Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / ExtractedStateEntry.cs / 1 / ExtractedStateEntry.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common.CommandTrees; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.Mapping.Update.Internal { ////// Represents the data contained in a StateEntry using internal data structures /// of the UpdatePipeline. /// internal struct ExtractedStateEntry { internal readonly EntityState State; internal readonly PropagatorResult Original; internal readonly PropagatorResult Current; internal readonly IEntityStateEntry Source; internal ExtractedStateEntry(UpdateTranslator translator, IEntityStateEntry stateEntry) { Debug.Assert(null != stateEntry, "stateEntry must not be null"); this.State = stateEntry.State; this.Source = stateEntry; switch (stateEntry.State) { case EntityState.Deleted: this.Original = translator.RecordConverter.ConvertOriginalValuesToPropagatorResult( stateEntry, null /* indicates all properties are modified */); this.Current = null; break; case EntityState.Unchanged: this.Original = translator.RecordConverter.ConvertOriginalValuesToPropagatorResult( stateEntry, Enumerable.Empty() /* indicates no properties are modified */); this.Current = translator.RecordConverter.ConvertCurrentValuesToPropagatorResult( stateEntry, Enumerable.Empty ()); break; case EntityState.Modified: this.Original = translator.RecordConverter.ConvertOriginalValuesToPropagatorResult( stateEntry, stateEntry.GetModifiedProperties()); this.Current = translator.RecordConverter.ConvertCurrentValuesToPropagatorResult( stateEntry, stateEntry.GetModifiedProperties()); break; case EntityState.Added: this.Original = null; this.Current = translator.RecordConverter.ConvertCurrentValuesToPropagatorResult( stateEntry, null /* indicates all properties are modified */); break; default: Debug.Fail("unexpected IEntityStateEntry.State for entity " + stateEntry.State); this.Original = null; this.Current = null; break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Common.CommandTrees; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.Mapping.Update.Internal { ////// Represents the data contained in a StateEntry using internal data structures /// of the UpdatePipeline. /// internal struct ExtractedStateEntry { internal readonly EntityState State; internal readonly PropagatorResult Original; internal readonly PropagatorResult Current; internal readonly IEntityStateEntry Source; internal ExtractedStateEntry(UpdateTranslator translator, IEntityStateEntry stateEntry) { Debug.Assert(null != stateEntry, "stateEntry must not be null"); this.State = stateEntry.State; this.Source = stateEntry; switch (stateEntry.State) { case EntityState.Deleted: this.Original = translator.RecordConverter.ConvertOriginalValuesToPropagatorResult( stateEntry, null /* indicates all properties are modified */); this.Current = null; break; case EntityState.Unchanged: this.Original = translator.RecordConverter.ConvertOriginalValuesToPropagatorResult( stateEntry, Enumerable.Empty() /* indicates no properties are modified */); this.Current = translator.RecordConverter.ConvertCurrentValuesToPropagatorResult( stateEntry, Enumerable.Empty ()); break; case