Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / IlGen / XmlILTrace.cs / 1 / XmlILTrace.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Xml; using System.Globalization; using System.Xml.Xsl.Qil; namespace System.Xml.Xsl.IlGen { ////// Helper class that facilitates tracing of ILGen. /// internal static class XmlILTrace { private const int MAX_REWRITES = 200; ////// Check environment variable in order to determine whether to write out trace files. This really should be a /// check of the configuration file, but System.Xml does not yet have a good tracing story. /// private static string dirName = null; private static bool alreadyCheckedEnabled = false; ////// True if tracing has been enabled (environment variable set). /// public static bool IsEnabled { get { // If environment variable has not yet been checked, do so now if (!alreadyCheckedEnabled) { try { dirName = Environment.GetEnvironmentVariable("XmlILTrace"); } catch (SecurityException) { // If user does not have access to environment variables, tracing will remain disabled } alreadyCheckedEnabled = true; } return (dirName != null); } } ////// If tracing is enabled, this method will delete the contents of "filename" in preparation for append /// operations. /// public static void PrepareTraceWriter(string fileName) { if (!IsEnabled) return; File.Delete(dirName + "\\" + fileName); } ////// If tracing is enabled, this method will open a TextWriter over "fileName" and return it. Otherwise, /// null will be returned. /// public static TextWriter GetTraceWriter(string fileName) { if (!IsEnabled) return null; return new StreamWriter(dirName + "\\" + fileName, true); } ////// Serialize Qil tree to "fileName", in the directory identified by "dirName". /// public static void WriteQil(QilExpression qil, string fileName) { if (!IsEnabled) return; XmlWriter w = XmlWriter.Create(dirName + "\\" + fileName); try { WriteQil(qil, w); } finally { w.Close(); } } ////// Trace ILGen optimizations and log them to "fileName". /// public static void TraceOptimizations(QilExpression qil, string fileName) { if (!IsEnabled) return; XmlWriter w = XmlWriter.Create(dirName + "\\" + fileName); w.WriteStartDocument(); w.WriteProcessingInstruction("xml-stylesheet", "href='qilo.xslt' type='text/xsl'"); w.WriteStartElement("QilOptimizer"); w.WriteAttributeString("timestamp", DateTime.Now.ToString(CultureInfo.InvariantCulture)); WriteQilRewrite(qil, w, null); try { // Then, rewrite the graph until "done" or some max value is reached. for (int i = 1; i < MAX_REWRITES; i++) { QilExpression qilTemp = (QilExpression) (new QilCloneVisitor(qil.Factory).Clone(qil)); XmlILOptimizerVisitor visitor = new XmlILOptimizerVisitor(qilTemp, !qilTemp.IsDebug); visitor.Threshold = i; qilTemp = visitor.Optimize(); // In debug code, ensure that QIL after N steps is correct QilValidationVisitor.Validate(qilTemp); // Trace the rewrite WriteQilRewrite(qilTemp, w, OptimizationToString(visitor.LastReplacement)); if (visitor.ReplacementCount < i) break; } } catch (Exception e) { if (!XmlException.IsCatchableException(e)) { throw; } w.WriteElementString("Exception", null, e.ToString()); throw; } finally { w.WriteEndElement(); w.WriteEndDocument(); w.Flush(); w.Close(); } } ////// Serialize Qil tree to writer "w". /// private static void WriteQil(QilExpression qil, XmlWriter w) { QilXmlWriter qw = new QilXmlWriter(w); qw.ToXml(qil); } ////// Serialize rewritten Qil tree to writer "w". /// private static void WriteQilRewrite(QilExpression qil, XmlWriter w, string rewriteName) { w.WriteStartElement("Diff"); if (rewriteName != null) w.WriteAttributeString("rewrite", rewriteName); WriteQil(qil, w); w.WriteEndElement(); } ////// Get friendly string