Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Threading / Interlocked.cs / 1305376 / Interlocked.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // //[....] namespace System.Threading { using System; using System.Security.Permissions; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.Versioning; using System.Runtime; // After much discussion, we decided the Interlocked class doesn't need // any HPA's for synchronization or external threading. They hurt C#'s // codegen for the yield keyword, and arguably they didn't protect much. // Instead, they penalized people (and compilers) for writing threadsafe // code. [System.Security.SecuritySafeCritical] // auto-generated public static class Interlocked { /****************************** * Increment * Implemented: int * long *****************************/ [ResourceExposure(ResourceScope.None)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static int Increment(ref int location) { return Add(ref location, 1); } [ResourceExposure(ResourceScope.None)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static long Increment(ref long location) { return Add(ref location, 1); } /****************************** * Decrement * Implemented: int * long *****************************/ [ResourceExposure(ResourceScope.None)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static int Decrement(ref int location) { return Add(ref location, -1); } [ResourceExposure(ResourceScope.None)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static long Decrement(ref long location) { return Add(ref location, -1); } /****************************** * Exchange * Implemented: int * long * float * double * Object * IntPtr *****************************/ [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern int Exchange(ref int location1, int value); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern long Exchange(ref long location1, long value); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern float Exchange(ref float location1, float value); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern double Exchange(ref double location1, double value); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern Object Exchange(ref Object location1, Object value); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern IntPtr Exchange(ref IntPtr location1, IntPtr value); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [System.Runtime.InteropServices.ComVisible(false)] public static T Exchange(ref T location1, T value) where T : class { _Exchange(__makeref(location1), __makeref(value)); //Since value is a local we use trash its data on return // The Exchange replaces the data with new data // so after the return "value" contains the original location1 //See ExchangeGeneric for more details return value; } [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern void _Exchange(TypedReference location1, TypedReference value); /****************************** * CompareExchange * Implemented: int * long * float * double * Object * IntPtr *****************************/ [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern int CompareExchange(ref int location1, int value, int comparand); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern long CompareExchange(ref long location1, long value, long comparand); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern float CompareExchange(ref float location1, float value, float comparand); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern double CompareExchange(ref double location1, double value, double comparand); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern Object CompareExchange(ref Object location1, Object value, Object comparand); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern IntPtr CompareExchange(ref IntPtr location1, IntPtr value, IntPtr comparand); /****************************************************************** * CompareExchange * * Notice how CompareExchange () uses the __makeref keyword * to create two TypedReferences before calling _CompareExchange(). * This is horribly slow. Ideally we would like CompareExchange () * to simply call CompareExchange(ref Object, Object, Object); * however, this would require casting a "ref T" into a "ref Object", * which is not legal in C#. * * Thus we opted to cheat, and hacked to JIT so that when it reads * the method body for CompareExchange () it gets back the * following IL: * * ldarg.0 * ldarg.1 * ldarg.2 * call