Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / documents / Application / DocumentApplicationJournalEntry.cs / 1 / DocumentApplicationJournalEntry.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Attached to custom journal entries created for changes in the DocumentApplication's // UI state. // // History: // 06/24/2005 - [....] created // //--------------------------------------------------------------------------- using System; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Navigation; using System.Windows.Controls; namespace MS.Internal.Documents.Application { ////// DocumentApplicationJournalEntry is not a real journal entry, just the CustomContentState /// attached to one. It wraps a DocumentApplicationState object, which is the actual view state. /// The split is needed because PresentationUI cannot access internal Framework classes and methods. /// [Serializable] internal sealed class DocumentApplicationJournalEntry : System.Windows.Navigation.CustomContentState { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Constructs a DocumentApplicationJournalEntry /// /// State of the DocumentApplication to journal /// Name of the journal entry to display in the UI. /// If this is null it will default to the URI source. public DocumentApplicationJournalEntry(object state, string name) { Invariant.Assert(state is DocumentApplicationState, "state should be of type DocumentApplicationState"); // Store parameters locally. _state = state; _displayName = name; } public DocumentApplicationJournalEntry(object state): this(state, null) { } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Used to reset the UI to state of this entry /// /// NavigationService currently running /// Navigation direction ////// Critical: set_StoredDocumentApplicationState is defined in a non-APTCA assembly. /// TreatAsSafe: call to set_StoredDocumentApplicationState does not entail any risk. /// [SecurityCritical, SecurityTreatAsSafe] public override void Replay(NavigationService navigationService, NavigationMode mode) { ContentControl navigator = (ContentControl)navigationService.INavigatorHost; // Find a reference to the DocumentViewer hosted in the NavigationWindow // On initial history navigation in the browser, the window's layout may not have been // done yet. ApplyTemplate() causes the viewer to be created. navigator.ApplyTemplate(); DocumentApplicationDocumentViewer docViewer = navigator.Template.FindName( "PUIDocumentApplicationDocumentViewer", navigator) as DocumentApplicationDocumentViewer; Debug.Assert(docViewer != null, "PUIDocumentApplicationDocumentViewer not found."); if (docViewer != null) { // Set the new state on the DocumentViewer if (_state is DocumentApplicationState) { docViewer.StoredDocumentApplicationState = (DocumentApplicationState)_state; } // Check that a Document exists. if (navigationService.Content != null) { IDocumentPaginatorSource document = navigationService.Content as IDocumentPaginatorSource; // If the document has already been paginated (could happen in the // case of a fragment navigation), then set the DocumentViewer to the // new state that was set. if ((document != null) && (document.DocumentPaginator.IsPageCountValid)) { docViewer.SetUIToStoredState(); } } } } public override string JournalEntryName { get { return _displayName; } } //------------------------------------------------------ // // Private Fields. // //------------------------------------------------------ // The DocumentApplicationState has been weakly-typed to avoid PresentationFramework // having a type dependency on PresentationUI. The perf impact of the weak // typed variables in this case was determined to be much less than forcing the load // of a new assembly when Assembly.GetTypes was called on PresentationFramework. private object _state; // DocumentApplicationState private string _displayName; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
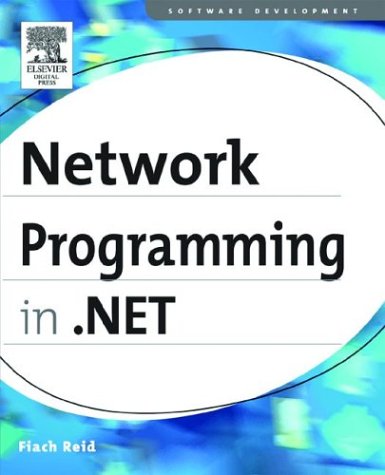
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyMetadata.cs
- UIElement3DAutomationPeer.cs
- NativeMethods.cs
- ZeroOpNode.cs
- GeometryModel3D.cs
- DateTimeStorage.cs
- ClientOptions.cs
- CreateUserWizardStep.cs
- IssuedTokenClientBehaviorsElementCollection.cs
- XmlTextWriter.cs
- ProfileModule.cs
- GorillaCodec.cs
- SqlWriter.cs
- OleDbTransaction.cs
- TrackingLocation.cs
- NetCodeGroup.cs
- BatchServiceHost.cs
- WindowsFont.cs
- ValueType.cs
- SelectionWordBreaker.cs
- MeasureItemEvent.cs
- FileSystemEventArgs.cs
- ReadOnlyHierarchicalDataSource.cs
- ApplicationContext.cs
- EasingQuaternionKeyFrame.cs
- Model3DGroup.cs
- objectresult_tresulttype.cs
- Line.cs
- TreeNodeEventArgs.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- SchemaManager.cs
- DrawingBrush.cs
- SHA1CryptoServiceProvider.cs
- DecimalAnimationBase.cs
- XamlFigureLengthSerializer.cs
- DispatcherHookEventArgs.cs
- CallContext.cs
- GcHandle.cs
- StrokeSerializer.cs
- ContextMarshalException.cs
- FontCacheUtil.cs
- SettingsPropertyNotFoundException.cs
- Converter.cs
- AutomationAttributeInfo.cs
- SyndicationItemFormatter.cs
- CompareInfo.cs
- CopyAction.cs
- HttpRuntime.cs
- SetStoryboardSpeedRatio.cs
- BinarySerializer.cs
- Drawing.cs
- TextMessageEncodingBindingElement.cs
- EntityException.cs
- PageFunction.cs
- WebPartZoneCollection.cs
- PropertyTabChangedEvent.cs
- RelationshipFixer.cs
- LowerCaseStringConverter.cs
- XomlCompilerParameters.cs
- XmlSchemaExporter.cs
- NameSpaceEvent.cs
- MailWriter.cs
- TextBox.cs
- ClientRolePrincipal.cs
- OrderedEnumerableRowCollection.cs
- BaseHashHelper.cs
- CodeTypeReference.cs
- autovalidator.cs
- TypeNameConverter.cs
- ImmutableObjectAttribute.cs
- HitTestDrawingContextWalker.cs
- HeaderCollection.cs
- SafeCryptoHandles.cs
- FreezableDefaultValueFactory.cs
- PathSegment.cs
- Misc.cs
- FaultException.cs
- StreamingContext.cs
- Match.cs
- AutoSizeToolBoxItem.cs
- InfoCardCryptoHelper.cs
- DetailsViewInsertedEventArgs.cs
- WebMessageEncodingBindingElement.cs
- HttpServerVarsCollection.cs
- DependencyObjectProvider.cs
- ProfilePropertyNameValidator.cs
- ListViewItem.cs
- Style.cs
- wmiprovider.cs
- DataObject.cs
- TextSearch.cs
- dbdatarecord.cs
- ControlCollection.cs
- _ChunkParse.cs
- OpCopier.cs
- EncodingTable.cs
- ControlPaint.cs
- SqlRemoveConstantOrderBy.cs
- NameNode.cs
- ConnectionPointCookie.cs