Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / TransformGroup.cs / 1305600 / TransformGroup.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: TransformGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region TransformGroup ////// The class definition for TransformGroup /// [ContentProperty("Children")] public sealed partial class TransformGroup : Transform { #region Constructors ////// Default Constructor /// public TransformGroup() { } #endregion #region Value ////// Return the current transformation value. /// public override Matrix Value { get { ReadPreamble(); TransformCollection children = Children; if ((children == null) || (children.Count == 0)) { return new Matrix(); } Matrix transform = children.Internal_GetItem(0).Value; for (int i = 1; i < children.Count; i++) { transform *= children.Internal_GetItem(i).Value; } return transform; } } #endregion #region IsIdentity ////// Returns true if transformation matches the identity transform. /// internal override bool IsIdentity { get { TransformCollection children = Children; if ((children == null) || (children.Count == 0)) { return true; } for (int i = 0; i < children.Count; i++) { if (!children.Internal_GetItem(i).IsIdentity) { return false; } } return true; } } #endregion #region Internal Methods internal override bool CanSerializeToString() { return false; } #endregion Internal Methods } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
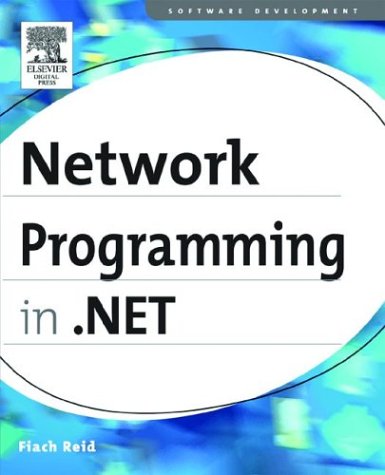
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlnsDictionary.cs
- assemblycache.cs
- WebHttpSecurity.cs
- HandleCollector.cs
- PermissionRequestEvidence.cs
- IntellisenseTextBox.cs
- _StreamFramer.cs
- Underline.cs
- Color.cs
- Assembly.cs
- RenderData.cs
- ItemCheckedEvent.cs
- TrackBar.cs
- IncrementalCompileAnalyzer.cs
- WebPermission.cs
- SqlOuterApplyReducer.cs
- WebPartDisplayMode.cs
- NativeMethods.cs
- DrawingGroup.cs
- DataFormats.cs
- WebPartMenu.cs
- TextProviderWrapper.cs
- SettingsContext.cs
- InternalTypeHelper.cs
- SystemBrushes.cs
- TextBoxRenderer.cs
- PropertyPathWorker.cs
- SqlBooleanizer.cs
- ScriptControlManager.cs
- LambdaCompiler.Lambda.cs
- ProcessHostFactoryHelper.cs
- ScriptManager.cs
- Highlights.cs
- ThreadSafeList.cs
- EntityCommandDefinition.cs
- ObjectViewListener.cs
- ParamArrayAttribute.cs
- ipaddressinformationcollection.cs
- PackWebResponse.cs
- AppDomain.cs
- PolyLineSegmentFigureLogic.cs
- exports.cs
- MenuCommandsChangedEventArgs.cs
- TextSelection.cs
- CodeDomSerializer.cs
- DbDataSourceEnumerator.cs
- NamespaceList.cs
- DoubleAnimationClockResource.cs
- Style.cs
- Facet.cs
- Pointer.cs
- SoapBinding.cs
- DocumentPageView.cs
- ApplicationContext.cs
- EndOfStreamException.cs
- MetadataPropertyAttribute.cs
- TableStyle.cs
- PowerStatus.cs
- ProtocolsConfigurationEntry.cs
- SerializationAttributes.cs
- SessionStateContainer.cs
- elementinformation.cs
- Section.cs
- MarkupWriter.cs
- WpfKnownMemberInvoker.cs
- ServicesUtilities.cs
- IdnMapping.cs
- EntityWithKeyStrategy.cs
- Int64Converter.cs
- XmlNodeReader.cs
- LayoutEngine.cs
- Base64Encoder.cs
- __FastResourceComparer.cs
- BamlTreeNode.cs
- Collection.cs
- DocumentSequence.cs
- EventBuilder.cs
- TrackingProfileSerializer.cs
- BaseParaClient.cs
- GridEntryCollection.cs
- GraphicsContainer.cs
- InheritanceContextHelper.cs
- DefaultProxySection.cs
- IisTraceListener.cs
- NumberFormatInfo.cs
- querybuilder.cs
- FormClosedEvent.cs
- __Filters.cs
- XMLUtil.cs
- ReadOnlyDataSourceView.cs
- Int16Animation.cs
- Wrapper.cs
- CompiledRegexRunnerFactory.cs
- DeviceContext.cs
- WindowsPrincipal.cs
- ClientSideProviderDescription.cs
- SafeNativeMethods.cs
- FrameworkContentElementAutomationPeer.cs
- Size3DConverter.cs
- SolidBrush.cs