baseAddress; IHttpHandler handler; object locker = new object(); [Fx.Tag.Cache( typeof(ServiceDeploymentInfo), Fx.Tag.CacheAttrition.None, Scope = "instance of declaring class", SizeLimit = "unbounded", Timeout = "infinite" )] static Hashtable routeServiceTable = new Hashtable(StringComparer.CurrentCultureIgnoreCase); public ServiceRouteHandler(string baseAddress, ServiceHostFactoryBase serviceHostFactory, Type webServiceType) { this.baseAddress = string.Format(CultureInfo.CurrentCulture, "~/{0}", baseAddress); if (webServiceType == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("webServiceType")); } if (serviceHostFactory == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("serviceHostFactory")); } string serviceType = webServiceType.AssemblyQualifiedName; AddServiceInfo(this.baseAddress, new ServiceDeploymentInfo(this.baseAddress, serviceHostFactory, serviceType)); } public IHttpHandler GetHttpHandler(RequestContext requestContext) { // we create httphandler only we the request map to the corresponding route. // we thus do not need to check whether the baseAddress has been added // even though Asp.Net allows duplicated routes but it picks the first match if (handler == null) { // use local lock to prevent multiple httphanders from being created lock (locker) { if (handler == null) { handler = new AspNetRouteServiceHttpHandler(this.baseAddress); MarkRouteAsActive(this.baseAddress); } } } return handler; } static void AddServiceInfo(string virtualPath, ServiceDeploymentInfo serviceInfo) { Fx.Assert(!string.IsNullOrEmpty(virtualPath), "virtualPath should not be empty or null"); Fx.Assert(serviceInfo != null, "serviceInfo should not be null"); // We cannot support dulicated route routes even Asp.Net route allows it try { routeServiceTable.Add(virtualPath, serviceInfo); } catch (ArgumentException) { throw FxTrace.Exception.Argument("virtualPath", SR.Hosting_RouteHasAlreadyBeenAdded(virtualPath)); } } public static ServiceDeploymentInfo GetServiceInfo(string normalizedVirtualPath) { return (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; } public static bool IsActiveAspNetRoute(string virtualPath) { bool isRouteService = false; if (!string.IsNullOrEmpty(virtualPath)) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[virtualPath]; if (serviceInfo != null) { isRouteService = serviceInfo.MessageHandledByRoute; } } return isRouteService; } // A route in routetable does not always mean the route will be picked // we update IsRouteService only when Asp.Net picks this route public static void MarkRouteAsActive(string normalizedVirtualPath) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; if (serviceInfo != null) { serviceInfo.MessageHandledByRoute = true; } } // a route should be marked as inactive in the case that CBA should be used public static void MarkARouteAsInactive(string normalizedVirtualPath) { ServiceDeploymentInfo serviceInfo = (ServiceDeploymentInfo)routeServiceTable[normalizedVirtualPath]; if (serviceInfo != null) { serviceInfo.MessageHandledByRoute = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
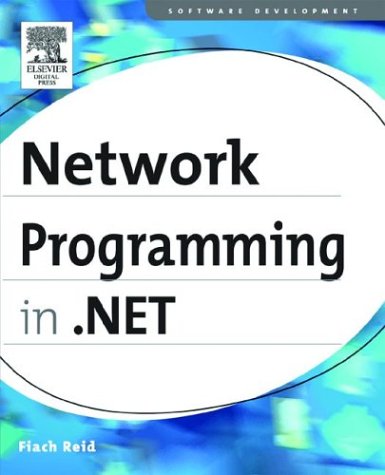
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MdiWindowListItemConverter.cs
- XmlDocument.cs
- HtmlUtf8RawTextWriter.cs
- TreeNodeBindingCollection.cs
- CodeVariableReferenceExpression.cs
- DependencySource.cs
- DocumentScope.cs
- EqualityComparer.cs
- XPathEmptyIterator.cs
- WindowsListViewSubItem.cs
- DataGridViewCellFormattingEventArgs.cs
- MessagingDescriptionAttribute.cs
- GenericEnumerator.cs
- ResourceDisplayNameAttribute.cs
- EventlogProvider.cs
- Decoder.cs
- MembershipSection.cs
- RectConverter.cs
- AssemblyBuilderData.cs
- Stack.cs
- StylusCollection.cs
- Win32Native.cs
- ConfigurationElement.cs
- EditingCommands.cs
- StylusPointPropertyInfo.cs
- ControlDesigner.cs
- ClientSponsor.cs
- SrgsRule.cs
- FontNamesConverter.cs
- OdbcReferenceCollection.cs
- DataGridViewTextBoxEditingControl.cs
- StrongTypingException.cs
- EnumerableRowCollection.cs
- Vector3DCollectionValueSerializer.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- EntityWrapper.cs
- GradientStop.cs
- DPCustomTypeDescriptor.cs
- QilBinary.cs
- SecurityException.cs
- JsonFormatReaderGenerator.cs
- GenericAuthenticationEventArgs.cs
- ConfigXmlWhitespace.cs
- HtmlButton.cs
- ISFTagAndGuidCache.cs
- HttpCookiesSection.cs
- MemberInfoSerializationHolder.cs
- QueryExecutionOption.cs
- FormViewModeEventArgs.cs
- DockPanel.cs
- BindingNavigator.cs
- InstanceDataCollection.cs
- DetailsViewDeletedEventArgs.cs
- TextRunProperties.cs
- DelimitedListTraceListener.cs
- TdsParserSessionPool.cs
- TextDecoration.cs
- RadialGradientBrush.cs
- ProcessHostServerConfig.cs
- MobileRedirect.cs
- DBPropSet.cs
- DisplayMemberTemplateSelector.cs
- ScrollEvent.cs
- DataKeyArray.cs
- UriSection.cs
- ISFTagAndGuidCache.cs
- ComIntegrationManifestGenerator.cs
- TableCellCollection.cs
- SqlTransaction.cs
- QilScopedVisitor.cs
- DefaultPrintController.cs
- EntityRecordInfo.cs
- SecurityContextKeyIdentifierClause.cs
- CallContext.cs
- ICollection.cs
- Transform3D.cs
- oledbmetadatacolumnnames.cs
- SqlMethods.cs
- TCPListener.cs
- HttpRuntimeSection.cs
- RijndaelManaged.cs
- ListItemCollection.cs
- CellQuery.cs
- WinEventWrap.cs
- PenThreadPool.cs
- precedingquery.cs
- LastQueryOperator.cs
- PasswordBox.cs
- HandleCollector.cs
- WindowsHyperlink.cs
- XamlFilter.cs
- QueryInterceptorAttribute.cs
- DoubleCollectionValueSerializer.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- BitmapDownload.cs
- RestHandler.cs
- UrlUtility.cs
- FormCollection.cs
- StatusBarItem.cs
- SpellerError.cs