Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / XmlAttributeProperties.cs / 1 / XmlAttributeProperties.cs
//---------------------------------------------------------------------------- // // File: XmlAttributeProperties.cs // // Description: // Attributes used by parser for Avalon // // // History: // 6/06/01: rogerg Created // 5/23/03: peterost Ported to wcp // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Diagnostics; using System.Reflection; using MS.Utility; #if !PBTCOMPILER using System.Windows; using System.Windows.Navigation; using System.Windows.Threading; #endif #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ////// A class to encapsulate XML-specific attributes of a DependencyObject /// #if PBTCOMPILER internal sealed class XmlAttributeProperties #else public sealed class XmlAttributeProperties #endif { #if !PBTCOMPILER #region Public Methods // This is a dummy contructor to prevent people // from being able to instantiate this class private XmlAttributeProperties() { } static XmlAttributeProperties() { XmlSpaceProperty = DependencyProperty.RegisterAttached("XmlSpace", typeof(string), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata("default")); XmlnsDictionaryProperty = DependencyProperty.RegisterAttached("XmlnsDictionary", typeof(XmlnsDictionary), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata((object)null, FrameworkPropertyMetadataOptions.Inherits)); XmlnsDefinitionProperty = DependencyProperty.RegisterAttached("XmlnsDefinition", typeof(string), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata(XamlReaderHelper.DefinitionNamespaceURI, FrameworkPropertyMetadataOptions.Inherits)); XmlNamespaceMapsProperty = DependencyProperty.RegisterAttached("XmlNamespaceMaps", typeof(Hashtable), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata(null, FrameworkPropertyMetadataOptions.Inherits)); } #if Lookups ////// Looks up the namespace corresponding to an XML namespace prefix /// /// The DependencyObject containing the namespace mappings to be /// used in the lookup /// The XML namespace prefix to look up ///The namespace corresponding to the given prefix if it exists, /// null otherwise public static string LookupNamespace(DependencyObject elem, string prefix) { if (elem == null) { throw new ArgumentNullException( "elem" ); } if (prefix == null) { throw new ArgumentNullException( "prefix" ); } // Walk up the parent chain until one of the parents has the passed prefix in // its xmlns dictionary, or until a null parent is reached. XmlnsDictionary d = GetXmlnsDictionary(elem); while ((null == d || null == d[prefix]) && null != elem) { elem = LogicalTreeHelper.GetParent(elem); if (elem != null) { d = GetXmlnsDictionary(elem); } } if (null != d) { return d[prefix]; } else { return null; } } ////// Looks up the XML prefix corresponding to a namespaceUri /// /// The DependencyObject containing the namespace mappings to /// be used in the lookup /// The namespaceUri to look up ////// string.Empty if the given namespace corresponds to the default namespace; otherwise, /// the XML prefix corresponding to the given namespace, or null if none exists /// public static string LookupPrefix(DependencyObject elem, string uri) { DependencyObject oldElem = elem; if (elem == null) { throw new ArgumentNullException( "elem" ); } if (uri == null) { throw new ArgumentNullException( "uri" ); } // Following the letter of the specification, the default namespace should take // precedence in the case of redundancy, so we check that first. if (DefaultNamespace(elem) == uri) return string.Empty; while (null != elem) { XmlnsDictionary d = GetXmlnsDictionary(elem); if (null != d) { // Search through all key/value pairs in the dictionary foreach (DictionaryEntry e in d) { if ((string)e.Value == uri && LookupNamespace(oldElem, (string)e.Key) == uri) { return (string)e.Key; } } } elem = LogicalTreeHelper.GetParent(elem); } return null; } // Get the Default Namespace same as LookupNamespace(e,String.Empty); ////// Returns the default namespaceUri for the given DependencyObject /// /// The element containing the namespace mappings to use in the lookup ///The default namespaceUri for the given Element public static string DefaultNamespace(DependencyObject e) { return LookupNamespace(e, string.Empty); } #endif #endregion Public Methods #region AttachedProperties ////// xml:space Element property /// [Browsable(false)] [Localizability(LocalizationCategory.NeverLocalize)] public static readonly DependencyProperty XmlSpaceProperty ; ////// Return value of xml:space on the passed DependencyObject /// [DesignerSerializationOptions(DesignerSerializationOptions.SerializeAsAttribute)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static