Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / AutoCompleteStringCollection.cs / 1305376 / AutoCompleteStringCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Collections; using System.Security.Permissions; using System.ComponentModel; ////// /// public class AutoCompleteStringCollection : IList { CollectionChangeEventHandler onCollectionChanged; private ArrayList data = new ArrayList(); public AutoCompleteStringCollection() { } ///Represents a collection of strings. ////// /// public string this[int index] { get { return ((string)data[index]); } set { OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, data[index])); data[index] = value; OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, value)); } } ///Represents the entry at the specified index of the ///. /// /// public int Count { get { return data.Count; } } ///Gets the number of strings in the /// ///. bool IList.IsReadOnly { get { return false; } } /// bool IList.IsFixedSize { get { return false; } } /// /// /// public event CollectionChangeEventHandler CollectionChanged { add { this.onCollectionChanged += value; } remove { this.onCollectionChanged -= value; } } ///[To be supplied.] ////// /// protected void OnCollectionChanged(CollectionChangeEventArgs e) { if (this.onCollectionChanged != null) { this.onCollectionChanged(this, e); } } ///[To be supplied.] ////// /// public int Add(string value) { int index = data.Add(value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, value)); return index; } ///Adds a string with the specified value to the /// ///. /// /// public void AddRange(string[] value) { if (value == null) { throw new ArgumentNullException("value"); } data.AddRange(value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///Copies the elements of a string array to the end of the ///. /// /// public void Clear() { data.Clear(); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///Removes all the strings from the /// ///. /// /// public bool Contains(string value) { return data.Contains(value); } ///Gets a value indicating whether the /// ///contains a string with the specified /// value. /// /// public void CopyTo(string[] array, int index) { data.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// /// public int IndexOf(string value) { return data.IndexOf(value); } ///Returns the index of the first occurrence of a string in /// the ///. /// /// public void Insert(int index, string value) { data.Insert(index, value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, value)); } ///Inserts a string into the ///at the specified /// index. /// /// public bool IsReadOnly { get { return false; } } ///Gets a value indicating whether the ///is read-only. /// /// public bool IsSynchronized { get { return false; } } ///Gets a value indicating whether access to the /// ////// is synchronized (thread-safe). /// /// public void Remove(string value) { data.Remove(value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, value)); } ///Removes a specific string from the /// ///. /// /// public void RemoveAt(int index) { string value = (string)data[index]; data.RemoveAt(index); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, value)); } ///Removes the string at the specified index of the ///. /// /// public object SyncRoot { [HostProtection(Synchronization=true)] [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] get { return this; } } ///Gets an object that can be used to synchronize access to the ///. object IList.this[int index] { get { return this[index]; } set { this[index] = (string)value; } } /// int IList.Add(object value) { return Add((string)value); } /// bool IList.Contains(object value) { return Contains((string) value); } /// int IList.IndexOf(object value) { return IndexOf((string)value); } /// void IList.Insert(int index, object value) { Insert(index, (string)value); } /// void IList.Remove(object value) { Remove((string)value); } /// void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } /// public IEnumerator GetEnumerator() { return data.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Collections; using System.Security.Permissions; using System.ComponentModel; ////// /// public class AutoCompleteStringCollection : IList { CollectionChangeEventHandler onCollectionChanged; private ArrayList data = new ArrayList(); public AutoCompleteStringCollection() { } ///Represents a collection of strings. ////// /// public string this[int index] { get { return ((string)data[index]); } set { OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, data[index])); data[index] = value; OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, value)); } } ///Represents the entry at the specified index of the ///. /// /// public int Count { get { return data.Count; } } ///Gets the number of strings in the /// ///. bool IList.IsReadOnly { get { return false; } } /// bool IList.IsFixedSize { get { return false; } } /// /// /// public event CollectionChangeEventHandler CollectionChanged { add { this.onCollectionChanged += value; } remove { this.onCollectionChanged -= value; } } ///[To be supplied.] ////// /// protected void OnCollectionChanged(CollectionChangeEventArgs e) { if (this.onCollectionChanged != null) { this.onCollectionChanged(this, e); } } ///[To