Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / StyleXamlTreeBuilder.cs / 1 / StyleXamlTreeBuilder.cs
/****************************************************************************\ * * File: StyleXamlTreeBuilder.cs * * Purpose: Class that builds a style object from XAML * * History: * 11/13/03: peterost Created * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; namespace System.Windows.Markup { ////// XamlTree builder is the TreeBuilder implementation that loads a Tree /// from XAML. /// internal class StyleXamlTreeBuilder : XamlTreeBuilder { #region Constructors ////// Constructor. Set up associated baml writer and xaml parser to /// create the triumvarate needed for parsing a style block. This is /// the main method to call to create the needed object graph for /// parsing. /// public StyleXamlTreeBuilder( ParserContext parserContext, XamlReaderHelper tokenReader, ReadWriteStreamManager streamManager, ParserStack bamlReaderStack, ArrayList rootList) { Debug.Assert(null != parserContext, "ParserContext is null"); Debug.Assert(null != tokenReader, "TokenReader is null"); Debug.Assert(null != parserContext.XamlTypeMapper, "ParserContext.XamlTypeMapper is null"); Debug.Assert(null != streamManager, "StreamManager is null"); XamlParseMode = tokenReader.XamlParseMode; // Fetch the previous BamlRecordReader XamlTreeBuilderBamlRecordWriter previousBamlRecordWriter = (XamlTreeBuilderBamlRecordWriter)tokenReader.ControllingXamlParser.BamlRecordWriter; BamlRecordReader previousBamlRecordReader = previousBamlRecordWriter.TreeBuilder.RecordReader; Parser = new StyleXamlParser(this, tokenReader, parserContext); Parser.StreamManager = streamManager; Parser.XamlParseMode = XamlParseMode; RecordWriter = new XamlTreeBuilderBamlRecordWriter(this, streamManager.WriterStream, parserContext, true /*isSerializer*/ ); // Give Writer to the Parser, since it can't be passed in constructor due to // circular dependency. Parser.BamlRecordWriter = RecordWriter; // The BamlRecordReader needs its own parser context ParserContext readerParserContext = previousBamlRecordReader.ParserContext; RecordReader = new StyleBamlRecordReader(streamManager.ReaderStream, null, null, readerParserContext, bamlReaderStack, rootList); } #endregion Constructors #region Overrides ////// Forward parsing directive onto xaml parser. /// ////// An array containing the root objects in the XAML stream /// public override object ParseFragment() { // Tell the parser the starting depth Parser.Parse(); // its okay for root to be null if its an empty file or the parse // was stopped. return GetRoot(); } #endregion Overrides ////// Forward the processing of a xaml node to the parser. This is done when there is /// an out-of-band record that needs to be added to the baml stream, such as the /// start of a Style tag. /// internal void ProcessXamlNode(XamlNode xamlNode) { bool cleanup = false; bool done = false; Parser.ProcessXamlNode(xamlNode, ref cleanup, ref done); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: StyleXamlTreeBuilder.cs * * Purpose: Class that builds a style object from XAML * * History: * 11/13/03: peterost Created * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; namespace System.Windows.Markup { ////// XamlTree builder is the TreeBuilder implementation that loads a Tree /// from XAML. /// internal class StyleXamlTreeBuilder : XamlTreeBuilder { #region Constructors ////// Constructor. Set up associated baml writer and xaml parser to /// create the triumvarate needed for parsing a style block. This is /// the main method to call to create the needed object graph for /// parsing. /// public StyleXamlTreeBuilder( ParserContext parserContext, XamlReaderHelper tokenReader, ReadWriteStreamManager streamManager, ParserStack bamlReaderStack, ArrayList rootList) { Debug.Assert(null != parserContext, "ParserContext is null"); Debug.Assert(null != tokenReader, "TokenReader is null"); Debug.Assert(null != parserContext.XamlTypeMapper, "ParserContext.XamlTypeMapper is null"); Debug.Assert(null != streamManager, "StreamManager is null"); XamlParseMode = tokenReader.XamlParseMode; // Fetch the previous