Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / SafeMILHandle.cs / 2 / SafeMILHandle.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // A safe way to deal with unmanaged MIL interface pointers. //--------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections; using System.Reflection; using MS.Internal; using MS.Win32; using System.Diagnostics; using System.Windows.Media; using System.Runtime; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using Microsoft.Win32.SafeHandles; using Microsoft.Internal; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { internal class SafeMILHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle isn't ready yet and later /// set the handle with SetHandle. SafeMILHandle owns the release /// of the handle. /// ////// Critical: This derives from a class that has a link demand and inheritance demand /// TreatAsSafe: Ok to call constructor /// [SecurityCritical,SecurityTreatAsSafe] internal SafeMILHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// SafeMILHandle owns the release of the parameter. /// ////// Critical: This code calls UpdateEstimatedSize. /// It is used to keep memory around /// [SecurityCritical] internal SafeMILHandle(IntPtr handle, long estimatedSize) : base(true) { SetHandle(handle); // // Hint the GC at the size of the unmanaged memory associated with // this object. We release pressure in the finalizer. // UpdateEstimatedSize(estimatedSize); } ////// Change our size to the new size specified /// ////// Critical: This code calls into AddMemoryPressure and RemoveMemoryPressure /// both of which have link demands. It is used to keep memory around /// [SecurityCritical] internal void UpdateEstimatedSize(long estimatedSize) { if (_gcPressure > 0) { MemoryPressure.Remove(_gcPressure); } _gcPressure = estimatedSize; if (_gcPressure > 0) { MemoryPressure.Add(_gcPressure); } } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid COM object. /// [SecurityCritical] protected override bool ReleaseHandle() { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref handle); // // We've released the unmangaed memory, so remove associated // GC pressure. // UpdateEstimatedSize(0); return true; } // // Estimated size in bytes of the unmanaged memory we are holding onto // private long _gcPressure; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // A safe way to deal with unmanaged MIL interface pointers. //--------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections; using System.Reflection; using MS.Internal; using MS.Win32; using System.Diagnostics; using System.Windows.Media; using System.Runtime; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using Microsoft.Win32.SafeHandles; using Microsoft.Internal; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { internal class SafeMILHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle isn't ready yet and later /// set the handle with SetHandle. SafeMILHandle owns the release /// of the handle. /// ////// Critical: This derives from a class that has a link demand and inheritance demand /// TreatAsSafe: Ok to call constructor /// [SecurityCritical,SecurityTreatAsSafe] internal SafeMILHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// SafeMILHandle owns the release of the parameter. /// ////// Critical: This code calls UpdateEstimatedSize. /// It is used to keep memory around /// [SecurityCritical] internal SafeMILHandle(IntPtr handle, long estimatedSize) : base(true) { SetHandle(handle); // // Hint the GC at the size of the unmanaged memory associated with // this object. We release pressure in the finalizer. // UpdateEstimatedSize(estimatedSize); } ////// Change our size to the new size specified /// ////// Critical: This code calls into AddMemoryPressure and RemoveMemoryPressure /// both of which have link demands. It is used to keep memory around /// [SecurityCritical] internal void UpdateEstimatedSize(long estimatedSize) { if (_gcPressure > 0) { MemoryPressure.Remove(_gcPressure); } _gcPressure = estimatedSize; if (_gcPressure > 0) { MemoryPressure.Add(_gcPressure); } } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid COM object. /// [SecurityCritical] protected override bool ReleaseHandle() { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref handle); // // We've released the unmangaed memory, so remove associated // GC pressure. // UpdateEstimatedSize(0); return true; } // // Estimated size in bytes of the unmanaged memory we are holding onto // private long _gcPressure; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
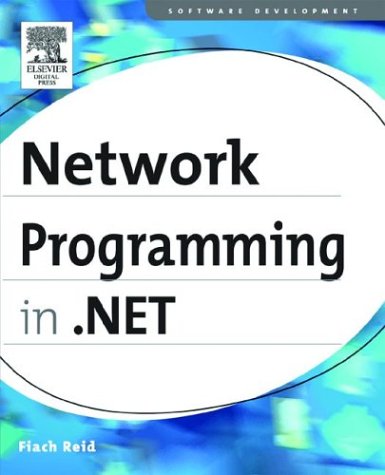
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberDomainMap.cs
- SQLByteStorage.cs
- ModulesEntry.cs
- CacheDependency.cs
- ValidatorUtils.cs
- StaticContext.cs
- FixedTextBuilder.cs
- ListView.cs
- VersionUtil.cs
- Funcletizer.cs
- PackageRelationshipCollection.cs
- BaseDataListPage.cs
- DocumentViewerBase.cs
- SpeechDetectedEventArgs.cs
- ServiceObjectContainer.cs
- ImageDrawing.cs
- RepeaterCommandEventArgs.cs
- Light.cs
- ClientRolePrincipal.cs
- Point3DAnimation.cs
- SBCSCodePageEncoding.cs
- ProxyDataContractResolver.cs
- XmlSchemaComplexContentRestriction.cs
- RectIndependentAnimationStorage.cs
- ContextMenuAutomationPeer.cs
- ImportCatalogPart.cs
- GenericEnumConverter.cs
- messageonlyhwndwrapper.cs
- InvalidPropValue.cs
- ValidationSummary.cs
- SqlAliasesReferenced.cs
- Rule.cs
- Expression.cs
- Expressions.cs
- VSWCFServiceContractGenerator.cs
- Authorization.cs
- AtomicFile.cs
- AppDomainUnloadedException.cs
- TextEditorThreadLocalStore.cs
- AttachmentCollection.cs
- StrokeNodeEnumerator.cs
- BrowserInteropHelper.cs
- ByteStorage.cs
- Resources.Designer.cs
- Span.cs
- ObjectStateManagerMetadata.cs
- PropertyCondition.cs
- Enum.cs
- CellCreator.cs
- _UncName.cs
- NavigationService.cs
- DeferredReference.cs
- EdmEntityTypeAttribute.cs
- MimeParameterWriter.cs
- DetailsViewDesigner.cs
- HitTestParameters.cs
- LockCookie.cs
- DataSvcMapFile.cs
- InputScope.cs
- WebProxyScriptElement.cs
- MasterPageParser.cs
- HiddenFieldPageStatePersister.cs
- SocketElement.cs
- DependencyProperty.cs
- WebSysDescriptionAttribute.cs
- ZipPackagePart.cs
- EllipticalNodeOperations.cs
- XmlSchemaInclude.cs
- BrowserCapabilitiesFactoryBase.cs
- HttpDebugHandler.cs
- Component.cs
- ToolStripItemImageRenderEventArgs.cs
- NodeFunctions.cs
- AggregatePushdown.cs
- Maps.cs
- FileSecurity.cs
- SqlProvider.cs
- GridView.cs
- DataGridViewRowsRemovedEventArgs.cs
- SafeEventLogReadHandle.cs
- SafeEventLogReadHandle.cs
- DataFieldConverter.cs
- FrameworkElement.cs
- TextDecorationCollection.cs
- PageThemeBuildProvider.cs
- SafeNativeMethodsMilCoreApi.cs
- SoapExtension.cs
- bidPrivateBase.cs
- DataSetUtil.cs
- Timeline.cs
- InvokeGenerator.cs
- OdbcDataReader.cs
- XmlTextAttribute.cs
- ToolStripComboBox.cs
- ColorTransform.cs
- HwndAppCommandInputProvider.cs
- UnknownBitmapDecoder.cs
- FlagsAttribute.cs
- propertyentry.cs
- EntityProviderServices.cs