Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / Microsoft / Win32 / SafeHandles / SafeWaitHandle.cs / 1305376 / SafeWaitHandle.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SafeWaitHandle ** ** ** A wrapper for Win32 events (mutexes, auto reset events, and ** manual reset events). Used by WaitHandle. ** ** ===========================================================*/ using System; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.Versioning; using Microsoft.Win32; using System.Threading; namespace Microsoft.Win32.SafeHandles { [System.Security.SecurityCritical] // auto-generated_required public sealed class SafeWaitHandle : SafeHandleZeroOrMinusOneIsInvalid { // Special case flags for Mutexes enables workaround for known OS bug at // http://support.microsoft.com/default.aspx?scid=kb;en-us;889318 // One machine-wide mutex serializes all OpenMutex and CloseHandle operations. // bIsMutex: if true, we need to grab machine-wide mutex before doing any Close ops. // Initialized to false by the runtime. private bool bIsMutex; // bIsMutex: if true, we need to avoid grabbing the machine-wide mutex before Close ops, // since that mutex is, of course, this very handle. // Initialized to false by the runtime. private bool bIsReservedMutex; // Called by P/Invoke marshaler private SafeWaitHandle() : base(true) { } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] public SafeWaitHandle(IntPtr existingHandle, bool ownsHandle) : base(ownsHandle) { SetHandle(existingHandle); } [System.Security.SecurityCritical] [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] override protected bool ReleaseHandle() { #if !FEATURE_CORECLR if (!bIsMutex || Environment.HasShutdownStarted) return Win32Native.CloseHandle(handle); bool bReturn = false; bool bMutexObtained = false; try { if (!bIsReservedMutex) { Mutex.AcquireReservedMutex(ref bMutexObtained); } bReturn = Win32Native.CloseHandle(handle); } finally { if (bMutexObtained) Mutex.ReleaseReservedMutex(); } return bReturn; #else return Win32Native.CloseHandle(handle); #endif } internal void SetAsMutex() { bIsMutex = true; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal void SetAsReservedMutex() { bIsReservedMutex = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
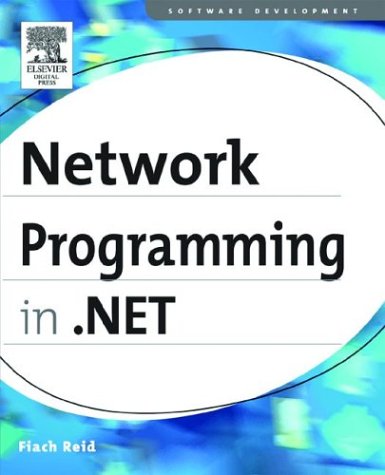
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IsolatedStorageFileStream.cs
- SqlDataSourceCache.cs
- Misc.cs
- ModuleBuilderData.cs
- CriticalHandle.cs
- XmlTypeAttribute.cs
- MemberAssignmentAnalysis.cs
- Button.cs
- EmptyElement.cs
- WebGetAttribute.cs
- AnonymousIdentificationModule.cs
- TemplatedWizardStep.cs
- CodeAccessPermission.cs
- UIElement3DAutomationPeer.cs
- GridViewColumnHeader.cs
- Qualifier.cs
- MouseBinding.cs
- TimeSpan.cs
- StatusBarDrawItemEvent.cs
- JsonFormatGeneratorStatics.cs
- ParallelTimeline.cs
- StyleCollection.cs
- XamlTypeWithExplicitNamespace.cs
- FloatUtil.cs
- followingquery.cs
- InitiatorSessionSymmetricTransportSecurityProtocol.cs
- Rotation3DAnimation.cs
- Utils.cs
- RightsManagementEncryptedStream.cs
- MobileUITypeEditor.cs
- RequiredAttributeAttribute.cs
- SlipBehavior.cs
- ToolboxItemAttribute.cs
- OdbcConnectionFactory.cs
- XsdDataContractExporter.cs
- Form.cs
- RunInstallerAttribute.cs
- Brush.cs
- InvalidOleVariantTypeException.cs
- DataGridViewCell.cs
- DriveNotFoundException.cs
- WindowsBrush.cs
- FlowDecisionLabelFeature.cs
- SQLDoubleStorage.cs
- KeysConverter.cs
- SqlAliaser.cs
- TypeProvider.cs
- XpsPartBase.cs
- XmlSerializer.cs
- PrincipalPermission.cs
- CodeDomExtensionMethods.cs
- PublisherMembershipCondition.cs
- Route.cs
- ClientBuildManagerCallback.cs
- RoleManagerEventArgs.cs
- RemotingServices.cs
- bidPrivateBase.cs
- XmlSerializerSection.cs
- SQLBinary.cs
- XPathAxisIterator.cs
- BitmapEffect.cs
- InstanceKeyCompleteException.cs
- VirtualPath.cs
- DateTimeOffsetConverter.cs
- DrawingImage.cs
- MediaSystem.cs
- IdnMapping.cs
- RecordBuilder.cs
- EnlistmentTraceIdentifier.cs
- RegexGroup.cs
- GeometryHitTestResult.cs
- DataGridViewIntLinkedList.cs
- AttributeEmitter.cs
- XmlNodeReader.cs
- FormDesigner.cs
- Evaluator.cs
- MemberNameValidator.cs
- ListBindableAttribute.cs
- TimeZone.cs
- PersonalizableTypeEntry.cs
- SchemaDeclBase.cs
- AvTraceDetails.cs
- ResourceSetExpression.cs
- BuildProviderAppliesToAttribute.cs
- SettingsSavedEventArgs.cs
- DrawingContextWalker.cs
- OperationSelectorBehavior.cs
- XsltOutput.cs
- WebPartTracker.cs
- EmbossBitmapEffect.cs
- FirstMatchCodeGroup.cs
- ListBoxItem.cs
- SafeRightsManagementPubHandle.cs
- MessagePartSpecification.cs
- UpdateManifestForBrowserApplication.cs
- CachedFontFamily.cs
- ExpressionLink.cs
- Int32EqualityComparer.cs
- PolyBezierSegment.cs
- OrderByExpression.cs