Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Security / Policy / IBuiltInEvidence.cs / 1 / IBuiltInEvidence.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // IBuiltInEvidence.cs // namespace System.Security.Policy { internal interface IBuiltInEvidence { int OutputToBuffer( char[] buffer, int position, bool verbose ); // Initializes a class according to data in the buffer. Returns new position within buffer int InitFromBuffer( char[] buffer, int position); int GetRequiredSize(bool verbose); } internal static class BuiltInEvidenceHelper { internal const char idApplicationDirectory = (char)0; #if !FEATURE_PAL internal const char idPublisher = (char)1; #endif //!FEATURE_PAL internal const char idStrongName = (char)2; internal const char idZone = (char)3; internal const char idUrl = (char)4; internal const char idWebPage = (char)5; internal const char idSite = (char)6; internal const char idPermissionRequestEvidence = (char)7; #if !FEATURE_PAL internal const char idHash = (char)8; #endif // !FEATURE_PAL internal const char idGac = (char)9; internal static void CopyIntToCharArray( int value, char[] buffer, int position ) { buffer[position ] = (char)((value >> 16) & 0x0000FFFF); buffer[position + 1] = (char)((value ) & 0x0000FFFF); } internal static int GetIntFromCharArray(char[] buffer, int position ) { int value = (int)buffer[position]; value = value << 16; value += (int)buffer[position + 1]; return value; } internal static void CopyLongToCharArray( long value, char[] buffer, int position ) { buffer[position ] = (char)((value >> 48) & 0x000000000000FFFF); buffer[position + 1] = (char)((value >> 32) & 0x000000000000FFFF); buffer[position + 2] = (char)((value >> 16) & 0x000000000000FFFF); buffer[position + 3] = (char)((value ) & 0x000000000000FFFF); } internal static long GetLongFromCharArray(char[] buffer, int position ) { long value = (long)buffer[position]; value = value << 16; value += (long)buffer[position + 1]; value = value << 16; value += (long)buffer[position + 2]; value = value << 16; value += (long)buffer[position + 3]; return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // IBuiltInEvidence.cs // namespace System.Security.Policy { internal interface IBuiltInEvidence { int OutputToBuffer( char[] buffer, int position, bool verbose ); // Initializes a class according to data in the buffer. Returns new position within buffer int InitFromBuffer( char[] buffer, int position); int GetRequiredSize(bool verbose); } internal static class BuiltInEvidenceHelper { internal const char idApplicationDirectory = (char)0; #if !FEATURE_PAL internal const char idPublisher = (char)1; #endif //!FEATURE_PAL internal const char idStrongName = (char)2; internal const char idZone = (char)3; internal const char idUrl = (char)4; internal const char idWebPage = (char)5; internal const char idSite = (char)6; internal const char idPermissionRequestEvidence = (char)7; #if !FEATURE_PAL internal const char idHash = (char)8; #endif // !FEATURE_PAL internal const char idGac = (char)9; internal static void CopyIntToCharArray( int value, char[] buffer, int position ) { buffer[position ] = (char)((value >> 16) & 0x0000FFFF); buffer[position + 1] = (char)((value ) & 0x0000FFFF); } internal static int GetIntFromCharArray(char[] buffer, int