Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / EditingCommands.cs / 1305600 / EditingCommands.cs
//---------------------------------------------------------------------------- // // File: EditingCommands.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Command definitions for Rich Text Editing. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System.Windows.Input; // Command using System.ComponentModel; // TypeConverter ////// Command definitions for Rich Text Editing. /// public static class EditingCommands { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties // Typing Commands // --------------- ////// ToggleInsert command. /// Changed typing mode between insertion and overtyping. /// public static RoutedUICommand ToggleInsert { get { return EnsureCommand(ref _ToggleInsert , "ToggleInsert" ); } } ////// Delete command. /// When selection is empty deletes the following character or paragraph separator. /// When selection is not empty deletes the selected content. /// Formatting of deleted content is not springloaded (unlike Backspace). /// public static RoutedUICommand Delete { get { return EnsureCommand(ref _Delete , "Delete" ); } } ////// Backspace command. /// When selection is empty deleted the previous character or paragraph separator. /// When selection is not empty deletes the selected content. /// Formatting of deleted content is springloaded (unlike Delete). /// Formatting for springloading is taken from the very first selected character. /// public static RoutedUICommand Backspace { get { return EnsureCommand(ref _Backspace , "Backspace" ); } } ////// DeleteNextWord command. /// public static RoutedUICommand DeleteNextWord { get { return EnsureCommand(ref _DeleteNextWord , "DeleteNextWord" ); } } ////// DeletePreviousWord command. /// public static RoutedUICommand DeletePreviousWord { get { return EnsureCommand(ref _DeletePreviousWord , "DeletePreviousWord" ); } } ////// EnterParagraphBreak command. /// Acts as if the user presses Enter key. The content of current selection is deleted (if not empty) /// like with Backspace command(performing formatting springloading), and then /// the structure of text elements is changed so that paragraph break appears /// at caret position. /// public static RoutedUICommand EnterParagraphBreak { get { return EnsureCommand(ref _EnterParagraphBreak , "EnterParagraphBreak" ); } } ////// EnterLineBreak command. /// public static RoutedUICommand EnterLineBreak { get { return EnsureCommand(ref _EnterLineBreak , "EnterLineBreak" ); } } ////// TabForward command. /// The behavior depends from the current condition of selection. /// If selection is non-empty then it redirects to a IncreaseIndentation command, /// so that all affected paragraphs become promoted (by increasing their Margin.Left property in RichTextBox /// or by inserting additional Tab charater in the beginning of each non-wrapped line). /// If the caret is in table cell then it moves to the next cell. /// If the caret is in the last table cell, then in creates new row in a table and moves /// the caret into first cell of that row. /// Otherwise Tab character is inserted in current position. /// public static RoutedUICommand TabForward { get { return EnsureCommand(ref _TabForward , "TabForward" ); } } ////// TabBackward command. /// The behavior depends from the current condition of selection. /// If selection is non-empty then it redirects to a DecreaseIndentation command, /// so that all affected paragraphs become promoted (by decreasing their Margin.Left property in RichTextBox /// or by deleting a Tab charater in the beginning of each non-wrapped line). /// If the caret is in table cell then it moves to the previous cell. /// Otherwise Tab character is inserted in current position. /// public static RoutedUICommand TabBackward { get { return EnsureCommand(ref _TabBackward , "TabBackward" ); } } // Caret navigation commands // ------------------------- ////// MoveRightByCharacter command. /// public static RoutedUICommand MoveRightByCharacter { get { return EnsureCommand(ref _MoveRightByCharacter , "MoveRightByCharacter" ); } } ////// MoveLeftByCharacter command. /// public static RoutedUICommand MoveLeftByCharacter { get { return EnsureCommand(ref _MoveLeftByCharacter , "MoveLeftByCharacter" ); } } ////// MoveRightByWord command. /// public static RoutedUICommand MoveRightByWord { get { return EnsureCommand(ref _MoveRightByWord , "MoveRightByWord" ); } } ////// MoveLeftByWord command. /// public static RoutedUICommand MoveLeftByWord { get { return EnsureCommand(ref _MoveLeftByWord , "MoveLeftByWord" ); } } ////// MoveDownByLine command. /// public static RoutedUICommand MoveDownByLine { get { return EnsureCommand(ref _MoveDownByLine , "MoveDownByLine" ); } } ////// MoveUpByLine command. /// public static RoutedUICommand MoveUpByLine { get { return EnsureCommand(ref _MoveUpByLine , "MoveUpByLine" ); } } ////// MoveDownByParagraph command. /// public static RoutedUICommand MoveDownByParagraph { get { return EnsureCommand(ref _MoveDownByParagraph , "MoveDownByParagraph" ); } } ////// MoveUpByParagraph command. /// public static RoutedUICommand MoveUpByParagraph { get { return EnsureCommand(ref _MoveUpByParagraph , "MoveUpByParagraph" ); } } ////// MoveDownByPage command. /// Corresponds to PgDn key on the keyboard. /// public static RoutedUICommand MoveDownByPage { get { return EnsureCommand(ref _MoveDownByPage , "MoveDownByPage" ); } } ////// MoveUpByPage command. /// Corresponds to PgUp key on the keyboard. /// public static RoutedUICommand MoveUpByPage { get { return EnsureCommand(ref _MoveUpByPage , "MoveUpByPage" ); } } ////// MoveToLineStart command. /// Corresponds to Home key on the keyboard. /// public static RoutedUICommand MoveToLineStart { get { return EnsureCommand(ref _MoveToLineStart , "MoveToLineStart" ); } } ////// MoveToLineEnd command. /// Corresponds to End key on the keyboard. /// public static RoutedUICommand MoveToLineEnd { get { return