be supplied.] ////// /// public int Add(string value) { int index = data.Add(value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, value)); return index; } ///Adds a string with the specified value to the /// ///. /// /// public void AddRange(string[] value) { if (value == null) { throw new ArgumentNullException("value"); } data.AddRange(value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///Copies the elements of a string array to the end of the ///. /// /// public void Clear() { data.Clear(); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///Removes all the strings from the /// ///. /// /// public bool Contains(string value) { return data.Contains(value); } ///Gets a value indicating whether the /// ///contains a string with the specified /// value. /// /// public void CopyTo(string[] array, int index) { data.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// /// public int IndexOf(string value) { return data.IndexOf(value); } ///Returns the index of the first occurrence of a string in /// the ///. /// /// public void Insert(int index, string value) { data.Insert(index, value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, value)); } ///Inserts a string into the ///at the specified /// index. /// /// public bool IsReadOnly { get { return false; } } ///Gets a value indicating whether the ///is read-only. /// /// public bool IsSynchronized { get { return false; } } ///Gets a value indicating whether access to the /// ////// is synchronized (thread-safe). /// /// public void Remove(string value) { data.Remove(value); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, value)); } ///Removes a specific string from the /// ///. /// /// public void RemoveAt(int index) { string value = (string)data[index]; data.RemoveAt(index); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, value)); } ///Removes the string at the specified index of the ///. /// /// public object SyncRoot { [HostProtection(Synchronization=true)] [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] get { return this; } } ///Gets an object that can be used to synchronize access to the ///. object IList.this[int index] { get { return this[index]; } set { this[index] = (string)value; } } /// int IList.Add(object value) { return Add((string)value); } /// bool IList.Contains(object value) { return Contains((string) value); } /// int IList.IndexOf(object value) { return IndexOf((string)value); } /// void IList.Insert(int index, object value) { Insert(index, (string)value); } /// void IList.Remove(object value) { Remove((string)value); } /// void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } /// public IEnumerator GetEnumerator() { return data.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
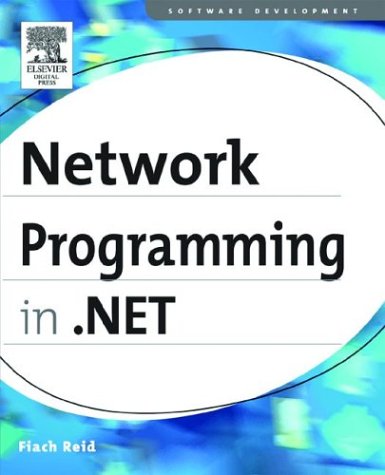
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InheritanceContextHelper.cs
- ColumnHeaderConverter.cs
- safelink.cs
- AccessDataSource.cs
- SimpleWorkerRequest.cs
- LoadGrammarCompletedEventArgs.cs
- XPathAncestorIterator.cs
- NotSupportedException.cs
- BuildResultCache.cs
- EnvelopedPkcs7.cs
- IItemProperties.cs
- StyleCollection.cs
- __FastResourceComparer.cs
- DefaultBindingPropertyAttribute.cs
- __Filters.cs
- TrustLevelCollection.cs
- FunctionDetailsReader.cs
- QilName.cs
- TrackPoint.cs
- UInt64Converter.cs
- ConnectionManagementElementCollection.cs
- CodeStatementCollection.cs
- WindowsListViewItemStartMenu.cs
- DataGridAutoFormat.cs
- CombinedGeometry.cs
- AttachmentCollection.cs
- StringInfo.cs
- NegatedCellConstant.cs
- DataGridColumnHeaderCollection.cs
- RangeValidator.cs
- UrlMappingsModule.cs
- Variable.cs
- EncryptedHeaderXml.cs
- Geometry3D.cs
- RightsManagementEncryptedStream.cs
- ErrorWebPart.cs
- Int32AnimationBase.cs
- SqlComparer.cs
- IISMapPath.cs
- IdentityReference.cs
- MetadataItemCollectionFactory.cs
- ProfessionalColors.cs
- ConditionalAttribute.cs
- PageContentAsyncResult.cs
- CollectionConverter.cs
- AlphabeticalEnumConverter.cs
- WebPartConnectionsDisconnectVerb.cs
- PropertyReference.cs
- BehaviorEditorPart.cs
- BindToObject.cs
- PropertyValueChangedEvent.cs
- EventsTab.cs
- ToolStripOverflowButton.cs
- VirtualPathProvider.cs
- KeyPressEvent.cs
- FormatterServices.cs
- OrderingInfo.cs
- RoleBoolean.cs
- Point3DValueSerializer.cs
- SerializationSectionGroup.cs
- UserControlParser.cs
- DataServiceBuildProvider.cs
- GlyphRun.cs
- PermissionSetTriple.cs
- RenderDataDrawingContext.cs
- LocatorGroup.cs
- SettingsAttributeDictionary.cs
- DiagnosticTrace.cs
- QilVisitor.cs
- MasterPage.cs
- ScriptRegistrationManager.cs
- versioninfo.cs
- HyperLinkField.cs
- WindowsButton.cs
- GroupBoxAutomationPeer.cs
- ApplicationManager.cs
- Roles.cs
- SequenceFullException.cs
- IndicCharClassifier.cs
- AllMembershipCondition.cs
- sqlpipe.cs
- TrustManagerPromptUI.cs
- GeneralTransformCollection.cs
- BufferedGraphicsContext.cs
- EarlyBoundInfo.cs
- HtmlTableRowCollection.cs
- RelationshipDetailsCollection.cs
- TransactedReceiveScope.cs
- BitArray.cs
- CodeTypeReference.cs
- FixedPageProcessor.cs
- Rectangle.cs
- FormatVersion.cs
- HtmlFormParameterReader.cs
- WasHttpModulesInstallComponent.cs
- GeneralTransform3D.cs
- HatchBrush.cs
- CheckBox.cs
- CodeIndexerExpression.cs
- EntryIndex.cs