Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Binary / JoinQueryOperator.cs / 1305376 / JoinQueryOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // JoinQueryOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A join operator takes a left query tree and a right query tree, and then yields the /// matching pairs between the two. LINQ supports equi-key-based joins. Hence, a key- /// selection function for the left and right data types will yield keys of the same /// type for both. We then merely have to match elements from the left with elements from /// the right that have the same exact key. Note that this is an inner join. In other /// words, outer elements with no matching inner elements do not appear in the output. /// /// @ internal sealed class JoinQueryOperator : BinaryQueryOperator { private readonly Func m_leftKeySelector; // The key selection routine for the outer (left) data source. private readonly Func m_rightKeySelector; // The key selection routine for the inner (right) data source. private readonly Func m_resultSelector; // The result selection routine. private readonly IEqualityComparer m_keyComparer; // An optional key comparison object. //---------------------------------------------------------------------------------------- // Constructs a new join operator. // internal JoinQueryOperator(ParallelQuery left, ParallelQuery right, Func leftKeySelector, Func rightKeySelector, Func resultSelector, IEqualityComparer keyComparer) :base(left, right) { Contract.Assert(left != null && right != null, "child data sources cannot be null"); Contract.Assert(leftKeySelector != null, "left key selector must not be null"); Contract.Assert(rightKeySelector != null, "right key selector must not be null"); Contract.Assert(resultSelector != null, "need a result selector function"); m_leftKeySelector = leftKeySelector; m_rightKeySelector = rightKeySelector; m_resultSelector = resultSelector; m_keyComparer = keyComparer; m_outputOrdered = LeftChild.OutputOrdered; SetOrdinalIndex(OrdinalIndexState.Shuffled); } public override void WrapPartitionedStream ( PartitionedStream leftStream, PartitionedStream rightStream, IPartitionedStreamRecipient outputRecipient, bool preferStriping, QuerySettings settings) { Contract.Assert(rightStream.PartitionCount == leftStream.PartitionCount); if (LeftChild.OutputOrdered) { WrapPartitionedStreamHelper ( ExchangeUtilities.HashRepartitionOrdered(leftStream, m_leftKeySelector, m_keyComparer, null, settings.CancellationState.MergedCancellationToken), rightStream, outputRecipient, settings.CancellationState.MergedCancellationToken); } else { WrapPartitionedStreamHelper ( ExchangeUtilities.HashRepartition(leftStream, m_leftKeySelector, m_keyComparer, null, settings.CancellationState.MergedCancellationToken), rightStream, outputRecipient, settings.CancellationState.MergedCancellationToken); } } //--------------------------------------------------------------------------------------- // This is a helper method. WrapPartitionedStream decides what type TLeftKey is going // to be, and then call this method with that key as a generic parameter. // private void WrapPartitionedStreamHelper ( PartitionedStream , TLeftKey> leftHashStream, PartitionedStream rightPartitionedStream, IPartitionedStreamRecipient outputRecipient, CancellationToken cancellationToken) { int partitionCount = leftHashStream.PartitionCount; PartitionedStream , int> rightHashStream = ExchangeUtilities.HashRepartition( rightPartitionedStream, m_rightKeySelector, m_keyComparer, null, cancellationToken); PartitionedStream outputStream = new PartitionedStream ( partitionCount, leftHashStream.KeyComparer, OrdinalIndexState); for (int i = 0; i < partitionCount; i++) { outputStream[i] = new HashJoinQueryOperatorEnumerator ( leftHashStream[i], rightHashStream[i], m_resultSelector, null, m_keyComparer, cancellationToken); } outputRecipient.Receive(outputStream); } internal