string GetXmlSpace(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (string)dependencyObject.GetValue(XmlSpaceProperty); } ////// Set value of xml:space on the passed DependencyObject /// public static void SetXmlSpace(DependencyObject dependencyObject, string value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlSpaceProperty, value); } ////// XmlnsDictionary Element property /// [Browsable(false)] public static readonly DependencyProperty XmlnsDictionaryProperty ; ////// Return value of XmlnsDictionary on the passed DependencyObject /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static XmlnsDictionary GetXmlnsDictionary(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (XmlnsDictionary)dependencyObject.GetValue(XmlnsDictionaryProperty); } ////// Set value of XmlnsDictionary on the passed DependencyObject /// public static void SetXmlnsDictionary(DependencyObject dependencyObject, XmlnsDictionary value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlnsDictionaryProperty, value); } ////// XmlnsDefinition Directory property /// [Browsable(false)] public static readonly DependencyProperty XmlnsDefinitionProperty ; ////// Return value of xmlns definition directory on the passed DependencyObject /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] [DesignerSerializationOptions(DesignerSerializationOptions.SerializeAsAttribute)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static string GetXmlnsDefinition(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (string)dependencyObject.GetValue(XmlnsDefinitionProperty); } ////// Set value of xmlns definition directory on the passed DependencyObject /// public static void SetXmlnsDefinition(DependencyObject dependencyObject, string value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlnsDefinitionProperty, value); } ////// XmlNamespaceMaps that map xml namespace uri to assembly/clr namespaces /// [Browsable(false)] public static readonly DependencyProperty XmlNamespaceMapsProperty ; ////// Return value of XmlNamespaceMaps on the passed DependencyObject /// ////// XmlNamespaceMaps map xml namespace uri to Assembly/CLR namespaces /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static string GetXmlNamespaceMaps(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (string)dependencyObject.GetValue(XmlNamespaceMapsProperty); } ////// Set value of XmlNamespaceMaps on the passed DependencyObject /// ////// XmlNamespaceMaps map xml namespace uri to Assembly/CLR namespaces /// public static void SetXmlNamespaceMaps(DependencyObject dependencyObject, string value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlNamespaceMapsProperty, value); } #endregion AttachedProperties #else private XmlAttributeProperties() { } #endif // temporal PBT #region Internal // Return the setter method info for XmlSpace internal static MethodInfo XmlSpaceSetter { get { if (_xmlSpaceSetter == null) { _xmlSpaceSetter = typeof(XmlAttributeProperties).GetMethod("SetXmlSpace", BindingFlags.Public | BindingFlags.Static); } return _xmlSpaceSetter; } } // These are special attributes that aren't mapped like other properties internal static readonly string XmlSpaceString = "xml:space"; internal static readonly string XmlLangString = "xml:lang"; internal static readonly string XmlnsDefinitionString = "xmlns"; private static MethodInfo _xmlSpaceSetter = null; #endregion Internal } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XmlAttributeProperties.cs // // Description: // Attributes used by parser for Avalon // // // History: // 6/06/01: rogerg Created // 5/23/03: peterost Ported to wcp // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Diagnostics; using System.Reflection; using MS.Utility; #if !PBTCOMPILER using System.Windows; using System.Windows.Navigation; using System.Windows.Threading; #endif #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ////// A class to encapsulate XML-specific attributes of a DependencyObject /// #if PBTCOMPILER internal sealed class XmlAttributeProperties #else public sealed class XmlAttributeProperties #endif { #if !PBTCOMPILER #region Public Methods // This is a dummy contructor to prevent people // from being able to instantiate this class private XmlAttributeProperties() { } static XmlAttributeProperties() { XmlSpaceProperty = DependencyProperty.RegisterAttached("XmlSpace", typeof(string), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata("default")); XmlnsDictionaryProperty = DependencyProperty.RegisterAttached("XmlnsDictionary", typeof(XmlnsDictionary), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata((object)null, FrameworkPropertyMetadataOptions.Inherits)); XmlnsDefinitionProperty = DependencyProperty.RegisterAttached("XmlnsDefinition", typeof(string), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata(XamlReaderHelper.DefinitionNamespaceURI, FrameworkPropertyMetadataOptions.Inherits)); XmlNamespaceMapsProperty = DependencyProperty.RegisterAttached("XmlNamespaceMaps", typeof(Hashtable), typeof(XmlAttributeProperties), new FrameworkPropertyMetadata(null, FrameworkPropertyMetadataOptions.Inherits)); } #if Lookups ////// Looks up the namespace corresponding to an XML namespace prefix /// /// The DependencyObject containing the namespace mappings to be /// used in the lookup /// The XML namespace prefix to look up ///The namespace corresponding to the given prefix if it exists, /// null otherwise public static string LookupNamespace(DependencyObject elem, string prefix) { if (elem == null) { throw new ArgumentNullException( "elem" ); } if (prefix == null) { throw new ArgumentNullException( "prefix" ); } // Walk up the parent chain until one of the parents has the passed prefix in // its xmlns dictionary, or until a null parent is reached. XmlnsDictionary d = GetXmlnsDictionary(elem); while ((null == d || null == d[prefix]) && null != elem) { elem = LogicalTreeHelper.GetParent(elem); if (elem != null) { d = GetXmlnsDictionary(elem); } } if (null != d) { return d[prefix]; } else { return null; } } ////// Looks up the XML prefix corresponding to a namespaceUri /// /// The DependencyObject containing the namespace mappings to /// be used in the lookup /// The namespaceUri to look up ////// string.Empty if the given namespace corresponds to the default namespace; otherwise, /// the XML prefix corresponding to the given namespace, or null if none exists /// public static string LookupPrefix(DependencyObject elem, string uri) { DependencyObject oldElem = elem; if (elem == null) { throw new ArgumentNullException( "elem" ); } if (uri == null) { throw new ArgumentNullException( "uri" ); } // Following the letter of the specification, the default namespace should take // precedence in the case of redundancy, so we check that first. if (DefaultNamespace(elem) == uri) return string.Empty; while (null != elem) { XmlnsDictionary d = GetXmlnsDictionary(elem); if (null != d) { // Search through all key/value pairs in the dictionary foreach (DictionaryEntry e in d) { if ((string)e.Value == uri && LookupNamespace(oldElem, (string)e.Key) == uri) { return (string)e.Key; } } } elem = LogicalTreeHelper.GetParent(elem); } return null; } // Get the Default Namespace same as LookupNamespace(e,String.Empty); ////// Returns the default namespaceUri for the given DependencyObject /// /// The element containing the namespace mappings to use in the lookup ///The default namespaceUri for the given Element public static string DefaultNamespace(DependencyObject e) { return LookupNamespace(e, string.Empty); } #endif #endregion Public Methods #region AttachedProperties ////// xml:space Element property /// [Browsable(false)] [Localizability(LocalizationCategory.NeverLocalize)] public static readonly DependencyProperty XmlSpaceProperty ; ////// Return value of xml:space on the passed DependencyObject /// [DesignerSerializationOptions(DesignerSerializationOptions.SerializeAsAttribute)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static string GetXmlSpace(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (string)dependencyObject.GetValue(XmlSpaceProperty); } ////// Set value of xml:space on the passed DependencyObject /// public static void SetXmlSpace(DependencyObject dependencyObject, string value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlSpaceProperty, value); } ////// XmlnsDictionary Element property /// [Browsable(false)] public static readonly DependencyProperty XmlnsDictionaryProperty ; ////// Return value of XmlnsDictionary on the passed DependencyObject /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static XmlnsDictionary GetXmlnsDictionary(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (XmlnsDictionary)dependencyObject.GetValue(XmlnsDictionaryProperty); } ////// Set value of XmlnsDictionary on the passed DependencyObject /// public static void SetXmlnsDictionary(DependencyObject dependencyObject, XmlnsDictionary value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlnsDictionaryProperty, value); } ////// XmlnsDefinition Directory property /// [Browsable(false)] public static readonly DependencyProperty XmlnsDefinitionProperty ; ////// Return value of xmlns definition directory on the passed DependencyObject /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] [DesignerSerializationOptions(DesignerSerializationOptions.SerializeAsAttribute)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static string GetXmlnsDefinition(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (string)dependencyObject.GetValue(XmlnsDefinitionProperty); } ////// Set value of xmlns definition directory on the passed DependencyObject /// public static void SetXmlnsDefinition(DependencyObject dependencyObject, string value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlnsDefinitionProperty, value); } ////// XmlNamespaceMaps that map xml namespace uri to assembly/clr namespaces /// [Browsable(false)] public static readonly DependencyProperty XmlNamespaceMapsProperty ; ////// Return value of XmlNamespaceMaps on the passed DependencyObject /// ////// XmlNamespaceMaps map xml namespace uri to Assembly/CLR namespaces /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static string GetXmlNamespaceMaps(DependencyObject dependencyObject) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } return (string)dependencyObject.GetValue(XmlNamespaceMapsProperty); } ////// Set value of XmlNamespaceMaps on the passed DependencyObject /// ////// XmlNamespaceMaps map xml namespace uri to Assembly/CLR namespaces /// public static void SetXmlNamespaceMaps(DependencyObject dependencyObject, string value) { if (dependencyObject == null) { throw new ArgumentNullException( "dependencyObject" ); } dependencyObject.SetValue(XmlNamespaceMapsProperty, value); } #endregion AttachedProperties #else private XmlAttributeProperties() { } #endif // temporal PBT #region Internal // Return the setter method info for XmlSpace internal static MethodInfo XmlSpaceSetter { get { if (_xmlSpaceSetter == null) { _xmlSpaceSetter = typeof(XmlAttributeProperties).GetMethod("SetXmlSpace", BindingFlags.Public | BindingFlags.Static); } return _xmlSpaceSetter; } } // These are special attributes that aren't mapped like other properties internal static readonly string XmlSpaceString = "xml:space"; internal static readonly string XmlLangString = "xml:lang"; internal static readonly string XmlnsDefinitionString = "xmlns"; private static MethodInfo _xmlSpaceSetter = null; #endregion Internal } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
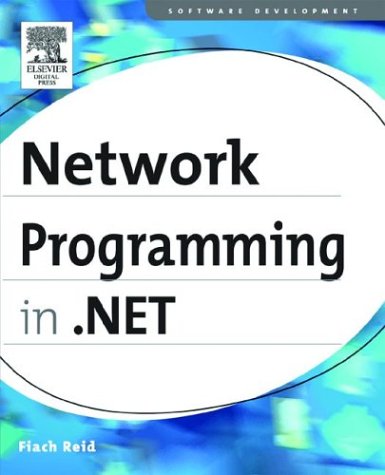
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509ThumbprintKeyIdentifierClause.cs
- EntityWrapperFactory.cs
- PackageStore.cs
- CorePropertiesFilter.cs
- HtmlInputFile.cs
- TypeGeneratedEventArgs.cs
- LogicalExpr.cs
- TaskFormBase.cs
- RowUpdatedEventArgs.cs
- ViewBox.cs
- SaveFileDialog.cs
- SQLBinary.cs
- CompilerState.cs
- ManifestBasedResourceGroveler.cs
- ListBindingConverter.cs
- EdmError.cs
- OutputCacheProfile.cs
- PackUriHelper.cs
- Fonts.cs
- HashAlgorithm.cs
- ProxyAttribute.cs
- Trace.cs
- DbConnectionClosed.cs
- XPathNavigatorReader.cs
- ComNativeDescriptor.cs
- FieldNameLookup.cs
- ExpressionConverter.cs
- CultureTable.cs
- XmlSchemaException.cs
- xml.cs
- ControlUtil.cs
- HttpFileCollection.cs
- BufferedGraphics.cs
- SocketAddress.cs
- MarkupCompiler.cs
- ApplicationHost.cs
- XmlAttribute.cs
- ForeignConstraint.cs
- TableSectionStyle.cs
- XmlSerializer.cs
- XmlBoundElement.cs
- MediaElementAutomationPeer.cs
- SystemNetworkInterface.cs
- SqlDataRecord.cs
- Mappings.cs
- DrawTreeNodeEventArgs.cs
- SafeCryptoHandles.cs
- AdvancedBindingPropertyDescriptor.cs
- PersonalizationStateInfoCollection.cs
- RoleBoolean.cs
- TemplatedAdorner.cs
- PopupControlService.cs
- AssemblyCache.cs
- SimpleRecyclingCache.cs
- WebConfigurationHost.cs
- EventLog.cs
- SrgsDocument.cs
- OptionUsage.cs
- HttpListenerPrefixCollection.cs
- ModulesEntry.cs
- TCPListener.cs
- WriteStateInfoBase.cs
- WebHostUnsafeNativeMethods.cs
- BaseParser.cs
- WorkflowValidationFailedException.cs
- CheckedPointers.cs
- LinqDataSourceUpdateEventArgs.cs
- DesignerActionVerbList.cs
- ResourcePermissionBase.cs
- ThreadAbortException.cs
- BitmapEffectInput.cs
- MobileComponentEditorPage.cs
- DataStreamFromComStream.cs
- PublisherIdentityPermission.cs
- SafeNativeMethods.cs
- CodeMemberEvent.cs
- AspNetSynchronizationContext.cs
- Table.cs
- clipboard.cs
- ConnectionProviderAttribute.cs
- XmlWhitespace.cs
- MultiTrigger.cs
- XXXInfos.cs
- FontWeightConverter.cs
- LinearKeyFrames.cs
- ConfigurationValues.cs
- ProgressBarAutomationPeer.cs
- TypeUtil.cs
- WebReference.cs
- KernelTypeValidation.cs
- Model3DGroup.cs
- OrCondition.cs
- ErrorFormatterPage.cs
- DataGridViewTopLeftHeaderCell.cs
- EntityDataSourceDesigner.cs
- IconHelper.cs
- HorizontalAlignConverter.cs
- DataGridLength.cs
- CapacityStreamGeometryContext.cs
- ToolStripMenuItemDesigner.cs