EnsureCommand(ref _MoveToLineEnd , "MoveToLineEnd" ); } } ////// MoveToDocumentStart command. /// public static RoutedUICommand MoveToDocumentStart { get { return EnsureCommand(ref _MoveToDocumentStart , "MoveToDocumentStart" ); } } ////// MoveToDocumentEnd command. /// public static RoutedUICommand MoveToDocumentEnd { get { return EnsureCommand(ref _MoveToDocumentEnd , "MoveToDocumentEnd" ); } } // Selection extension commands // ---------------------------- ////// SelectRightByCharacter command. /// public static RoutedUICommand SelectRightByCharacter { get { return EnsureCommand(ref _SelectRightByCharacter , "SelectRightByCharacter" ); } } ////// SelectLeftByCharacter command. /// public static RoutedUICommand SelectLeftByCharacter { get { return EnsureCommand(ref _SelectLeftByCharacter , "SelectLeftByCharacter" ); } } ////// SelectRightByWord command. /// public static RoutedUICommand SelectRightByWord { get { return EnsureCommand(ref _SelectRightByWord , "SelectRightByWord" ); } } ////// SelectLeftbyWord command. /// public static RoutedUICommand SelectLeftByWord { get { return EnsureCommand(ref _SelectLeftByWord , "SelectLeftByWord" ); } } ////// SelectDownByLine command. /// public static RoutedUICommand SelectDownByLine { get { return EnsureCommand(ref _SelectDownByLine , "SelectDownByLine" ); } } ////// SelectUpByLine command. /// public static RoutedUICommand SelectUpByLine { get { return EnsureCommand(ref _SelectUpByLine , "SelectUpByLine" ); } } ////// SelectDownByParagraph command. /// public static RoutedUICommand SelectDownByParagraph { get { return EnsureCommand(ref _SelectDownByParagraph , "SelectDownByParagraph" ); } } ////// SelectUpByParagraph command. /// public static RoutedUICommand SelectUpByParagraph { get { return EnsureCommand(ref _SelectUpByParagraph , "SelectUpByParagraph" ); } } ////// SelectDownByPage command. /// public static RoutedUICommand SelectDownByPage { get { return EnsureCommand(ref _SelectDownByPage , "SelectDownByPage" ); } } ////// SelectUpByPage command. /// public static RoutedUICommand SelectUpByPage { get { return EnsureCommand(ref _SelectUpByPage , "SelectUpByPage" ); } } ////// SelectToLineStart command. /// public static RoutedUICommand SelectToLineStart { get { return EnsureCommand(ref _SelectToLineStart , "SelectToLineStart" ); } } ////// SelectToLineEnd command. /// public static RoutedUICommand SelectToLineEnd { get { return EnsureCommand(ref _SelectToLineEnd , "SelectToLineEnd" ); } } ////// SelectToDocumentStart command. /// public static RoutedUICommand SelectToDocumentStart { get { return EnsureCommand(ref _SelectToDocumentStart , "SelectToDocumentStart" ); } } ////// SelectToDocumentEnd command. /// public static RoutedUICommand SelectToDocumentEnd { get { return EnsureCommand(ref _SelectToDocumentEnd , "SelectToDocumentEnd" ); } } // Character editing commands // -------------------------- ////// ToggleBold command. /// When command argument is present applies provided value to a selected range. /// When command argument is null applies an value of FontWeight opposite to the one taken from the first /// character of selected range. /// When selection is empty and within a word, the same operation is applied to this word. /// When empty selection is between words or in the process of typing /// the property is springloaded. /// public static RoutedUICommand ToggleBold { get { return EnsureCommand(ref _ToggleBold , "ToggleBold" ); } } ////// ToggleItalic command. /// public static RoutedUICommand ToggleItalic { get { return EnsureCommand(ref _ToggleItalic , "ToggleItalic" ); } } ////// ToggleUnderline command. /// public static RoutedUICommand ToggleUnderline { get { return EnsureCommand(ref _ToggleUnderline , "ToggleUnderline" ); } } ////// ToggleSubscript command. /// public static RoutedUICommand ToggleSubscript { get { return EnsureCommand(ref _ToggleSubscript , "ToggleSubscript" ); } } ////// ToggleSuperscript command. /// public static RoutedUICommand ToggleSuperscript { get { return EnsureCommand(ref _ToggleSuperscript , "ToggleSuperscript" ); } } ////// IncreaseFontSize command. /// public static RoutedUICommand IncreaseFontSize { get { return EnsureCommand(ref _IncreaseFontSize , "IncreaseFontSize" ); } } ////// DecreaseFontSize command. /// public static RoutedUICommand DecreaseFontSize { get { return EnsureCommand(ref _DecreaseFontSize , "DecreaseFontSize" ); } } // Paragraph editing commands // -------------------------- ////// AlignLeft command. /// public static RoutedUICommand AlignLeft { get { return EnsureCommand(ref _AlignLeft , "AlignLeft" ); } } ////// AlightCenter command. /// public static RoutedUICommand AlignCenter { get { return EnsureCommand(ref _AlignCenter , "AlignCenter" ); } } ////// AlignRight command. /// public static RoutedUICommand AlignRight { get { return EnsureCommand(ref _AlignRight , "AlignRight" ); } } ////// AlignJustify command. /// public static RoutedUICommand AlignJustify { get { return EnsureCommand(ref _AlignJustify , "AlignJustify" ); } } // List editing commands // --------------------- ////// ToggelBullets command. /// When command argument is present it must be of ListMarkerStyle value; /// this value is set as a marker style to all selected list items. /// When command argument is null the command toggles a marker style /// by circle over all predefined non-numeric list marker styles. /// The circle includes no-list state as well. /// public static RoutedUICommand ToggleBullets { get { return EnsureCommand(ref _ToggleBullets , "ToggleBullets" ); } } ////// ToggelNumbers command. /// When command argument is present it must be of ListMarkerStyle value; /// this value is set as a marker style to all selected list items. /// When command argument is null the command toggles a marker style /// by circle over all predefined numeric list marker styles /// The circle includes no-list state as well. /// public static RoutedUICommand ToggleNumbering { get { return EnsureCommand(ref _ToggleNumbering , "ToggleNumbering" ); } } ////// IncreaseIndentation command. /// public static RoutedUICommand IncreaseIndentation { get { return EnsureCommand(ref _IncreaseIndentation, "IncreaseIndentation"); } } ////// DecreaseIndentation command. /// public static RoutedUICommand DecreaseIndentation { get { return EnsureCommand(ref _DecreaseIndentation, "DecreaseIndentation"); } } // Spelling commands // --------------------- ////// Corrects a misspelled word at the insertion position. /// public static RoutedUICommand CorrectSpellingError { get { return EnsureCommand(ref _CorrectSpellingError, "CorrectSpellingError" ); } } ////// Ignores all instances of the misspelled word at the insertion position. /// public static RoutedUICommand IgnoreSpellingError { get { return EnsureCommand(ref _IgnoreSpellingError, "IgnoreSpellingError" ); } } #endregion Public Properties //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties // NOTE: The following pieces of code must be maintained consistently // f) Re-expose via reflection in Lexicon // Typing commands internal static RoutedUICommand Space { get { return EnsureCommand(ref _Space , "Space" ); } } internal static RoutedUICommand ShiftSpace { get { return EnsureCommand(ref _ShiftSpace , "ShiftSpace" ); } } // Caret navigation commands // ------------------------- internal static RoutedUICommand MoveToColumnStart { get { return EnsureCommand(ref _MoveToColumnStart , "MoveToColumnStart" ); } } internal static RoutedUICommand MoveToColumnEnd { get { return EnsureCommand(ref _MoveToColumnEnd , "MoveToColumnEnd" ); } } internal static RoutedUICommand MoveToWindowTop { get { return EnsureCommand(ref _MoveToWindowTop , "MoveToWindowTop" ); } } internal static RoutedUICommand MoveToWindowBottom { get { return EnsureCommand(ref _MoveToWindowBottom , "MoveToWindowBottom" ); } } // Selection extension commands // ---------------------------- internal static RoutedUICommand SelectToColumnStart { get { return EnsureCommand(ref _SelectToColumnStart , "SelectToColumnStart" ); } } internal static RoutedUICommand SelectToColumnEnd { get { return EnsureCommand(ref _SelectToColumnEnd , "SelectToColumnEnd" ); } } internal static RoutedUICommand SelectToWindowTop { get { return EnsureCommand(ref _SelectToWindowTop , "SelectToWindowTop" ); } } internal static RoutedUICommand SelectToWindowBottom { get { return EnsureCommand(ref _SelectToWindowBottom , "SelectToWindowBottom" ); } } // Character editing commands // -------------------------- internal static RoutedUICommand ResetFormat { get { return EnsureCommand(ref _ResetFormat , "ResetFormat" ); } } internal static RoutedUICommand ToggleSpellCheck { get { return EnsureCommand(ref _ToggleSpellCheck , "ToggleSpellCheck" ); } } internal static RoutedUICommand ApplyFontSize { get { return EnsureCommand(ref _ApplyFontSize , "ApplyFontSize" ); } } internal static RoutedUICommand ApplyFontFamily { get { return EnsureCommand(ref _ApplyFontFamily , "ApplyFontFamily" ); } } internal static RoutedUICommand ApplyForeground { get { return EnsureCommand(ref _ApplyForeground , "ApplyForeground" ); } } internal static RoutedUICommand ApplyBackground { get { return EnsureCommand(ref _ApplyBackground , "ApplyBackground" ); } } internal static RoutedUICommand ApplyInlineFlowDirectionRTL { get { return EnsureCommand(ref _ApplyInlineFlowDirectionRTL , "ApplyInlineFlowDirectionRTL" ); } } internal static RoutedUICommand ApplyInlineFlowDirectionLTR { get { return EnsureCommand(ref _ApplyInlineFlowDirectionLTR , "ApplyInlineFlowDirectionLTR" ); } } // Paragraph editing commands // -------------------------- internal static RoutedUICommand ApplySingleSpace { get { return EnsureCommand(ref _ApplySingleSpace , "ApplySingleSpace" ); } } internal static RoutedUICommand ApplyOneAndAHalfSpace { get { return EnsureCommand(ref _ApplyOneAndAHalfSpace , "ApplyOneAndAHalfSpace" ); } } internal static RoutedUICommand ApplyDoubleSpace { get { return EnsureCommand(ref _ApplyDoubleSpace , "ApplyDoubleSpace" ); } } internal static RoutedUICommand ApplyParagraphFlowDirectionRTL { get { return EnsureCommand(ref _ApplyParagraphFlowDirectionRTL , "ApplyParagraphFlowDirectionRTL" ); } } internal static RoutedUICommand ApplyParagraphFlowDirectionLTR { get { return EnsureCommand(ref _ApplyParagraphFlowDirectionLTR , "ApplyParagraphFlowDirectionLTR" ); } } // CopyPaste Commands // ------------------ internal static RoutedUICommand CopyFormat { get { return EnsureCommand(ref _CopyFormat , "CopyFormat" ); } } internal static RoutedUICommand PasteFormat { get { return EnsureCommand(ref _PasteFormat , "PasteFormat" ); } } // List editing commands // --------------------- internal static RoutedUICommand RemoveListMarkers { get { return EnsureCommand(ref _RemoveListMarkers , "RemoveListMarkers" ); } } // Table editing commands // ---------------------- internal static RoutedUICommand InsertTable { get { return EnsureCommand(ref _InsertTable , "InsertTable" ); } } internal static RoutedUICommand InsertRows { get { return EnsureCommand(ref _InsertRows , "InsertRows" ); } } internal static RoutedUICommand InsertColumns { get { return EnsureCommand(ref _InsertColumns , "InsertColumns" ); } } internal static RoutedUICommand DeleteRows { get { return EnsureCommand(ref _DeleteRows , "DeleteRows" ); } } internal static RoutedUICommand DeleteColumns { get { return EnsureCommand(ref _DeleteColumns , "DeleteColumns" ); } } internal static RoutedUICommand MergeCells { get { return EnsureCommand(ref _MergeCells , "MergeCells" ); } } internal static RoutedUICommand SplitCell { get { return EnsureCommand(ref _SplitCell , "SplitCell" ); } } #endregion Public Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Initializes a static command definition - by demand private static RoutedUICommand EnsureCommand(ref RoutedUICommand command, string commandPropertyName) { lock (_synchronize) { if (command == null) { // command = new RoutedUICommand(commandPropertyName, commandPropertyName, typeof(EditingCommands)); } } return command; } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties private static object _synchronize = new object(); // Input commands // -------------- private static RoutedUICommand _ToggleInsert; private static RoutedUICommand _Delete; private static RoutedUICommand _Backspace; private static RoutedUICommand _DeleteNextWord; private static RoutedUICommand _DeletePreviousWord; private static RoutedUICommand _EnterParagraphBreak; private static RoutedUICommand _EnterLineBreak; private static RoutedUICommand _TabForward; private static RoutedUICommand _TabBackward; private static RoutedUICommand _Space; private static RoutedUICommand _ShiftSpace; // Caret navigation commands // ------------------------- private static RoutedUICommand _MoveRightByCharacter; private static RoutedUICommand _MoveLeftByCharacter; private static RoutedUICommand _MoveRightByWord; private static RoutedUICommand _MoveLeftByWord; private static RoutedUICommand _MoveDownByLine; private static RoutedUICommand _MoveUpByLine; private static RoutedUICommand _MoveDownByParagraph; private static RoutedUICommand _MoveUpByParagraph; private static RoutedUICommand _MoveDownByPage; private static RoutedUICommand _MoveUpByPage; private static RoutedUICommand _MoveToLineStart; private static RoutedUICommand _MoveToLineEnd; private static RoutedUICommand _MoveToColumnStart; private static RoutedUICommand _MoveToColumnEnd; private static RoutedUICommand _MoveToWindowTop; private static RoutedUICommand _MoveToWindowBottom; private static RoutedUICommand _MoveToDocumentStart; private static RoutedUICommand _MoveToDocumentEnd; // Selection extension commands // ---------------------------- private static RoutedUICommand _SelectRightByCharacter; private static RoutedUICommand _SelectLeftByCharacter; private static RoutedUICommand _SelectRightByWord; private static RoutedUICommand _SelectLeftByWord; private static RoutedUICommand _SelectDownByLine; private static RoutedUICommand _SelectUpByLine; private static RoutedUICommand _SelectDownByParagraph; private static RoutedUICommand _SelectUpByParagraph; private static RoutedUICommand _SelectDownByPage; private static RoutedUICommand _SelectUpByPage; private static RoutedUICommand _SelectToLineStart; private static RoutedUICommand _SelectToLineEnd; private static RoutedUICommand _SelectToColumnStart; private static RoutedUICommand _SelectToColumnEnd; private static RoutedUICommand _SelectToWindowTop; private static RoutedUICommand _SelectToWindowBottom; private static RoutedUICommand _SelectToDocumentStart; private static RoutedUICommand _SelectToDocumentEnd; // Character editing commands // -------------------------- private static RoutedUICommand _CopyFormat; private static RoutedUICommand _PasteFormat; private static RoutedUICommand _ResetFormat; private static RoutedUICommand _ToggleBold; private static RoutedUICommand _ToggleItalic; private static RoutedUICommand _ToggleUnderline; private static RoutedUICommand _ToggleSubscript; private static RoutedUICommand _ToggleSuperscript; private static RoutedUICommand _IncreaseFontSize; private static RoutedUICommand _DecreaseFontSize; private static RoutedUICommand _ApplyFontSize; private static RoutedUICommand _ApplyFontFamily; private static RoutedUICommand _ApplyForeground; private static RoutedUICommand _ApplyBackground; private static RoutedUICommand _ToggleSpellCheck; private static RoutedUICommand _ApplyInlineFlowDirectionRTL; private static RoutedUICommand _ApplyInlineFlowDirectionLTR; // Paragraph editing commands // -------------------------- private static RoutedUICommand _AlignLeft; private static RoutedUICommand _AlignCenter; private static RoutedUICommand _AlignRight; private static RoutedUICommand _AlignJustify; private static RoutedUICommand _ApplySingleSpace; private static RoutedUICommand _ApplyOneAndAHalfSpace; private static RoutedUICommand _ApplyDoubleSpace; private static RoutedUICommand _IncreaseIndentation; private static RoutedUICommand _DecreaseIndentation; private static RoutedUICommand _ApplyParagraphFlowDirectionRTL; private static RoutedUICommand _ApplyParagraphFlowDirectionLTR; // List editing commands // --------------------- private static RoutedUICommand _RemoveListMarkers; private static RoutedUICommand _ToggleBullets; private static RoutedUICommand _ToggleNumbering; // Table editing commands // ---------------------- private static RoutedUICommand _InsertTable; private static RoutedUICommand _InsertRows; private static RoutedUICommand _InsertColumns; private static RoutedUICommand _DeleteRows; private static RoutedUICommand _DeleteColumns; private static RoutedUICommand _MergeCells; private static RoutedUICommand _SplitCell; // Spelling Commands // ----------------- private static RoutedUICommand _CorrectSpellingError; private static RoutedUICommand _IgnoreSpellingError; #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: EditingCommands.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Command definitions for Rich Text Editing. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System.Windows.Input; // Command using System.ComponentModel; // TypeConverter ////// Command definitions for Rich Text Editing. /// public static class EditingCommands { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties // Typing Commands // --------------- ////// ToggleInsert command. /// Changed typing mode between insertion and overtyping. /// public static RoutedUICommand ToggleInsert { get { return EnsureCommand(ref _ToggleInsert , "ToggleInsert" ); } } ////// Delete command. /// When selection is empty deletes the following character or paragraph separator. /// When selection is not empty deletes the selected content. /// Formatting of deleted content is not springloaded (unlike Backspace). /// public static RoutedUICommand Delete { get { return EnsureCommand(ref _Delete , "Delete" ); } } ////// Backspace command. /// When selection is empty deleted the previous character or