Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / NetworkInformation / SystemMulticastIPAddressInformation.cs / 1 / SystemMulticastIPAddressInformation.cs
////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; /// /// Provides support for ip configuation information and statistics. /// /// Specifies the Multicast addresses for an interface. ///OS < XP ////// internal class SystemMulticastIPAddressInformation:MulticastIPAddressInformation { private IpAdapterAddress adapterAddress; private SystemIPAddressInformation innerInfo; private SystemMulticastIPAddressInformation() { } /* // Consider removing. internal SystemMulticastIPAddressInformation(IpAdapterInfo ipAdapterInfo, IPAddress address){ innerInfo = new SystemIPAddressInformation(address); DateTime tempdate = new DateTime(1970,1,1); tempdate = tempdate.AddSeconds(ipAdapterInfo.leaseExpires); dhcpLeaseLifetime = (long)((tempdate - DateTime.UtcNow).TotalSeconds); } */ internal SystemMulticastIPAddressInformation(IpAdapterAddress adapterAddress, IPAddress ipAddress){ innerInfo = new SystemIPAddressInformation(adapterAddress,ipAddress); this.adapterAddress = adapterAddress; } ///public override IPAddress Address{get {return innerInfo.Address;}} /// /// The address is a cluster address and shouldn't be used by most applications. public override bool IsTransient{ get { return (innerInfo.IsTransient); } } ////// This address can be used for DNS. public override bool IsDnsEligible{ get { return (innerInfo.IsDnsEligible); } } ///public override PrefixOrigin PrefixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return PrefixOrigin.Other; } } /// public override SuffixOrigin SuffixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return SuffixOrigin.Other; } } /// /// IPv6 only. Specifies the duplicate address detection state. Only supported /// for IPv6. If called on an IPv4 address, will throw a "not supported" exception. public override DuplicateAddressDetectionState DuplicateAddressDetectionState{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return DuplicateAddressDetectionState.Invalid; } } ////// Specifies the valid lifetime of the address in seconds. public override long AddressValidLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return 0; } } ////// Specifies the prefered lifetime of the address in seconds. public override long AddressPreferredLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return 0; } } ////// /// Specifies the prefered lifetime of the address in seconds. public override long DhcpLeaseLifetime{ get { return 0; } } //helper method that marshals the addressinformation into the classes internal static MulticastIPAddressInformationCollection ToAddressInformationCollection(IntPtr ptr) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. MulticastIPAddressInformationCollection addressList = new MulticastIPAddressInformationCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterAddress addr = (IpAdapterAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(new SystemMulticastIPAddressInformation(addr,ep.Address)); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(new SystemMulticastIPAddressInformation(addr,ep.Address)); } return addressList; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. ////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; /// /// Provides support for ip configuation information and statistics. /// /// Specifies the Multicast addresses for an interface. ///OS < XP ////// internal class SystemMulticastIPAddressInformation:MulticastIPAddressInformation { private IpAdapterAddress adapterAddress; private SystemIPAddressInformation innerInfo; private SystemMulticastIPAddressInformation() { } /* // Consider removing. internal SystemMulticastIPAddressInformation(IpAdapterInfo ipAdapterInfo, IPAddress address){ innerInfo = new SystemIPAddressInformation(address); DateTime tempdate = new DateTime(1970,1,1); tempdate = tempdate.AddSeconds(ipAdapterInfo.leaseExpires); dhcpLeaseLifetime = (long)((tempdate - DateTime.UtcNow).TotalSeconds); } */ internal SystemMulticastIPAddressInformation(IpAdapterAddress adapterAddress, IPAddress ipAddress){ innerInfo = new SystemIPAddressInformation(adapterAddress,ipAddress); this.adapterAddress = adapterAddress; } ///public override IPAddress Address{get {return innerInfo.Address;}} /// /// The address is a cluster address and shouldn't be used by most