Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / ListBoxItem.cs / 1 / ListBoxItem.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Input; using System.Windows.Controls.Primitives; using System.Windows.Shapes; using System; // Disable CS3001: Warning as Error: not CLS-compliant #pragma warning disable 3001 namespace System.Windows.Controls { ////// Control that implements a selectable item inside a ListBox. /// [DefaultEvent("Selected")] public class ListBoxItem : ContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// public ListBoxItem() : base() { } static ListBoxItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(typeof(ListBoxItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ListBoxItem)); KeyboardNavigation.DirectionalNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Once)); KeyboardNavigation.TabNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Local)); } #endregion //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Indicates whether this ListBoxItem is selected. /// public static readonly DependencyProperty IsSelectedProperty = Selector.IsSelectedProperty.AddOwner(typeof(ListBoxItem), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault | FrameworkPropertyMetadataOptions.Journal, new PropertyChangedCallback(OnIsSelectedChanged))); ////// Indicates whether this ListBoxItem is selected. /// [Bindable(true), Category("Appearance")] public bool IsSelected { get { return (bool) GetValue(IsSelectedProperty); } set { SetValue(IsSelectedProperty, BooleanBoxes.Box(value)); } } private static void OnIsSelectedChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ListBoxItem listItem = d as ListBoxItem; bool isSelected = (bool) e.NewValue; Selector parentSelector = listItem.ParentSelector; if (parentSelector != null) { parentSelector.RaiseIsSelectedChangedAutomationEvent(listItem, isSelected); } if (isSelected) { listItem.OnSelected(new RoutedEventArgs(Selector.SelectedEvent, listItem)); } else { listItem.OnUnselected(new RoutedEventArgs(Selector.UnselectedEvent, listItem)); } } ////// Event indicating that the IsSelected property is now true. /// /// Event arguments protected virtual void OnSelected(RoutedEventArgs e) { HandleIsSelectedChanged(true, e); } ////// Event indicating that the IsSelected property is now false. /// /// Event arguments protected virtual void OnUnselected(RoutedEventArgs e) { HandleIsSelectedChanged(false, e); } private void HandleIsSelectedChanged(bool newValue, RoutedEventArgs e) { RaiseEvent(e); } #endregion #region Events ////// Raised when the item's IsSelected property becomes true. /// public static readonly RoutedEvent SelectedEvent = Selector.SelectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes true. /// public event RoutedEventHandler Selected { add { AddHandler(SelectedEvent, value); } remove { RemoveHandler(SelectedEvent, value); } } ////// Raised when the item's IsSelected property becomes false. /// public static readonly RoutedEvent UnselectedEvent = Selector.UnselectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes false. /// public event RoutedEventHandler Unselected { add { AddHandler(UnselectedEvent, value); } remove { RemoveHandler(UnselectedEvent, value); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ListBoxItemWrapperAutomationPeer(this); } ///) /// /// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Left); } base.OnMouseLeftButtonDown(e); } ////// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseRightButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Right); } base.OnMouseRightButtonDown(e); } private void HandleMouseButtonDown(MouseButton mouseButton) { if (Selector.UiGetIsSelectable(this) && Focus()) { ListBox parent = ParentListBox; if (parent != null) { parent.NotifyListItemClicked(this, mouseButton); } } } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseEnter(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } if (IsMouseOver) { ListBox parent = ParentListBox; if (parent != null && Mouse.LeftButton == MouseButtonState.Pressed) { parent.NotifyListItemMouseDragged(this); } } base.OnMouseEnter(e); } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseLeave(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } base.OnMouseLeave(e); } ////// Called when the visual parent of this element changes. /// /// protected internal override void OnVisualParentChanged(DependencyObject oldParent) { ItemsControl oldItemsControl = null; if (VisualTreeHelper.GetParent(this) == null) { if (IsKeyboardFocusWithin) { // This ListBoxItem had focus but was removed from the tree. // The normal behavior is for focus to become null, but we would rather that // focus go to the parent ListBox. // Use the oldParent to get a reference to the ListBox that this ListBoxItem used to be in. // The oldParent's ItemsOwner should be the ListBox. oldItemsControl = ItemsControl.GetItemsOwner(oldParent); } } else { // When the item is added to the tree while grouping, the item will need to // update its IsSelected property. This is currently less optimal than the non-grouped // case where IsSelected is updated directly on the child because each selected // child here will have to search the selected items collection (but usually this // is a small number and will only happen when some style changed and caused everything // to be re-generated). Selector selector = ParentSelector; if (selector != null) { if ((selector.ItemContainerGenerator.GroupStyle != null) && !IsSelected) { object item = selector.ItemContainerGenerator.ItemFromContainer(this); if ((item != null) && selector._selectedItems.Contains(item)) { IsSelected = true; } } } } base.OnVisualParentChanged(oldParent); // If earlier, we decided to set focus to the old parent ListBox, do it here // after calling base so that the state for IsKeyboardFocusWithin is updated correctly. if (oldItemsControl != null) { oldItemsControl.Focus(); } } #endregion //------------------------------------------------------------------- // // Implementation // //-------------------------------------------------------------------- #region Implementation private ListBox ParentListBox { get { return ParentSelector as ListBox; } } internal Selector ParentSelector { get { return ItemsControl.ItemsControlFromItemContainer(this) as Selector; } } #endregion #if OLD_AUTOMATION // left here for reference only as it is still used by MonthCalendar ////// DependencyProperty for SelectionContainer property. /// internal static readonly DependencyProperty SelectionContainerProperty = DependencyProperty.RegisterAttached("SelectionContainer", typeof(UIElement), typeof(ListBoxItem), new FrameworkPropertyMetadata((UIElement)null)); #endif #region Private Fields DispatcherOperation parentNotifyDraggedOperation = null; #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Input; using System.Windows.Controls.Primitives; using System.Windows.Shapes; using System; // Disable CS3001: Warning as Error: not CLS-compliant #pragma warning disable 3001 namespace System.Windows.Controls { ////// Control that implements a selectable item inside a ListBox. /// [DefaultEvent("Selected")] public class ListBoxItem : ContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// public ListBoxItem() : base() { } static ListBoxItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(typeof(ListBoxItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ListBoxItem)); KeyboardNavigation.DirectionalNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Once)); KeyboardNavigation.TabNavigationProperty.OverrideMetadata(typeof(ListBoxItem), new FrameworkPropertyMetadata(KeyboardNavigationMode.Local)); } #endregion //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Indicates whether this ListBoxItem is selected. /// public static readonly DependencyProperty IsSelectedProperty = Selector.IsSelectedProperty.AddOwner(typeof(ListBoxItem), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault | FrameworkPropertyMetadataOptions.Journal, new PropertyChangedCallback(OnIsSelectedChanged))); ////// Indicates whether this ListBoxItem is selected. /// [Bindable(true), Category("Appearance")] public