paragraph separator. /// When selection is not empty deletes the selected content. /// Formatting of deleted content is springloaded (unlike Delete). /// Formatting for springloading is taken from the very first selected character. /// public static RoutedUICommand Backspace { get { return EnsureCommand(ref _Backspace , "Backspace" ); } } ////// DeleteNextWord command. /// public static RoutedUICommand DeleteNextWord { get { return EnsureCommand(ref _DeleteNextWord , "DeleteNextWord" ); } } ////// DeletePreviousWord command. /// public static RoutedUICommand DeletePreviousWord { get { return EnsureCommand(ref _DeletePreviousWord , "DeletePreviousWord" ); } } ////// EnterParagraphBreak command. /// Acts as if the user presses Enter key. The content of current selection is deleted (if not empty) /// like with Backspace command(performing formatting springloading), and then /// the structure of text elements is changed so that paragraph break appears /// at caret position. /// public static RoutedUICommand EnterParagraphBreak { get { return EnsureCommand(ref _EnterParagraphBreak , "EnterParagraphBreak" ); } } ////// EnterLineBreak command. /// public static RoutedUICommand EnterLineBreak { get { return EnsureCommand(ref _EnterLineBreak , "EnterLineBreak" ); } } ////// TabForward command. /// The behavior depends from the current condition of selection. /// If selection is non-empty then it redirects to a IncreaseIndentation command, /// so that all affected paragraphs become promoted (by increasing their Margin.Left property in RichTextBox /// or by inserting additional Tab charater in the beginning of each non-wrapped line). /// If the caret is in table cell then it moves to the next cell. /// If the caret is in the last table cell, then in creates new row in a table and moves /// the caret into first cell of that row. /// Otherwise Tab character is inserted in current position. /// public static RoutedUICommand TabForward { get { return EnsureCommand(ref _TabForward , "TabForward" ); } } ////// TabBackward command. /// The behavior depends from the current condition of selection. /// If selection is non-empty then it redirects to a DecreaseIndentation command, /// so that all affected paragraphs become promoted (by decreasing their Margin.Left property in RichTextBox /// or by deleting a Tab charater in the beginning of each non-wrapped line). /// If the caret is in table cell then it moves to the previous cell. /// Otherwise Tab character is inserted in current position. /// public static RoutedUICommand TabBackward { get { return EnsureCommand(ref _TabBackward , "TabBackward" ); } } // Caret navigation commands // ------------------------- ////// MoveRightByCharacter command. /// public static RoutedUICommand MoveRightByCharacter { get { return EnsureCommand(ref _MoveRightByCharacter , "MoveRightByCharacter" ); } } ////// MoveLeftByCharacter command. /// public static RoutedUICommand MoveLeftByCharacter { get { return EnsureCommand(ref _MoveLeftByCharacter , "MoveLeftByCharacter" ); } } ////// MoveRightByWord command. /// public static RoutedUICommand MoveRightByWord { get { return EnsureCommand(ref _MoveRightByWord , "MoveRightByWord" ); } } ////// MoveLeftByWord command. /// public static RoutedUICommand MoveLeftByWord { get { return EnsureCommand(ref _MoveLeftByWord , "MoveLeftByWord" ); } } ////// MoveDownByLine command. /// public static RoutedUICommand MoveDownByLine { get { return EnsureCommand(ref _MoveDownByLine , "MoveDownByLine" ); } } ////// MoveUpByLine command. /// public static RoutedUICommand MoveUpByLine { get { return EnsureCommand(ref _MoveUpByLine , "MoveUpByLine" ); } } ////// MoveDownByParagraph command. /// public static RoutedUICommand MoveDownByParagraph { get { return EnsureCommand(ref _MoveDownByParagraph , "MoveDownByParagraph" ); } } ////// MoveUpByParagraph command. /// public static RoutedUICommand MoveUpByParagraph { get { return EnsureCommand(ref _MoveUpByParagraph , "MoveUpByParagraph" ); } } ////// MoveDownByPage command. /// Corresponds to PgDn key on the keyboard. /// public static RoutedUICommand MoveDownByPage { get { return EnsureCommand(ref _MoveDownByPage , "MoveDownByPage" ); } } ////// MoveUpByPage command. /// Corresponds to PgUp key on the keyboard. /// public static RoutedUICommand MoveUpByPage { get { return EnsureCommand(ref _MoveUpByPage , "MoveUpByPage" ); } } ////// MoveToLineStart command. /// Corresponds to Home key on the keyboard. /// public static RoutedUICommand MoveToLineStart { get { return EnsureCommand(ref _MoveToLineStart , "MoveToLineStart" ); } } ////// MoveToLineEnd command. /// Corresponds to End key on the keyboard. /// public static RoutedUICommand MoveToLineEnd { get { return EnsureCommand(ref _MoveToLineEnd , "MoveToLineEnd" ); } } ////// MoveToDocumentStart command. /// public static RoutedUICommand MoveToDocumentStart { get { return EnsureCommand(ref _MoveToDocumentStart , "MoveToDocumentStart" ); } } ////// MoveToDocumentEnd command. /// public static RoutedUICommand MoveToDocumentEnd { get { return EnsureCommand(ref _MoveToDocumentEnd , "MoveToDocumentEnd" ); } } // Selection extension commands // ---------------------------- ////// SelectRightByCharacter command. /// public static RoutedUICommand SelectRightByCharacter { get { return EnsureCommand(ref _SelectRightByCharacter , "SelectRightByCharacter" ); } } ////// SelectLeftByCharacter command. /// public static RoutedUICommand SelectLeftByCharacter { get { return EnsureCommand(ref _SelectLeftByCharacter , "SelectLeftByCharacter" ); } } ////// SelectRightByWord command. /// public static RoutedUICommand SelectRightByWord { get { return EnsureCommand(ref _SelectRightByWord , "SelectRightByWord" ); } } ////// SelectLeftbyWord command. /// public static RoutedUICommand SelectLeftByWord { get { return EnsureCommand(ref _SelectLeftByWord , "SelectLeftByWord" ); } } ////// SelectDownByLine command. /// public static RoutedUICommand SelectDownByLine { get { return