Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / Drawing / System / Drawing / Design / ContentAlignmentEditor.cs / 1 / ContentAlignmentEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using System.ComponentModel.Design; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.Design; ////// /// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class ContentAlignmentEditor : UITypeEditor { private ContentUI contentUI; ///Provides a ///for /// visually editing content alignment. /// /// public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { object returnValue = value; if (provider != null) { IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); if (edSvc != null) { if (contentUI == null) { contentUI = new ContentUI(); } contentUI.Start(edSvc, value); edSvc.DropDownControl(contentUI); value = contentUI.Value; contentUI.End(); } } return value; } ////// Edits the given object value using /// the editor style provided by GetEditStyle. /// ////// /// public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.DropDown; } ////// Gets the editing style of the Edit method. /// ////// /// Control we use to provide the content alignment UI. /// private class ContentUI : Control { private IWindowsFormsEditorService edSvc; private object value; private RadioButton topLeft = new RadioButton(); private RadioButton topCenter = new RadioButton(); private RadioButton topRight = new RadioButton(); private RadioButton middleLeft = new RadioButton(); private RadioButton middleCenter = new RadioButton(); private RadioButton middleRight = new RadioButton(); private RadioButton bottomLeft = new RadioButton(); private RadioButton bottomCenter = new RadioButton(); private RadioButton bottomRight = new RadioButton(); ////// /// public ContentUI() { InitComponent(); } ////// /// private ContentAlignment Align { get { if (topLeft.Checked) { return ContentAlignment.TopLeft; } else if (topCenter.Checked) { return ContentAlignment.TopCenter; } else if (topRight.Checked) { return ContentAlignment.TopRight; } else if (middleLeft.Checked) { return ContentAlignment.MiddleLeft; } else if (middleCenter.Checked) { return ContentAlignment.MiddleCenter; } else if (middleRight.Checked) { return ContentAlignment.MiddleRight; } else if (bottomLeft.Checked) { return ContentAlignment.BottomLeft; } else if (bottomCenter.Checked) { return ContentAlignment.BottomCenter; } else { return ContentAlignment.BottomRight; } } set { switch (value) { case ContentAlignment.TopLeft: topLeft.Checked = true; break; case ContentAlignment.TopCenter: topCenter.Checked = true; break; case ContentAlignment.TopRight: topRight.Checked = true; break; case ContentAlignment.MiddleLeft: middleLeft.Checked = true; break; case ContentAlignment.MiddleCenter: middleCenter.Checked = true; break; case ContentAlignment.MiddleRight: middleRight.Checked = true; break; case ContentAlignment.BottomLeft: bottomLeft.Checked = true; break; case ContentAlignment.BottomCenter: bottomCenter.Checked = true; break; case ContentAlignment.BottomRight: bottomRight.Checked = true; break; } } } protected override bool ShowFocusCues { get { return true; } } ////// /// public object Value { get { return value; } } ////// /// public void End() { edSvc = null; value = null; } ////// /// [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] private void InitComponent() { this.Size = new Size(125, 89); this.BackColor = SystemColors.Control; this.ForeColor = SystemColors.ControlText; this.AccessibleName = SR.GetString(SR.ContentAlignmentEditorAccName); topLeft.Size = new Size (24, 25); topLeft.TabIndex = 8; topLeft.Text = ""; topLeft.Appearance = Appearance.Button; topLeft.Click += new EventHandler(this.OptionClick); topLeft.AccessibleName = SR.GetString(SR.ContentAlignmentEditorTopLeftAccName); topCenter.Anchor = AnchorStyles.Top | AnchorStyles.Left | AnchorStyles.Right; topCenter.Location = new Point(32, 0); topCenter.Size = new Size(59, 25); topCenter.TabIndex = 0; topCenter.Text = ""; topCenter.Appearance = Appearance.Button; topCenter.Click += new EventHandler(this.OptionClick); topCenter.AccessibleName = SR.GetString(SR.ContentAlignmentEditorTopCenterAccName); topRight.Anchor = AnchorStyles.Top | AnchorStyles.Right; topRight.Location = new Point(99, 0); topRight.Size = new Size(24, 25); topRight.TabIndex = 1; topRight.Text = ""; topRight.Appearance = Appearance.Button; topRight.Click += new EventHandler(this.OptionClick); topRight.AccessibleName = SR.GetString(SR.ContentAlignmentEditorTopRightAccName); middleLeft.Location = new Point(0, 32); middleLeft.Size = new Size(24, 25); middleLeft.TabIndex = 2; middleLeft.Text = ""; middleLeft.Appearance = Appearance.Button; middleLeft.Click += new EventHandler(this.OptionClick); middleLeft.AccessibleName = SR.GetString(SR.ContentAlignmentEditorMiddleLeftAccName); middleCenter.Anchor = AnchorStyles.Top | AnchorStyles.Left | AnchorStyles.Right; middleCenter.Location = new Point(32, 32); middleCenter.Size = new Size(59, 25); middleCenter.TabIndex = 3; middleCenter.Text = ""; middleCenter.Appearance = Appearance.Button; middleCenter.Click += new EventHandler(this.OptionClick); middleCenter.AccessibleName = SR.GetString(SR.ContentAlignmentEditorMiddleCenterAccName); middleRight.Anchor = AnchorStyles.Top | AnchorStyles.Right; middleRight.Location = new Point(99, 32); middleRight.Size = new Size(24, 25); middleRight.TabIndex = 4; middleRight.Text = ""; middleRight.Appearance = Appearance.Button; middleRight.Click += new EventHandler(this.OptionClick); middleRight.AccessibleName = SR.GetString(SR.ContentAlignmentEditorMiddleRightAccName); bottomLeft.Location = new Point(0, 64); bottomLeft.Size = new Size(24, 25); bottomLeft.TabIndex = 5; bottomLeft.Text = ""; bottomLeft.Appearance = Appearance.Button; bottomLeft.Click += new EventHandler(this.OptionClick); bottomLeft.AccessibleName = SR.GetString(SR.ContentAlignmentEditorBottomLeftAccName); bottomCenter.Anchor = AnchorStyles.Top | AnchorStyles.Left | AnchorStyles.Right; bottomCenter.Location = new Point(32, 64); bottomCenter.Size = new Size(59, 25); bottomCenter.TabIndex = 6; bottomCenter.Text = ""; bottomCenter.Appearance = Appearance.Button; bottomCenter.Click += new EventHandler(this.OptionClick); bottomCenter.AccessibleName = SR.GetString(SR.ContentAlignmentEditorBottomCenterAccName); bottomRight.Anchor = AnchorStyles.Top | AnchorStyles.Right; bottomRight.Location = new Point(99, 64); bottomRight.Size = new Size(24, 25); bottomRight.TabIndex = 7; bottomRight.Text = ""; bottomRight.Appearance = Appearance.Button; bottomRight.Click += new EventHandler(this.OptionClick); bottomRight.AccessibleName = SR.GetString(SR.ContentAlignmentEditorBottomRightAccName); this.Controls.Clear(); this.Controls.AddRange(new Control[]{ bottomRight, bottomCenter, bottomLeft, middleRight, middleCenter, middleLeft, topRight, topCenter, topLeft}); } protected