Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / HandleDictionary.cs / 1 / HandleDictionary.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using Microsoft.InfoCards.Diagnostics; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Xml; // // Summary: // Implements a dictionary of Handles. The GetNewHandle method will return a handle value that is guaranteed // to be unique, an object of type TVal can then be associated with that handle value. // internal class HandleDictionary< TVal > { const int m_MaxSessionCount = Int32.MaxValue / 2; Dictionary< int, TVal > m_internalDictionary; Random m_random; // // Summary: // Returns the max number of handles this dictionary can contain. // public int MaxSize { get { return m_MaxSessionCount; } } // // Summary: // Gets the object associated with the handle value passed in as Key. // public TVal this[ int Key ] { get { return m_internalDictionary[ Key ]; } set { if ( !ContainsHandle( Key ) ) { throw IDT.ThrowHelperError( new KeyNotFoundException() ); } m_internalDictionary[ Key ] = value; } } public HandleDictionary() { m_internalDictionary = new Dictionary< int, TVal >(); m_random = new Random(); } public int GetNewHandle() { // // Limit the number of crypto sessions to make certain that // finding a random id is efficient. // if( m_internalDictionary.Count >= m_MaxSessionCount ) { throw IDT.ThrowHelperError( new MaxSessionCountExceededException() ); } // // Look for an open slot. // int handle = m_random.Next(); while( m_internalDictionary.ContainsKey( handle ) || 0 == handle ) { handle = m_random.Next(); } // // Reserve a spot for this handle. // m_internalDictionary[ handle ] = default( TVal ); return handle; } // // Summary: // Removes a handle and it's value from the collection. // public bool Remove( int key ) { return m_internalDictionary.Remove( key ); } // // Summary: // Returns true if the handle value is contained in the collection. // public bool ContainsHandle( int key ) { return m_internalDictionary.ContainsKey( key ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
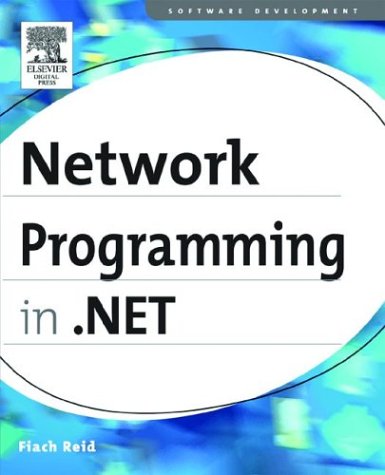
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DropDownList.cs
- QuotedPrintableStream.cs
- WindowProviderWrapper.cs
- ModifierKeysConverter.cs
- BulletedListEventArgs.cs
- Overlapped.cs
- EllipseGeometry.cs
- QilPatternVisitor.cs
- DataListDesigner.cs
- XmlnsCache.cs
- OracleConnection.cs
- InvalidDataException.cs
- SchemaConstraints.cs
- TdsParserStateObject.cs
- XXXInfos.cs
- LambdaCompiler.cs
- ProviderIncompatibleException.cs
- XmlAnyElementAttribute.cs
- MessageQueueEnumerator.cs
- FontEmbeddingManager.cs
- IChannel.cs
- WMIInterop.cs
- EntityDataSourceDataSelection.cs
- FontFamily.cs
- ScrollBar.cs
- BaseAsyncResult.cs
- ToolStripItemImageRenderEventArgs.cs
- GenericTypeParameterBuilder.cs
- ExpressionTextBox.xaml.cs
- ControlCodeDomSerializer.cs
- OLEDB_Util.cs
- FormViewRow.cs
- SchemaCompiler.cs
- MouseDevice.cs
- WindowsSysHeader.cs
- HandlerBase.cs
- DataGridViewTextBoxColumn.cs
- Model3DCollection.cs
- SafeFileMapViewHandle.cs
- ExpressionDumper.cs
- ContextQuery.cs
- DefaultTraceListener.cs
- EmptyQuery.cs
- ListControlActionList.cs
- AttachedAnnotationChangedEventArgs.cs
- InstanceKeyCompleteException.cs
- DelegatingTypeDescriptionProvider.cs
- InputEventArgs.cs
- EventQueueState.cs
- MessageHeaderException.cs
- WhileDesigner.cs
- StoreItemCollection.cs
- TraceSection.cs
- XmlDeclaration.cs
- Composition.cs
- MultipleViewPattern.cs
- LogAppendAsyncResult.cs
- XmlChoiceIdentifierAttribute.cs
- EmptyStringExpandableObjectConverter.cs
- Win32.cs
- coordinator.cs
- CharEntityEncoderFallback.cs
- GraphicsPath.cs
- CommandTreeTypeHelper.cs
- UnauthorizedWebPart.cs
- DataGridViewCellStyleChangedEventArgs.cs
- ImageButton.cs
- XmlSchemaAttributeGroupRef.cs
- safesecurityhelperavalon.cs
- XmlILIndex.cs
- AccessorTable.cs
- WebConfigurationHostFileChange.cs
- BlurBitmapEffect.cs
- ChannelProtectionRequirements.cs
- MessageBox.cs
- UDPClient.cs
- MenuEventArgs.cs
- CallTemplateAction.cs
- Line.cs
- OperatorExpressions.cs
- AuthorizationRule.cs
- PathFigure.cs
- WebPartCatalogAddVerb.cs
- BinaryVersion.cs
- TraceHandler.cs
- ChtmlTextWriter.cs
- ToolStripDropDownButton.cs
- DecoderReplacementFallback.cs
- JapaneseCalendar.cs
- DbConnectionPoolOptions.cs
- SafeThreadHandle.cs
- ReadOnlyDataSource.cs
- ClientFormsIdentity.cs
- XmlSchemaAttributeGroupRef.cs
- SqlAggregateChecker.cs
- RunWorkerCompletedEventArgs.cs
- SystemDiagnosticsSection.cs
- RuleSetReference.cs
- dataprotectionpermissionattribute.cs
- LoaderAllocator.cs