Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / Sockets / TCPListener.cs / 1305376 / TCPListener.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Sockets { using System; using System.Net; using System.Security.Permissions; ////// public class TcpListener { IPEndPoint m_ServerSocketEP; Socket m_ServerSocket; bool m_Active; bool m_ExclusiveAddressUse; ///The ///class provide TCP services at a higher level of abstraction than the /// class. is used to create a host process that /// listens for connections from TCP clients. /// public TcpListener(IPEndPoint localEP) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "TcpListener", localEP); if (localEP == null) { throw new ArgumentNullException("localEP"); } m_ServerSocketEP = localEP; m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if(Logging.On)Logging.Exit(Logging.Sockets, this, "TcpListener", null); } ////// Initializes a new instance of the TcpListener class with the specified local /// end point. /// ////// public TcpListener(IPAddress localaddr, int port) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "TcpListener", localaddr); if (localaddr == null) { throw new ArgumentNullException("localaddr"); } if (!ValidationHelper.ValidateTcpPort(port)) { throw new ArgumentOutOfRangeException("port"); } m_ServerSocketEP = new IPEndPoint(localaddr, port); m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if(Logging.On)Logging.Exit(Logging.Sockets, this, "TcpListener", null); } // implementation picks an address for client ////// Initializes a new instance of the TcpListener class that listens to the /// specified IP address and port. /// ////// /// [Obsolete("This method has been deprecated. Please use TcpListener(IPAddress localaddr, int port) instead. http://go.microsoft.com/fwlink/?linkid=14202")] public TcpListener(int port){ if (!ValidationHelper.ValidateTcpPort(port)) throw new ArgumentOutOfRangeException("port"); m_ServerSocketEP = new IPEndPoint(IPAddress.Any, port); m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); } ////// Initiailizes a new instance of the TcpListener class /// that listens on the specified /// port. /// ////// public Socket Server { get { return m_ServerSocket; } } ////// Used by the class to provide the underlying network socket. /// ////// protected bool Active { get { return m_Active; } } ////// Used /// by the class to indicate that the listener's socket has been bound to a port /// and started listening. /// ////// public EndPoint LocalEndpoint { get { return m_Active ? m_ServerSocket.LocalEndPoint : m_ServerSocketEP; } } public bool ExclusiveAddressUse { get { return m_ServerSocket.ExclusiveAddressUse; } set{ if (m_Active) { throw new InvalidOperationException(SR.GetString(SR.net_tcplistener_mustbestopped)); } m_ServerSocket.ExclusiveAddressUse = value; m_ExclusiveAddressUse = value; } } public void AllowNatTraversal(bool allowed) { if (m_Active) { throw new InvalidOperationException(SR.GetString(SR.net_tcplistener_mustbestopped)); } if (allowed) { m_ServerSocket.SetIPProtectionLevel(IPProtectionLevel.Unrestricted); } else { m_ServerSocket.SetIPProtectionLevel(IPProtectionLevel.EdgeRestricted); } } // Start/stop the listener ////// Gets the m_Active EndPoint for the local listener socket. /// ////// public void Start() { Start((int)SocketOptionName.MaxConnections); } public void Start(int backlog) { if (backlog > (int)SocketOptionName.MaxConnections || backlog < 0) { throw new ArgumentOutOfRangeException("backlog"); } if(Logging.On)Logging.Enter(Logging.Sockets, this, "Start", null); GlobalLog.Print("TCPListener::Start()"); if (m_ServerSocket == null) throw new