Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / PtsHost / RunClient.cs / 1305600 / RunClient.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: RunClient.cs // // Description: RunClient represents opaque data storage associated with runs // consumed by TextFormatter. // // History: // 05/05/2003 : [....] - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; using MS.Internal.Text; using MS.Internal.Documents; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Inline object run. /// internal sealed class InlineObjectRun : TextEmbeddedObject { ////// Constructor. /// /// Number of text position in the text array occupied by the inline object. /// UIElement representing the inline object. /// Text run properties for the inline object. /// Paragraph - the host of the inline object. internal InlineObjectRun(int cch, UIElement element, TextRunProperties textProps, TextParagraph host) { _cch = cch; _textProps = textProps; _host = host; _inlineUIContainer = (InlineUIContainer)LogicalTreeHelper.GetParent(element); } ////// Get inline object's measurement metrics. /// /// Remaining paragraph width. ///Inline object metrics. public override TextEmbeddedObjectMetrics Format(double remainingParagraphWidth) { Size desiredSize = _host.MeasureChild(this); // Make sure that LS/PTS limitations are not exceeded for object's size. TextDpi.EnsureValidObjSize(ref desiredSize); double baseline = desiredSize.Height; double baselineOffsetValue = (double)UIElementIsland.Root.GetValue(TextBlock.BaselineOffsetProperty); if(!DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = baselineOffsetValue; } return new TextEmbeddedObjectMetrics(desiredSize.Width, desiredSize.Height, baseline); } ////// Get computed bounding box of the inline object. /// /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. ///Computed bounding box size of text object. public override Rect ComputeBoundingBox(bool rightToLeft, bool sideways) { // Initially assume that bounding box is the same as layout box. // NOTE: PTS requires bounding box during line formatting. This eventually triggers // TextFormatter to call this function. // But at that time computed size is not available yet. Use desired size instead. Size size = UIElementIsland.Root.DesiredSize; double baseline = !sideways ? size.Height : size.Width; double baselineOffsetValue = (double)UIElementIsland.Root.GetValue(TextBlock.BaselineOffsetProperty); if (!sideways && !DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = (double) baselineOffsetValue; } return new Rect(0, -baseline, sideways ? size.Height : size.Width, sideways ? size.Width : size.Height); } ////// Draw the inline object. /// /// Drawing context. /// Origin where the object is drawn. /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. public override void Draw(DrawingContext drawingContext, Point origin, bool rightToLeft, bool sideways) { // Inline object has its own visual and it is attached to a visual // tree during arrange process. // Do nothing here. } ////// Reference to character buffer. /// public override CharacterBufferReference CharacterBufferReference { get { return new CharacterBufferReference(String.Empty, 0); } } ////// Length of the inline object run. /// public override int Length { get { return _cch; } } ////// A set of properties shared by every characters in the run /// public override TextRunProperties Properties { get { return _textProps; } } ////// Line break condition before the inline object. /// public override LineBreakCondition BreakBefore { get { return LineBreakCondition.BreakDesired; } } ////// Line break condition after the inline object. /// public override LineBreakCondition BreakAfter { get { return LineBreakCondition.BreakDesired; } } ////// Flag indicates whether inline object has fixed size regardless of where /// it is placed within a line. /// public override bool HasFixedSize { get { // Size of inline object is not dependent on position in the line. return true; } } ////// UIElementIsland representing embedded Element Layout island within content world. /// internal UIElementIsland UIElementIsland { get { return _inlineUIContainer.UIElementIsland; } } ////// Number of text position in the text array occupied by the inline object. /// private readonly int _cch; ////// Text run properties for the inline object. /// private readonly TextRunProperties _textProps; ////// Paragraph - the host of the inline object. /// private readonly TextParagraph _host; ////// Inline UI Container associated with this run client /// private InlineUIContainer _inlineUIContainer; } ////// Floating object run. /// internal sealed class FloatingRun : TextHidden { internal FloatingRun(int length, bool figure) : base(length) { _figure = figure; } internal bool Figure { get { return _figure; } } private readonly bool _figure; } ////// Custom paragraph break run. /// internal sealed class ParagraphBreakRun : TextEndOfParagraph { internal ParagraphBreakRun(int length, PTS.FSFLRES breakReason) : base(length) { BreakReason = breakReason; } internal readonly PTS.FSFLRES BreakReason; } ////// Custom line break run. /// internal sealed class LineBreakRun : TextEndOfLine { internal LineBreakRun(int length, PTS.FSFLRES breakReason) : base(length) { BreakReason = breakReason; } internal readonly PTS.FSFLRES BreakReason; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: RunClient.cs // // Description: RunClient represents opaque data storage associated with runs // consumed by TextFormatter. // // History: // 05/05/2003 : [....] - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; using MS.Internal.Text; using MS.Internal.Documents; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Inline object run. /// internal sealed class InlineObjectRun : TextEmbeddedObject { ////// Constructor. /// /// Number of text position in the text array occupied by the inline object. /// UIElement representing the inline object. /// Text run properties for the inline object. /// Paragraph - the host of the inline object. internal InlineObjectRun(int cch, UIElement element, TextRunProperties textProps, TextParagraph host) { _cch = cch; _textProps = textProps; _host = host; _inlineUIContainer = (InlineUIContainer)LogicalTreeHelper.GetParent(element); } ////// Get inline object's measurement metrics. /// /// Remaining paragraph width. ///Inline object metrics. public override TextEmbeddedObjectMetrics Format(double remainingParagraphWidth) { Size desiredSize = _host.MeasureChild(this); // Make sure that LS/PTS limitations are not exceeded for object's size. TextDpi.EnsureValidObjSize(ref desiredSize); double baseline = desiredSize.Height; double baselineOffsetValue = (double)UIElementIsland.Root.GetValue(TextBlock.BaselineOffsetProperty); if(!DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = baselineOffsetValue; } return new TextEmbeddedObjectMetrics(desiredSize.Width, desiredSize.Height, baseline); } ////// Get computed bounding box of the inline object. /// /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. ///Computed bounding box size of text object. public override Rect ComputeBoundingBox(bool rightToLeft, bool sideways) { // Initially assume that bounding box is the same as layout box. // NOTE: PTS requires bounding box during line formatting. This eventually triggers // TextFormatter to call this function. // But at that time computed size is not available yet. Use desired size instead. Size size = UIElementIsland.Root.DesiredSize; double baseline = !sideways ? size.Height : size.Width; double baselineOffsetValue = (double)UIElementIsland.Root.GetValue(TextBlock.BaselineOffsetProperty); if (!sideways && !DoubleUtil.IsNaN(baselineOffsetValue)) { baseline = (double) baselineOffsetValue; } return new Rect(0, -baseline, sideways ? size.Height : size.Width, sideways ? size.Width : size.Height); } ////// Draw the inline object. /// /// Drawing context. /// Origin where the object is drawn. /// Run is drawn from right to left. /// Run is drawn with its side parallel to baseline. public override void Draw(DrawingContext drawingContext, Point origin, bool rightToLeft, bool sideways) { // Inline object has its own visual and it is attached to a visual // tree during arrange process. // Do nothing here. } ////// Reference to character buffer. /// public override CharacterBufferReference CharacterBufferReference { get { return new CharacterBufferReference(String.Empty, 0); } } ////// Length of the inline object run. /// public override int Length { get { return _cch; } } ////// A set of properties shared by every characters in the run /// public override TextRunProperties Properties { get { return _textProps; } } ////// Line break condition before the inline object. /// public override LineBreakCondition BreakBefore { get { return