override bool IsInputKey(System.Windows.Forms.Keys keyData) { switch (keyData) { case Keys.Left: case Keys.Right: case Keys.Up: case Keys.Down: //here, we will return false, 'cause we want the arrow keys //to get picked up by the process key method below return false; } return base.IsInputKey(keyData); } ////// /// private void OptionClick(object sender, EventArgs e) { value = Align; edSvc.CloseDropDown(); } ////// /// public void Start(IWindowsFormsEditorService edSvc, object value) { this.edSvc = edSvc; this.value = value; ContentAlignment align; if (value == null) { align = ContentAlignment.MiddleLeft; } else { align = (ContentAlignment)value; } Align = align; } ////// /// Here, we handle the return, tab, and escape keys appropriately /// protected override bool ProcessDialogKey(Keys keyData) { RadioButton checkedControl = CheckedControl; if ((keyData & Keys.KeyCode) == Keys.Left) { if (checkedControl == bottomRight) { CheckedControl = bottomCenter; } else if (checkedControl == middleRight) { CheckedControl = middleCenter; } else if (checkedControl == topRight) { CheckedControl = topCenter; } else if (checkedControl == bottomCenter) { CheckedControl = bottomLeft; } else if (checkedControl == middleCenter) { CheckedControl = middleLeft; } else if (checkedControl == topCenter) { CheckedControl = topLeft; } return true; } else if ((keyData & Keys.KeyCode) == Keys.Right) { if (checkedControl == bottomLeft) { CheckedControl = bottomCenter; } else if (checkedControl == middleLeft) { CheckedControl = middleCenter; } else if (checkedControl == topLeft) { CheckedControl = topCenter; } else if (checkedControl == bottomCenter) { CheckedControl = bottomRight; } else if (checkedControl == middleCenter) { CheckedControl = middleRight; } else if (checkedControl == topCenter) { CheckedControl = topRight; } return true; } else if ((keyData & Keys.KeyCode) == Keys.Up) { if (checkedControl == bottomRight) { CheckedControl = middleRight; } else if (checkedControl == middleRight) { CheckedControl = topRight; } else if (checkedControl == bottomCenter) { CheckedControl = middleCenter; } else if (checkedControl == middleCenter) { CheckedControl = topCenter; } else if (checkedControl == bottomLeft) { CheckedControl = middleLeft; } else if (checkedControl == middleLeft) { CheckedControl = topLeft; } return true; } else if ((keyData & Keys.KeyCode) == Keys.Down) { if (checkedControl == topRight) { CheckedControl = middleRight; } else if (checkedControl == middleRight) { CheckedControl = bottomRight; } else if (checkedControl == topCenter) { CheckedControl = middleCenter; } else if (checkedControl == middleCenter) { CheckedControl = bottomCenter; } else if (checkedControl == topLeft) { CheckedControl = middleLeft; } else if (checkedControl == middleLeft) { CheckedControl = bottomLeft; } return true; } else if ((keyData & Keys.KeyCode) == Keys.Space) { this.OptionClick(this, EventArgs.Empty); return true; } else if ((keyData & Keys.KeyCode) == Keys.Return && (keyData & (Keys.Alt | Keys.Control)) == 0) { this.OptionClick(this, EventArgs.Empty); return true; } else if ((keyData & Keys.KeyCode) == Keys.Escape && (keyData & (Keys.Alt | Keys.Control)) == 0) { edSvc.CloseDropDown(); return true; } else if ((keyData & Keys.KeyCode) == Keys.Tab && (keyData & (Keys.Alt | Keys.Control)) == 0) { int nextTabIndex = CheckedControl.TabIndex + ((keyData & Keys.Shift) == 0 ? 