Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / BidOverLoads.cs / 1305376 / BidOverLoads.cs
//------------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //----------------------------------------------------------------------------------- // // Note: Supressions for the FxCop rule CA2129 were added as it is being incorrectly flagged in this case. // SecuritySafeCritical code is allowed to call non-public SecurityCritical code. // using System; using System.Text; using System.Security; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Runtime.Versioning; internal static partial class EntityBid { private const string dllName = "System.Data.dll, " + AssemblyRef.SystemData; #region PutStr internal static void PutStrChunked(System.String str) { // It sure would be nice if this were something that didn't have to be chunked... if (TraceOn && IsInitialized) { RawPutStrChuncked(str); } } // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] private static void RawPutStrChuncked(System.String str) { for (int i = 0; i < str.Length; i += 1000) { NativeMethods.PutStr(modID, UIntPtr.Zero, UIntPtr.Zero, str.Substring(i, Math.Min(str.Length - i, 1000))); } } #endregion #region PlanCompiler internal static bool PlanCompilerOn { get { return IsOn(ApiGroup.PlanCompiler) && (IsInitialized); } } internal static void PlanCompilerPutStr(System.String str) { if (PlanCompilerOn) { RawPutStrChuncked(str); } } // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal static void PlanCompilerTrace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (PlanCompilerOn) NativeMethods.Trace(modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } #endregion #region ScopeAuto internal struct ScopeAuto : IDisposable { // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal ScopeAuto(string fmtPrintfW, System.Int32 arg) { if (EntityBid.ScopeOn && EntityBid.IsInitialized) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out _hscp, fmtPrintfW, arg); } else { _hscp = EntityBid.NoData; } } // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal ScopeAuto(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (EntityBid.ScopeOn && EntityBid.IsInitialized) { NativeMethods.ScopeEnter(modID, UIntPtr.Zero, UIntPtr.Zero, out _hscp, fmtPrintfW, a1, a2); } else { _hscp = EntityBid.NoData; } } // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] public void Dispose() { if (EntityBid.ScopeOn && EntityBid.IsInitialized && _hscp != EntityBid.NoData) { NativeMethods.ScopeLeave(modID, UIntPtr.Zero, UIntPtr.Zero, ref _hscp); } // NOTE: In contrast with standalone ScopeLeave, // there is no need to assign "EntityBid.NoData" to _hscp. } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] private IntPtr _hscp; } #endregion #region Trace with 1 parameter // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal static void Trace(string fmtPrintfW, System.Int32 a1) { if (EntityBid.TraceOn && EntityBid.IsInitialized) NativeMethods.Trace(modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1); } #endregion #region Trace with 2 parameters // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2) { if (EntityBid.TraceOn && EntityBid.IsInitialized) NativeMethods.Trace(modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.String a2) { if (EntityBid.TraceOn && EntityBid.IsInitialized) NativeMethods.Trace(modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal static void Trace(string fmtPrintfW, System.String a1, System.String a2) { if (EntityBid.TraceOn && EntityBid.IsInitialized) NativeMethods.Trace(modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2); } #endregion #region Trace with 3 parameters // See note in file header about this suppression. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2129:SecurityTransparentCodeShouldNotReferenceNonpublicSecurityCriticalCode")] [SecuritySafeCritical] [EntityBidMethod] internal static void Trace(string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3) { if (EntityBid.TraceOn && EntityBid.IsInitialized) NativeMethods.Trace(modID, UIntPtr.Zero, UIntPtr.Zero, fmtPrintfW, a1, a2, a3); } #endregion // // Interop calls to pluggable hooks [SuppressUnmanagedCodeSecurity] applied // [SuppressUnmanagedCodeSecurity] private static partial class NativeMethods { #region Trace with 1 parameter [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] [ResourceExposure(ResourceScope.None)] //The