Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / DataObjectFieldAttribute.cs / 1 / DataObjectFieldAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.Security.Permissions; ////// Represents a field of a DataObject. Use this attribute on a field to indicate /// properties such as primary key, identity, nullability, and length. /// [AttributeUsage(AttributeTargets.Property)] public sealed class DataObjectFieldAttribute : Attribute { private bool _primaryKey; private bool _isIdentity; private bool _isNullable; private int _length; public DataObjectFieldAttribute(bool primaryKey) : this(primaryKey, false, false, -1) { } public DataObjectFieldAttribute(bool primaryKey, bool isIdentity) : this(primaryKey, isIdentity, false, -1) { } public DataObjectFieldAttribute(bool primaryKey, bool isIdentity, bool isNullable) : this(primaryKey, isIdentity, isNullable, -1){ } public DataObjectFieldAttribute(bool primaryKey, bool isIdentity, bool isNullable, int length) { _primaryKey = primaryKey; _isIdentity = isIdentity; _isNullable = isNullable; _length = length; } public bool IsIdentity { get { return _isIdentity; } } public bool IsNullable { get { return _isNullable; } } public int Length { get { return _length; } } public bool PrimaryKey { get { return _primaryKey; } } public override bool Equals(object obj) { if (obj == this) { return true; } DataObjectFieldAttribute other = obj as DataObjectFieldAttribute; return (other != null) && (other.IsIdentity == IsIdentity) && (other.IsNullable == IsNullable) && (other.Length == Length) && (other.PrimaryKey == PrimaryKey); } public override int GetHashCode() { return base.GetHashCode(); } } }
Link Menu
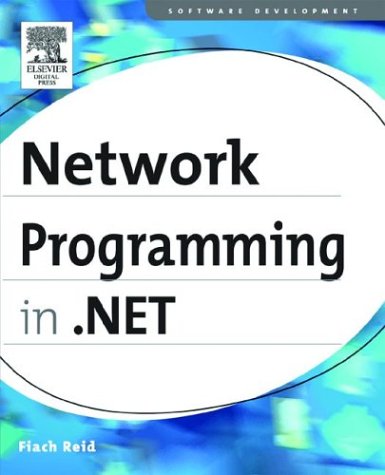
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextElementEditingBehaviorAttribute.cs
- KeyboardNavigation.cs
- SQLString.cs
- xmlfixedPageInfo.cs
- TableLayout.cs
- InertiaExpansionBehavior.cs
- ToolBarButton.cs
- ApplicationProxyInternal.cs
- DataAdapter.cs
- RectangleHotSpot.cs
- DocumentScope.cs
- SimpleHandlerFactory.cs
- DictionaryContent.cs
- SqlUDTStorage.cs
- SqlNodeTypeOperators.cs
- WebPart.cs
- ListParaClient.cs
- WsrmTraceRecord.cs
- InvalidOperationException.cs
- ToolStripPanelRow.cs
- RecognitionResult.cs
- xmlformatgeneratorstatics.cs
- HttpCacheParams.cs
- FakeModelPropertyImpl.cs
- ContextMenuStripActionList.cs
- SimpleMailWebEventProvider.cs
- OdbcUtils.cs
- WriterOutput.cs
- DbException.cs
- VectorCollection.cs
- AnonymousIdentificationModule.cs
- StringPropertyBuilder.cs
- CodeTypeReference.cs
- KerberosTicketHashIdentifierClause.cs
- GridViewSortEventArgs.cs
- CodePageEncoding.cs
- CalloutQueueItem.cs
- WorkBatch.cs
- MenuItemCollection.cs
- Source.cs
- ColumnMapCopier.cs
- PassportPrincipal.cs
- StateChangeEvent.cs
- NotifyParentPropertyAttribute.cs
- PauseStoryboard.cs
- OdbcErrorCollection.cs
- Walker.cs
- BuildResultCache.cs
- SqlDataAdapter.cs
- DataRowView.cs
- TableStyle.cs
- PageCatalogPartDesigner.cs
- InvokeGenerator.cs
- Speller.cs
- StreamUpdate.cs
- ViewValidator.cs
- BamlRecordReader.cs
- TableColumnCollection.cs
- SortedList.cs
- SqlResolver.cs
- SmiSettersStream.cs
- X509UI.cs
- DataRowCollection.cs
- TransformCollection.cs
- TypefaceMap.cs
- AnnotationHelper.cs
- ContainerParaClient.cs
- Label.cs
- ConnectionPoint.cs
- UrlEncodedParameterWriter.cs
- TextFindEngine.cs
- ComplexPropertyEntry.cs
- PageHandlerFactory.cs
- DrawingContextDrawingContextWalker.cs
- TableSectionStyle.cs
- HttpProcessUtility.cs
- HashCodeCombiner.cs
- PerfCounters.cs
- DataGridViewComboBoxColumn.cs
- HttpContext.cs
- MetadataItemEmitter.cs
- Marshal.cs
- Matrix.cs
- StringSource.cs
- AnimationClock.cs
- UnknownExceptionActionHelper.cs
- RotationValidation.cs
- SafeArrayTypeMismatchException.cs
- WindowsListBox.cs
- ContractsBCL.cs
- ExtendedProtectionPolicy.cs
- ConnectionPointCookie.cs
- MessageQueuePermission.cs
- WorkflowMessageEventHandler.cs
- GeneratedContractType.cs
- SendMessageRecord.cs
- FactoryGenerator.cs
- TemplateKeyConverter.cs
- Fonts.cs
- PropertyDescriptor.cs