InvalidOperationException(SR.GetString(SR.net_InvalidSocketHandle)); //already listening if (m_Active) { if(Logging.On)Logging.Exit(Logging.Sockets, this, "Start", null); return; } m_ServerSocket.Bind(m_ServerSocketEP); try { m_ServerSocket.Listen(backlog); } // When there is an exception unwind previous actions (bind etc) catch (SocketException) { Stop(); throw; } m_Active = true; if(Logging.On)Logging.Exit(Logging.Sockets, this, "Start", null); } ////// Starts listening to network requests. /// ////// public void Stop() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "Stop", null); GlobalLog.Print("TCPListener::Stop()"); if (m_ServerSocket != null) { m_ServerSocket.Close(); m_ServerSocket = null; } m_Active = false; m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if (m_ExclusiveAddressUse) { m_ServerSocket.ExclusiveAddressUse = true; } if(Logging.On)Logging.Exit(Logging.Sockets, this, "Stop", null); } // Determine if there are pending connections ////// Closes the network connection. /// ////// public bool Pending() { if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); return m_ServerSocket.Poll(0, SelectMode.SelectRead); } // Accept the first pending connection ////// Determine if there are pending connection requests. /// ////// public Socket AcceptSocket() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "AcceptSocket", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); Socket socket = m_ServerSocket.Accept(); if(Logging.On)Logging.Exit(Logging.Sockets, this, "AcceptSocket", socket); return socket; } // UEUE ////// Accepts a pending connection request. /// ////// public TcpClient AcceptTcpClient() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "AcceptTcpClient", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); Socket acceptedSocket = m_ServerSocket.Accept(); TcpClient returnValue = new TcpClient(acceptedSocket); if(Logging.On)Logging.Exit(Logging.Sockets, this, "AcceptTcpClient", returnValue); return returnValue; } //methods [HostProtection(ExternalThreading=true)] public IAsyncResult BeginAcceptSocket(AsyncCallback callback, object state) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "BeginAcceptSocket", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); IAsyncResult result = m_ServerSocket.BeginAccept(callback,state); if(Logging.On)Logging.Exit(Logging.Sockets, this, "BeginAcceptSocket", null); return result; } public Socket EndAcceptSocket(IAsyncResult asyncResult){ if(Logging.On)Logging.Enter(Logging.Sockets, this, "EndAcceptSocket", null); if (asyncResult == null) { throw new ArgumentNullException("asyncResult"); } LazyAsyncResult lazyResult = asyncResult as LazyAsyncResult; Socket asyncSocket = lazyResult == null ? null : lazyResult.AsyncObject as Socket; if (asyncSocket == null) { throw new ArgumentException(SR.GetString(SR.net_io_invalidasyncresult), "asyncResult"); } // This will throw ObjectDisposedException if Stop() has been called. Socket socket = asyncSocket.EndAccept(asyncResult); if(Logging.On)Logging.Exit(Logging.Sockets, this, "EndAcceptSocket", socket); return socket; } [HostProtection(ExternalThreading=true)] public IAsyncResult BeginAcceptTcpClient(AsyncCallback callback, object state) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "BeginAcceptTcpClient", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); IAsyncResult result = m_ServerSocket.BeginAccept(callback,state); if(Logging.On)Logging.Exit(Logging.Sockets, this, "BeginAcceptTcpClient", null); return result; } public TcpClient EndAcceptTcpClient(IAsyncResult asyncResult){ if(Logging.On)Logging.Enter(Logging.Sockets, this, "EndAcceptTcpClient", null); if (asyncResult == null) { throw