Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Common / QueryCache / QueryCacheKey.cs / 2 / QueryCacheKey.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Collections.Generic; using System.Text; ////// represents an abstract cache key /// internal abstract class QueryCacheKey { #region Constants protected const int EstimatedParameterStringSize = 20; #endregion #region Fields ////// entry hit counter /// private uint _hitCount; ////// aging index /// private int _agingIndex; ////// default string comparison kind - Ordinal /// protected static StringComparison _stringComparison = StringComparison.Ordinal; #endregion #region Constructor protected QueryCacheKey() { _hitCount = 1; } #endregion #region Abstract Methods ////// Determines whether two instances of QueryCacheContext are equal. /// Equality is value based. /// /// ///public abstract override bool Equals( object obj ); /// /// Returns QueryCacheContext instance HashCode /// ///public abstract override int GetHashCode(); #endregion #region Internal API /// /// Cache entry hit count /// internal uint HitCount { get { return _hitCount; } set { _hitCount = value; } } ////// Gets/Sets Aging index for cache entry /// internal int AgingIndex { get { return _agingIndex; } set { _agingIndex = value; } } ////// Updates hit count /// internal void UpdateHit() { if (uint.MaxValue != _hitCount) { unchecked { _hitCount++; } } } ////// default string comparer /// /// /// ///protected virtual bool Equals( string s, string t ) { return String.Equals(s, t, _stringComparison); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Collections.Generic; using System.Text; ////// represents an abstract cache key /// internal abstract class QueryCacheKey { #region Constants protected const int EstimatedParameterStringSize = 20; #endregion #region Fields ////// entry hit counter /// private uint _hitCount; ////// aging index /// private int _agingIndex; ////// default string comparison kind - Ordinal /// protected static StringComparison _stringComparison = StringComparison.Ordinal; #endregion #region Constructor protected QueryCacheKey() { _hitCount = 1; } #endregion #region Abstract Methods ////// Determines whether two instances of QueryCacheContext are equal. /// Equality is value based. /// /// ///public abstract override bool Equals( object obj ); /// /// Returns QueryCacheContext instance HashCode /// ///public abstract override int GetHashCode(); #endregion #region Internal API /// /// Cache entry hit count /// internal uint HitCount { get { return _hitCount; } set { _hitCount = value; } } ////// Gets/Sets Aging index for cache entry /// internal int AgingIndex { get { return _agingIndex; } set { _agingIndex = value; } } ////// Updates hit count /// internal void UpdateHit() { if (uint.MaxValue != _hitCount) { unchecked { _hitCount++; } } } ////// default string comparer /// /// /// ///protected virtual bool Equals( string s, string t ) { return String.Equals(s, t, _stringComparison); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
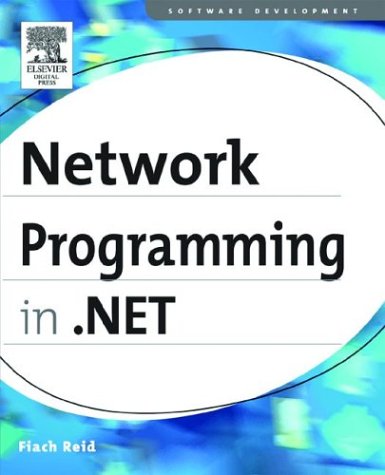
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GlyphRunDrawing.cs
- Base64Decoder.cs
- MemberRelationshipService.cs
- TypeConvertions.cs
- VScrollProperties.cs
- StickyNoteHelper.cs
- TypeInfo.cs
- HGlobalSafeHandle.cs
- BindingSource.cs
- AssemblyAssociatedContentFileAttribute.cs
- OleDbParameter.cs
- PropertyCollection.cs
- _HeaderInfo.cs
- SubpageParagraph.cs
- SapiRecoInterop.cs
- DataGridViewCellConverter.cs
- InvalidAsynchronousStateException.cs
- CodeMethodReturnStatement.cs
- ReferencedType.cs
- ControlAdapter.cs
- ValidationResult.cs
- ImageBrush.cs
- Form.cs
- UIAgentAsyncEndRequest.cs
- MLangCodePageEncoding.cs
- LicenseManager.cs
- BaseProcessor.cs
- SmtpNegotiateAuthenticationModule.cs
- Duration.cs
- DynamicMetaObjectBinder.cs
- CollectionsUtil.cs
- PassportAuthenticationModule.cs
- LingerOption.cs
- Single.cs
- XmlDataCollection.cs
- RequestCachePolicy.cs
- ConfigXmlText.cs
- LookupTables.cs
- XmlBindingWorker.cs
- DrawingDrawingContext.cs
- StrokeNodeEnumerator.cs
- FileFormatException.cs
- OleCmdHelper.cs
- Interlocked.cs
- StorageComplexPropertyMapping.cs
- XmlHierarchicalEnumerable.cs
- SystemDropShadowChrome.cs
- View.cs
- dataSvcMapFileLoader.cs
- SpotLight.cs
- DrawingVisualDrawingContext.cs
- ThicknessConverter.cs
- XamlBrushSerializer.cs
- WebPartEditVerb.cs
- wgx_commands.cs
- PathFigure.cs
- HashMembershipCondition.cs
- TimeSpan.cs
- DivideByZeroException.cs
- OpenTypeLayout.cs
- FormViewInsertedEventArgs.cs
- ClientSession.cs
- ListManagerBindingsCollection.cs
- DefaultValueTypeConverter.cs
- Event.cs
- Tile.cs
- QueryConverter.cs
- EasingFunctionBase.cs
- BuiltInExpr.cs
- PnrpPermission.cs
- CaseInsensitiveHashCodeProvider.cs
- SignedXml.cs
- ScriptingJsonSerializationSection.cs
- ConfigXmlAttribute.cs
- EndOfStreamException.cs
- EntityModelSchemaGenerator.cs
- CheckBoxField.cs
- MimeObjectFactory.cs
- Item.cs
- Deserializer.cs
- ProxyGenerationError.cs
- SymbolType.cs
- PropertyChangedEventArgs.cs
- RuleSet.cs
- DataTableClearEvent.cs
- BitmapEffectInput.cs
- RepeatInfo.cs
- TextTreeUndo.cs
- KeyNotFoundException.cs
- ApplicationBuildProvider.cs
- ExpandoClass.cs
- SettingsPropertyWrongTypeException.cs
- Vector3DKeyFrameCollection.cs
- TextSerializer.cs
- ListBoxAutomationPeer.cs
- SystemParameters.cs
- SpellCheck.cs
- FocusManager.cs
- SqlUtils.cs
- UntypedNullExpression.cs