EnsureCommand(ref _SelectDownByLine , "SelectDownByLine" ); } } ////// SelectUpByLine command. /// public static RoutedUICommand SelectUpByLine { get { return EnsureCommand(ref _SelectUpByLine , "SelectUpByLine" ); } } ////// SelectDownByParagraph command. /// public static RoutedUICommand SelectDownByParagraph { get { return EnsureCommand(ref _SelectDownByParagraph , "SelectDownByParagraph" ); } } ////// SelectUpByParagraph command. /// public static RoutedUICommand SelectUpByParagraph { get { return EnsureCommand(ref _SelectUpByParagraph , "SelectUpByParagraph" ); } } ////// SelectDownByPage command. /// public static RoutedUICommand SelectDownByPage { get { return EnsureCommand(ref _SelectDownByPage , "SelectDownByPage" ); } } ////// SelectUpByPage command. /// public static RoutedUICommand SelectUpByPage { get { return EnsureCommand(ref _SelectUpByPage , "SelectUpByPage" ); } } ////// SelectToLineStart command. /// public static RoutedUICommand SelectToLineStart { get { return EnsureCommand(ref _SelectToLineStart , "SelectToLineStart" ); } } ////// SelectToLineEnd command. /// public static RoutedUICommand SelectToLineEnd { get { return EnsureCommand(ref _SelectToLineEnd , "SelectToLineEnd" ); } } ////// SelectToDocumentStart command. /// public static RoutedUICommand SelectToDocumentStart { get { return EnsureCommand(ref _SelectToDocumentStart , "SelectToDocumentStart" ); } } ////// SelectToDocumentEnd command. /// public static RoutedUICommand SelectToDocumentEnd { get { return EnsureCommand(ref _SelectToDocumentEnd , "SelectToDocumentEnd" ); } } // Character editing commands // -------------------------- ////// ToggleBold command. /// When command argument is present applies provided value to a selected range. /// When command argument is null applies an value of FontWeight opposite to the one taken from the first /// character of selected range. /// When selection is empty and within a word, the same operation is applied to this word. /// When empty selection is between words or in the process of typing /// the property is springloaded. /// public static RoutedUICommand ToggleBold { get { return EnsureCommand(ref _ToggleBold , "ToggleBold" ); } } ////// ToggleItalic command. /// public static RoutedUICommand ToggleItalic { get { return EnsureCommand(ref _ToggleItalic , "ToggleItalic" ); } } ////// ToggleUnderline command. /// public static RoutedUICommand ToggleUnderline { get { return EnsureCommand(ref _ToggleUnderline , "ToggleUnderline" ); } } ////// ToggleSubscript command. /// public static RoutedUICommand ToggleSubscript { get { return EnsureCommand(ref _ToggleSubscript , "ToggleSubscript" ); } } ////// ToggleSuperscript command. /// public static RoutedUICommand ToggleSuperscript { get { return EnsureCommand(ref _ToggleSuperscript , "ToggleSuperscript" ); } } ////// IncreaseFontSize command. /// public static RoutedUICommand IncreaseFontSize { get { return EnsureCommand(ref _IncreaseFontSize , "IncreaseFontSize" ); } } ////// DecreaseFontSize command. /// public static RoutedUICommand DecreaseFontSize { get { return EnsureCommand(ref _DecreaseFontSize , "DecreaseFontSize" ); } } // Paragraph editing commands // -------------------------- ////// AlignLeft command. /// public static RoutedUICommand AlignLeft { get { return EnsureCommand(ref _AlignLeft , "AlignLeft" ); } } ////// AlightCenter command. /// public static RoutedUICommand AlignCenter { get { return EnsureCommand(ref _AlignCenter , "AlignCenter" ); } } ////// AlignRight command. /// public static RoutedUICommand AlignRight { get { return EnsureCommand(ref _AlignRight , "AlignRight" ); } } ////// AlignJustify command. /// public static RoutedUICommand AlignJustify { get { return EnsureCommand(ref _AlignJustify , "AlignJustify" ); } } // List editing commands // --------------------- ////// ToggelBullets command. /// When command argument is present it must be of ListMarkerStyle value; /// this value is set as a marker style to all selected list items. /// When command argument is null the command toggles a marker style /// by circle over all predefined non-numeric list marker styles. /// The circle includes no-list state as well. /// public static RoutedUICommand ToggleBullets { get { return EnsureCommand(ref _ToggleBullets , "ToggleBullets" ); } } ////// ToggelNumbers command. /// When command argument is present it must be of ListMarkerStyle value; /// this value is set as a marker style to all selected list items. /// When command argument is null the command toggles a marker style /// by circle over all predefined numeric list marker styles /// The circle includes no-list state as well. /// public static RoutedUICommand ToggleNumbering { get { return EnsureCommand(ref _ToggleNumbering , "ToggleNumbering" ); } } ////// IncreaseIndentation command. /// public static RoutedUICommand IncreaseIndentation { get { return EnsureCommand(ref _IncreaseIndentation, "IncreaseIndentation"); } } ////// DecreaseIndentation command. /// public static RoutedUICommand DecreaseIndentation { get { return EnsureCommand(ref _DecreaseIndentation, "DecreaseIndentation"); } } // Spelling commands // --------------------- ////// Corrects a misspelled word at the insertion position. /// public static RoutedUICommand CorrectSpellingError { get { return EnsureCommand(ref _CorrectSpellingError, "CorrectSpellingError" ); } } ////// Ignores all instances of the misspelled word at the insertion position. /// public static RoutedUICommand IgnoreSpellingError { get { return EnsureCommand(ref _IgnoreSpellingError, "IgnoreSpellingError" ); } } #endregion Public Properties //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties // NOTE: The following pieces of code must be maintained consistently // f) Re-expose via reflection in Lexicon // Typing commands internal static RoutedUICommand Space { get { return EnsureCommand(ref _Space , "Space" ); } } internal static RoutedUICommand ShiftSpace { get { return EnsureCommand(ref _ShiftSpace , "ShiftSpace" ); } } // Caret