bool IsSelected { get { return (bool) GetValue(IsSelectedProperty); } set { SetValue(IsSelectedProperty, BooleanBoxes.Box(value)); } } private static void OnIsSelectedChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ListBoxItem listItem = d as ListBoxItem; bool isSelected = (bool) e.NewValue; Selector parentSelector = listItem.ParentSelector; if (parentSelector != null) { parentSelector.RaiseIsSelectedChangedAutomationEvent(listItem, isSelected); } if (isSelected) { listItem.OnSelected(new RoutedEventArgs(Selector.SelectedEvent, listItem)); } else { listItem.OnUnselected(new RoutedEventArgs(Selector.UnselectedEvent, listItem)); } } ////// Event indicating that the IsSelected property is now true. /// /// Event arguments protected virtual void OnSelected(RoutedEventArgs e) { HandleIsSelectedChanged(true, e); } ////// Event indicating that the IsSelected property is now false. /// /// Event arguments protected virtual void OnUnselected(RoutedEventArgs e) { HandleIsSelectedChanged(false, e); } private void HandleIsSelectedChanged(bool newValue, RoutedEventArgs e) { RaiseEvent(e); } #endregion #region Events ////// Raised when the item's IsSelected property becomes true. /// public static readonly RoutedEvent SelectedEvent = Selector.SelectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes true. /// public event RoutedEventHandler Selected { add { AddHandler(SelectedEvent, value); } remove { RemoveHandler(SelectedEvent, value); } } ////// Raised when the item's IsSelected property becomes false. /// public static readonly RoutedEvent UnselectedEvent = Selector.UnselectedEvent.AddOwner(typeof(ListBoxItem)); ////// Raised when the item's IsSelected property becomes false. /// public event RoutedEventHandler Unselected { add { AddHandler(UnselectedEvent, value); } remove { RemoveHandler(UnselectedEvent, value); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ListBoxItemWrapperAutomationPeer(this); } ///) /// /// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Left); } base.OnMouseLeftButtonDown(e); } ////// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseRightButtonDown(MouseButtonEventArgs e) { if (!e.Handled) { // e.Handled = true; HandleMouseButtonDown(MouseButton.Right); } base.OnMouseRightButtonDown(e); } private void HandleMouseButtonDown(MouseButton mouseButton) { if (Selector.UiGetIsSelectable(this) && Focus()) { ListBox parent = ParentListBox; if (parent != null) { parent.NotifyListItemClicked(this, mouseButton); } } } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseEnter(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } if (IsMouseOver) { ListBox parent = ParentListBox; if (parent != null && Mouse.LeftButton == MouseButtonState.Pressed) { parent.NotifyListItemMouseDragged(this); } } base.OnMouseEnter(e); } ////// Called when IsMouseOver changes on this element. /// /// protected override void OnMouseLeave(MouseEventArgs e) { // abort any drag operation we have queued. if (parentNotifyDraggedOperation != null) { parentNotifyDraggedOperation.Abort(); parentNotifyDraggedOperation = null; } base.OnMouseLeave(e); } ////// Called when the visual parent of this element changes. /// /// protected internal override void OnVisualParentChanged(DependencyObject oldParent) { ItemsControl oldItemsControl = null; if (VisualTreeHelper.GetParent(this) == null) { if (IsKeyboardFocusWithin) { // This ListBoxItem had focus but was removed from the tree. // The normal behavior is for focus to become null, but we would rather that // focus go to the parent ListBox. // Use the oldParent to get a reference to the ListBox that this ListBoxItem used to be in. // The oldParent's ItemsOwner should be the ListBox. oldItemsControl = ItemsControl.GetItemsOwner(oldParent); } } else { // When the item is added to the tree while grouping, the item will need to // update its IsSelected property. This is currently less optimal than the non-grouped // case where IsSelected is updated directly on the child because each selected // child here will have to search the selected