override QueryResults Open(QuerySettings settings, bool preferStriping) { QueryResults leftResults = LeftChild.Open(settings, false); QueryResults rightResults = RightChild.Open(settings, false); return new BinaryQueryOperatorResults(leftResults, rightResults, this, settings, false); } //--------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { IEnumerable wrappedLeftChild = CancellableEnumerable.Wrap(LeftChild.AsSequentialQuery(token), token); IEnumerable wrappedRightChild = CancellableEnumerable.Wrap(RightChild.AsSequentialQuery(token), token); return wrappedLeftChild.Join( wrappedRightChild, m_leftKeySelector, m_rightKeySelector, m_resultSelector, m_keyComparer); } //--------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // JoinQueryOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A join operator takes a left query tree and a right query tree, and then yields the /// matching pairs between the two. LINQ supports equi-key-based joins. Hence, a key- /// selection function for the left and right data types will yield keys of the same /// type for both. We then merely have to match elements from the left with elements from /// the right that have the same exact key. Note that this is an inner join. In other /// words, outer elements with no matching inner elements do not appear in the output. /// /// @ internal sealed class JoinQueryOperator : BinaryQueryOperator { private readonly Func m_leftKeySelector; // The key selection routine for the outer (left) data source. private readonly Func m_rightKeySelector; // The key selection routine for the inner (right) data source. private readonly Func m_resultSelector; // The result selection routine. private readonly IEqualityComparer m_keyComparer; // An optional key comparison object. //---------------------------------------------------------------------------------------- // Constructs a new join operator. // internal JoinQueryOperator(ParallelQuery left, ParallelQuery right, Func leftKeySelector, Func rightKeySelector, Func resultSelector, IEqualityComparer keyComparer) :base(left, right) { Contract.Assert(left != null && right != null, "child data sources cannot be null"); Contract.Assert(leftKeySelector != null, "left key selector must not be null"); Contract.Assert(rightKeySelector != null, "right key selector must not be null"); Contract.Assert(resultSelector != null, "need a result selector function"); m_leftKeySelector = leftKeySelector; m_rightKeySelector = rightKeySelector; m_resultSelector = resultSelector; m_keyComparer = keyComparer; m_outputOrdered = LeftChild.OutputOrdered; SetOrdinalIndex(OrdinalIndexState.Shuffled); } public override void WrapPartitionedStream ( PartitionedStream leftStream, PartitionedStream rightStream, IPartitionedStreamRecipient outputRecipient, bool preferStriping, QuerySettings settings) { Contract.Assert(rightStream.PartitionCount == leftStream.PartitionCount); if (LeftChild.OutputOrdered) { WrapPartitionedStreamHelper ( ExchangeUtilities.HashRepartitionOrdered(leftStream, m_leftKeySelector, m_keyComparer, null, settings.CancellationState.MergedCancellationToken), rightStream, outputRecipient, settings.CancellationState.MergedCancellationToken); } else { WrapPartitionedStreamHelper ( ExchangeUtilities.HashRepartition(leftStream, m_leftKeySelector, m_keyComparer, null, settings.CancellationState.MergedCancellationToken), rightStream, outputRecipient, settings.CancellationState.MergedCancellationToken); } } //--------------------------------------------------------------------------------------- // This is a helper method. WrapPartitionedStream decides what type TLeftKey is going // to be, and then call this method with that key as a generic parameter. // private void WrapPartitionedStreamHelper ( PartitionedStream , TLeftKey> leftHashStream, PartitionedStream rightPartitionedStream, IPartitionedStreamRecipient outputRecipient, CancellationToken