new ArgumentNullException("asyncResult"); } LazyAsyncResult lazyResult = asyncResult as LazyAsyncResult; Socket asyncSocket = lazyResult == null ? null : lazyResult.AsyncObject as Socket; if (asyncSocket == null) { throw new ArgumentException(SR.GetString(SR.net_io_invalidasyncresult), "asyncResult"); } // This will throw ObjectDisposedException if Stop() has been called. Socket socket = asyncSocket.EndAccept(asyncResult); if(Logging.On)Logging.Exit(Logging.Sockets, this, "EndAcceptTcpClient", socket); return new TcpClient(socket); } }; // class TcpListener } // namespace System.Net.Sockets // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Sockets { using System; using System.Net; using System.Security.Permissions; ////// public class TcpListener { IPEndPoint m_ServerSocketEP; Socket m_ServerSocket; bool m_Active; bool m_ExclusiveAddressUse; ///The ///class provide TCP services at a higher level of abstraction than the /// class. is used to create a host process that /// listens for connections from TCP clients. /// public TcpListener(IPEndPoint localEP) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "TcpListener", localEP); if (localEP == null) { throw new ArgumentNullException("localEP"); } m_ServerSocketEP = localEP; m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if(Logging.On)Logging.Exit(Logging.Sockets, this, "TcpListener", null); } ////// Initializes a new instance of the TcpListener class with the specified local /// end point. /// ////// public TcpListener(IPAddress localaddr, int port) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "TcpListener", localaddr); if (localaddr == null) { throw new ArgumentNullException("localaddr"); } if (!ValidationHelper.ValidateTcpPort(port)) { throw new ArgumentOutOfRangeException("port"); } m_ServerSocketEP = new IPEndPoint(localaddr, port); m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if(Logging.On)Logging.Exit(Logging.Sockets, this, "TcpListener", null); } // implementation picks an address for client ////// Initializes a new instance of the TcpListener class that listens to the /// specified IP address and port. /// ////// /// [Obsolete("This method has been deprecated. Please use TcpListener(IPAddress localaddr, int port) instead. http://go.microsoft.com/fwlink/?linkid=14202")] public TcpListener(int port){ if (!ValidationHelper.ValidateTcpPort(port)) throw new ArgumentOutOfRangeException("port"); m_ServerSocketEP = new IPEndPoint(IPAddress.Any, port); m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); } ////// Initiailizes a new instance of the TcpListener class /// that listens on the specified /// port. /// ////// public Socket Server { get { return m_ServerSocket; } } ////// Used by the class to provide the underlying network socket. /// ////// protected bool Active { get { return m_Active; } } ////// Used /// by the class to indicate that the listener's socket has been bound to a port /// and started listening. /// ////// public EndPoint LocalEndpoint { get { return m_Active ? m_ServerSocket.LocalEndPoint : m_ServerSocketEP; } } public bool ExclusiveAddressUse { get { return m_ServerSocket.ExclusiveAddressUse; } set{ if (m_Active) { throw new InvalidOperationException(SR.GetString(SR.net_tcplistener_mustbestopped)); } m_ServerSocket.ExclusiveAddressUse = value; m_ExclusiveAddressUse = value; } } public void AllowNatTraversal(bool allowed) { if (m_Active) { throw new InvalidOperationException(SR.GetString(SR.net_tcplistener_mustbestopped)); } if (allowed) { m_ServerSocket.SetIPProtectionLevel(IPProtectionLevel.Unrestricted); } else { m_ServerSocket.SetIPProtectionLevel(IPProtectionLevel.EdgeRestricted); } } // Start/stop