Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / NegatedCellConstant.cs / 1 / NegatedCellConstant.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common.Utils; using System.Text; using System.Diagnostics; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.Structures { // A class that represents NOT(elements), e.g., NOT(1, 2, NULL), i.e., // all values other than null, 1 and 2 internal class NegatedCellConstant : CellConstant { #region Constructors // effects: Creates NOT(NULL} internal static NegatedCellConstant CreateNotNull() { NegatedCellConstant result = new NegatedCellConstant(); result.m_negatedDomain.Add(CellConstant.Null); return result; } // effects: Creates an empty domain private NegatedCellConstant() { m_negatedDomain = new Set(CellConstant.EqualityComparer); } // requires: values has no NegatedCellConstant // effects: Creates a negated constant with the values in it internal NegatedCellConstant(IEnumerable values) { m_negatedDomain = new Set (values, CellConstant.EqualityComparer); Debug.Assert(CheckRepInvariantLocal()); } #endregion #region Fields private Set m_negatedDomain; // e.g., NOT(1, 2, Undefined) #endregion #region Properties internal IEnumerable Elements { get { return m_negatedDomain;} } #endregion #region Methods // effects: Returns true if this contains "constant" internal bool Contains(CellConstant constant) { return m_negatedDomain.Contains(constant); } // effects: Returns true if this represents "NotNull" precisely internal override bool IsNotNull() { return m_negatedDomain.Count == 1 && m_negatedDomain.Contains(CellConstant.Null); } // effects: Returns true if this contains "NotNull" internal override bool HasNotNull() { return m_negatedDomain.Contains(CellConstant.Null); } internal override bool CheckRepInvariant() { return CheckRepInvariantLocal(); } // effects: Make sure that this negated constant's invariants are valid internal bool CheckRepInvariantLocal() { // NOT cannot contain negated cell constants foreach (CellConstant constant in Elements) { Debug.Assert(constant as NegatedCellConstant == null, "NegatedCellConstant in NegatedCellConstant?"); } return true; } // effects: Returns a hash value for this protected override int GetHash() { int result = 0; foreach (CellConstant constant in m_negatedDomain) { result ^= CellConstant.EqualityComparer.GetHashCode(constant); } return result; } // effects: Returns true if right has the same contents as this protected override bool IsEqualTo(CellConstant right) { NegatedCellConstant rightNegatedConstant = right as NegatedCellConstant; if (rightNegatedConstant == null) { return false; } return m_negatedDomain.SetEquals(rightNegatedConstant.m_negatedDomain); } // effects: Given a set of positive constants (constants) and this, // generates a Cql expression, e.g., 7, NOT(7, NULL) means NOT NULL // 7, 8, NOT(7, 8, 9, 10) Means NOT(9, 10) // Note: NOT(9, NULL) is NOT (a is 9 OR a is NULL) = // A IS NOT 9 AND A IS NOT NULL internal StringBuilder AsCql(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, false); } // effects: Given a set of constants, generates "Slot = X AND Slot = Y" or "Slot <> X AND Slot <> Y" (depending ifGenerateEquals) private static void GenerateAndsForSlot(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool anyAdded, bool userString) { foreach (CellConstant constant in constants) { if (anyAdded) { builder.Append(" AND "); } anyAdded = true; if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" <>"); constant.ToCompactString(builder); } else { path.AsCql(builder, blockAlias); builder.Append(" <>"); constant.AsCql(builder, path, blockAlias); } } } private