Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Animation / KeyTime.cs / 1 / KeyTime.cs
// KeyTime.cs // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// A KeyTime is use to specify when relative to the time of an animation /// that a KeyFrame takes place. /// [TypeConverter(typeof(KeyTimeConverter))] public struct KeyTime : IEquatable{ private object _value; private KeyTimeType _type; #region Static Create Methods /// /// Creates a KeyTime that represents a Percent value. /// /// /// The percent value provided as a double value between 0.0 and 1.0. /// public static KeyTime FromPercent(double percent) { if (percent < 0.0 || percent > 1.0) { throw new ArgumentOutOfRangeException("percent", SR.Get(SRID.Animation_KeyTime_InvalidPercentValue, percent)); } KeyTime keyTime = new KeyTime(); keyTime._value = percent; keyTime._type = KeyTimeType.Percent; return keyTime; } ////// /// /// public static KeyTime FromTimeSpan(TimeSpan timeSpan) { if (timeSpan < TimeSpan.Zero) { throw new ArgumentOutOfRangeException("timeSpan", SR.Get(SRID.Animation_KeyTime_LessThanZero, timeSpan)); } KeyTime keyTime = new KeyTime(); keyTime._value = timeSpan; keyTime._type = KeyTimeType.TimeSpan; return keyTime; } ////// /// ///public static KeyTime Uniform { get { KeyTime keyTime = new KeyTime(); keyTime._type = KeyTimeType.Uniform; return keyTime; } } /// /// /// ///public static KeyTime Paced { get { KeyTime keyTime = new KeyTime(); keyTime._type = KeyTimeType.Paced; return keyTime; } } #endregion #region Operators /// /// Returns true if two KeyTimes are equal. /// public static bool Equals(KeyTime keyTime1, KeyTime keyTime2) { if (keyTime1._type == keyTime2._type) { switch (keyTime1._type) { case KeyTimeType.Uniform: break; case KeyTimeType.Paced: break; case KeyTimeType.Percent: if ((double)keyTime1._value != (double)keyTime2._value) { return false; } break; case KeyTimeType.TimeSpan: if ((TimeSpan)keyTime1._value != (TimeSpan)keyTime2._value) { return false; } break; } return true; } else { return false; } } ////// Returns true if two KeyTimes are equal. /// public static bool operator ==(KeyTime keyTime1, KeyTime keyTime2) { return KeyTime.Equals(keyTime1, keyTime2); } ////// Returns true if two KeyTimes are not equal. /// public static bool operator !=(KeyTime keyTime1, KeyTime keyTime2) { return !KeyTime.Equals(keyTime1, keyTime2); } #endregion #region IEquatable/// /// Returns true if two KeyTimes are equal. /// public bool Equals(KeyTime value) { return KeyTime.Equals(this, value); } #endregion #region Object Overrides ////// Implementation of public override bool Equals(object value) { if ( value == null || !(value is KeyTime)) { return false; } return this == (KeyTime)value; } ///Object.Equals . ////// Implementation of public override int GetHashCode() { // If we don't have a value (uniform, or paced) then use the type // to determine the hash code if (_value != null) { return _value.GetHashCode(); } else { return _type.GetHashCode(); } } ///Object.GetHashCode . ////// Generates a string representing this KeyTime. /// ///The generated string. public override string ToString() { KeyTimeConverter converter = new KeyTimeConverter(); return converter.ConvertToString(this); } #endregion #region Implicit Converters ////// Implicitly creates a KeyTime value from a Time value. /// /// The Time value. ///A new KeyTime. public static implicit operator KeyTime(TimeSpan timeSpan) { return KeyTime.FromTimeSpan(timeSpan); } #endregion #region Public Properties ////// The Time value for this KeyTime. /// ////// Thrown if the