LineBreakCondition.BreakDesired; } } ////// Line break condition after the inline object. /// public override LineBreakCondition BreakAfter { get { return LineBreakCondition.BreakDesired; } } ////// Flag indicates whether inline object has fixed size regardless of where /// it is placed within a line. /// public override bool HasFixedSize { get { // Size of inline object is not dependent on position in the line. return true; } } ////// UIElementIsland representing embedded Element Layout island within content world. /// internal UIElementIsland UIElementIsland { get { return _inlineUIContainer.UIElementIsland; } } ////// Number of text position in the text array occupied by the inline object. /// private readonly int _cch; ////// Text run properties for the inline object. /// private readonly TextRunProperties _textProps; ////// Paragraph - the host of the inline object. /// private readonly TextParagraph _host; ////// Inline UI Container associated with this run client /// private InlineUIContainer _inlineUIContainer; } ////// Floating object run. /// internal sealed class FloatingRun : TextHidden { internal FloatingRun(int length, bool figure) : base(length) { _figure = figure; } internal bool Figure { get { return _figure; } } private readonly bool _figure; } ////// Custom paragraph break run. /// internal sealed class ParagraphBreakRun : TextEndOfParagraph { internal ParagraphBreakRun(int length, PTS.FSFLRES breakReason) : base(length) { BreakReason = breakReason; } internal readonly PTS.FSFLRES BreakReason; } ////// Custom line break run. /// internal sealed class LineBreakRun : TextEndOfLine { internal LineBreakRun(int length, PTS.FSFLRES breakReason) : base(length) { BreakReason = breakReason; } internal readonly PTS.FSFLRES BreakReason; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
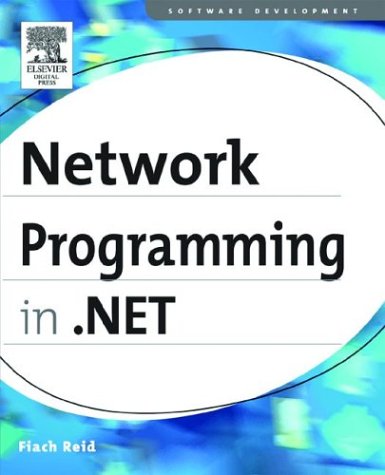
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerRegionCollection.cs
- WebPartPersonalization.cs
- CodeMethodReturnStatement.cs
- TextureBrush.cs
- NameValuePermission.cs
- Bitmap.cs
- CryptoStream.cs
- DbConnectionHelper.cs
- LinkDescriptor.cs
- HGlobalSafeHandle.cs
- XmlUtil.cs
- Localizer.cs
- SelectionHighlightInfo.cs
- GeometryModel3D.cs
- ByteAnimation.cs
- GrammarBuilderBase.cs
- Policy.cs
- DependencyProperty.cs
- ValidatingPropertiesEventArgs.cs
- XmlSchema.cs
- FillRuleValidation.cs
- XmlCDATASection.cs
- IPHostEntry.cs
- sqlcontext.cs
- GridViewRowEventArgs.cs
- ImageClickEventArgs.cs
- ScrollChangedEventArgs.cs
- ResolveMatchesMessageCD1.cs
- CodeAssignStatement.cs
- DBDataPermission.cs
- ComboBoxAutomationPeer.cs
- WindowsClaimSet.cs
- uribuilder.cs
- NamespaceImport.cs
- ObjectViewQueryResultData.cs
- TranslateTransform3D.cs
- SqlInternalConnectionSmi.cs
- securitycriticaldataformultiplegetandset.cs
- QueryGeneratorBase.cs
- DataGridViewCellStyleConverter.cs
- XmlBaseReader.cs
- DataServiceException.cs
- ButtonPopupAdapter.cs
- dataobject.cs
- PanelContainerDesigner.cs
- WebConfigurationHostFileChange.cs
- HMACSHA1.cs
- MsmqMessageProperty.cs
- CodeSnippetExpression.cs
- DataSourceListEditor.cs
- ControlBindingsCollection.cs
- ItemType.cs
- WindowPatternIdentifiers.cs
- CountdownEvent.cs
- ResourceDisplayNameAttribute.cs
- EpmContentDeSerializerBase.cs
- UncommonField.cs
- TextParaClient.cs
- CheckBoxPopupAdapter.cs
- ActiveDocumentEvent.cs
- ReceiveSecurityHeaderEntry.cs
- TextSearch.cs
- ResourceDescriptionAttribute.cs
- CodeObjectCreateExpression.cs
- odbcmetadatacolumnnames.cs
- TraceInternal.cs
- HtmlControlPersistable.cs
- EntityDataSourceContainerNameConverter.cs
- XmlLanguage.cs
- Keyboard.cs
- BindingExpressionUncommonField.cs
- CatchBlock.cs
- ResourcePart.cs
- CheckBox.cs
- Stackframe.cs
- RadioButtonList.cs
- RegexCompilationInfo.cs
- WebPartAuthorizationEventArgs.cs
- IgnoreSectionHandler.cs
- LongCountAggregationOperator.cs
- EntityDataSourceDataSelection.cs
- BitmapMetadataBlob.cs
- HttpListenerException.cs
- WebPartPersonalization.cs
- MulticastIPAddressInformationCollection.cs
- Preprocessor.cs
- XmlSequenceWriter.cs
- XmlDocumentFragment.cs
- GenericEnumerator.cs
- GlyphRunDrawing.cs
- TextMessageEncodingBindingElement.cs
- XamlWrappingReader.cs
- RegistryDataKey.cs
- WebPartPersonalization.cs
- ELinqQueryState.cs
- HostUtils.cs
- HostDesigntimeLicenseContext.cs
- WS2007FederationHttpBindingCollectionElement.cs
- TextRange.cs
- Divide.cs