Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / GenericArgumentsUpdater.cs / 1305376 / GenericArgumentsUpdater.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Activities; using System.Diagnostics; using System.Linq; using System.Runtime; using System.Activities.Presentation.View; using System.Windows.Threading; class GenericArgumentUpdater { EditingContext context; const string TypeArgumentPropertyName = "TypeArgument"; public GenericArgumentUpdater(EditingContext context) { this.context = context; } public void AddSupportForUpdatingTypeArgument(Type modelItemType) { AttachedPropertytypeArgumentProperty = new AttachedProperty { Name = TypeArgumentPropertyName, OwnerType = modelItemType, Getter = (modelItem) => GetTypeArgument(modelItem), Setter = (modelItem, value) => UpdateTypeArgument(modelItem, value), IsBrowsable = true }; this.context.Services.GetService ().AddProperty(typeArgumentProperty); } private static void UpdateTypeArgument(ModelItem modelItem, Type value) { Type oldModelItemType = modelItem.ItemType; Fx.Assert(oldModelItemType.GetGenericArguments().Count() == 1, "we only support changing a single type parameter ?"); Type newModelItemType = oldModelItemType.GetGenericTypeDefinition().MakeGenericType(value); Fx.Assert(newModelItemType != null, "New model item type needs to be non null or we cannot proceed further"); ModelItem newModelItem = ModelFactory.CreateItem(modelItem.GetEditingContext(), Activator.CreateInstance(newModelItemType)); MorphHelper.MorphObject(modelItem, newModelItem); MorphHelper.MorphProperties(modelItem, newModelItem); if (oldModelItemType.IsSubclassOf(typeof(Activity)) && newModelItemType.IsSubclassOf(typeof(Activity))) { if (string.Equals((string)modelItem.Properties["DisplayName"].ComputedValue, GetActivityDefaultName(oldModelItemType), StringComparison.Ordinal)) { newModelItem.Properties["DisplayName"].SetValue(GetActivityDefaultName(newModelItemType)); } } DesignerView designerView = modelItem.GetEditingContext().Services.GetService (); if (designerView != null) { Dispatcher.CurrentDispatcher.BeginInvoke(DispatcherPriority.Render, (Action)(() => { if (designerView.RootDesigner != null && ((WorkflowViewElement)designerView.RootDesigner).ModelItem == modelItem) { designerView.MakeRootDesigner(newModelItem, true); } Selection.SelectOnly(modelItem.GetEditingContext(), newModelItem); })); } } private static Type GetTypeArgument(ModelItem modelItem) { Fx.Assert(modelItem.ItemType.GetGenericArguments().Count() == 1, "we only support changing a single type parameter ?"); return modelItem.ItemType.GetGenericArguments()[0]; } private static string GetActivityDefaultName(Type activityType) { Fx.Assert(activityType.IsSubclassOf(typeof(Activity)), "activityType is not a subclass of System.Activities.Activity"); Activity activity = (Activity)Activator.CreateInstance(activityType); return activity.DisplayName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Activities; using System.Diagnostics; using System.Linq; using System.Runtime; using System.Activities.Presentation.View; using System.Windows.Threading; class GenericArgumentUpdater { EditingContext context; const string TypeArgumentPropertyName = "TypeArgument"; public GenericArgumentUpdater(EditingContext context) { this.context = context; } public void AddSupportForUpdatingTypeArgument(Type modelItemType) { AttachedProperty typeArgumentProperty = new AttachedProperty { Name = TypeArgumentPropertyName, OwnerType = modelItemType, Getter = (modelItem) => GetTypeArgument(modelItem), Setter = (modelItem, value) => UpdateTypeArgument(modelItem, value), IsBrowsable = true }; this.context.Services.GetService ().AddProperty(typeArgumentProperty); } private static void UpdateTypeArgument(ModelItem modelItem, Type value) { Type oldModelItemType = modelItem.ItemType; Fx.Assert(oldModelItemType.GetGenericArguments().Count() == 1, "we only support changing a single type parameter ?"); Type newModelItemType = oldModelItemType.GetGenericTypeDefinition().MakeGenericType(value); Fx.Assert(newModelItemType != null, "New model item type needs to be non null or we