Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Text / LineMetrics.cs / 1305600 / LineMetrics.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: LineMetrics.cs // // Description: Cached metrics of a text line. // // History: // 04/25/2003 : [....] - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.Text { // --------------------------------------------------------------------- // Storage for metrics of a formatted line. // --------------------------------------------------------------------- internal struct LineMetrics { // ------------------------------------------------------------------ // Constructor. // // wrappingWidth - wrapping width for the line // length - number or characters in the line // width - width of the line // height - height of the line // baseline - baseline of the line // hasInlineObjects - has inline objects? // ----------------------------------------------------------------- internal LineMetrics( #if DEBUG double wrappingWidth, #endif int length, double width, double height, double baseline, bool hasInlineObjects, TextLineBreak textLineBreak) { #if DEBUG _wrappingWidth = wrappingWidth; #endif _start = 0; _width = width; _height = height; _baseline = baseline; _textLineBreak = textLineBreak; _packedData = ((uint) length & LengthMask) | (hasInlineObjects ? HasInlineObjectsMask : 0); } internal LineMetrics(LineMetrics source, double start, double width) { #if DEBUG _wrappingWidth = source.WrappingWidth; #endif _start = start; _width = width; _height = source.Height; _baseline = source.Baseline; _textLineBreak = source.TextLineBreak; _packedData = source._packedData | HasBeenUpdatedMask; } ////// Disposes linebreak object /// internal LineMetrics Dispose(bool returnUpdatedMetrics) { if(_textLineBreak != null) { _textLineBreak.Dispose(); if (returnUpdatedMetrics) { return new LineMetrics( #if DEBUG _wrappingWidth, #endif Length, _width, _height, _baseline, HasInlineObjects, null); } } return this; } #if DEBUG // ------------------------------------------------------------------ // Wrapping width for the line. // ------------------------------------------------------------------ internal double WrappingWidth { get { return _wrappingWidth; } } private double _wrappingWidth; #endif // ----------------------------------------------------------------- // Number or characters in the line. // ------------------------------------------------------------------ internal int Length { get { return (int) (_packedData & LengthMask); } } private uint _packedData; // ----------------------------------------------------------------- // Width of the line. // ----------------------------------------------------------------- internal double Width { get { Debug.Assert((_packedData & HasBeenUpdatedMask) != 0); return _width; } } private double _width; // ----------------------------------------------------------------- // Height of the line. // ------------------------------------------------------------------ internal double Height { get { return _height; } } private double _height; // ----------------------------------------------------------------- // Start of the line. Distance from paragraph edge to line start. // ------------------------------------------------------------------ internal double Start { get { Debug.Assert((_packedData & HasBeenUpdatedMask) != 0); return _start; } } private double _start; // ------------------------------------------------------------------ // Baseline offset of the line. // ----------------------------------------------------------------- internal double Baseline { get { return _baseline; } } private double _baseline; // ------------------------------------------------------------------ // Has inline objects? // ----------------------------------------------------------------- internal bool HasInlineObjects { get { return (_packedData & HasInlineObjectsMask) != 0; } } // ----------------------------------------------------------------- // Line break for formatting. (Line Break In) // ----------------------------------------------------------------- internal TextLineBreak TextLineBreak { get { return _textLineBreak; } } private TextLineBreak _textLineBreak; private static readonly uint HasBeenUpdatedMask = 0x40000000; private static readonly uint LengthMask = 0x3FFFFFFF; private static readonly uint HasInlineObjectsMask = 0x80000000; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
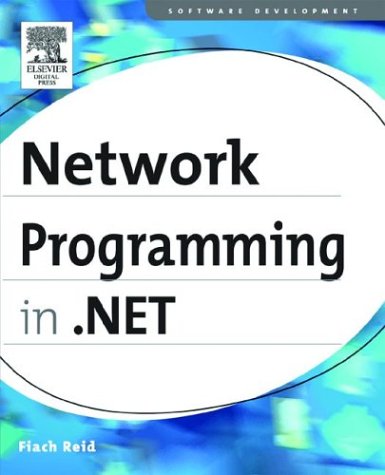
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _ServiceNameStore.cs
- DataViewListener.cs
- AuthenticationService.cs
- MetadataUtil.cs
- DES.cs
- TypeLoadException.cs
- XmlSchemaSequence.cs
- TrackingRecord.cs
- TextDocumentView.cs
- SizeFConverter.cs
- UnsafeNativeMethods.cs
- BindingExpressionBase.cs
- BitVector32.cs
- VisualStyleRenderer.cs
- ReferencedCollectionType.cs
- RoutedEventArgs.cs
- SystemIPGlobalProperties.cs
- InternalsVisibleToAttribute.cs
- NameNode.cs
- InputProviderSite.cs
- SrgsRule.cs
- Roles.cs
- WindowsToolbarItemAsMenuItem.cs
- DataSvcMapFileSerializer.cs
- XmlHierarchicalDataSourceView.cs
- Viewport2DVisual3D.cs
- XPathScanner.cs
- EdmSchemaAttribute.cs
- XhtmlBasicFormAdapter.cs
- RawStylusInputCustomData.cs
- xdrvalidator.cs
- QueryInterceptorAttribute.cs
- SponsorHelper.cs
- SqlUnionizer.cs
- TextPattern.cs
- RankException.cs
- Exception.cs
- SecurityElement.cs
- LabelLiteral.cs
- ComponentEvent.cs
- OutputCacheSettingsSection.cs
- WriteFileContext.cs
- AttachedPropertyBrowsableAttribute.cs
- JoinQueryOperator.cs
- UxThemeWrapper.cs
- Resources.Designer.cs
- ButtonBase.cs
- ElementNotEnabledException.cs
- HttpHeaderCollection.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- DataBoundControlHelper.cs
- HttpCacheVary.cs
- ConfigurationValidatorAttribute.cs
- CodeExpressionStatement.cs
- DynamicVirtualDiscoSearcher.cs
- SelectionChangedEventArgs.cs
- ThemeDictionaryExtension.cs
- HashAlgorithm.cs
- HostProtectionPermission.cs
- DbProviderSpecificTypePropertyAttribute.cs
- CustomGrammar.cs
- MenuItemStyle.cs
- String.cs
- TargetControlTypeAttribute.cs
- AdapterUtil.cs
- RectangleHotSpot.cs
- ToolStripScrollButton.cs
- StylusLogic.cs
- CodeMemberProperty.cs
- ColumnTypeConverter.cs
- XmlSchemaElement.cs
- TextUtf8RawTextWriter.cs
- WaitHandleCannotBeOpenedException.cs
- Pair.cs
- WebPartHelpVerb.cs
- CompositionAdorner.cs
- GPRECT.cs
- BitmapScalingModeValidation.cs
- CompositeTypefaceMetrics.cs
- ReferencedAssembly.cs
- UdpTransportSettingsElement.cs
- StorageEntityTypeMapping.cs
- HTTPNotFoundHandler.cs
- TimeZone.cs
- OdbcErrorCollection.cs
- StringConcat.cs
- DBNull.cs
- Socket.cs
- XmlCDATASection.cs
- SystemWebSectionGroup.cs
- DaylightTime.cs
- WebPartActionVerb.cs
- ControlEvent.cs
- RijndaelCryptoServiceProvider.cs
- WebServiceMethodData.cs
- TextServicesDisplayAttribute.cs
- DataGridColumnDropSeparator.cs
- SqlReferenceCollection.cs
- EnlistmentTraceIdentifier.cs
- BaseServiceProvider.cs