description of an ILGen optimization. /// private static string OptimizationToString(int opt) { string s = Enum.GetName(typeof(XmlILOptimization), opt); if (s.StartsWith("Introduce", StringComparison.Ordinal)) { return s.Substring(9) + " introduction"; } else if (s.StartsWith("Eliminate", StringComparison.Ordinal)) { return s.Substring(9) + " elimination"; } else if (s.StartsWith("Commute", StringComparison.Ordinal)) { return s.Substring(7) + " commutation"; } else if (s.StartsWith("Fold", StringComparison.Ordinal)) { return s.Substring(4) + " folding"; } else if (s.StartsWith("Misc", StringComparison.Ordinal)) { return s.Substring(4); } return s; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Xml; using System.Globalization; using System.Xml.Xsl.Qil; namespace System.Xml.Xsl.IlGen { ////// Helper class that facilitates tracing of ILGen. /// internal static class XmlILTrace { private const int MAX_REWRITES = 200; ////// Check environment variable in order to determine whether to write out trace files. This really should be a /// check of the configuration file, but System.Xml does not yet have a good tracing story. /// private static string dirName = null; private static bool alreadyCheckedEnabled = false; ////// True if tracing has been enabled (environment variable set). /// public static bool IsEnabled { get { // If environment variable has not yet been checked, do so now if (!alreadyCheckedEnabled) { try { dirName = Environment.GetEnvironmentVariable("XmlILTrace"); } catch (SecurityException) { // If user does not have access to environment variables, tracing will remain disabled } alreadyCheckedEnabled = true; } return (dirName != null); } } ////// If tracing is enabled, this method will delete the contents of "filename" in preparation for append /// operations. /// public static void PrepareTraceWriter(string fileName) { if (!IsEnabled) return; File.Delete(dirName + "\\" + fileName); } ////// If tracing is enabled, this method will open a TextWriter over "fileName" and return it. Otherwise, /// null will be returned. /// public static TextWriter GetTraceWriter(string fileName) { if (!IsEnabled) return null; return new StreamWriter(dirName + "\\" + fileName, true); } ////// Serialize Qil tree to "fileName", in the directory identified by "dirName". /// public static void WriteQil(QilExpression qil, string fileName) { if (!IsEnabled) return; XmlWriter w = XmlWriter.Create(dirName + "\\" + fileName); try { WriteQil(qil, w); } finally { w.Close(); } } ////// Trace ILGen optimizations and log them to "fileName". /// public static void TraceOptimizations(QilExpression qil, string fileName) { if (!IsEnabled) return; XmlWriter w = XmlWriter.Create(dirName + "\\" + fileName); w.WriteStartDocument(); w.WriteProcessingInstruction("xml-stylesheet", "href='qilo.xslt' type='text/xsl'"); w.WriteStartElement("QilOptimizer"); w.WriteAttributeString("timestamp", DateTime.Now.ToString(CultureInfo.InvariantCulture)); WriteQilRewrite(qil, w, null); try { // Then, rewrite the graph until "done" or some max value is reached. for (int i = 1; i < MAX_REWRITES; i++) { QilExpression qilTemp = (QilExpression) (new QilCloneVisitor(qil.Factory).Clone(qil)); XmlILOptimizerVisitor visitor = new XmlILOptimizerVisitor(qilTemp, !qilTemp.IsDebug); visitor.Threshold = i; qilTemp = visitor.Optimize(); // In debug code, ensure that QIL after N steps is correct QilValidationVisitor.Validate(qilTemp); // Trace the rewrite WriteQilRewrite(qilTemp, w, OptimizationToString(visitor.LastReplacement)); if (visitor.ReplacementCount < i) break; } } catch (Exception e) { if (!XmlException.IsCatchableException(e)) { throw; } w.WriteElementString("Exception", null, e.ToString()); throw; } finally { w.WriteEndElement(); w.WriteEndDocument(); w.Flush(); w.Close(); } } ////// Serialize Qil tree to writer "w". /// private static void WriteQil(QilExpression qil, XmlWriter w) { QilXmlWriter qw = new QilXmlWriter(w); qw.ToXml(qil); } ////// Serialize rewritten Qil tree to writer "w". /// private static void WriteQilRewrite(QilExpression qil, XmlWriter w, string rewriteName) { w.WriteStartElement("Diff"); if (rewriteName != null) w.WriteAttributeString("rewrite", rewriteName); WriteQil(qil, w); w.WriteEndElement(); } ////// Get friendly string description of an ILGen optimization. /// private static string OptimizationToString(int opt) { string s = Enum.GetName(typeof(XmlILOptimization), opt); if (s.StartsWith("Introduce", StringComparison.Ordinal)) { return s.Substring(9) + " introduction"; } else if (s.StartsWith("Eliminate", StringComparison.Ordinal)) { return s.Substring(9) + " elimination"; } else if (s.StartsWith("Commute", StringComparison.Ordinal)) { return s.Substring(7) + " commutation"; } else if (s.StartsWith("Fold", StringComparison.Ordinal)) { return s.Substring(4) + " folding"; } else if (s.StartsWith("Misc", StringComparison.Ordinal)) { return s.Substring(4); } return s; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
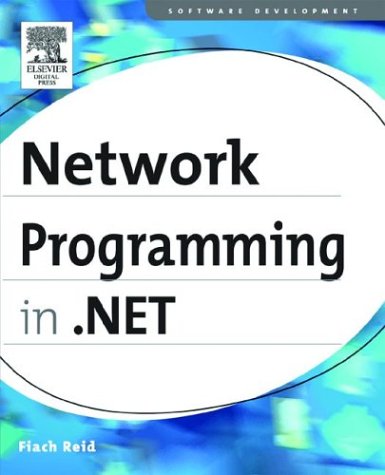
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509PeerCertificateAuthentication.cs
- EventToken.cs
- AuthorizationContext.cs
- RowsCopiedEventArgs.cs
- CardSpaceSelector.cs
- UriTemplateTrieNode.cs
- MutexSecurity.cs
- TableColumn.cs
- ObjectDataSource.cs
- _ListenerResponseStream.cs
- Qualifier.cs
- EventProviderWriter.cs
- StrokeDescriptor.cs
- BinaryFormatter.cs
- CacheMemory.cs
- PropertyChangedEventArgs.cs
- XmlReflectionImporter.cs
- WindowsTab.cs
- XsdBuilder.cs
- EntityContainerRelationshipSetEnd.cs
- CompilerTypeWithParams.cs
- ArraySortHelper.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- BitmapCodecInfo.cs
- UnmanagedMarshal.cs
- DefinitionBase.cs
- CustomAttributeSerializer.cs
- ICollection.cs
- RegexInterpreter.cs
- BindingSourceDesigner.cs
- StylusPointProperty.cs
- DataGridRow.cs
- ValidationErrorInfo.cs
- RowToParametersTransformer.cs
- itemelement.cs
- CreateUserWizard.cs
- TemplateBindingExtensionConverter.cs
- EditingCommands.cs
- TextChange.cs
- ListBoxItemAutomationPeer.cs
- AdRotator.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- TimeSpanParse.cs
- MultiView.cs
- IdentityHolder.cs
- UnsafeNetInfoNativeMethods.cs
- EncodingInfo.cs
- BrowserCapabilitiesCodeGenerator.cs
- SafeNativeMethods.cs
- TextTreeTextNode.cs
- CompositeKey.cs
- CodeSnippetTypeMember.cs
- StreamResourceInfo.cs
- XmlBaseReader.cs
- MissingManifestResourceException.cs
- RelationshipFixer.cs
- SizeF.cs
- XmlLoader.cs
- WSSecurityPolicy11.cs
- ClientData.cs
- WriteableBitmap.cs
- FileSystemWatcher.cs
- FontTypeConverter.cs
- BroadcastEventHelper.cs
- StringSorter.cs
- LinqTreeNodeEvaluator.cs
- SafeFileHandle.cs
- NavigateEvent.cs
- ToolboxComponentsCreatedEventArgs.cs
- UInt32Storage.cs
- HttpCacheParams.cs
- UiaCoreTypesApi.cs
- VSWCFServiceContractGenerator.cs
- GeneratedContractType.cs
- TraceUtils.cs
- SocketElement.cs
- ExtractedStateEntry.cs
- ShapeTypeface.cs
- securitymgrsite.cs
- ZoneMembershipCondition.cs
- RemotingException.cs
- ModelTreeEnumerator.cs
- SelectionItemProviderWrapper.cs
- PropertyMetadata.cs
- StoreAnnotationsMap.cs
- EventItfInfo.cs
- QueryCacheKey.cs
- ActivityInstanceMap.cs
- AlternationConverter.cs
- TextServicesManager.cs
- TraceHelpers.cs
- SerTrace.cs
- CompoundFileReference.cs
- App.cs
- IgnoreDataMemberAttribute.cs
- EncryptedKeyIdentifierClause.cs
- OwnerDrawPropertyBag.cs
- XPathParser.cs
- ListViewAutomationPeer.cs
- TextRunCache.cs