StringBuilder ToStringHelper(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull, bool userString) { bool isNullable = path.IsNullable; // Remove all the constants from negated and then print NOT // .. AND NOT .. AND NOT ... Set negatedConstants = new Set (Elements, CellConstant.EqualityComparer); foreach (CellConstant constant in constants) { if (constant.Equals(this)) { continue; } Debug.Assert(negatedConstants.Contains(constant), "Negated constant must contain all positive constants"); negatedConstants.Remove(constant); } if (negatedConstants.Count == 0) { // All constants cancel out builder.Append("true"); return builder; } bool hasNull = negatedConstants.Contains(CellConstant.Null); negatedConstants.Remove(CellConstant.Null); // We always add IS NOT NULL if the property is nullable (and we // cannot skip IS NOT NULL). Also, if the domain contains NOT // NULL, we must add it bool anyAdded = false; if (hasNull || (isNullable && canSkipIsNotNull == false)) { if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" is not NULL"); } else { path.AsCql(builder, blockAlias); builder.Append(" IS NOT NULL"); } anyAdded = true; } GenerateAndsForSlot(builder, blockAlias, negatedConstants, path, anyAdded, userString); return builder; } internal StringBuilder AsUserString(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, true); } internal override string ToUserString() { if (IsNotNull()) { return System.Data.Entity.Strings.ViewGen_NotNull; } else { StringBuilder builder = new StringBuilder(); bool isFirst = true; foreach (CellConstant constant in m_negatedDomain) { // Skip printing out Null if m_negatedDomain has other values if (m_negatedDomain.Count > 1 && constant.IsNull()) { continue; } if (isFirst == false) { builder.Append(System.Data.Entity.Strings.ViewGen_CommaBlank); } isFirst = false; builder.Append(constant.ToUserString()); } StringBuilder result = new StringBuilder(); result.Append(Strings.ViewGen_NegatedCellConstant_0(builder.ToString())); return result.ToString(); } } internal override void ToCompactString(StringBuilder builder) { // Special code for NOT NULL if (IsNotNull()) { builder.Append("NOT_NULL"); } else { builder.Append("NOT("); StringUtil.ToCommaSeparatedStringSorted(builder, m_negatedDomain); builder.Append(")"); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common.Utils; using System.Text; using System.Diagnostics; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.Structures { // A class that represents NOT(elements), e.g., NOT(1, 2, NULL), i.e., // all values other than null, 1 and 2 internal class NegatedCellConstant : CellConstant { #region Constructors // effects: Creates NOT(NULL} internal static NegatedCellConstant CreateNotNull() { NegatedCellConstant result = new NegatedCellConstant(); result.m_negatedDomain.Add(CellConstant.Null); return result; } // effects: Creates an empty domain private NegatedCellConstant() { m_negatedDomain = new Set(CellConstant.EqualityComparer); } // requires: values has no NegatedCellConstant // effects: Creates a negated constant with the values in it internal NegatedCellConstant(IEnumerable values) { m_negatedDomain = new Set (values, CellConstant.EqualityComparer); Debug.Assert(CheckRepInvariantLocal()); } #endregion #region Fields private Set m_negatedDomain; // e.g., NOT(1, 2, Undefined) #endregion #region Properties internal IEnumerable Elements { get { return m_negatedDomain;} } #endregion #region Methods // effects: Returns true if this contains "constant" internal bool Contains(CellConstant constant) { return m_negatedDomain.Contains(constant); } // effects: Returns true if this represents "NotNull" precisely internal override bool IsNotNull() { return m_negatedDomain.Count == 1 && m_negatedDomain.Contains(CellConstant.Null); } // effects: Returns true if this contains "NotNull" internal override bool HasNotNull() { return m_negatedDomain.Contains(CellConstant.Null); } internal override bool CheckRepInvariant() { return CheckRepInvariantLocal(); } // effects: Make sure that this negated constant's invariants are valid internal bool CheckRepInvariantLocal() { // NOT cannot contain negated cell constants foreach (CellConstant constant in Elements) { Debug.Assert(constant as NegatedCellConstant == null, "NegatedCellConstant in NegatedCellConstant?"); } return true; } // effects: Returns a hash value for this protected override int GetHash() { int result = 0; foreach (CellConstant constant in m_negatedDomain) { result ^= CellConstant.EqualityComparer.GetHashCode(constant); } return result; } // effects: Returns true if right has the same contents as this protected override bool IsEqualTo(CellConstant right) { NegatedCellConstant rightNegatedConstant = right as NegatedCellConstant; if (rightNegatedConstant == null) { return false; } return m_negatedDomain.SetEquals(rightNegatedConstant.m_negatedDomain); } // effects: Given a set of positive constants (constants) and this, // generates a Cql expression, e.g., 7, NOT(7, NULL) means NOT NULL // 7, 8, NOT(7, 8, 9, 10) Means NOT(9, 10) // Note: NOT(9, NULL) is NOT (a is 9 OR a is NULL) = // A IS NOT 9 AND A IS NOT NULL internal StringBuilder AsCql(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, false); } // effects: Given a set of constants, generates "Slot = X AND Slot = Y" or "Slot <> X AND Slot <> Y" (depending ifGenerateEquals) private static void GenerateAndsForSlot(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool anyAdded, bool userString) { foreach (CellConstant constant in constants) { if (anyAdded) { builder.Append(" AND "); } anyAdded = true; if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" <>"); constant.ToCompactString(builder); } else { path.AsCql(builder, blockAlias); builder.Append(" <>"); constant.AsCql(builder, path, blockAlias); } } } private StringBuilder ToStringHelper(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull, bool userString) { bool isNullable = path.IsNullable; // Remove all the constants from negated and then print NOT // .. AND NOT .. AND NOT ... Set negatedConstants = new Set (Elements, CellConstant.EqualityComparer); foreach (CellConstant constant in constants) { if (constant.Equals(this)) { continue; } Debug.Assert(negatedConstants.Contains(constant), "Negated constant must contain all positive constants"); negatedConstants.Remove(constant); } if (negatedConstants.Count == 0) { // All constants cancel out builder.Append("true"); return builder; } bool hasNull = negatedConstants.Contains(CellConstant.Null); negatedConstants.Remove(CellConstant.Null); // We always add IS NOT NULL if the property is nullable (and we // cannot skip IS NOT NULL). Also, if the domain contains NOT // NULL, we must add it bool anyAdded = false; if (hasNull || (isNullable && canSkipIsNotNull == false)) { if (userString) { path.ToCompactString(builder, blockAlias); builder.Append(" is not NULL"); } else { path.AsCql(builder, blockAlias); builder.Append(" IS NOT NULL"); } anyAdded = true; } GenerateAndsForSlot(builder, blockAlias, negatedConstants, path, anyAdded, userString); return builder; } internal StringBuilder AsUserString(StringBuilder builder, string blockAlias, Set constants, MemberPath path, bool canSkipIsNotNull) { return ToStringHelper(builder, blockAlias, constants, path, canSkipIsNotNull, true); } internal