BamlRecordReader XamlTreeBuilderBamlRecordWriter previousBamlRecordWriter = (XamlTreeBuilderBamlRecordWriter)tokenReader.ControllingXamlParser.BamlRecordWriter; BamlRecordReader previousBamlRecordReader = previousBamlRecordWriter.TreeBuilder.RecordReader; Parser = new StyleXamlParser(this, tokenReader, parserContext); Parser.StreamManager = streamManager; Parser.XamlParseMode = XamlParseMode; RecordWriter = new XamlTreeBuilderBamlRecordWriter(this, streamManager.WriterStream, parserContext, true /*isSerializer*/ ); // Give Writer to the Parser, since it can't be passed in constructor due to // circular dependency. Parser.BamlRecordWriter = RecordWriter; // The BamlRecordReader needs its own parser context ParserContext readerParserContext = previousBamlRecordReader.ParserContext; RecordReader = new StyleBamlRecordReader(streamManager.ReaderStream, null, null, readerParserContext, bamlReaderStack, rootList); } #endregion Constructors #region Overrides ////// Forward parsing directive onto xaml parser. /// ////// An array containing the root objects in the XAML stream /// public override object ParseFragment() { // Tell the parser the starting depth Parser.Parse(); // its okay for root to be null if its an empty file or the parse // was stopped. return GetRoot(); } #endregion Overrides ////// Forward the processing of a xaml node to the parser. This is done when there is /// an out-of-band record that needs to be added to the baml stream, such as the /// start of a Style tag. /// internal void ProcessXamlNode(XamlNode xamlNode) { bool cleanup = false; bool done = false; Parser.ProcessXamlNode(xamlNode, ref cleanup, ref done); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
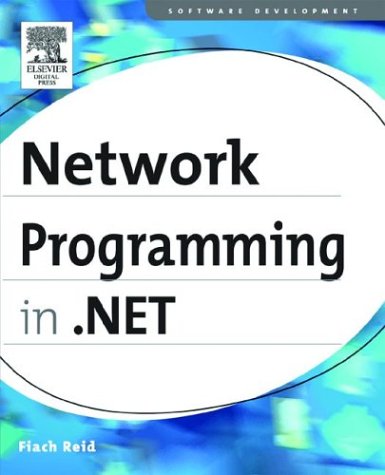
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultBinder.cs
- DesigntimeLicenseContextSerializer.cs
- SingleObjectCollection.cs
- TextPointer.cs
- ObjectReferenceStack.cs
- StylusEditingBehavior.cs
- ObsoleteAttribute.cs
- ImageListImage.cs
- TextHidden.cs
- DesignColumn.cs
- ProxyElement.cs
- OciLobLocator.cs
- FormatterServices.cs
- Double.cs
- DeferredTextReference.cs
- TypeConverterHelper.cs
- StrokeCollection.cs
- NamespaceImport.cs
- AudioDeviceOut.cs
- RulePatternOps.cs
- EventWaitHandleSecurity.cs
- ChannelServices.cs
- WindowsListView.cs
- MenuItem.cs
- HostExecutionContextManager.cs
- RequestCacheManager.cs
- SqlCachedBuffer.cs
- SqlClientMetaDataCollectionNames.cs
- DataAdapter.cs
- MultilineStringConverter.cs
- ListSortDescription.cs
- SettingsPropertyCollection.cs
- Hash.cs
- ApplicationId.cs
- SqlDataSourceQueryConverter.cs
- StaticFileHandler.cs
- SymLanguageVendor.cs
- Renderer.cs
- DefaultAuthorizationContext.cs
- PageThemeCodeDomTreeGenerator.cs
- WebControlsSection.cs
- ThreadAttributes.cs
- DiagnosticStrings.cs
- AvTraceDetails.cs
- WizardStepBase.cs
- HtmlSelect.cs
- HtmlMeta.cs
- Brush.cs
- HwndSubclass.cs
- GlyphCache.cs
- GridViewColumnHeader.cs
- HttpValueCollection.cs
- ISessionStateStore.cs
- XmlCollation.cs
- Visual3DCollection.cs
- DuplicateWaitObjectException.cs
- ISSmlParser.cs
- DetailsViewDeletedEventArgs.cs
- SingleAnimation.cs
- Stack.cs
- DbConnectionHelper.cs
- NamespaceQuery.cs
- DispatcherEventArgs.cs
- RewritingSimplifier.cs
- FormatVersion.cs
- ClassicBorderDecorator.cs
- IssuedTokenClientBehaviorsElementCollection.cs
- TreeViewItem.cs
- PersonalizationProvider.cs
- XmlSchemaCompilationSettings.cs
- ClosableStream.cs
- TextRenderer.cs
- SqlMethodAttribute.cs
- DefaultValueConverter.cs
- DependencyPropertyKey.cs
- ListViewHitTestInfo.cs
- Span.cs
- TraceProvider.cs
- PeerDuplexChannelListener.cs
- MachineSettingsSection.cs
- OdbcRowUpdatingEvent.cs
- Helpers.cs
- Transform3DGroup.cs
- RenderDataDrawingContext.cs
- XmlSerializerFactory.cs
- DataListItemCollection.cs
- XmlChildEnumerator.cs
- DropSource.cs
- Brush.cs
- SafeFileMappingHandle.cs
- BooleanExpr.cs
- XmlSchemaComplexType.cs
- ControlParameter.cs
- XmlDataSourceNodeDescriptor.cs
- TextAutomationPeer.cs
- SelfIssuedAuthRSACryptoProvider.cs
- __TransparentProxy.cs
- ClientSettingsStore.cs
- DocumentApplicationJournalEntry.cs
- WCFModelStrings.Designer.cs