System.Threading.Interlocked::CompareExchange(ref Object, Object, Object) * ret * * See getILIntrinsicImplementationForInterlocked() in VM\JitInterface.cpp * for details. *****************************************************************/ [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [System.Runtime.InteropServices.ComVisible(false)] public static T CompareExchange (ref T location1, T value, T comparand) where T : class { // _CompareExchange() passes back the value read from location1 via local named 'value' _CompareExchange(__makeref(location1), __makeref(value), comparand); return value; } [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern void _CompareExchange(TypedReference location1, TypedReference value, Object comparand); // BCL-internal overload that returns success via a ref bool param, useful for reliable spin locks. [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static extern int CompareExchange(ref int location1, int value, int comparand, ref bool succeeded); /****************************** * Add * Implemented: int * long *****************************/ [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static extern int ExchangeAdd(ref int location1, int value); [ResourceExposure(ResourceScope.None)] [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern long ExchangeAdd(ref long location1, long value); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static int Add(ref int location1, int value) { return ExchangeAdd(ref location1, value) + value; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] #if !FEATURE_CORECLR [TargetedPatchingOptOut("Performance critical to inline across NGen image boundaries")] #endif public static long Add(ref long location1, long value) { return ExchangeAdd(ref location1, value) + value; } /****************************** * Read *****************************/ public static long Read(ref long location) { return Interlocked.CompareExchange(ref location,0,0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
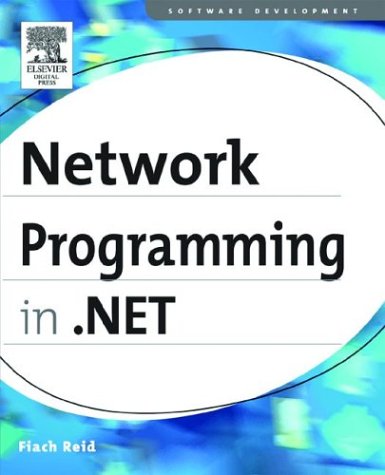
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfessionalColors.cs
- UIElementParaClient.cs
- Stream.cs
- Graphics.cs
- AuthorizationContext.cs
- ContextStaticAttribute.cs
- HtmlImage.cs
- DataServiceClientException.cs
- ColorConverter.cs
- GridViewPageEventArgs.cs
- ToolStripRenderEventArgs.cs
- Light.cs
- ExtendLockCommand.cs
- WaitHandle.cs
- TdsEnums.cs
- ToolStripPanelRenderEventArgs.cs
- RangeValuePatternIdentifiers.cs
- TaskFileService.cs
- TargetFrameworkAttribute.cs
- CompensationHandlingFilter.cs
- TextBox.cs
- HttpCachePolicy.cs
- XamlTreeBuilderBamlRecordWriter.cs
- XmlArrayAttribute.cs
- StorageBasedPackageProperties.cs
- DocumentReference.cs
- ScopedKnownTypes.cs
- SimpleType.cs
- ErrorsHelper.cs
- RelationshipEnd.cs
- AutoGeneratedFieldProperties.cs
- BamlLocalizabilityResolver.cs
- Style.cs
- SqlStatistics.cs
- ISessionStateStore.cs
- ContentAlignmentEditor.cs
- XPathAxisIterator.cs
- SQLByte.cs
- HtmlInputImage.cs
- DataServiceResponse.cs
- HyperLinkStyle.cs
- AbandonedMutexException.cs
- AndMessageFilterTable.cs
- TextEditorParagraphs.cs
- DrawingAttributesDefaultValueFactory.cs
- UniformGrid.cs
- BaseValidatorDesigner.cs
- WebConfigurationFileMap.cs
- DataGridViewHeaderCell.cs
- TrackingServices.cs
- AutoSizeToolBoxItem.cs
- PageThemeParser.cs
- OutputCacheProfileCollection.cs
- ThaiBuddhistCalendar.cs
- OutKeywords.cs
- RuleSettingsCollection.cs
- OutputCacheProfileCollection.cs
- BasicKeyConstraint.cs
- TaiwanCalendar.cs
- HttpModulesSection.cs
- DataViewSetting.cs
- NativeMethods.cs
- ComponentEvent.cs
- LambdaExpression.cs
- VariableExpressionConverter.cs
- HighlightVisual.cs
- FileEnumerator.cs
- SynchronizingStream.cs
- ZipIOLocalFileHeader.cs
- UrlParameterReader.cs
- ElementFactory.cs
- AppDomainCompilerProxy.cs
- DoubleCollectionValueSerializer.cs
- BindingExpressionBase.cs
- FlowDocumentPage.cs
- TransformedBitmap.cs
- ping.cs
- IntegerFacetDescriptionElement.cs
- GcSettings.cs
- PreviewKeyDownEventArgs.cs
- ListViewPagedDataSource.cs
- PageCodeDomTreeGenerator.cs
- VirtualDirectoryMapping.cs
- ListViewContainer.cs
- ToolTipAutomationPeer.cs
- SRGSCompiler.cs
- SafeFreeMibTable.cs
- SizeFConverter.cs
- MarshalDirectiveException.cs
- VectorValueSerializer.cs
- MenuStrip.cs
- DbInsertCommandTree.cs
- DataGridViewBindingCompleteEventArgs.cs
- Camera.cs
- ToolStripHighContrastRenderer.cs
- EntityDataSourceDesigner.cs
- Label.cs
- TripleDES.cs
- AutomationProperty.cs
- AQNBuilder.cs