Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / System / IO / Packaging / PackageProperties.cs / 1 / PackageProperties.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This abstract class provides access to the "core properties" a Metro document. // The "core properties" are a subset of the standard OLE property sets // SummaryInformation and DocumentSummaryInformation, and include such properties // as Title and Subject. // // There are two concrete derived classes. PackagePackageProperties represents the // core properties of a normal unencrypted Metro document, physically represented // as a Zip archive. EncryptedPackagePackageProperties represents the core properties // of an RM-protected Metro document, physically represented by an OLE compound // file containing a well-known stream in which a Metro Zip archive, encrypted // in its entirety, is stored. // // History: // 06/22/2005: [....]: Initial implementation. // //----------------------------------------------------------------------------- using System; using System.IO.Packaging; namespace System.IO.Packaging { ////// This class provides access to the "core properties", such as Title and /// Subject, of an RM-protected Metro package. These properties are a subset of /// of the standard OLE property sets SummaryInformation and /// DocumentSummaryInformation. /// public abstract class PackageProperties : IDisposable { #region IDisposable ////// Allow the object to clean up all resources it holds (both managed and /// unmanaged), and ensure that the resources won't be released a /// second time by removing it from the finalization queue. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// This default implementation is provided for subclasses that do not /// make use of the IDisposable functionality. /// protected virtual void Dispose(bool disposing) { } #endregion IDisposable //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties #region SummaryInformation properties ////// The title. /// public abstract string Title { get; set; } ////// The topic of the contents. /// public abstract string Subject { get; set; } ////// The primary creator. The identification is environment-specific and /// can consist of a name, email address, employee ID, etc. It is /// recommended that this value be only as verbose as necessary to /// identify the individual. /// public abstract string Creator { get; set; } ////// A delimited set of keywords to support searching and indexing. This /// is typically a list of terms that are not available elsewhere in the /// properties. /// public abstract string Keywords { get; set; } ////// The description or abstract of the contents. /// public abstract string Description { get; set; } ////// The user who performed the last modification. The identification is /// environment-specific and can consist of a name, email address, /// employee ID, etc. It is recommended that this value be only as /// verbose as necessary to identify the individual. /// public abstract string LastModifiedBy { get; set; } ////// The revision number. This value indicates the number of saves or /// revisions. The application is responsible for updating this value /// after each revision. /// public abstract string Revision { get; set; } ////// The date and time of the last printing. /// public abstract NullableLastPrinted { get; set; } /// /// The creation date and time. /// public abstract NullableCreated { get; set; } /// /// The date and time of the last modification. /// public abstract NullableModified { get; set; } #endregion SummaryInformation properties #region DocumentSummaryInformation properties /// /// The category. This value is typically used by UI applications to create navigation /// controls. /// public abstract string Category { get; set; } ////// A unique identifier. /// public abstract string Identifier { get; set; } ////// The type of content represented, generally defined by a specific /// use and intended audience. Example values include "Whitepaper", /// "Security Bulletin", and "Exam". (This property is distinct from /// MIME content types as defined in RFC 2045.) /// public abstract string ContentType { get; set; } ////// The primary language of the package content. The language tag is /// composed of one or more parts: A primary language subtag and a /// (possibly empty) series of subsequent subtags, for example, "EN-US". /// These values MUST follow the convention specified in RFC 3066. /// public abstract string Language { get; set; } ////// The version number. This value is set by the user or by the application. /// public abstract string Version { get; set; } ////// The status of the content. Example values include "Draft", /// "Reviewed", and "Final". /// public abstract string ContentStatus { get; set; } #endregion DocumentSummaryInformation properties #endregion Public Properties } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
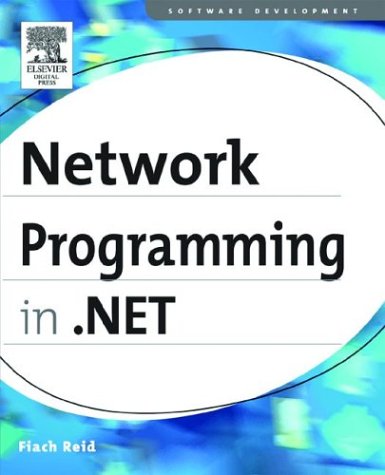
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DeferrableContent.cs
- HTTPNotFoundHandler.cs
- URI.cs
- ItemAutomationPeer.cs
- OleDbErrorCollection.cs
- EmptyCollection.cs
- TemplateXamlParser.cs
- DataRelationPropertyDescriptor.cs
- FloaterBaseParagraph.cs
- CancelEventArgs.cs
- StateElement.cs
- EdmRelationshipRoleAttribute.cs
- MatrixTransform.cs
- PackageProperties.cs
- Int32Rect.cs
- ByteFacetDescriptionElement.cs
- GenericUriParser.cs
- RectValueSerializer.cs
- PrimitiveXmlSerializers.cs
- ClipboardProcessor.cs
- FileVersion.cs
- AttachmentCollection.cs
- LinqDataSourceContextEventArgs.cs
- HtmlInputReset.cs
- HtmlPhoneCallAdapter.cs
- GroupItem.cs
- FilterInvalidBodyAccessException.cs
- ArrayList.cs
- RoutedEventHandlerInfo.cs
- Int64.cs
- QEncodedStream.cs
- AssertUtility.cs
- Propagator.JoinPropagator.cs
- DataObjectSettingDataEventArgs.cs
- MenuTracker.cs
- XmlTextReader.cs
- BamlResourceContent.cs
- InProcStateClientManager.cs
- SQLDoubleStorage.cs
- MenuItemStyleCollectionEditor.cs
- DrawingCollection.cs
- StringKeyFrameCollection.cs
- TypeToken.cs
- remotingproxy.cs
- OperationExecutionFault.cs
- DbModificationCommandTree.cs
- TextServicesContext.cs
- RoleManagerSection.cs
- QueryGeneratorBase.cs
- AnchoredBlock.cs
- XmlNodeWriter.cs
- BindingExpressionUncommonField.cs
- WindowsFormsLinkLabel.cs
- ServiceModelSecurityTokenTypes.cs
- TreeNode.cs
- DefaultMergeHelper.cs
- AliasGenerator.cs
- DeviceContext.cs
- Documentation.cs
- HttpCachePolicyElement.cs
- PropertyTabChangedEvent.cs
- unsafenativemethodsother.cs
- HttpRawResponse.cs
- CommandConverter.cs
- DataServiceHostWrapper.cs
- clipboard.cs
- ImageCodecInfoPrivate.cs
- UsernameTokenFactoryCredential.cs
- ListQueryResults.cs
- HeaderedContentControl.cs
- ClientSettingsProvider.cs
- HScrollProperties.cs
- StackSpiller.Temps.cs
- OutputChannelBinder.cs
- AssociationTypeEmitter.cs
- NativeMethods.cs
- IISUnsafeMethods.cs
- AuthenticationSection.cs
- SiteMapDataSource.cs
- CachingHintValidation.cs
- ECDiffieHellmanCng.cs
- MimeTypeAttribute.cs
- CompiledQuery.cs
- StreamGeometry.cs
- RoleService.cs
- CmsInterop.cs
- DbMetaDataCollectionNames.cs
- XmlWellformedWriter.cs
- DocumentViewerBaseAutomationPeer.cs
- DefaultParameterValueAttribute.cs
- PropertyEmitterBase.cs
- TypeDescriptionProvider.cs
- TextRunProperties.cs
- SystemIPInterfaceStatistics.cs
- CrossSiteScriptingValidation.cs
- FieldNameLookup.cs
- DateRangeEvent.cs
- StrokeCollection.cs
- Select.cs
- XPathQueryGenerator.cs