type of this KeyTime isn't KeyTimeType.TimeSpan. /// public TimeSpan TimeSpan { get { if (_type == KeyTimeType.TimeSpan) { return (TimeSpan)_value; } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(); } } } ////// The percent value for this KeyTime. /// ////// Thrown if the type of this KeyTime isn't KeyTimeType.Percent. /// public double Percent { get { if (_type == KeyTimeType.Percent) { return (double)_value; } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(); } } } ////// The type of this KeyTime. /// public KeyTimeType Type { get { return _type; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // KeyTime.cs // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// A KeyTime is use to specify when relative to the time of an animation /// that a KeyFrame takes place. /// [TypeConverter(typeof(KeyTimeConverter))] public struct KeyTime : IEquatable{ private object _value; private KeyTimeType _type; #region Static Create Methods /// /// Creates a KeyTime that represents a Percent value. /// /// /// The percent value provided as a double value between 0.0 and 1.0. /// public static KeyTime FromPercent(double percent) { if (percent < 0.0 || percent > 1.0) { throw new ArgumentOutOfRangeException("percent", SR.Get(SRID.Animation_KeyTime_InvalidPercentValue, percent)); } KeyTime keyTime = new KeyTime(); keyTime._value = percent; keyTime._type = KeyTimeType.Percent; return keyTime; } ////// /// /// public static KeyTime FromTimeSpan(TimeSpan timeSpan) { if (timeSpan < TimeSpan.Zero) { throw new ArgumentOutOfRangeException("timeSpan", SR.Get(SRID.Animation_KeyTime_LessThanZero, timeSpan)); } KeyTime keyTime = new KeyTime(); keyTime._value = timeSpan; keyTime._type = KeyTimeType.TimeSpan; return keyTime; } ////// /// ///public static KeyTime Uniform { get { KeyTime keyTime = new KeyTime(); keyTime._type = KeyTimeType.Uniform; return keyTime; } } /// /// /// ///public static KeyTime Paced { get { KeyTime keyTime = new KeyTime(); keyTime._type = KeyTimeType.Paced; return keyTime; } } #endregion #region Operators /// /// Returns true if two KeyTimes are equal. /// public static bool Equals(KeyTime keyTime1, KeyTime keyTime2) { if (keyTime1._type == keyTime2._type) { switch (keyTime1._type) { case KeyTimeType.Uniform: break; case KeyTimeType.Paced: break; case KeyTimeType.Percent: if ((double)keyTime1._value != (double)keyTime2._value) { return false; } break; case KeyTimeType.TimeSpan: if ((TimeSpan)keyTime1._value != (TimeSpan)keyTime2._value) { return false; } break; } return true; } else { return false; } } ////// Returns true if two KeyTimes are equal. /// public static bool operator ==(KeyTime keyTime1, KeyTime keyTime2) { return KeyTime.Equals(keyTime1, keyTime2); } ////// Returns true if two KeyTimes are not equal. /// public static bool operator !=(KeyTime keyTime1, KeyTime keyTime2) { return !KeyTime.Equals(keyTime1, keyTime2); } #endregion #region IEquatable/// /// Returns true if two KeyTimes are equal. /// public bool Equals(KeyTime value) { return KeyTime.Equals(this, value); } #endregion #region Object Overrides ////// Implementation of public override bool Equals(object value) { if ( value == null || !