1 : -1); if (nextTabIndex < 0) { nextTabIndex = Controls.Count - 1; } else if (nextTabIndex >= Controls.Count) { nextTabIndex = 0; } for (int i = 0; i < Controls.Count; i++ ) { if (Controls[i] is RadioButton && Controls[i].TabIndex == nextTabIndex) { CheckedControl = (RadioButton)Controls[i]; return true; } } return true; } return base.ProcessDialogKey(keyData); } ////// /// Gets/Sets the checked control value of our editor /// private RadioButton CheckedControl { get { for (int i =0; i < Controls.Count; i++) { if (Controls[i] is RadioButton && ((RadioButton)Controls[i]).Checked == true) { return (RadioButton)Controls[i]; } } return middleLeft; } set { CheckedControl.Checked = false; value.Checked = true; // RadioButton::Checked will tell Accessibility that State and Name changed. // Tell Accessibility that focus changed as well. if (value.IsHandleCreated) { UnsafeNativeMethods.NotifyWinEvent((int) AccessibleEvents.Focus, new HandleRef(value, value.Handle), UnsafeNativeMethods.OBJID_CLIENT, 0); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
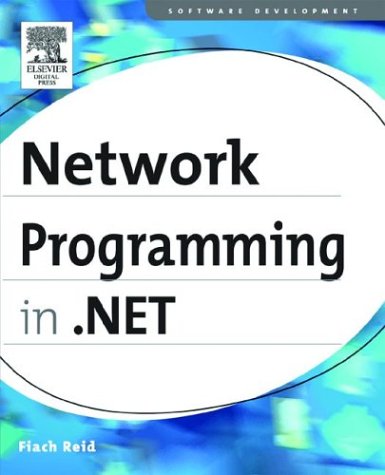
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StandardCommands.cs
- TextTreeText.cs
- SelectedCellsChangedEventArgs.cs
- MobileControlsSection.cs
- HttpWebRequest.cs
- GenericTypeParameterBuilder.cs
- CodeCommentStatementCollection.cs
- MailWebEventProvider.cs
- Style.cs
- SeekStoryboard.cs
- ExtensionDataObject.cs
- Visual3DCollection.cs
- ObjectAssociationEndMapping.cs
- TraceUtils.cs
- PlacementWorkspace.cs
- ViewValidator.cs
- ReadOnlyTernaryTree.cs
- DataListItem.cs
- ConfigurationLockCollection.cs
- SerializationObjectManager.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- TreeNodeBindingCollection.cs
- HistoryEventArgs.cs
- MembershipSection.cs
- XmlILConstructAnalyzer.cs
- Point.cs
- DiscardableAttribute.cs
- ServiceDescription.cs
- PrintPreviewControl.cs
- DataBoundControlParameterTarget.cs
- BindingOperations.cs
- EventsTab.cs
- SecurityStandardsManager.cs
- BehaviorEditorPart.cs
- ScrollBarRenderer.cs
- LoginName.cs
- FlowSwitch.cs
- ErrorHandler.cs
- RoleGroup.cs
- KerberosReceiverSecurityToken.cs
- AspCompat.cs
- UiaCoreApi.cs
- ConstraintEnumerator.cs
- RtfToXamlLexer.cs
- RegionData.cs
- BitmapFrameEncode.cs
- SchemaMerger.cs
- StrokeRenderer.cs
- RsaSecurityToken.cs
- AllMembershipCondition.cs
- NetSectionGroup.cs
- BamlRecordHelper.cs
- WindowsTitleBar.cs
- ReferenceEqualityComparer.cs
- UInt32Converter.cs
- SortFieldComparer.cs
- Mouse.cs
- BaseDataBoundControl.cs
- ScrollProperties.cs
- PackageProperties.cs
- BindingObserver.cs
- AttributeInfo.cs
- MessageHeader.cs
- DataListAutoFormat.cs
- SelectorAutomationPeer.cs
- StatusBarItemAutomationPeer.cs
- TemplatePropertyEntry.cs
- HwndKeyboardInputProvider.cs
- SchemaDeclBase.cs
- AvTraceDetails.cs
- DynamicDiscoSearcher.cs
- RowSpanVector.cs
- ExpandCollapseProviderWrapper.cs
- XsdValidatingReader.cs
- ObjectRef.cs
- CommonDialog.cs
- WindowsSlider.cs
- assertwrapper.cs
- DetailsView.cs
- PersonalizationProviderHelper.cs
- Converter.cs
- VarRemapper.cs
- XsltSettings.cs
- DataError.cs
- Speller.cs
- EntityTypeEmitter.cs
- FixedTextContainer.cs
- AsymmetricSignatureDeformatter.cs
- InkCanvas.cs
- NameSpaceEvent.cs
- tibetanshape.cs
- VectorValueSerializer.cs
- NonSerializedAttribute.cs
- OleDbCommand.cs
- DSACryptoServiceProvider.cs
- oledbmetadatacolumnnames.cs
- TextElementAutomationPeer.cs
- DataBinding.cs
- ActiveXHelper.cs
- EventMemberCodeDomSerializer.cs