cancellationToken) { int partitionCount = leftHashStream.PartitionCount; PartitionedStream , int> rightHashStream = ExchangeUtilities.HashRepartition( rightPartitionedStream, m_rightKeySelector, m_keyComparer, null, cancellationToken); PartitionedStream outputStream = new PartitionedStream ( partitionCount, leftHashStream.KeyComparer, OrdinalIndexState); for (int i = 0; i < partitionCount; i++) { outputStream[i] = new HashJoinQueryOperatorEnumerator ( leftHashStream[i], rightHashStream[i], m_resultSelector, null, m_keyComparer, cancellationToken); } outputRecipient.Receive(outputStream); } internal override QueryResults Open(QuerySettings settings, bool preferStriping) { QueryResults leftResults = LeftChild.Open(settings, false); QueryResults rightResults = RightChild.Open(settings, false); return new BinaryQueryOperatorResults(leftResults, rightResults, this, settings, false); } //--------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { IEnumerable wrappedLeftChild = CancellableEnumerable.Wrap(LeftChild.AsSequentialQuery(token), token); IEnumerable wrappedRightChild = CancellableEnumerable.Wrap(RightChild.AsSequentialQuery(token), token); return wrappedLeftChild.Join( wrappedRightChild, m_leftKeySelector, m_rightKeySelector, m_resultSelector, m_keyComparer); } //--------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
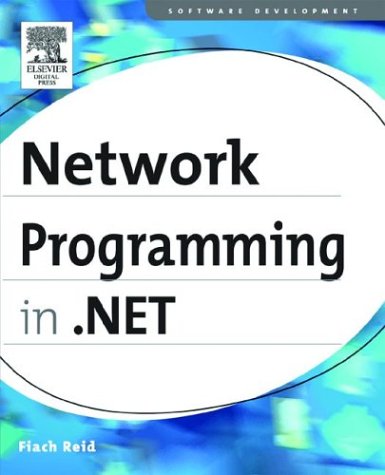
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegistrationServices.cs
- TextComposition.cs
- AsymmetricSignatureFormatter.cs
- RichTextBox.cs
- complextypematerializer.cs
- PageAsyncTaskManager.cs
- OperationInvokerTrace.cs
- DictationGrammar.cs
- ObjectPropertyMapping.cs
- _LocalDataStoreMgr.cs
- ListViewItemEventArgs.cs
- CriticalHandle.cs
- ButtonPopupAdapter.cs
- BrowserTree.cs
- HttpAsyncResult.cs
- SeekableReadStream.cs
- TreeIterators.cs
- SystemColors.cs
- AllMembershipCondition.cs
- AnnotationResource.cs
- DataRecordInternal.cs
- MethodAccessException.cs
- BaseTemplateParser.cs
- SessionParameter.cs
- DesignerDataSourceView.cs
- XPathDocumentIterator.cs
- TextServicesProperty.cs
- UnmanagedMemoryStream.cs
- DesignTimeData.cs
- XNodeSchemaApplier.cs
- PointLightBase.cs
- SafeArrayRankMismatchException.cs
- AutomationAttributeInfo.cs
- EventSchemaTraceListener.cs
- OleDbRowUpdatedEvent.cs
- DrawingAttributeSerializer.cs
- PassportAuthentication.cs
- BitmapSourceSafeMILHandle.cs
- FtpWebRequest.cs
- Drawing.cs
- WeakRefEnumerator.cs
- ResourceContainer.cs
- ProxyWebPartManager.cs
- SessionMode.cs
- CaseStatement.cs
- WebPart.cs
- DSASignatureFormatter.cs
- SqlDataSourceAdvancedOptionsForm.cs
- SqlExpander.cs
- SqlDataSourceCustomCommandEditor.cs
- RootDesignerSerializerAttribute.cs
- ContainerParaClient.cs
- HtmlInputRadioButton.cs
- RadioButton.cs
- Internal.cs
- CreateUserErrorEventArgs.cs
- TargetControlTypeCache.cs
- Exceptions.cs
- Script.cs
- PixelShader.cs
- LinkLabel.cs
- PageAsyncTask.cs
- DbConnectionPoolGroupProviderInfo.cs
- DrawingContextDrawingContextWalker.cs
- DispatcherOperation.cs
- InkSerializer.cs
- JumpTask.cs
- SqlOuterApplyReducer.cs
- HttpPostedFile.cs
- ElementsClipboardData.cs
- ClientFormsAuthenticationMembershipProvider.cs
- FileChangeNotifier.cs
- WebServiceMethodData.cs
- DocumentOutline.cs
- ResourceReferenceKeyNotFoundException.cs
- ThumbButtonInfo.cs
- IndicFontClient.cs
- TraceRecords.cs
- SafeThemeHandle.cs
- BuildResult.cs
- NumericUpDownAcceleration.cs
- ByteStorage.cs
- DirectoryGroupQuery.cs
- CreateRefExpr.cs
- DateTimeConstantAttribute.cs
- XPathNavigatorReader.cs
- ConnectionInterfaceCollection.cs
- CompoundFileDeflateTransform.cs
- InputScopeAttribute.cs
- WebPartExportVerb.cs
- QueryNode.cs
- ClientOptions.cs
- NetDataContractSerializer.cs
- CreateUserErrorEventArgs.cs
- DataError.cs
- EFAssociationProvider.cs
- DataGridViewImageCell.cs
- SqlCacheDependency.cs
- ActivationServices.cs
- CodeExpressionRuleDeclaration.cs