Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / HGlobalSafeHandle.cs / 1 / HGlobalSafeHandle.cs
//---------------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Stream Helper. // Allocates a global memory buffer to do marshaling between a // binary and a structured data. The global memory size increases and // never shrinks. // using System; using System.Runtime.InteropServices; namespace System.Speech.Internal { ////// Encapsulate SafeHandle for Win32 Memory Handles /// internal sealed class HGlobalSafeHandle : SafeHandle { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal HGlobalSafeHandle () : base (IntPtr.Zero, true) { } // This destructor will run only if the Dispose method // does not get called. ~HGlobalSafeHandle () { Dispose (false); } protected override void Dispose (bool disposing) { ReleaseHandle (); base.Dispose (disposing); GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods ////// /// /// ///internal IntPtr Buffer (int size) { if (size > _bufferSize) { if (_bufferSize == 0) { SetHandle (Marshal.AllocHGlobal (size)); } else { SetHandle (Marshal.ReAllocHGlobal (handle, (IntPtr) size)); } GC.AddMemoryPressure (size - _bufferSize); _bufferSize = size; } return handle; } /// /// True if the no memory is allocated /// ///public override bool IsInvalid { get { return handle == IntPtr.Zero; } } #endregion //******************************************************************** // // Protected Methods // //******************************************************************** #region Protected Methods /// /// Releases the Win32 Memory handle /// ///protected override bool ReleaseHandle () { if (handle != IntPtr.Zero) { // Reset the extra information given to the GC if (_bufferSize > 0) { GC.RemoveMemoryPressure (_bufferSize); _bufferSize = 0; } Marshal.FreeHGlobal (handle); handle = IntPtr.Zero; return true; } return false; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private int _bufferSize; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Stream Helper. // Allocates a global memory buffer to do marshaling between a // binary and a structured data. The global memory size increases and // never shrinks. // using System; using System.Runtime.InteropServices; namespace System.Speech.Internal { ////// Encapsulate SafeHandle for Win32 Memory Handles /// internal sealed class HGlobalSafeHandle : SafeHandle { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal HGlobalSafeHandle () : base (IntPtr.Zero, true) { } // This destructor will run only if the Dispose method // does not get called. ~HGlobalSafeHandle () { Dispose (false); } protected override void Dispose (bool disposing) { ReleaseHandle (); base.Dispose (disposing); GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods ////// /// /// ///internal IntPtr Buffer (int size) { if (size > _bufferSize) { if (_bufferSize == 0) { SetHandle (Marshal.AllocHGlobal (size)); } else { SetHandle (Marshal.ReAllocHGlobal (handle, (IntPtr) size)); } GC.AddMemoryPressure (size - _bufferSize); _bufferSize = size; } return handle; } /// /// True if the no memory is allocated /// ///public override bool IsInvalid { get { return handle == IntPtr.Zero; } } #endregion //******************************************************************** // // Protected Methods // //******************************************************************** #region Protected Methods /// /// Releases the Win32 Memory handle /// ///protected override bool ReleaseHandle () { if (handle != IntPtr.Zero) { // Reset the extra information given to the GC if (_bufferSize > 0) { GC.RemoveMemoryPressure (_bufferSize); _bufferSize = 0; } Marshal.FreeHGlobal (handle); handle = IntPtr.Zero; return true; } return false; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private int _bufferSize; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
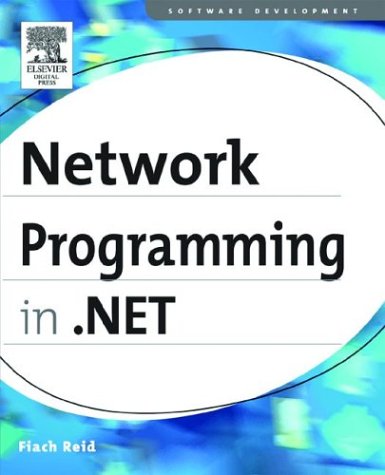
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogRestartAreaEnumerator.cs
- ImageMapEventArgs.cs
- MatrixTransform3D.cs
- _NetRes.cs
- DoubleLinkListEnumerator.cs
- SafeTimerHandle.cs
- IisNotInstalledException.cs
- Buffer.cs
- ObjectMemberMapping.cs
- CharConverter.cs
- HotSpotCollection.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- ImageAttributes.cs
- CompModSwitches.cs
- MergeFilterQuery.cs
- StorageBasedPackageProperties.cs
- TextServicesProperty.cs
- DecimalAnimationUsingKeyFrames.cs
- DebugInfoGenerator.cs
- PresentationSource.cs
- XmlDomTextWriter.cs
- AudienceUriMode.cs
- HttpCookie.cs
- ClickablePoint.cs
- ContainerSelectorActiveEvent.cs
- Context.cs
- NameNode.cs
- RecognizedAudio.cs
- DesignerDataColumn.cs
- SqlConnectionStringBuilder.cs
- VScrollBar.cs
- SupportingTokenBindingElement.cs
- QilInvokeLateBound.cs
- regiisutil.cs
- HostProtectionPermission.cs
- CodeNamespaceImportCollection.cs
- ListCollectionView.cs
- Style.cs
- SQLMembershipProvider.cs
- CategoriesDocumentFormatter.cs
- TextPattern.cs
- assemblycache.cs
- SecurityKeyUsage.cs
- SamlNameIdentifierClaimResource.cs
- CultureSpecificStringDictionary.cs
- CodeMemberMethod.cs
- PeerNeighborManager.cs
- CultureInfoConverter.cs
- XamlPointCollectionSerializer.cs
- VBCodeProvider.cs
- CodeLinePragma.cs
- ImageSourceValueSerializer.cs
- TimeSpan.cs
- ExpanderAutomationPeer.cs
- Timer.cs
- TraceUtility.cs
- PackageProperties.cs
- ColumnMapTranslator.cs
- Set.cs
- UniqueIdentifierService.cs
- CompilationLock.cs
- Switch.cs
- RectValueSerializer.cs
- WindowsContainer.cs
- Latin1Encoding.cs
- DesignerOptions.cs
- MemberInfoSerializationHolder.cs
- HashSet.cs
- ConvertEvent.cs
- QuaternionValueSerializer.cs
- ApplicationHost.cs
- FormView.cs
- FatalException.cs
- TypeUtil.cs
- ConnectionInterfaceCollection.cs
- ScriptingSectionGroup.cs
- IPHostEntry.cs
- ProcessThreadCollection.cs
- BitmapInitialize.cs
- Int16Animation.cs
- InputReferenceExpression.cs
- AutomationElement.cs
- ToolboxItemCollection.cs
- SqlParameter.cs
- Accessors.cs
- XmlSerializerVersionAttribute.cs
- AttachedPropertyBrowsableAttribute.cs
- GenericPrincipal.cs
- Storyboard.cs
- OuterGlowBitmapEffect.cs
- _NTAuthentication.cs
- ExceptionHelpers.cs
- FilteredXmlReader.cs
- PermissionSet.cs
- ComPlusServiceHost.cs
- ToolboxDataAttribute.cs
- StateWorkerRequest.cs
- DoubleCollectionConverter.cs
- RulePatternOps.cs
- SoapProtocolImporter.cs