EntityState.Modified: this.Original = translator.RecordConverter.ConvertOriginalValuesToPropagatorResult( stateEntry, stateEntry.GetModifiedProperties()); this.Current = translator.RecordConverter.ConvertCurrentValuesToPropagatorResult( stateEntry, stateEntry.GetModifiedProperties()); break; case EntityState.Added: this.Original = null; this.Current = translator.RecordConverter.ConvertCurrentValuesToPropagatorResult( stateEntry, null /* indicates all properties are modified */); break; default: Debug.Fail("unexpected IEntityStateEntry.State for entity " + stateEntry.State); this.Original = null; this.Current = null; break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
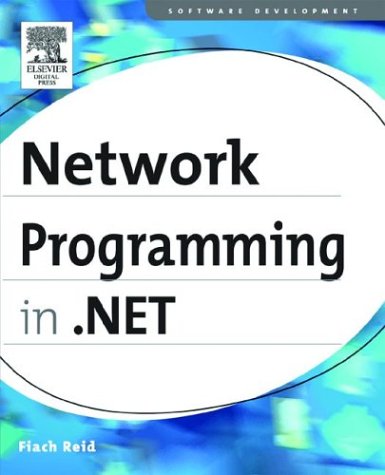
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataSourceWizardForm.cs
- ReferenceConverter.cs
- GcHandle.cs
- MouseGesture.cs
- BitmapEffectDrawingContextState.cs
- String.cs
- ItemChangedEventArgs.cs
- BoolExpr.cs
- ColumnReorderedEventArgs.cs
- VectorAnimation.cs
- AdCreatedEventArgs.cs
- ObjectPropertyMapping.cs
- TempFiles.cs
- InternalDuplexBindingElement.cs
- ArgumentNullException.cs
- DirectoryNotFoundException.cs
- ApplicationFileParser.cs
- ComEventsHelper.cs
- ConvertersCollection.cs
- Stack.cs
- PasswordRecovery.cs
- X509Certificate.cs
- BmpBitmapEncoder.cs
- DataGridParentRows.cs
- EventLogEntry.cs
- SchemaDeclBase.cs
- OLEDB_Enum.cs
- TextProperties.cs
- RootBuilder.cs
- RegexBoyerMoore.cs
- VerticalAlignConverter.cs
- FormatterConverter.cs
- ValidationHelpers.cs
- UserMapPath.cs
- Material.cs
- OleStrCAMarshaler.cs
- SoapMessage.cs
- TypeDescriptionProvider.cs
- PersistChildrenAttribute.cs
- LayoutTable.cs
- ObjectViewEntityCollectionData.cs
- KeyValueInternalCollection.cs
- ToolStripDropDownClosedEventArgs.cs
- RemoteWebConfigurationHostServer.cs
- XmlWellformedWriter.cs
- WebPartConnectVerb.cs
- ActivityCompletionCallbackWrapper.cs
- InfocardExtendedInformationCollection.cs
- AnimatedTypeHelpers.cs
- SynchronizationContextHelper.cs
- PeerToPeerException.cs
- UTF7Encoding.cs
- BitmapEffectrendercontext.cs
- StdValidatorsAndConverters.cs
- SerialErrors.cs
- IntPtr.cs
- SettingsProviderCollection.cs
- sqlinternaltransaction.cs
- EmptyQuery.cs
- Substitution.cs
- PageWrapper.cs
- PerfCounters.cs
- QueryInterceptorAttribute.cs
- _NestedSingleAsyncResult.cs
- TransformGroup.cs
- TemplateBaseAction.cs
- PageAdapter.cs
- LinqDataSourceUpdateEventArgs.cs
- DataBinder.cs
- NegationPusher.cs
- InputElement.cs
- ILGenerator.cs
- EllipseGeometry.cs
- HeaderedContentControl.cs
- TimeZoneNotFoundException.cs
- TagPrefixAttribute.cs
- ExtractorMetadata.cs
- DataGridViewCellLinkedList.cs
- SafeViewOfFileHandle.cs
- LeftCellWrapper.cs
- XamlToRtfParser.cs
- SwitchLevelAttribute.cs
- FormatConvertedBitmap.cs
- assemblycache.cs
- RenderOptions.cs
- EventLogPermissionAttribute.cs
- LogicalCallContext.cs
- CompilationRelaxations.cs
- ExpressionPrefixAttribute.cs
- DataListCommandEventArgs.cs
- QueryExpr.cs
- DataGridViewSelectedCellCollection.cs
- SystemEvents.cs
- XmlSchemaInclude.cs
- NamedPipeTransportManager.cs
- WSFederationHttpSecurityElement.cs
- CustomValidator.cs
- ReliableOutputConnection.cs
- RequiredFieldValidator.cs
- LoginUtil.cs