Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / XmlDataSourceNodeDescriptor.cs / 1 / XmlDataSourceNodeDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Drawing.Design; using System.Security.Permissions; using System.Text; using System.Web; using System.Web.UI; using System.Web.Util; using System.Xml; using System.Xml.XPath; using AttributeCollection = System.ComponentModel.AttributeCollection; ////// internal sealed class XmlDataSourceNodeDescriptor : ICustomTypeDescriptor, IXPathNavigable { private XmlNode _node; ////// Creates a new instance of XmlDataSourceView. /// public XmlDataSourceNodeDescriptor(XmlNode node) { Debug.Assert(node != null, "Did not expect null node"); _node = node; } AttributeCollection ICustomTypeDescriptor.GetAttributes() { return AttributeCollection.Empty; } string ICustomTypeDescriptor.GetClassName() { return GetType().Name; } string ICustomTypeDescriptor.GetComponentName() { return null; } TypeConverter ICustomTypeDescriptor.GetConverter() { return null; } EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return null; } PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return null; } object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return null; } EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return null; } EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attrs) { return null; } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return ((ICustomTypeDescriptor)this).GetProperties(null); } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attrFilter) { System.Collections.Generic.Listlist = new System.Collections.Generic.List (); XmlAttributeCollection attrs = _node.Attributes; if (attrs != null) { for (int i = 0; i < attrs.Count; i++) { list.Add(new XmlDataSourcePropertyDescriptor(attrs[i].Name)); } } return new PropertyDescriptorCollection(list.ToArray()); } object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { if (pd is XmlDataSourcePropertyDescriptor) { return this; } return null; } XPathNavigator IXPathNavigable.CreateNavigator() { return _node.CreateNavigator(); } private class XmlDataSourcePropertyDescriptor : PropertyDescriptor { private string _name; public XmlDataSourcePropertyDescriptor(string name) : base(name, null) { _name = name; } public override Type ComponentType { get { return typeof(XmlDataSourceNodeDescriptor); } } public override bool IsReadOnly { get { return true; } } public override Type PropertyType { get { return typeof(string); } } public override bool CanResetValue(object o) { return false; } public override object GetValue(object o) { XmlDataSourceNodeDescriptor node = o as XmlDataSourceNodeDescriptor; if (node != null) { XmlAttributeCollection attrs = node._node.Attributes; if (attrs != null) { XmlAttribute attr = attrs[_name]; if (attr != null) { return attr.Value; } } } return String.Empty; } public override void ResetValue(object o) { } public override void SetValue(object o, object value) { } public override bool ShouldSerializeValue(object o) { return true; } } } }
Link Menu
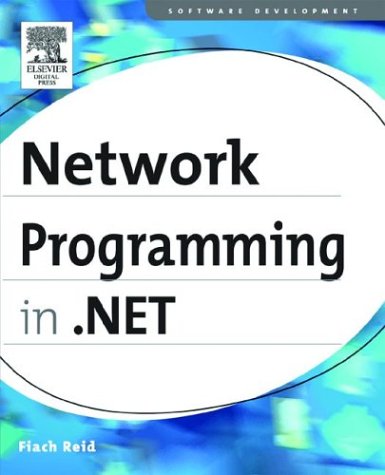
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConstructorArgumentAttribute.cs
- NonParentingControl.cs
- StateBag.cs
- UnsafeMethods.cs
- StreamingContext.cs
- ConstraintStruct.cs
- MetaType.cs
- TextPointerBase.cs
- XmlDataSource.cs
- x509utils.cs
- AlternateViewCollection.cs
- MemberDomainMap.cs
- User.cs
- ParameterToken.cs
- DynamicObjectAccessor.cs
- BaseCodeDomTreeGenerator.cs
- GeometryValueSerializer.cs
- NullableBoolConverter.cs
- LazyTextWriterCreator.cs
- InputLangChangeRequestEvent.cs
- RoutedEventConverter.cs
- CompilerLocalReference.cs
- TagMapInfo.cs
- HttpContextWrapper.cs
- IdentityReference.cs
- XPathEmptyIterator.cs
- GACMembershipCondition.cs
- DBBindings.cs
- ItemCollection.cs
- BitArray.cs
- _HeaderInfo.cs
- XPathCompileException.cs
- SchemaHelper.cs
- UnauthorizedAccessException.cs
- SqlConnectionHelper.cs
- InvalidOleVariantTypeException.cs
- CollaborationHelperFunctions.cs
- CharacterString.cs
- DataContract.cs
- HybridDictionary.cs
- DataGridViewTextBoxColumn.cs
- WinEventTracker.cs
- CatalogZoneBase.cs
- ToolStripItemEventArgs.cs
- DataViewSetting.cs
- ActivityInterfaces.cs
- TextFormatterHost.cs
- Accessors.cs
- HttpCachePolicyWrapper.cs
- PerfCounterSection.cs
- WinEventQueueItem.cs
- AutoResetEvent.cs
- EntityCommandDefinition.cs
- ToolConsole.cs
- ProviderException.cs
- WebPartUserCapability.cs
- HostedHttpRequestAsyncResult.cs
- HandleRef.cs
- Label.cs
- BehaviorDragDropEventArgs.cs
- MimeMultiPart.cs
- SortedList.cs
- ConnectionConsumerAttribute.cs
- Instrumentation.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- XmlElementAttributes.cs
- ProjectionPathBuilder.cs
- ResourcePermissionBase.cs
- XmlDataCollection.cs
- TextEditorParagraphs.cs
- MembershipValidatePasswordEventArgs.cs
- SchemaLookupTable.cs
- DataGridClipboardHelper.cs
- ApplicationHost.cs
- ContentFileHelper.cs
- SchemeSettingElement.cs
- ManagedCodeMarkers.cs
- IteratorFilter.cs
- AccessText.cs
- ListSourceHelper.cs
- RuntimeResourceSet.cs
- SqlConnectionString.cs
- GraphicsPath.cs
- HtmlElementErrorEventArgs.cs
- ButtonAutomationPeer.cs
- PhysicalOps.cs
- ListViewUpdateEventArgs.cs
- Matrix3DConverter.cs
- LocatorGroup.cs
- ImpersonationContext.cs
- ElementProxy.cs
- ComboBox.cs
- HttpTransportSecurity.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- XmlSchemaCompilationSettings.cs
- FixedSOMTable.cs
- ArgumentOutOfRangeException.cs
- ClientConvert.cs
- PersistenceTypeAttribute.cs
- TagMapCollection.cs