Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / CodeDOM / CodeNamespaceImportCollection.cs / 1 / CodeNamespaceImportCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using System.Collections; using System.Runtime.InteropServices; using System.Globalization; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeNamespaceImportCollection : IList { private ArrayList data = new ArrayList(); private Hashtable keys = new Hashtable(StringComparer.OrdinalIgnoreCase); ////// Manages a collection of ///objects. /// /// public CodeNamespaceImport this[int index] { get { return ((CodeNamespaceImport)data[index]); } set { data[index] = value; SyncKeys(); } } ////// Indexer method that provides collection access. /// ////// public int Count { get { return data.Count; } } ////// Gets or sets the number of namespaces in the collection. /// ///bool IList.IsReadOnly { get { return false; } } /// bool IList.IsFixedSize { get { return false; } } /// /// public void Add(CodeNamespaceImport value) { if (!keys.ContainsKey(value.Namespace)) { keys[value.Namespace] = value; data.Add(value); } } ////// Adds a namespace import to the collection. /// ////// public void AddRange(CodeNamespaceImport[] value) { if (value == null) { throw new ArgumentNullException("value"); } foreach (CodeNamespaceImport c in value) { Add(c); } } ////// Adds a set of ///objects to the collection. /// /// public void Clear() { data.Clear(); keys.Clear(); } ////// Clears the collection of members. /// ////// private void SyncKeys() { keys = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach(CodeNamespaceImport c in this) { keys[c.Namespace] = c; } } ////// Makes the collection of keys synchronised with the data. /// ////// public IEnumerator GetEnumerator() { return data.GetEnumerator(); } ////// Gets an enumerator that enumerates the collection members. /// ///object IList.this[int index] { get { return this[index]; } set { this[index] = (CodeNamespaceImport)value; SyncKeys(); } } /// int ICollection.Count { get { return Count; } } /// bool ICollection.IsSynchronized { get { return false; } } /// object ICollection.SyncRoot { get { return null; } } /// void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// int IList.Add(object value) { return data.Add((CodeNamespaceImport)value); } /// void IList.Clear() { Clear(); } /// bool IList.Contains(object value) { return data.Contains(value); } /// int IList.IndexOf(object value) { return data.IndexOf((CodeNamespaceImport)value); } /// void IList.Insert(int index, object value) { data.Insert(index, (CodeNamespaceImport)value); SyncKeys(); } /// void IList.Remove(object value) { data.Remove((CodeNamespaceImport)value); SyncKeys(); } /// void IList.RemoveAt(int index) { data.RemoveAt(index); SyncKeys(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using System.Collections; using System.Runtime.InteropServices; using System.Globalization; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeNamespaceImportCollection : IList { private ArrayList data = new ArrayList(); private Hashtable keys = new Hashtable(StringComparer.OrdinalIgnoreCase); ////// Manages a collection of ///objects. /// /// public CodeNamespaceImport this[int index] { get { return ((CodeNamespaceImport)data[index]); } set { data[index] = value; SyncKeys(); } } ////// Indexer method that provides collection access. /// ////// public int Count { get { return data.Count; } } ////// Gets or sets the number of namespaces in the collection. /// ///bool IList.IsReadOnly { get { return false; } } /// bool IList.IsFixedSize { get { return false; } } /// /// public void Add(CodeNamespaceImport value) { if (!keys.ContainsKey(value.Namespace)) { keys[value.Namespace] = value; data.Add(value); } } ////// Adds a namespace import to the collection. /// ////// public void AddRange(CodeNamespaceImport[] value) { if (value == null) { throw new ArgumentNullException("value"); } foreach (CodeNamespaceImport c in value) { Add(c); } } ////// Adds a set of ///objects to the collection. /// /// public void Clear() { data.Clear(); keys.Clear(); } ////// Clears the collection of members. /// ////// private void SyncKeys() { keys = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach(CodeNamespaceImport c in this) { keys[c.Namespace] = c; } } ////// Makes the collection of keys synchronised with the data. /// ////// public IEnumerator GetEnumerator() { return data.GetEnumerator(); } ////// Gets an enumerator that enumerates the collection members. /// ///object IList.this[int index] { get { return this[index]; } set { this[index] = (CodeNamespaceImport)value; SyncKeys(); } } /// int ICollection.Count { get { return Count; } } /// bool ICollection.IsSynchronized { get { return false; } } /// object ICollection.SyncRoot { get { return null; } } /// void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// int IList.Add(object value) { return data.Add((CodeNamespaceImport)value); } /// void IList.Clear() { Clear(); } /// bool IList.Contains(object value) { return data.Contains(value); } /// int IList.IndexOf(object value) { return data.IndexOf((CodeNamespaceImport)value); } /// void IList.Insert(int index, object value) { data.Insert(index, (CodeNamespaceImport)value); SyncKeys(); } /// void IList.Remove(object value) { data.Remove((CodeNamespaceImport)value); SyncKeys(); } /// void IList.RemoveAt(int index) { data.RemoveAt(index); SyncKeys(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
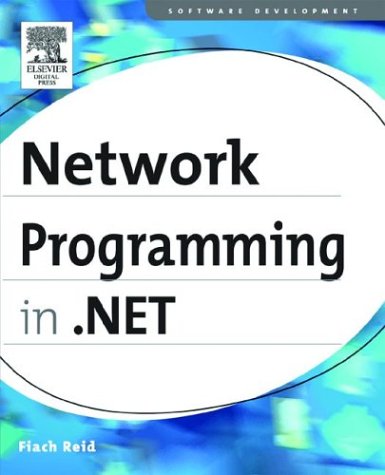
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeBuilder.cs
- SqlRecordBuffer.cs
- NetworkCredential.cs
- MasterPage.cs
- RepeaterItemCollection.cs
- BitmapEffectDrawingContextWalker.cs
- BookmarkManager.cs
- ResourceContainer.cs
- AutoCompleteStringCollection.cs
- MetaForeignKeyColumn.cs
- DataGridViewToolTip.cs
- IItemContainerGenerator.cs
- TableLayoutSettings.cs
- HostingEnvironmentException.cs
- TextTreeObjectNode.cs
- Thumb.cs
- TextEditorTables.cs
- ResourceReferenceExpression.cs
- GeometryGroup.cs
- FloaterParagraph.cs
- DrawingState.cs
- CompilerHelpers.cs
- FileSystemInfo.cs
- WebPartUserCapability.cs
- ServiceOperationWrapper.cs
- ThreadAbortException.cs
- SelectionItemPattern.cs
- StreamResourceInfo.cs
- BehaviorEditorPart.cs
- HtmlInputRadioButton.cs
- InkCanvas.cs
- ExeConfigurationFileMap.cs
- RemotingSurrogateSelector.cs
- WebPartZone.cs
- ChangeBlockUndoRecord.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- NamespaceInfo.cs
- TransactionScope.cs
- DateTime.cs
- RemoteWebConfigurationHostServer.cs
- HtmlMeta.cs
- TextFormattingConverter.cs
- DataTableCollection.cs
- XPathConvert.cs
- Gdiplus.cs
- QualifierSet.cs
- TextBreakpoint.cs
- BitmapEffectInputData.cs
- SqlFunctionAttribute.cs
- SiteIdentityPermission.cs
- CryptoHelper.cs
- HtmlTitle.cs
- RemoteCryptoTokenProvider.cs
- SecurityContext.cs
- SelectionHighlightInfo.cs
- PersonalizableTypeEntry.cs
- MetricEntry.cs
- NameNode.cs
- TransformDescriptor.cs
- DependencyObjectType.cs
- TreeView.cs
- GestureRecognizer.cs
- TrustLevel.cs
- ListControlStringCollectionEditor.cs
- DigitShape.cs
- TextParagraphView.cs
- SettingsPropertyValueCollection.cs
- SvcMapFile.cs
- ThreadPoolTaskScheduler.cs
- RelativeSource.cs
- VerificationAttribute.cs
- PropertyValueChangedEvent.cs
- CheckBoxField.cs
- InsufficientMemoryException.cs
- BamlRecordReader.cs
- FixedSOMTextRun.cs
- SqlDataSourceQueryEditorForm.cs
- DeferredTextReference.cs
- DispatcherHooks.cs
- cookiecontainer.cs
- SchemaTableColumn.cs
- SQLRoleProvider.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- TemplateBindingExtension.cs
- AuthenticatedStream.cs
- XmlProcessingInstruction.cs
- MarginsConverter.cs
- SpeechSynthesizer.cs
- DoubleCollectionConverter.cs
- GetParentChain.cs
- SqlConnectionManager.cs
- RemoteWebConfigurationHost.cs
- DBConnectionString.cs
- smtpconnection.cs
- QueryOptionExpression.cs
- AliasGenerator.cs
- RegexTree.cs
- CharacterString.cs
- DocumentAutomationPeer.cs
- DesignerVerbCollection.cs