the listener ////// Gets the m_Active EndPoint for the local listener socket. /// ////// public void Start() { Start((int)SocketOptionName.MaxConnections); } public void Start(int backlog) { if (backlog > (int)SocketOptionName.MaxConnections || backlog < 0) { throw new ArgumentOutOfRangeException("backlog"); } if(Logging.On)Logging.Enter(Logging.Sockets, this, "Start", null); GlobalLog.Print("TCPListener::Start()"); if (m_ServerSocket == null) throw new InvalidOperationException(SR.GetString(SR.net_InvalidSocketHandle)); //already listening if (m_Active) { if(Logging.On)Logging.Exit(Logging.Sockets, this, "Start", null); return; } m_ServerSocket.Bind(m_ServerSocketEP); try { m_ServerSocket.Listen(backlog); } // When there is an exception unwind previous actions (bind etc) catch (SocketException) { Stop(); throw; } m_Active = true; if(Logging.On)Logging.Exit(Logging.Sockets, this, "Start", null); } ////// Starts listening to network requests. /// ////// public void Stop() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "Stop", null); GlobalLog.Print("TCPListener::Stop()"); if (m_ServerSocket != null) { m_ServerSocket.Close(); m_ServerSocket = null; } m_Active = false; m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if (m_ExclusiveAddressUse) { m_ServerSocket.ExclusiveAddressUse = true; } if(Logging.On)Logging.Exit(Logging.Sockets, this, "Stop", null); } // Determine if there are pending connections ////// Closes the network connection. /// ////// public bool Pending() { if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); return m_ServerSocket.Poll(0, SelectMode.SelectRead); } // Accept the first pending connection ////// Determine if there are pending connection requests. /// ////// public Socket AcceptSocket() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "AcceptSocket", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); Socket socket = m_ServerSocket.Accept(); if(Logging.On)Logging.Exit(Logging.Sockets, this, "AcceptSocket", socket); return socket; } // UEUE ////// Accepts a pending connection request. /// ////// public TcpClient AcceptTcpClient() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "AcceptTcpClient", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); Socket acceptedSocket = m_ServerSocket.Accept(); TcpClient returnValue = new TcpClient(acceptedSocket); if(Logging.On)Logging.Exit(Logging.Sockets, this, "AcceptTcpClient", returnValue); return returnValue; } //methods [HostProtection(ExternalThreading=true)] public IAsyncResult BeginAcceptSocket(AsyncCallback callback, object state) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "BeginAcceptSocket", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); IAsyncResult result = m_ServerSocket.BeginAccept(callback,state); if(Logging.On)Logging.Exit(Logging.Sockets, this, "BeginAcceptSocket", null); return result; } public Socket EndAcceptSocket(IAsyncResult asyncResult){ if(Logging.On)Logging.Enter(Logging.Sockets, this, "EndAcceptSocket", null); if (asyncResult == null) { throw new ArgumentNullException("asyncResult"); } LazyAsyncResult lazyResult = asyncResult as LazyAsyncResult; Socket asyncSocket = lazyResult == null ? null : lazyResult.AsyncObject as Socket; if (asyncSocket == null) { throw new ArgumentException(SR.GetString(SR.net_io_invalidasyncresult), "asyncResult"); } // This will throw ObjectDisposedException if Stop() has been called. Socket socket = asyncSocket.EndAccept(asyncResult); if(Logging.On)Logging.Exit(Logging.Sockets, this, "EndAcceptSocket", socket); return socket; } [HostProtection(ExternalThreading=true)] public IAsyncResult BeginAcceptTcpClient(AsyncCallback