Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / InertiaExpansionBehavior.cs / 1305600 / InertiaExpansionBehavior.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Input.Manipulations; namespace System.Windows.Input { ////// Provides information about the inertia behavior. /// public class InertiaExpansionBehavior { ////// Instantiates a new instance of this class. /// public InertiaExpansionBehavior() { } ////// Instantiates a new instance of this class. /// internal InertiaExpansionBehavior(Vector initialVelocity) { _initialVelocity = initialVelocity; } ////// The initial rate of change of size of the element at the start of the inertia phase. /// public Vector InitialVelocity { get { return _initialVelocity; } set { _isInitialVelocitySet = true; _initialVelocity = value; } } ////// The desired rate of change of velocity. /// public double DesiredDeceleration { get { return _desiredDeceleration; } set { if (Double.IsInfinity(value) || Double.IsNaN(value)) { throw new ArgumentOutOfRangeException("value"); } _isDesiredDecelerationSet = true; _desiredDeceleration = value; _isDesiredExpansionSet = false; _desiredExpansion = new Vector(double.NaN, double.NaN); } } ////// The desired total change in size. /// public Vector DesiredExpansion { get { return _desiredExpansion; } set { _isDesiredExpansionSet = true; _desiredExpansion = value; _isDesiredDecelerationSet = false; _desiredDeceleration = double.NaN; } } public double InitialRadius { get { return _initialRadius; } set { _isInitialRadiusSet = true; _initialRadius = value; } } internal bool CanUseForInertia() { return _isInitialVelocitySet || _isInitialRadiusSet || _isDesiredDecelerationSet || _isDesiredExpansionSet; } internal static void ApplyParameters(InertiaExpansionBehavior behavior, InertiaProcessor2D processor, Vector initialVelocity) { if (behavior != null && behavior.CanUseForInertia()) { InertiaExpansionBehavior2D behavior2D = new InertiaExpansionBehavior2D(); if (behavior._isInitialVelocitySet) { behavior2D.InitialVelocityX = (float)behavior._initialVelocity.X; behavior2D.InitialVelocityY = (float)behavior._initialVelocity.Y; } else { behavior2D.InitialVelocityX = (float)initialVelocity.X; behavior2D.InitialVelocityY = (float)initialVelocity.Y; } if (behavior._isDesiredDecelerationSet) { behavior2D.DesiredDeceleration = (float)behavior._desiredDeceleration; } if (behavior._isDesiredExpansionSet) { behavior2D.DesiredExpansionX = (float)behavior._desiredExpansion.X; behavior2D.DesiredExpansionY = (float)behavior._desiredExpansion.Y; } if (behavior._isInitialRadiusSet) { behavior2D.InitialRadius = (float)behavior._initialRadius; } processor.ExpansionBehavior = behavior2D; } } private bool _isInitialVelocitySet; private Vector _initialVelocity = new Vector(double.NaN, double.NaN); private bool _isDesiredDecelerationSet; private double _desiredDeceleration = double.NaN; private bool _isDesiredExpansionSet; private Vector _desiredExpansion = new Vector(double.NaN, double.NaN); private bool _isInitialRadiusSet; private double _initialRadius = 1.0; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
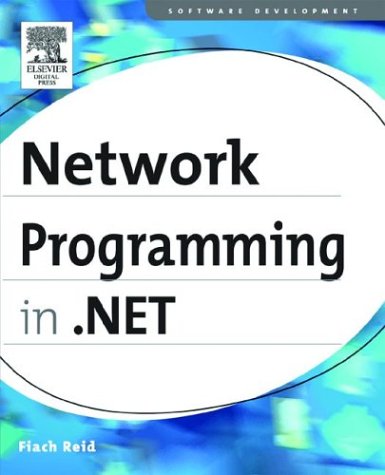
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DigitalSignatureProvider.cs
- Parallel.cs
- MethodBody.cs
- Table.cs
- ImpersonateTokenRef.cs
- DatePickerTextBox.cs
- SequenceDesignerAccessibleObject.cs
- XamlStream.cs
- JoinCqlBlock.cs
- XPathExpr.cs
- CuspData.cs
- BitmapMetadataBlob.cs
- ResourcesBuildProvider.cs
- JpegBitmapDecoder.cs
- EventHandlersStore.cs
- SafeBitVector32.cs
- EntityDataSourceDataSelection.cs
- SettingsAttributeDictionary.cs
- DataGridViewLinkCell.cs
- WebPartConnectionsConnectVerb.cs
- SafeSecurityHandles.cs
- SoapInteropTypes.cs
- DriveNotFoundException.cs
- SmiSettersStream.cs
- Matrix.cs
- ConnectionConsumerAttribute.cs
- DataSourceCollectionBase.cs
- GraphicsState.cs
- PackageRelationshipCollection.cs
- WebRequestModuleElement.cs
- ConditionalAttribute.cs
- ReceiveActivityValidator.cs
- SubordinateTransaction.cs
- ProgressiveCrcCalculatingStream.cs
- DictionaryGlobals.cs
- DataBoundControlAdapter.cs
- DetailsViewPagerRow.cs
- AccessDataSourceView.cs
- ResourceReferenceExpression.cs
- CircleHotSpot.cs
- WebPartTransformer.cs
- TextParaClient.cs
- DataSourceControl.cs
- HwndHostAutomationPeer.cs
- IdentifierService.cs
- TogglePattern.cs
- ProtocolsConfiguration.cs
- SettingsProperty.cs
- Transactions.cs
- CompositeCollection.cs
- CompoundFileReference.cs
- Sentence.cs
- FormViewRow.cs
- DataGridLinkButton.cs
- CommonXSendMessage.cs
- FieldToken.cs
- CssClassPropertyAttribute.cs
- HttpWebRequestElement.cs
- HtmlFormParameterWriter.cs
- StackSpiller.cs
- DbMetaDataColumnNames.cs
- HtmlElementCollection.cs
- EmbossBitmapEffect.cs
- iisPickupDirectory.cs
- Int16AnimationUsingKeyFrames.cs
- CryptoKeySecurity.cs
- HijriCalendar.cs
- ScrollPattern.cs
- TextStore.cs
- MsmqAppDomainProtocolHandler.cs
- DefaultValueAttribute.cs
- LicenseManager.cs
- DependencyProperty.cs
- AdditionalEntityFunctions.cs
- StyleXamlTreeBuilder.cs
- EncoderNLS.cs
- CheckBoxPopupAdapter.cs
- DateTimeUtil.cs
- PhonemeEventArgs.cs
- ScriptMethodAttribute.cs
- MD5.cs
- ToolTipAutomationPeer.cs
- CaseExpr.cs
- IOThreadScheduler.cs
- HttpClientCertificate.cs
- NumberEdit.cs
- PropertyInfoSet.cs
- AccessorTable.cs
- ClockGroup.cs
- UrlAuthFailedErrorFormatter.cs
- prompt.cs
- InplaceBitmapMetadataWriter.cs
- XmlValidatingReaderImpl.cs
- Scalars.cs
- XamlTypeMapper.cs
- EndpointAddressAugust2004.cs
- AssociationTypeEmitter.cs
- ExpandedWrapper.cs
- XmlSchemaSimpleContent.cs
- DefaultSection.cs