(value is KeyTime)) { return false; } return this == (KeyTime)value; } ///Object.Equals . ////// Implementation of public override int GetHashCode() { // If we don't have a value (uniform, or paced) then use the type // to determine the hash code if (_value != null) { return _value.GetHashCode(); } else { return _type.GetHashCode(); } } ///Object.GetHashCode . ////// Generates a string representing this KeyTime. /// ///The generated string. public override string ToString() { KeyTimeConverter converter = new KeyTimeConverter(); return converter.ConvertToString(this); } #endregion #region Implicit Converters ////// Implicitly creates a KeyTime value from a Time value. /// /// The Time value. ///A new KeyTime. public static implicit operator KeyTime(TimeSpan timeSpan) { return KeyTime.FromTimeSpan(timeSpan); } #endregion #region Public Properties ////// The Time value for this KeyTime. /// ////// Thrown if the type of this KeyTime isn't KeyTimeType.TimeSpan. /// public TimeSpan TimeSpan { get { if (_type == KeyTimeType.TimeSpan) { return (TimeSpan)_value; } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(); } } } ////// The percent value for this KeyTime. /// ////// Thrown if the type of this KeyTime isn't KeyTimeType.Percent. /// public double Percent { get { if (_type == KeyTimeType.Percent) { return (double)_value; } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(); } } } ////// The type of this KeyTime. /// public KeyTimeType Type { get { return _type; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
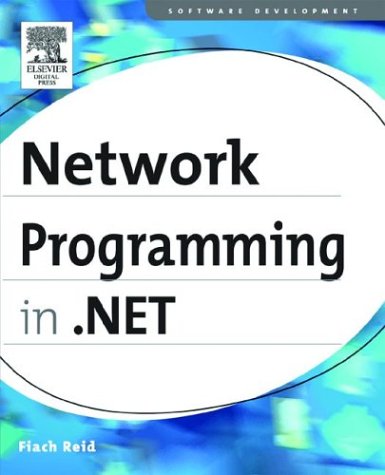
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyControlCollection.cs
- PolicyManager.cs
- ZipPackagePart.cs
- Point.cs
- COM2PictureConverter.cs
- StyleSheetRefUrlEditor.cs
- WSHttpSecurityElement.cs
- ControlBuilderAttribute.cs
- CachedBitmap.cs
- ClientScriptManagerWrapper.cs
- XmlResolver.cs
- ClassDataContract.cs
- Underline.cs
- OdbcParameterCollection.cs
- DeferredReference.cs
- InternalMappingException.cs
- SiteMapSection.cs
- Int16Converter.cs
- FtpRequestCacheValidator.cs
- HMAC.cs
- WindowPattern.cs
- ObjectDataSourceSelectingEventArgs.cs
- COM2ColorConverter.cs
- EventRecordWrittenEventArgs.cs
- XmlSerializerSection.cs
- RSAOAEPKeyExchangeFormatter.cs
- SchemaLookupTable.cs
- LayoutEngine.cs
- Command.cs
- XmlAttributeProperties.cs
- CorrelationService.cs
- SaveCardRequest.cs
- Composition.cs
- DelayedRegex.cs
- ContextQuery.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- ClonableStack.cs
- remotingproxy.cs
- CryptographicAttribute.cs
- HMACRIPEMD160.cs
- MatrixConverter.cs
- XPathBinder.cs
- Utils.cs
- RelationshipDetailsCollection.cs
- MenuAdapter.cs
- namescope.cs
- DecoderBestFitFallback.cs
- AssociationEndMember.cs
- SourceInterpreter.cs
- ProxySimple.cs
- TypeUtil.cs
- SignatureResourcePool.cs
- BuildResultCache.cs
- XmlSchemaAttributeGroupRef.cs
- BatchStream.cs
- listitem.cs
- ConfigurationException.cs
- SimpleWorkerRequest.cs
- ExpandoObject.cs
- AsyncInvokeOperation.cs
- BitmapEffectInputConnector.cs
- FtpCachePolicyElement.cs
- WmlLinkAdapter.cs
- ListItem.cs
- EntryPointNotFoundException.cs
- x509utils.cs
- KeyboardEventArgs.cs
- XmlWellformedWriterHelpers.cs
- DateTimeOffsetAdapter.cs
- KeyManager.cs
- sqlpipe.cs
- FileDialogCustomPlace.cs
- EmptyElement.cs
- ActiveXSite.cs
- ReferencedAssemblyResolver.cs
- WebServiceHost.cs
- XamlInterfaces.cs
- SqlServices.cs
- ToolboxDataAttribute.cs
- EntityTypeEmitter.cs
- SpellerInterop.cs
- DrawingGroup.cs
- ArrangedElement.cs
- SearchForVirtualItemEventArgs.cs
- ParserExtension.cs
- RpcAsyncResult.cs
- InternalsVisibleToAttribute.cs
- UseLicense.cs
- TreeNodeClickEventArgs.cs
- StrongNameMembershipCondition.cs
- ComponentDispatcher.cs
- DataContractJsonSerializer.cs
- MailBnfHelper.cs
- SelectionEditor.cs
- EntityCollectionChangedParams.cs
- NativeMethods.cs
- KeyEventArgs.cs
- DiscoveryDefaults.cs
- WindowsFormsSynchronizationContext.cs
- KeyValueSerializer.cs