callback, object state) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "BeginAcceptTcpClient", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); IAsyncResult result = m_ServerSocket.BeginAccept(callback,state); if(Logging.On)Logging.Exit(Logging.Sockets, this, "BeginAcceptTcpClient", null); return result; } public TcpClient EndAcceptTcpClient(IAsyncResult asyncResult){ if(Logging.On)Logging.Enter(Logging.Sockets, this, "EndAcceptTcpClient", null); if (asyncResult == null) { throw new ArgumentNullException("asyncResult"); } LazyAsyncResult lazyResult = asyncResult as LazyAsyncResult; Socket asyncSocket = lazyResult == null ? null : lazyResult.AsyncObject as Socket; if (asyncSocket == null) { throw new ArgumentException(SR.GetString(SR.net_io_invalidasyncresult), "asyncResult"); } // This will throw ObjectDisposedException if Stop() has been called. Socket socket = asyncSocket.EndAccept(asyncResult); if(Logging.On)Logging.Exit(Logging.Sockets, this, "EndAcceptTcpClient", socket); return new TcpClient(socket); } }; // class TcpListener } // namespace System.Net.Sockets // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
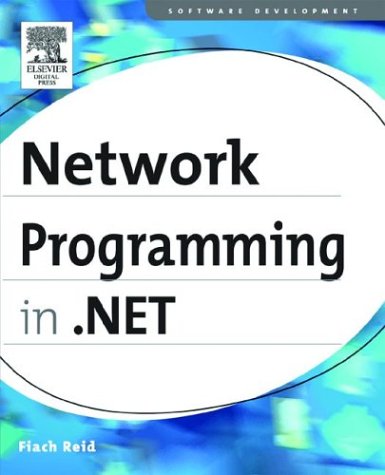
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IdentitySection.cs
- ColorInterpolationModeValidation.cs
- PtsHelper.cs
- PublisherIdentityPermission.cs
- FixedFindEngine.cs
- AxParameterData.cs
- WebServiceAttribute.cs
- StrongNameKeyPair.cs
- WebBrowserSiteBase.cs
- MimeAnyImporter.cs
- Content.cs
- SkipStoryboardToFill.cs
- ToolZoneDesigner.cs
- HtmlInputRadioButton.cs
- WinOEToolBoxItem.cs
- Expander.cs
- ProxyGenerator.cs
- TextContainer.cs
- BindingWorker.cs
- AttributeCollection.cs
- SubclassTypeValidator.cs
- FieldCollectionEditor.cs
- MdiWindowListStrip.cs
- SolidColorBrush.cs
- Executor.cs
- DataControlFieldCollection.cs
- SchemaImporterExtensionElement.cs
- SecurityContext.cs
- CultureSpecificStringDictionary.cs
- PropertyNames.cs
- TextShapeableCharacters.cs
- QueryStatement.cs
- XmlSchemaAll.cs
- IgnoreSectionHandler.cs
- RotateTransform3D.cs
- SqlExpressionNullability.cs
- X509Certificate2.cs
- RoutedEvent.cs
- SettingsPropertyWrongTypeException.cs
- ExceptionHandlersDesigner.cs
- Resources.Designer.cs
- CatalogPartChrome.cs
- TransformerTypeCollection.cs
- RelationshipType.cs
- DataBoundLiteralControl.cs
- ProtectedConfigurationSection.cs
- QEncodedStream.cs
- OleDbRowUpdatingEvent.cs
- FlowLayoutPanel.cs
- ObjectDataProvider.cs
- PropertyEntry.cs
- RotationValidation.cs
- RelationshipEndCollection.cs
- WindowsListView.cs
- EndpointFilterProvider.cs
- Fx.cs
- DynamicRenderer.cs
- StaticExtension.cs
- DataControlCommands.cs
- ShaderRenderModeValidation.cs
- EntityDataSourceContainerNameItem.cs
- XsdDuration.cs
- XPathException.cs
- DifferencingCollection.cs
- Vector.cs
- XmlNamespaceMappingCollection.cs
- EncoderExceptionFallback.cs
- Hyperlink.cs
- TypeConverter.cs
- GridEntryCollection.cs
- SqlDataRecord.cs
- BatchStream.cs
- MsmqHostedTransportManager.cs
- ManagementObjectSearcher.cs
- FileDataSourceCache.cs
- RangeValidator.cs
- xmlfixedPageInfo.cs
- NegationPusher.cs
- ZipIOExtraFieldZip64Element.cs
- CommentEmitter.cs
- Nodes.cs
- AffineTransform3D.cs
- codemethodreferenceexpression.cs
- SafeBitVector32.cs
- HwndSourceParameters.cs
- PopOutPanel.cs
- TransformerTypeCollection.cs
- RolePrincipal.cs
- TypeUnloadedException.cs
- ScriptControlDescriptor.cs
- LiteralControl.cs
- EntityDesignerBuildProvider.cs
- TabItemWrapperAutomationPeer.cs
- NameGenerator.cs
- NativeWindow.cs
- BaseDataListDesigner.cs
- DataGridViewTopLeftHeaderCell.cs
- OleDbEnumerator.cs
- ItemType.cs
- JsonWriter.cs