override string ToUserString() { if (IsNotNull()) { return System.Data.Entity.Strings.ViewGen_NotNull; } else { StringBuilder builder = new StringBuilder(); bool isFirst = true; foreach (CellConstant constant in m_negatedDomain) { // Skip printing out Null if m_negatedDomain has other values if (m_negatedDomain.Count > 1 && constant.IsNull()) { continue; } if (isFirst == false) { builder.Append(System.Data.Entity.Strings.ViewGen_CommaBlank); } isFirst = false; builder.Append(constant.ToUserString()); } StringBuilder result = new StringBuilder(); result.Append(Strings.ViewGen_NegatedCellConstant_0(builder.ToString())); return result.ToString(); } } internal override void ToCompactString(StringBuilder builder) { // Special code for NOT NULL if (IsNotNull()) { builder.Append("NOT_NULL"); } else { builder.Append("NOT("); StringUtil.ToCommaSeparatedStringSorted(builder, m_negatedDomain); builder.Append(")"); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
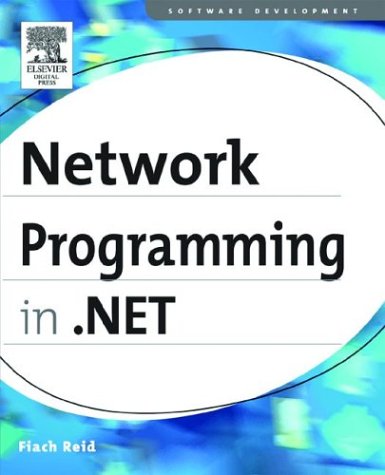
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogQuery.cs
- IApplicationTrustManager.cs
- XmlFormatMapping.cs
- AsyncPostBackTrigger.cs
- XmlSchemaSimpleContentExtension.cs
- TabControlAutomationPeer.cs
- sqlpipe.cs
- WinEventQueueItem.cs
- TypedLocationWrapper.cs
- WebConfigurationManager.cs
- GenericParameterDataContract.cs
- SystemIPv4InterfaceProperties.cs
- Itemizer.cs
- TabRenderer.cs
- HtmlGenericControl.cs
- ObjectDataSourceSelectingEventArgs.cs
- TransformGroup.cs
- MarkupObject.cs
- RemotingSurrogateSelector.cs
- PackagePart.cs
- WorkflowApplicationCompletedException.cs
- Style.cs
- CacheDependency.cs
- WebResponse.cs
- CommonObjectSecurity.cs
- ProfileManager.cs
- XmlWriterTraceListener.cs
- List.cs
- HtmlFormParameterReader.cs
- GridViewCancelEditEventArgs.cs
- TranslateTransform3D.cs
- Canvas.cs
- DATA_BLOB.cs
- LabelEditEvent.cs
- ExpressionEditorAttribute.cs
- ThemeableAttribute.cs
- LogicalMethodInfo.cs
- SEHException.cs
- AmbientLight.cs
- InvalidComObjectException.cs
- ConnectionManagementElementCollection.cs
- TextRangeProviderWrapper.cs
- PageCatalogPart.cs
- Rect3D.cs
- CssStyleCollection.cs
- ClientProxyGenerator.cs
- TextRangeSerialization.cs
- TypeUsage.cs
- TextDecorationCollectionConverter.cs
- InstanceData.cs
- LinqDataSourceUpdateEventArgs.cs
- SaveWorkflowAsyncResult.cs
- SizeIndependentAnimationStorage.cs
- DoubleLink.cs
- CurrentChangingEventArgs.cs
- DataGridViewHitTestInfo.cs
- ChtmlTextWriter.cs
- RepeatButtonAutomationPeer.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- QilStrConcat.cs
- SettingsPropertyValueCollection.cs
- IsolatedStorage.cs
- KeyValueConfigurationCollection.cs
- CaseInsensitiveHashCodeProvider.cs
- GrammarBuilderPhrase.cs
- shaperfactoryquerycacheentry.cs
- ApplicationTrust.cs
- OrderablePartitioner.cs
- QueryableDataSourceView.cs
- CorrelationResolver.cs
- ValueType.cs
- ImageListUtils.cs
- TemplateControlBuildProvider.cs
- BuiltInExpr.cs
- HitTestParameters3D.cs
- ActivationArguments.cs
- HyperlinkAutomationPeer.cs
- SystemMulticastIPAddressInformation.cs
- SchemaMapping.cs
- _ProxyRegBlob.cs
- EdmToObjectNamespaceMap.cs
- CodeRemoveEventStatement.cs
- ExpandCollapsePattern.cs
- recordstatescratchpad.cs
- Enum.cs
- DesignTimeTemplateParser.cs
- GcHandle.cs
- LambdaCompiler.Binary.cs
- InternalConfigRoot.cs
- SecurityCapabilities.cs
- WebBodyFormatMessageProperty.cs
- ProcessHostServerConfig.cs
- HttpConfigurationSystem.cs
- RoleGroupCollection.cs
- FlowLayoutSettings.cs
- HtmlInputControl.cs
- CallContext.cs
- CreateUserWizard.cs
- ChooseAction.cs
- TiffBitmapDecoder.cs