cannot proceed further"); ModelItem newModelItem = ModelFactory.CreateItem(modelItem.GetEditingContext(), Activator.CreateInstance(newModelItemType)); MorphHelper.MorphObject(modelItem, newModelItem); MorphHelper.MorphProperties(modelItem, newModelItem); if (oldModelItemType.IsSubclassOf(typeof(Activity)) && newModelItemType.IsSubclassOf(typeof(Activity))) { if (string.Equals((string)modelItem.Properties["DisplayName"].ComputedValue, GetActivityDefaultName(oldModelItemType), StringComparison.Ordinal)) { newModelItem.Properties["DisplayName"].SetValue(GetActivityDefaultName(newModelItemType)); } } DesignerView designerView = modelItem.GetEditingContext().Services.GetService (); if (designerView != null) { Dispatcher.CurrentDispatcher.BeginInvoke(DispatcherPriority.Render, (Action)(() => { if (designerView.RootDesigner != null && ((WorkflowViewElement)designerView.RootDesigner).ModelItem == modelItem) { designerView.MakeRootDesigner(newModelItem, true); } Selection.SelectOnly(modelItem.GetEditingContext(), newModelItem); })); } } private static Type GetTypeArgument(ModelItem modelItem) { Fx.Assert(modelItem.ItemType.GetGenericArguments().Count() == 1, "we only support changing a single type parameter ?"); return modelItem.ItemType.GetGenericArguments()[0]; } private static string GetActivityDefaultName(Type activityType) { Fx.Assert(activityType.IsSubclassOf(typeof(Activity)), "activityType is not a subclass of System.Activities.Activity"); Activity activity = (Activity)Activator.CreateInstance(activityType); return activity.DisplayName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
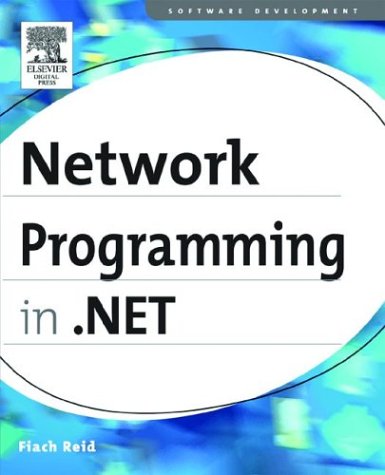
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinqToSqlWrapper.cs
- UIElementParagraph.cs
- AmbiguousMatchException.cs
- DBCommand.cs
- ToolbarAUtomationPeer.cs
- MediaTimeline.cs
- DropDownList.cs
- PathGeometry.cs
- SerialErrors.cs
- InfiniteIntConverter.cs
- SynchronizationLockException.cs
- HtmlControlPersistable.cs
- XmlILTrace.cs
- BuildProviderUtils.cs
- BitmapImage.cs
- TcpClientSocketManager.cs
- ValidationPropertyAttribute.cs
- CodeAttributeDeclaration.cs
- TreeNodeStyleCollectionEditor.cs
- AttributeTable.cs
- NullableLongSumAggregationOperator.cs
- VirtualDirectoryMapping.cs
- DataGridPreparingCellForEditEventArgs.cs
- ActivityStatusChangeEventArgs.cs
- RulePatternOps.cs
- SkinBuilder.cs
- TextTrailingWordEllipsis.cs
- StreamInfo.cs
- ArrangedElement.cs
- ScrollPattern.cs
- PageThemeParser.cs
- SendingRequestEventArgs.cs
- XmlAtomicValue.cs
- NonSerializedAttribute.cs
- Memoizer.cs
- EventlogProvider.cs
- HtmlMeta.cs
- ButtonFlatAdapter.cs
- ReferenceCountedObject.cs
- MeasureItemEvent.cs
- AllMembershipCondition.cs
- ViewBase.cs
- HideDisabledControlAdapter.cs
- Quaternion.cs
- ValueTable.cs
- SynchronizationFilter.cs
- MenuRendererStandards.cs
- DoubleAnimation.cs
- KnownAssembliesSet.cs
- CompositeActivityTypeDescriptorProvider.cs
- Operator.cs
- ClientSettings.cs
- Control.cs
- PointCollection.cs
- ReachDocumentReferenceSerializerAsync.cs
- XmlAttributeProperties.cs
- ImplicitInputBrush.cs
- InputLanguageProfileNotifySink.cs
- EventMappingSettings.cs
- ResourceSetExpression.cs
- WebPartRestoreVerb.cs
- CodeNamespaceImport.cs
- UrlPath.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- FlowLayoutPanel.cs
- MethodBuilder.cs
- XmlUtf8RawTextWriter.cs
- EventSetter.cs
- DataViewListener.cs
- SecuritySessionServerSettings.cs
- TypeSemantics.cs
- SystemWebCachingSectionGroup.cs
- XsltConvert.cs
- Processor.cs
- WithStatement.cs
- Queue.cs
- ResourceReferenceKeyNotFoundException.cs
- DefaultValidator.cs
- HierarchicalDataSourceControl.cs
- WindowsListViewGroupHelper.cs
- SafeReversePInvokeHandle.cs
- WebPartVerb.cs
- RowVisual.cs
- CodeDefaultValueExpression.cs
- ServiceProviders.cs
- Control.cs
- InvokeDelegate.cs
- UnitySerializationHolder.cs
- FusionWrap.cs
- XmlMtomReader.cs
- MenuRendererClassic.cs
- CatalogZone.cs
- InProcStateClientManager.cs
- Console.cs
- ScrollableControl.cs
- Annotation.cs
- OdbcConnection.cs
- EditingCoordinator.cs
- FileAuthorizationModule.cs
- XmlAnyAttributeAttribute.cs