applications. public override bool IsTransient{ get { return (innerInfo.IsTransient); } } ////// This address can be used for DNS. public override bool IsDnsEligible{ get { return (innerInfo.IsDnsEligible); } } ///public override PrefixOrigin PrefixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return PrefixOrigin.Other; } } /// public override SuffixOrigin SuffixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return SuffixOrigin.Other; } } /// /// IPv6 only. Specifies the duplicate address detection state. Only supported /// for IPv6. If called on an IPv4 address, will throw a "not supported" exception. public override DuplicateAddressDetectionState DuplicateAddressDetectionState{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return DuplicateAddressDetectionState.Invalid; } } ////// Specifies the valid lifetime of the address in seconds. public override long AddressValidLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return 0; } } ////// Specifies the prefered lifetime of the address in seconds. public override long AddressPreferredLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return 0; } } ////// /// Specifies the prefered lifetime of the address in seconds. public override long DhcpLeaseLifetime{ get { return 0; } } //helper method that marshals the addressinformation into the classes internal static MulticastIPAddressInformationCollection ToAddressInformationCollection(IntPtr ptr) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. MulticastIPAddressInformationCollection addressList = new MulticastIPAddressInformationCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterAddress addr = (IpAdapterAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(new SystemMulticastIPAddressInformation(addr,ep.Address)); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(new SystemMulticastIPAddressInformation(addr,ep.Address)); } return addressList; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
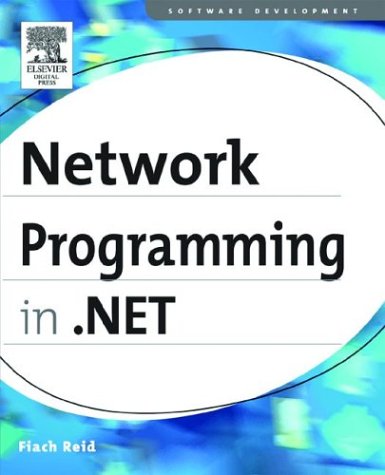
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CLSCompliantAttribute.cs
- SqlUtil.cs
- MetadataUtilsSmi.cs
- DataServicePagingProviderWrapper.cs
- TypedReference.cs
- QueryAccessibilityHelpEvent.cs
- ActivityCompletionCallbackWrapper.cs
- FileDataSourceCache.cs
- MainMenu.cs
- BackgroundWorker.cs
- AlignmentXValidation.cs
- DtdParser.cs
- SoapFault.cs
- TailPinnedEventArgs.cs
- SignatureHelper.cs
- SourceSwitch.cs
- TypedAsyncResult.cs
- DbConnectionHelper.cs
- ToggleButton.cs
- IPCCacheManager.cs
- DispatchWrapper.cs
- XMLSchema.cs
- X509SecurityTokenAuthenticator.cs
- OrderPreservingPipeliningSpoolingTask.cs
- CommandManager.cs
- ItemsPanelTemplate.cs
- SqlException.cs
- DynamicPropertyHolder.cs
- LoginView.cs
- DrawItemEvent.cs
- BitmapEffectGeneralTransform.cs
- FrugalList.cs
- Context.cs
- errorpatternmatcher.cs
- WsatAdminException.cs
- PingReply.cs
- XmlTextAttribute.cs
- PrePrepareMethodAttribute.cs
- ObjectNotFoundException.cs
- RoleManagerEventArgs.cs
- DbParameterCollectionHelper.cs
- SafeFileHandle.cs
- SqlAliaser.cs
- ListViewCommandEventArgs.cs
- XamlHostingSection.cs
- QueryUtil.cs
- Parsers.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- WebConfigurationFileMap.cs
- StateRuntime.cs
- RegexCaptureCollection.cs
- XPathNavigatorReader.cs
- CompositeFontParser.cs
- ObjectQueryProvider.cs
- InternalCompensate.cs
- ServiceDescriptions.cs
- ColorConvertedBitmapExtension.cs
- WebResourceAttribute.cs
- ProcessHost.cs
- ReceiveSecurityHeaderElementManager.cs
- ResourceContainer.cs
- HttpValueCollection.cs
- LookupNode.cs
- StorageMappingFragment.cs
- FloatUtil.cs
- Interlocked.cs
- QuaternionRotation3D.cs
- ToolStripRenderEventArgs.cs
- BufferedWebEventProvider.cs
- ChangePassword.cs
- Aggregates.cs
- MimeTypePropertyAttribute.cs
- NonClientArea.cs
- FormatConvertedBitmap.cs
- ActivationArguments.cs
- Condition.cs
- FontFaceLayoutInfo.cs
- KeyGesture.cs
- SimpleWebHandlerParser.cs
- ProfileSettingsCollection.cs
- SqlXmlStorage.cs
- ListDesigner.cs
- BaseConfigurationRecord.cs
- SymbolTable.cs
- FaultBookmark.cs
- MsdtcWrapper.cs
- SMSvcHost.cs
- ButtonPopupAdapter.cs
- NetworkAddressChange.cs
- BaseWebProxyFinder.cs
- BoolExpression.cs
- BindingContext.cs
- DataGridViewComboBoxEditingControl.cs
- StickyNoteAnnotations.cs
- TriState.cs
- AssociationType.cs
- EventProxy.cs
- DockingAttribute.cs
- CalloutQueueItem.cs
- ComNativeDescriptor.cs