navigation commands // ------------------------- internal static RoutedUICommand MoveToColumnStart { get { return EnsureCommand(ref _MoveToColumnStart , "MoveToColumnStart" ); } } internal static RoutedUICommand MoveToColumnEnd { get { return EnsureCommand(ref _MoveToColumnEnd , "MoveToColumnEnd" ); } } internal static RoutedUICommand MoveToWindowTop { get { return EnsureCommand(ref _MoveToWindowTop , "MoveToWindowTop" ); } } internal static RoutedUICommand MoveToWindowBottom { get { return EnsureCommand(ref _MoveToWindowBottom , "MoveToWindowBottom" ); } } // Selection extension commands // ---------------------------- internal static RoutedUICommand SelectToColumnStart { get { return EnsureCommand(ref _SelectToColumnStart , "SelectToColumnStart" ); } } internal static RoutedUICommand SelectToColumnEnd { get { return EnsureCommand(ref _SelectToColumnEnd , "SelectToColumnEnd" ); } } internal static RoutedUICommand SelectToWindowTop { get { return EnsureCommand(ref _SelectToWindowTop , "SelectToWindowTop" ); } } internal static RoutedUICommand SelectToWindowBottom { get { return EnsureCommand(ref _SelectToWindowBottom , "SelectToWindowBottom" ); } } // Character editing commands // -------------------------- internal static RoutedUICommand ResetFormat { get { return EnsureCommand(ref _ResetFormat , "ResetFormat" ); } } internal static RoutedUICommand ToggleSpellCheck { get { return EnsureCommand(ref _ToggleSpellCheck , "ToggleSpellCheck" ); } } internal static RoutedUICommand ApplyFontSize { get { return EnsureCommand(ref _ApplyFontSize , "ApplyFontSize" ); } } internal static RoutedUICommand ApplyFontFamily { get { return EnsureCommand(ref _ApplyFontFamily , "ApplyFontFamily" ); } } internal static RoutedUICommand ApplyForeground { get { return EnsureCommand(ref _ApplyForeground , "ApplyForeground" ); } } internal static RoutedUICommand ApplyBackground { get { return EnsureCommand(ref _ApplyBackground , "ApplyBackground" ); } } internal static RoutedUICommand ApplyInlineFlowDirectionRTL { get { return EnsureCommand(ref _ApplyInlineFlowDirectionRTL , "ApplyInlineFlowDirectionRTL" ); } } internal static RoutedUICommand ApplyInlineFlowDirectionLTR { get { return EnsureCommand(ref _ApplyInlineFlowDirectionLTR , "ApplyInlineFlowDirectionLTR" ); } } // Paragraph editing commands // -------------------------- internal static RoutedUICommand ApplySingleSpace { get { return EnsureCommand(ref _ApplySingleSpace , "ApplySingleSpace" ); } } internal static RoutedUICommand ApplyOneAndAHalfSpace { get { return EnsureCommand(ref _ApplyOneAndAHalfSpace , "ApplyOneAndAHalfSpace" ); } } internal static RoutedUICommand ApplyDoubleSpace { get { return EnsureCommand(ref _ApplyDoubleSpace , "ApplyDoubleSpace" ); } } internal static RoutedUICommand ApplyParagraphFlowDirectionRTL { get { return EnsureCommand(ref _ApplyParagraphFlowDirectionRTL , "ApplyParagraphFlowDirectionRTL" ); } } internal static RoutedUICommand ApplyParagraphFlowDirectionLTR { get { return EnsureCommand(ref _ApplyParagraphFlowDirectionLTR , "ApplyParagraphFlowDirectionLTR" ); } } // CopyPaste Commands // ------------------ internal static RoutedUICommand CopyFormat { get { return EnsureCommand(ref _CopyFormat , "CopyFormat" ); } } internal static RoutedUICommand PasteFormat { get { return EnsureCommand(ref _PasteFormat , "PasteFormat" ); } } // List editing commands // --------------------- internal static RoutedUICommand RemoveListMarkers { get { return EnsureCommand(ref _RemoveListMarkers , "RemoveListMarkers" ); } } // Table editing commands // ---------------------- internal static RoutedUICommand InsertTable { get { return EnsureCommand(ref _InsertTable , "InsertTable" ); } } internal static RoutedUICommand InsertRows { get { return EnsureCommand(ref _InsertRows , "InsertRows" ); } } internal static RoutedUICommand InsertColumns { get { return EnsureCommand(ref _InsertColumns , "InsertColumns" ); } } internal static RoutedUICommand DeleteRows { get { return EnsureCommand(ref _DeleteRows , "DeleteRows" ); } } internal static RoutedUICommand DeleteColumns { get { return EnsureCommand(ref _DeleteColumns , "DeleteColumns" ); } } internal static RoutedUICommand MergeCells { get { return EnsureCommand(ref _MergeCells , "MergeCells" ); } } internal static RoutedUICommand SplitCell { get { return EnsureCommand(ref _SplitCell , "SplitCell" ); } } #endregion Public Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Initializes a static command definition - by demand private static RoutedUICommand EnsureCommand(ref RoutedUICommand command, string commandPropertyName) { lock (_synchronize) { if (command == null) { // command = new RoutedUICommand(commandPropertyName, commandPropertyName, typeof(EditingCommands)); } } return command; } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties private static object _synchronize = new object(); // Input commands // -------------- private static RoutedUICommand _ToggleInsert; private static RoutedUICommand _Delete; private static RoutedUICommand _Backspace; private static RoutedUICommand _DeleteNextWord; private static RoutedUICommand _DeletePreviousWord; private static RoutedUICommand _EnterParagraphBreak; private static RoutedUICommand _EnterLineBreak; private static RoutedUICommand _TabForward; private static RoutedUICommand _TabBackward; private static RoutedUICommand _Space; private static RoutedUICommand _ShiftSpace; // Caret navigation commands // ------------------------- private static RoutedUICommand _MoveRightByCharacter; private static RoutedUICommand _MoveLeftByCharacter; private static RoutedUICommand _MoveRightByWord; private static RoutedUICommand _MoveLeftByWord; private static RoutedUICommand _MoveDownByLine; private static RoutedUICommand _MoveUpByLine; private static RoutedUICommand _MoveDownByParagraph; private static RoutedUICommand _MoveUpByParagraph; private static RoutedUICommand _MoveDownByPage; private static RoutedUICommand _MoveUpByPage; private static RoutedUICommand _MoveToLineStart; private static RoutedUICommand _MoveToLineEnd; private static RoutedUICommand _MoveToColumnStart; private static RoutedUICommand _MoveToColumnEnd; private static RoutedUICommand _MoveToWindowTop; private static RoutedUICommand _MoveToWindowBottom; private static RoutedUICommand _MoveToDocumentStart; private static RoutedUICommand _MoveToDocumentEnd; // Selection extension commands // ---------------------------- private static RoutedUICommand _SelectRightByCharacter; private static RoutedUICommand _SelectLeftByCharacter; private static RoutedUICommand _SelectRightByWord; private static RoutedUICommand _SelectLeftByWord; private static RoutedUICommand _SelectDownByLine; private static RoutedUICommand _SelectUpByLine; private static RoutedUICommand _SelectDownByParagraph; private static RoutedUICommand _SelectUpByParagraph; private static RoutedUICommand _SelectDownByPage; private static RoutedUICommand _SelectUpByPage; private static RoutedUICommand _SelectToLineStart; private static RoutedUICommand _SelectToLineEnd; private static RoutedUICommand _SelectToColumnStart; private static RoutedUICommand _SelectToColumnEnd; private static RoutedUICommand _SelectToWindowTop; private static RoutedUICommand _SelectToWindowBottom; private static RoutedUICommand _SelectToDocumentStart; private static RoutedUICommand _SelectToDocumentEnd; // Character editing commands // -------------------------- private static RoutedUICommand _CopyFormat; private static RoutedUICommand _PasteFormat; private static RoutedUICommand _ResetFormat; private static RoutedUICommand _ToggleBold; private static RoutedUICommand _ToggleItalic; private static RoutedUICommand _ToggleUnderline; private static RoutedUICommand _ToggleSubscript; private static RoutedUICommand _ToggleSuperscript; private static RoutedUICommand _IncreaseFontSize; private static RoutedUICommand _DecreaseFontSize; private static RoutedUICommand _ApplyFontSize; private static RoutedUICommand _ApplyFontFamily; private static RoutedUICommand _ApplyForeground; private static RoutedUICommand _ApplyBackground; private static RoutedUICommand _ToggleSpellCheck; private static RoutedUICommand _ApplyInlineFlowDirectionRTL; private static RoutedUICommand _ApplyInlineFlowDirectionLTR; // Paragraph editing commands // -------------------------- private static RoutedUICommand _AlignLeft; private static RoutedUICommand _AlignCenter; private static RoutedUICommand _AlignRight; private static RoutedUICommand _AlignJustify; private static RoutedUICommand _ApplySingleSpace; private static RoutedUICommand _ApplyOneAndAHalfSpace; private static RoutedUICommand _ApplyDoubleSpace; private static RoutedUICommand _IncreaseIndentation; private static RoutedUICommand _DecreaseIndentation; private static RoutedUICommand _ApplyParagraphFlowDirectionRTL; private static RoutedUICommand _ApplyParagraphFlowDirectionLTR; // List editing commands // --------------------- private static RoutedUICommand _RemoveListMarkers; private static RoutedUICommand _ToggleBullets; private static RoutedUICommand _ToggleNumbering; // Table editing commands // ---------------------- private static RoutedUICommand _InsertTable; private static RoutedUICommand _InsertRows; private static RoutedUICommand _InsertColumns; private static RoutedUICommand _DeleteRows; private static RoutedUICommand _DeleteColumns; private static RoutedUICommand _MergeCells; private static RoutedUICommand _SplitCell; // Spelling Commands // ----------------- private static RoutedUICommand _CorrectSpellingError; private static RoutedUICommand _IgnoreSpellingError; #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
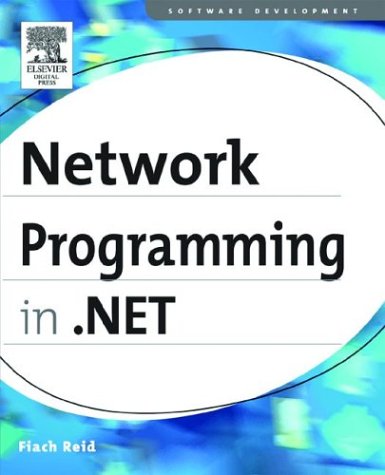
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SortKey.cs
- ToolStripTextBox.cs
- SqlConnection.cs
- FragmentQueryKB.cs
- codemethodreferenceexpression.cs
- MenuCommands.cs
- LinkUtilities.cs
- AQNBuilder.cs
- NamespaceInfo.cs
- EUCJPEncoding.cs
- ReaderWriterLockWrapper.cs
- LineVisual.cs
- HtmlToClrEventProxy.cs
- AutomationPropertyInfo.cs
- ObjectDataProvider.cs
- ReflectionHelper.cs
- WorkflowApplicationException.cs
- LongValidatorAttribute.cs
- WebPartManager.cs
- Bits.cs
- HitTestFilterBehavior.cs
- DocumentScope.cs
- Pair.cs
- SafeThemeHandle.cs
- sqlpipe.cs
- EntityCommandDefinition.cs
- NameValuePermission.cs
- IndexedString.cs
- CharacterMetrics.cs
- CodePropertyReferenceExpression.cs
- TransformPatternIdentifiers.cs
- MessageVersion.cs
- DispatcherObject.cs
- ArgumentOutOfRangeException.cs
- Geometry.cs
- Debug.cs
- Model3D.cs
- ResourceExpressionBuilder.cs
- Win32PrintDialog.cs
- DataGridColumnHeadersPresenter.cs
- EndEvent.cs
- SQLMembershipProvider.cs
- CssTextWriter.cs
- RegistrationProxy.cs
- InheritanceContextChangedEventManager.cs
- WebPartsSection.cs
- ViewPort3D.cs
- SecurityDescriptor.cs
- TripleDES.cs
- ResourceProperty.cs
- VisualStyleInformation.cs
- PasswordRecoveryAutoFormat.cs
- webclient.cs
- DecoderFallbackWithFailureFlag.cs
- DesignTimeVisibleAttribute.cs
- PrintDocument.cs
- CacheRequest.cs
- SrgsRule.cs
- HMACRIPEMD160.cs
- ToolStripDropDown.cs
- PeerIPHelper.cs
- LocalBuilder.cs
- CuspData.cs
- OutputScopeManager.cs
- StringComparer.cs
- SelectionEditor.cs
- ConnectionInterfaceCollection.cs
- HttpWrapper.cs
- CachedPathData.cs
- FixedFindEngine.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- SizeAnimationBase.cs
- DiscoveryInnerClientAdhocCD1.cs
- PriorityQueue.cs
- PropertyEntry.cs
- WinFormsSecurity.cs
- KeyProperty.cs
- FontDriver.cs
- DiscoveryClientDocuments.cs
- XmlnsCache.cs
- IndexedWhereQueryOperator.cs
- ClientUtils.cs
- nulltextnavigator.cs
- MexHttpsBindingCollectionElement.cs
- IBuiltInEvidence.cs
- EntityDataSource.cs
- TimeoutValidationAttribute.cs
- CounterSampleCalculator.cs
- TextEncodedRawTextWriter.cs
- WmlPageAdapter.cs
- ImageInfo.cs
- GenerateHelper.cs
- TabletDevice.cs
- UmAlQuraCalendar.cs
- ImageDrawing.cs
- Socket.cs
- ListItem.cs
- TypedElement.cs
- SqlCommandBuilder.cs
- Decoder.cs