method does not expose any resource extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1); #endregion #region Trace with 2 parameters [ResourceExposure(ResourceScope.None)] //The method does not expose any resource [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int64 a2); [ResourceExposure(ResourceScope.None)] //The method does not expose any resource [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2); [ResourceExposure(ResourceScope.None)] //The method does not expose any resource [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.String a1, System.String a2); #endregion #region Trace with 3 parameters [ResourceExposure(ResourceScope.None)] //The method does not expose any resource [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3); [ResourceExposure(ResourceScope.None)] //The method does not expose any resource [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] [ResourceExposure(ResourceScope.None)] //The method does not expose any resource extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.String a2, System.String a3); #endregion #region Trace with 4 parameters [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] [ResourceExposure(ResourceScope.None)] //The method does not expose any resource extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.Int32 a3, System.Int32 a4); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] [DllImport(dllName, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.Cdecl, EntryPoint = "DllBidTraceCW")] [ResourceExposure(ResourceScope.None)] //The method does not expose any resource extern internal static void Trace(IntPtr hID, UIntPtr src, UIntPtr info, string fmtPrintfW, System.Int32 a1, System.Int32 a2, System.String a3, System.String a4); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
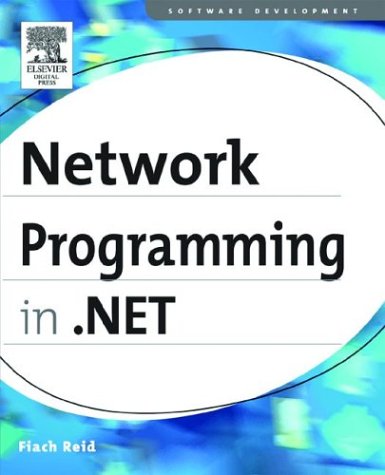
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataArtifactLoaderFile.cs
- ModifierKeysValueSerializer.cs
- XmlComment.cs
- DataRelationCollection.cs
- UTF8Encoding.cs
- _ProxyChain.cs
- SortQuery.cs
- SqlNotificationEventArgs.cs
- SqlUtil.cs
- MonikerHelper.cs
- MetadataItemSerializer.cs
- HttpPostServerProtocol.cs
- DataGridViewTextBoxColumn.cs
- XmlSchemaProviderAttribute.cs
- DataGridViewCellValueEventArgs.cs
- WsdlBuildProvider.cs
- SafeArchiveContext.cs
- RtType.cs
- FontDialog.cs
- PermissionRequestEvidence.cs
- WinInetCache.cs
- TreeNodeMouseHoverEvent.cs
- EventProxy.cs
- FormViewPageEventArgs.cs
- MetadataCollection.cs
- OperationCanceledException.cs
- SafeRightsManagementEnvironmentHandle.cs
- QilLiteral.cs
- MessageQueue.cs
- HtmlWindowCollection.cs
- EventListener.cs
- Trace.cs
- AttributeInfo.cs
- UserMapPath.cs
- SoapFormatterSinks.cs
- SafeBitVector32.cs
- SqlDataSourceFilteringEventArgs.cs
- XmlNodeReader.cs
- OutputCacheSection.cs
- Roles.cs
- EnumMemberAttribute.cs
- ModifierKeysValueSerializer.cs
- ModelItemDictionary.cs
- SqlCacheDependencySection.cs
- UnsafeNativeMethodsMilCoreApi.cs
- FrameworkContentElement.cs
- ClosableStream.cs
- Page.cs
- SqlDependencyUtils.cs
- SqlDataSourceView.cs
- PersonalizationDictionary.cs
- SafeRightsManagementQueryHandle.cs
- TrustLevelCollection.cs
- SByteStorage.cs
- Transform3DCollection.cs
- FixedSOMImage.cs
- ModuleConfigurationInfo.cs
- DataGridViewCellCollection.cs
- ExpressionConverter.cs
- RpcAsyncResult.cs
- WindowsAuthenticationEventArgs.cs
- ImageConverter.cs
- TCPListener.cs
- TextServicesContext.cs
- WindowsListViewGroupSubsetLink.cs
- WindowsSpinner.cs
- Pens.cs
- XmlQueryTypeFactory.cs
- ContractAdapter.cs
- AuthenticatedStream.cs
- SortDescriptionCollection.cs
- HttpStreamXmlDictionaryReader.cs
- SchemaImporter.cs
- AbandonedMutexException.cs
- X509ClientCertificateCredentialsElement.cs
- OutOfProcStateClientManager.cs
- CustomErrorsSectionWrapper.cs
- ListViewPagedDataSource.cs
- XamlWriter.cs
- JavascriptXmlWriterWrapper.cs
- WebPartEditorOkVerb.cs
- ConnectionOrientedTransportChannelFactory.cs
- WebPartsPersonalization.cs
- ValidatingPropertiesEventArgs.cs
- DocumentXPathNavigator.cs
- RSAPKCS1KeyExchangeFormatter.cs
- Substitution.cs
- _SSPIWrapper.cs
- QueueProcessor.cs
- Module.cs
- HwndSourceParameters.cs
- ComponentResourceKey.cs
- RotateTransform3D.cs
- XmlElement.cs
- ParseElementCollection.cs
- RTLAwareMessageBox.cs
- ActivityCodeDomReferenceService.cs
- isolationinterop.cs
- DateTimeFormatInfoScanner.cs
- XmlSchemaInfo.cs