items collection (but usually this // is a small number and will only happen when some style changed and caused everything // to be re-generated). Selector selector = ParentSelector; if (selector != null) { if ((selector.ItemContainerGenerator.GroupStyle != null) && !IsSelected) { object item = selector.ItemContainerGenerator.ItemFromContainer(this); if ((item != null) && selector._selectedItems.Contains(item)) { IsSelected = true; } } } } base.OnVisualParentChanged(oldParent); // If earlier, we decided to set focus to the old parent ListBox, do it here // after calling base so that the state for IsKeyboardFocusWithin is updated correctly. if (oldItemsControl != null) { oldItemsControl.Focus(); } } #endregion //------------------------------------------------------------------- // // Implementation // //-------------------------------------------------------------------- #region Implementation private ListBox ParentListBox { get { return ParentSelector as ListBox; } } internal Selector ParentSelector { get { return ItemsControl.ItemsControlFromItemContainer(this) as Selector; } } #endregion #if OLD_AUTOMATION // left here for reference only as it is still used by MonthCalendar ////// DependencyProperty for SelectionContainer property. /// internal static readonly DependencyProperty SelectionContainerProperty = DependencyProperty.RegisterAttached("SelectionContainer", typeof(UIElement), typeof(ListBoxItem), new FrameworkPropertyMetadata((UIElement)null)); #endif #region Private Fields DispatcherOperation parentNotifyDraggedOperation = null; #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
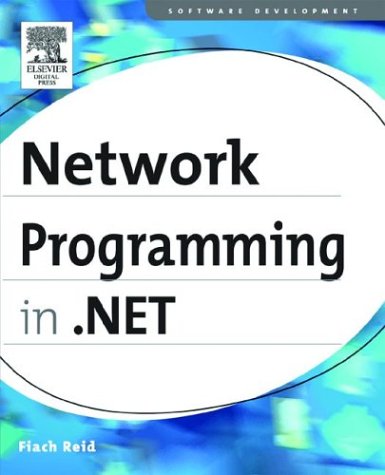
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberAccessException.cs
- XmlSchemaObjectCollection.cs
- DbConnectionPool.cs
- Debug.cs
- _CommandStream.cs
- DataObjectSettingDataEventArgs.cs
- BufferedGraphicsContext.cs
- CodeTypeParameter.cs
- ShortcutKeysEditor.cs
- InputManager.cs
- DockPanel.cs
- GrammarBuilderPhrase.cs
- WebPartActionVerb.cs
- ClientSponsor.cs
- StateRuntime.cs
- BypassElementCollection.cs
- IssuanceLicense.cs
- HttpHostedTransportConfiguration.cs
- NgenServicingAttributes.cs
- DesignBindingPicker.cs
- TypeUnloadedException.cs
- Descriptor.cs
- GeneralTransform3D.cs
- ControlHelper.cs
- RegexCapture.cs
- Themes.cs
- Part.cs
- Context.cs
- StylusSystemGestureEventArgs.cs
- DbBuffer.cs
- StringInfo.cs
- HtmlInputRadioButton.cs
- SerializationFieldInfo.cs
- StringFunctions.cs
- StandardBindingReliableSessionElement.cs
- WebBodyFormatMessageProperty.cs
- ByteAnimation.cs
- IsolatedStorageFile.cs
- BypassElementCollection.cs
- SqlDataSource.cs
- RotateTransform3D.cs
- PersistChildrenAttribute.cs
- NavigatingCancelEventArgs.cs
- HttpCookieCollection.cs
- DataGridRowsPresenter.cs
- LineSegment.cs
- EllipseGeometry.cs
- XmlDigitalSignatureProcessor.cs
- WebResourceAttribute.cs
- LogicalTreeHelper.cs
- SmtpLoginAuthenticationModule.cs
- XomlCompilerResults.cs
- DataControlButton.cs
- BitmapPalette.cs
- Registry.cs
- MouseActionConverter.cs
- XmlNamespaceDeclarationsAttribute.cs
- D3DImage.cs
- MouseCaptureWithinProperty.cs
- HashMembershipCondition.cs
- XmlObjectSerializer.cs
- AccessDataSourceWizardForm.cs
- Image.cs
- WebServiceTypeData.cs
- ObservableDictionary.cs
- AsyncResult.cs
- AuthenticationSection.cs
- UIntPtr.cs
- StandardTransformFactory.cs
- FormsAuthenticationEventArgs.cs
- XmlLoader.cs
- IteratorDescriptor.cs
- AudioFileOut.cs
- RegionInfo.cs
- XPathException.cs
- DataControlHelper.cs
- TreeViewImageIndexConverter.cs
- FixedDSBuilder.cs
- DataContractJsonSerializer.cs
- NativeMethods.cs
- Pointer.cs
- CqlBlock.cs
- MailMessage.cs
- CommandPlan.cs
- ToolbarAUtomationPeer.cs
- SendingRequestEventArgs.cs
- translator.cs
- SqlBulkCopyColumnMappingCollection.cs
- TransactionScope.cs
- DirtyTextRange.cs
- CLSCompliantAttribute.cs
- ResourcePart.cs
- PropagatorResult.cs
- WorkflowWebService.cs
- OutputCacheProfileCollection.cs
- ExecutionContext.cs
- XPathBinder.cs
- Typeface.cs
- StorageAssociationSetMapping.cs
- HtmlInputHidden.cs