Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / CompositeKey.cs / 2 / CompositeKey.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using KeyComponent = System.Collections.Generic.KeyValuePair; using System.Data.Common.Utils; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; namespace System.Data.Mapping.Update.Internal { /// /// Represents a key composed of multiple parts. /// internal class CompositeKey { #region Fields ////// Gets components of this composite key. /// internal readonly KeyComponent[] KeyComponents; #endregion #region Constructors ////// Initialize a new composite key using the given constant values. Order is important. /// /// Constants composing key internal CompositeKey(PropagatorResult[] constants) { Debug.Assert(null != constants, "key values must be given"); KeyComponents = new KeyComponent[constants.Length]; for (int i = 0; i < constants.Length; i++) { PropagatorResult constant = constants[i]; Debug.Assert(null != constant, "all key values must be set"); Int64 identifier = constant.Identifier; KeyComponents[i] = new KeyComponent(constant, identifier); } } #endregion #region Methods ////// Creates a key comparer operating in the context of the given translator. /// internal static IEqualityComparerCreateComparer(KeyManager keyManager) { return new CompositeKeyComparer(keyManager); } #endregion /// /// Equality and comparison implementation for composite keys. /// private class CompositeKeyComparer : IEqualityComparer{ private readonly KeyManager _manager; internal CompositeKeyComparer(KeyManager manager) { _manager = EntityUtil.CheckArgumentNull(manager, "manager"); } // determines equality by comparing each key component public bool Equals(CompositeKey left, CompositeKey right) { // Short circuit the comparison if we know the other reference is equivalent if (object.ReferenceEquals(left, right)) { return true; } // If either side is null, return false order (both can't be null because of // the previous check) if (null == left || null == right) { return false; } Debug.Assert(null != left.KeyComponents && null != right.KeyComponents, "(Update/JoinPropagator) CompositeKey must be initialized"); if (left.KeyComponents.Length != right.KeyComponents.Length) { return false; } for (int i = 0; i < left.KeyComponents.Length; i++) { object leftValue = GetValue(left.KeyComponents[i]); object rightValue = GetValue(right.KeyComponents[i]); if (!CdpEqualityComparer.DefaultEqualityComparer.Equals(leftValue, rightValue)) { return false; } } return true; } // creates a hash code by XORing hash codes for all key components. public int GetHashCode(CompositeKey key) { EntityUtil.CheckArgumentNull(key, "key"); int result = 0; foreach (KeyComponent keyComponent in key.KeyComponents) { result ^= GetValue(keyComponent).GetHashCode(); } return result; } // Gets the value to use for equality checks given a key component private object GetValue(KeyComponent keyComponent) { if (keyComponent.Value == PropagatorResult.NullIdentifier) { // no identifier exists for this key component, so use the actual key // value Debug.Assert(null != keyComponent.Key && null != keyComponent.Key, "key value must not be null"); return keyComponent.Key.GetSimpleValue(); } else { // use the identifier rather than the actual value (since uniqueness // is not guaranteed for client generated key values in the object layer) return _manager.GetCanonicalIdentifier(keyComponent.Value); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using KeyComponent = System.Collections.Generic.KeyValuePair; using System.Data.Common.Utils; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; namespace System.Data.Mapping.Update.Internal { /// /// Represents a key composed of multiple parts. /// internal class CompositeKey { #region Fields ////// Gets components of this composite key. /// internal readonly KeyComponent[] KeyComponents; #endregion #region Constructors ////// Initialize a new composite key using the given constant values. Order is important. /// /// Constants composing key internal CompositeKey(PropagatorResult[] constants) { Debug.Assert(null != constants, "key values must be given"); KeyComponents = new KeyComponent[constants.Length]; for (int i = 0; i < constants.Length; i++) { PropagatorResult constant = constants[i]; Debug.Assert(null != constant, "all key values must be set"); Int64 identifier = constant.Identifier; KeyComponents[i] = new KeyComponent(constant, identifier); } } #endregion #region Methods ////// Creates a key comparer operating in the context of the given translator. /// internal static IEqualityComparerCreateComparer(KeyManager keyManager) { return new