Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / SelfIssuedAuthRSACryptoProvider.cs / 1 / SelfIssuedAuthRSACryptoProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // namespace Microsoft.InfoCards { using System; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.ServiceModel; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using System.Runtime.InteropServices; using System.Security.Cryptography; using System.IdentityModel; using System.Security.Cryptography.Xml; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Collections.ObjectModel; using System.Collections.Generic; // // Summary: // This class provides indirect access an RSACryptoServiceProvider - this allows us to // protect the private key better // internal class SelfIssuedAuthRSACryptoProvider : RSA { RSACryptoServiceProvider m_rsa; // // Summary: // Given a pointer to a CryptoHandle create a new instance of this class. // public SelfIssuedAuthRSACryptoProvider( RSACryptoServiceProvider rsa ) : base() { m_rsa = rsa; } public override String SignatureAlgorithm { get { return m_rsa.SignatureAlgorithm; } } public override String KeyExchangeAlgorithm { get { return m_rsa.KeyExchangeAlgorithm; } } public bool IsPublicOnly() { return m_rsa.PublicOnly; } public override byte[ ] EncryptValue( byte[ ] rgb ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } public override byte[ ] DecryptValue( byte[ ] rgb ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } public byte[ ] Decrypt( byte[ ] inData, bool fAOEP ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } public byte[ ] Encrypt( byte[ ] inData, bool fAOEP ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } public byte[ ] SignHash( byte[ ] hash, string hashAlgOid ) { IDT.ThrowInvalidArgumentConditional( null == hash || 0 == hash.Length, "hash" ); IDT.ThrowInvalidArgumentConditional( String.IsNullOrEmpty( hashAlgOid ), "hashAlgOid" ); return m_rsa.SignHash( hash, hashAlgOid ); } public bool VerifyHash( byte[ ] hash, string hashAlgOid, byte[ ] sig ) { IDT.ThrowInvalidArgumentConditional( null == hash || 0 == hash.Length, "hash" ); IDT.ThrowInvalidArgumentConditional( String.IsNullOrEmpty( hashAlgOid ), "hashAlgOid" ); IDT.ThrowInvalidArgumentConditional( null == sig || 0 == sig.Length, "sig" ); return m_rsa.VerifyHash( hash, hashAlgOid, sig ); } public override RSAParameters ExportParameters( bool includePrivateParameters ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } public override string ToXmlString( bool includePrivateParameters ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } public override void FromXmlString( string xmlString ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } public override void ImportParameters( System.Security.Cryptography.RSAParameters parameters ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } protected override void Dispose( bool disposing ) { if( null != m_rsa ) { ( ( IDisposable )m_rsa ).Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
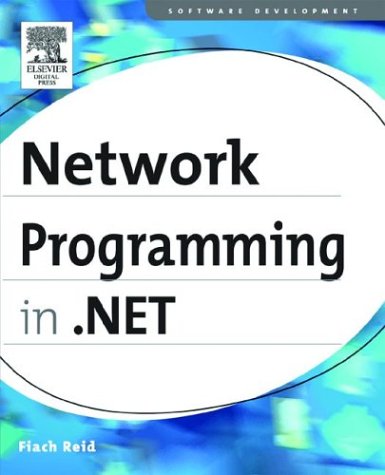
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringFunctions.cs
- DataGridItem.cs
- Group.cs
- XmlImplementation.cs
- MessageDecoder.cs
- SharedPersonalizationStateInfo.cs
- CapabilitiesSection.cs
- XmlDocumentViewSchema.cs
- DataRecordInternal.cs
- FocusTracker.cs
- TextBoxLine.cs
- PropertyEmitter.cs
- EmptyStringExpandableObjectConverter.cs
- GridViewColumnCollection.cs
- WindowsTitleBar.cs
- WebUtil.cs
- XmlMembersMapping.cs
- PrintSchema.cs
- Ops.cs
- ModelTreeEnumerator.cs
- ReadWriteSpinLock.cs
- ICollection.cs
- VirtualizingStackPanel.cs
- PointKeyFrameCollection.cs
- Token.cs
- DesignerActionVerbList.cs
- ThicknessKeyFrameCollection.cs
- OdbcTransaction.cs
- PnrpPermission.cs
- TypeNameHelper.cs
- Helpers.cs
- Int64.cs
- HelpProvider.cs
- ArrangedElementCollection.cs
- QualificationDataItem.cs
- URIFormatException.cs
- Quaternion.cs
- PenThreadWorker.cs
- LinkLabel.cs
- XmlSchemaAppInfo.cs
- ProviderMetadataCachedInformation.cs
- UpdatePanelTriggerCollection.cs
- LayoutTable.cs
- PixelShader.cs
- ListViewItem.cs
- XPathDocument.cs
- Brushes.cs
- OdbcEnvironment.cs
- XmlTextReaderImplHelpers.cs
- CustomAssemblyResolver.cs
- ObjectDataSourceFilteringEventArgs.cs
- HtmlAnchor.cs
- DropShadowBitmapEffect.cs
- GridViewRowPresenterBase.cs
- ListViewItemCollectionEditor.cs
- Unit.cs
- EventTask.cs
- SmtpReplyReader.cs
- LineMetrics.cs
- MenuAdapter.cs
- CodeArrayCreateExpression.cs
- ComponentResourceKeyConverter.cs
- SQLInt16.cs
- ContentPresenter.cs
- Metadata.cs
- ComNativeDescriptor.cs
- xmlfixedPageInfo.cs
- oledbmetadatacollectionnames.cs
- Compilation.cs
- VarInfo.cs
- OwnerDrawPropertyBag.cs
- SQLInt32Storage.cs
- PopupRoot.cs
- ControllableStoryboardAction.cs
- PageAsyncTaskManager.cs
- TransactionalPackage.cs
- CounterSetInstanceCounterDataSet.cs
- ComPlusAuthorization.cs
- SoundPlayer.cs
- RegexCode.cs
- HandleInitializationContext.cs
- HandlerBase.cs
- UpdatableWrapper.cs
- ConnectionManagementSection.cs
- PublisherMembershipCondition.cs
- RadioButtonPopupAdapter.cs
- ChannelServices.cs
- ZoomPercentageConverter.cs
- SafeNativeMethodsOther.cs
- MachineKeyConverter.cs
- EndpointDesigner.cs
- DescriptionAttribute.cs
- GlyphRun.cs
- ButtonBase.cs
- SymmetricAlgorithm.cs
- Process.cs
- IProducerConsumerCollection.cs
- BindingManagerDataErrorEventArgs.cs
- SettingsPropertyValue.cs
- OdbcEnvironment.cs