position ) { int value = (int)buffer[position]; value = value << 16; value += (int)buffer[position + 1]; return value; } internal static void CopyLongToCharArray( long value, char[] buffer, int position ) { buffer[position ] = (char)((value >> 48) & 0x000000000000FFFF); buffer[position + 1] = (char)((value >> 32) & 0x000000000000FFFF); buffer[position + 2] = (char)((value >> 16) & 0x000000000000FFFF); buffer[position + 3] = (char)((value ) & 0x000000000000FFFF); } internal static long GetLongFromCharArray(char[] buffer, int position ) { long value = (long)buffer[position]; value = value << 16; value += (long)buffer[position + 1]; value = value << 16; value += (long)buffer[position + 2]; value = value << 16; value += (long)buffer[position + 3]; return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
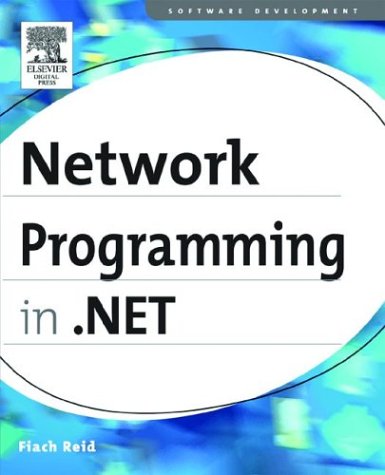
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusPointPropertyInfoDefaults.cs
- MinMaxParagraphWidth.cs
- WsrmFault.cs
- ApplicationActivator.cs
- ImageDrawing.cs
- SerialStream.cs
- TargetConverter.cs
- XsltArgumentList.cs
- SchemaMapping.cs
- SqlBulkCopyColumnMappingCollection.cs
- SelectingProviderEventArgs.cs
- DataPointer.cs
- XPathDocumentIterator.cs
- CatalogPartCollection.cs
- HwndHostAutomationPeer.cs
- HandlerMappingMemo.cs
- ActiveXContainer.cs
- ServiceContractListItem.cs
- AncestorChangedEventArgs.cs
- RadioButtonPopupAdapter.cs
- ColumnHeaderConverter.cs
- SoapExtensionImporter.cs
- UriTemplate.cs
- MenuItemBinding.cs
- SpecialFolderEnumConverter.cs
- XPathExpr.cs
- SafeNativeMethods.cs
- DrawingGroup.cs
- OleDbCommand.cs
- UrlMappingsSection.cs
- HtmlInputText.cs
- CreateUserWizardStep.cs
- FillErrorEventArgs.cs
- DataGridViewComboBoxEditingControl.cs
- SafeSystemMetrics.cs
- TextParagraph.cs
- TreeViewHitTestInfo.cs
- ConfigXmlCDataSection.cs
- FormsAuthenticationCredentials.cs
- XPathEmptyIterator.cs
- XmlSiteMapProvider.cs
- JsonWriterDelegator.cs
- MarkupWriter.cs
- ValidatorCompatibilityHelper.cs
- CodeNamespaceImportCollection.cs
- DocumentReferenceCollection.cs
- RunClient.cs
- ServiceModelPerformanceCounters.cs
- EndSelectCardRequest.cs
- WebPartsSection.cs
- SamlEvidence.cs
- WmlValidatorAdapter.cs
- RouteItem.cs
- DataServiceQueryOfT.cs
- DWriteFactory.cs
- WorkflowQueueInfo.cs
- ImageBrush.cs
- MissingMethodException.cs
- ValidationUtility.cs
- ItemList.cs
- ComboBoxAutomationPeer.cs
- DynamicObjectAccessor.cs
- HttpServerVarsCollection.cs
- ExpressionNormalizer.cs
- InputBinder.cs
- WebServiceResponse.cs
- ThicknessAnimation.cs
- XmlSubtreeReader.cs
- Axis.cs
- ListBox.cs
- SoapReflectionImporter.cs
- ApplicationTrust.cs
- CompositeKey.cs
- StreamWithDictionary.cs
- GiveFeedbackEvent.cs
- ButtonBaseAdapter.cs
- RelativeSource.cs
- SystemParameters.cs
- Misc.cs
- PolyLineSegmentFigureLogic.cs
- selecteditemcollection.cs
- Domain.cs
- InputLanguageEventArgs.cs
- SortKey.cs
- TypeElement.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- RemotingConfigParser.cs
- ScriptControl.cs
- EventSetter.cs
- CqlLexer.cs
- PropertyValueChangedEvent.cs
- SerializationInfo.cs
- DirtyTextRange.cs
- GacUtil.cs
- EventPropertyMap.cs
- SafeRightsManagementQueryHandle.cs
- WindowsPrincipal.cs
- ComplexPropertyEntry.cs
- PreservationFileReader.cs
- ClientRuntime.cs