CompositeKeyComparer(keyManager); } #endregion /// /// Equality and comparison implementation for composite keys. /// private class CompositeKeyComparer : IEqualityComparer{ private readonly KeyManager _manager; internal CompositeKeyComparer(KeyManager manager) { _manager = EntityUtil.CheckArgumentNull(manager, "manager"); } // determines equality by comparing each key component public bool Equals(CompositeKey left, CompositeKey right) { // Short circuit the comparison if we know the other reference is equivalent if (object.ReferenceEquals(left, right)) { return true; } // If either side is null, return false order (both can't be null because of // the previous check) if (null == left || null == right) { return false; } Debug.Assert(null != left.KeyComponents && null != right.KeyComponents, "(Update/JoinPropagator) CompositeKey must be initialized"); if (left.KeyComponents.Length != right.KeyComponents.Length) { return false; } for (int i = 0; i < left.KeyComponents.Length; i++) { object leftValue = GetValue(left.KeyComponents[i]); object rightValue = GetValue(right.KeyComponents[i]); if (!CdpEqualityComparer.DefaultEqualityComparer.Equals(leftValue, rightValue)) { return false; } } return true; } // creates a hash code by XORing hash codes for all key components. public int GetHashCode(CompositeKey key) { EntityUtil.CheckArgumentNull(key, "key"); int result = 0; foreach (KeyComponent keyComponent in key.KeyComponents) { result ^= GetValue(keyComponent).GetHashCode(); } return result; } // Gets the value to use for equality checks given a key component private object GetValue(KeyComponent keyComponent) { if (keyComponent.Value == PropagatorResult.NullIdentifier) { // no identifier exists for this key component, so use the actual key // value Debug.Assert(null != keyComponent.Key && null != keyComponent.Key, "key value must not be null"); return keyComponent.Key.GetSimpleValue(); } else { // use the identifier rather than the actual value (since uniqueness // is not guaranteed for client generated key values in the object layer) return _manager.GetCanonicalIdentifier(keyComponent.Value); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
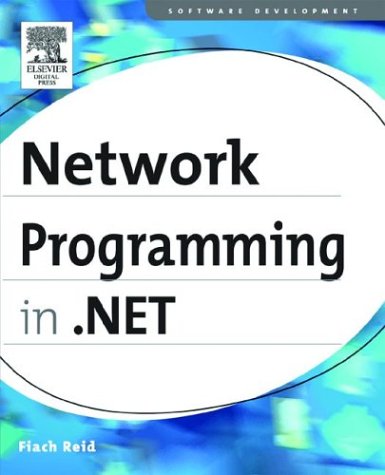
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SettingsBase.cs
- BuildProviderCollection.cs
- XhtmlStyleClass.cs
- _ListenerRequestStream.cs
- _NestedMultipleAsyncResult.cs
- RectAnimationUsingKeyFrames.cs
- ProcessModelInfo.cs
- RenderData.cs
- MultiTouchSystemGestureLogic.cs
- UnsafeNativeMethods.cs
- CompilerErrorCollection.cs
- TreeNodeStyleCollection.cs
- AnimatedTypeHelpers.cs
- ChtmlTextBoxAdapter.cs
- CellLabel.cs
- SettingsPropertyIsReadOnlyException.cs
- AsyncDataRequest.cs
- NameService.cs
- DynamicDataManager.cs
- DataGridRelationshipRow.cs
- _FtpControlStream.cs
- ExtenderProviderService.cs
- CharAnimationUsingKeyFrames.cs
- UpdatePanel.cs
- AutomationElementIdentifiers.cs
- RedirectionProxy.cs
- GroupPartitionExpr.cs
- DataGridCell.cs
- ScrollItemProviderWrapper.cs
- Metafile.cs
- DSACryptoServiceProvider.cs
- DocumentCollection.cs
- CacheChildrenQuery.cs
- XmlBinaryReader.cs
- util.cs
- GlobalizationSection.cs
- PrimitiveXmlSerializers.cs
- CodeVariableReferenceExpression.cs
- DataGridViewCellLinkedList.cs
- InkSerializer.cs
- DiagnosticsElement.cs
- LoadGrammarCompletedEventArgs.cs
- ModuleConfigurationInfo.cs
- XmlAnyElementAttributes.cs
- InputEventArgs.cs
- _NativeSSPI.cs
- XmlSchemaCollection.cs
- DataColumnPropertyDescriptor.cs
- WebHttpEndpoint.cs
- KnownAssemblyEntry.cs
- ListBase.cs
- DbXmlEnabledProviderManifest.cs
- ServiceAppDomainAssociationProvider.cs
- SHA1Cng.cs
- mediaclock.cs
- ProfilePropertyNameValidator.cs
- GlyphRunDrawing.cs
- AnimationTimeline.cs
- DayRenderEvent.cs
- DataFormats.cs
- Rfc2898DeriveBytes.cs
- DesignerHost.cs
- CurrentChangingEventManager.cs
- StructuredTypeEmitter.cs
- HwndSourceKeyboardInputSite.cs
- DomainUpDown.cs
- Error.cs
- DaylightTime.cs
- TextMessageEncodingBindingElement.cs
- PermissionAttributes.cs
- ErrorFormatter.cs
- LongMinMaxAggregationOperator.cs
- CapabilitiesAssignment.cs
- XmlAttributeCollection.cs
- dtdvalidator.cs
- IISMapPath.cs
- TypeUsageBuilder.cs
- SourceChangedEventArgs.cs
- LineVisual.cs
- BoundField.cs
- DataServiceQueryOfT.cs
- WindowVisualStateTracker.cs
- GlyphInfoList.cs
- DataGridItemCollection.cs
- _NestedSingleAsyncResult.cs
- ListControl.cs
- EventItfInfo.cs
- _SslState.cs
- AssemblyBuilderData.cs
- WinEventQueueItem.cs
- RadioButtonList.cs
- BitmapEffectDrawing.cs
- EventItfInfo.cs
- SafeEventLogReadHandle.cs
- OpenTypeLayoutCache.cs
- JavaScriptSerializer.cs
- Fx.cs
- StateInitialization.cs
- SQLBoolean.cs
- PointConverter.cs