Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / Host / DesignSurfaceCollection.cs / 1 / DesignSurfaceCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; ////// /// Provides a read-only collection of design surfaces. /// public sealed class DesignSurfaceCollection : ICollection { private DesignerCollection _designers; ////// Initializes a new instance of the DesignSurfaceCollection class /// internal DesignSurfaceCollection(DesignerCollection designers) { _designers = designers; if (_designers == null) { _designers = new DesignerCollection(null); } } ////// /// Gets number of design surfaces in the collection. /// public int Count { get { return _designers.Count; } } ////// /// Gets or sets the document at the specified index. /// public DesignSurface this[int index] { get { IDesignerHost host = _designers[index]; DesignSurface surface = host.GetService(typeof(DesignSurface)) as DesignSurface; if (surface == null) { throw new NotSupportedException(); } return surface; } } ////// /// Creates and retrieves a new enumerator for this collection. /// public IEnumerator GetEnumerator() { return new DesignSurfaceEnumerator(_designers.GetEnumerator()); } ////// int ICollection.Count { get { return Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return null; } } /// /// void ICollection.CopyTo(Array array, int index) { foreach(DesignSurface surface in this) { array.SetValue(surface, index++); } } public void CopyTo(DesignSurface[] array, int index) { ((ICollection)this).CopyTo(array, index); } /// /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// /// Enumerator that performs the conversion from designer host /// to design surface. /// private class DesignSurfaceEnumerator : IEnumerator { private IEnumerator _designerEnumerator; internal DesignSurfaceEnumerator(IEnumerator designerEnumerator) { _designerEnumerator = designerEnumerator; } public object Current { get { IDesignerHost host = (IDesignerHost)_designerEnumerator.Current; DesignSurface surface = host.GetService(typeof(DesignSurface)) as DesignSurface; if (surface == null) { throw new NotSupportedException(); } return surface; } } public bool MoveNext() { return _designerEnumerator.MoveNext(); } public void Reset() { _designerEnumerator.Reset(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
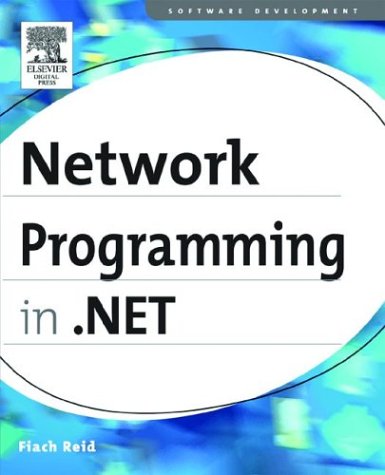
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecondaryViewProvider.cs
- DynamicField.cs
- VirtualizedCellInfoCollection.cs
- WindowHelperService.cs
- KnownBoxes.cs
- LZCodec.cs
- SqlTrackingQuery.cs
- VariableQuery.cs
- OleDbException.cs
- GridViewRow.cs
- OleDbParameter.cs
- Properties.cs
- NotSupportedException.cs
- WindowsRebar.cs
- FtpRequestCacheValidator.cs
- InstanceOwnerQueryResult.cs
- BitmapScalingModeValidation.cs
- DataServiceQueryContinuation.cs
- EntityContainer.cs
- ErrorStyle.cs
- CancellationScope.cs
- Expressions.cs
- HitTestWithGeometryDrawingContextWalker.cs
- ProjectionPathSegment.cs
- DiscoveryVersion.cs
- HttpProfileGroupBase.cs
- TraceContextEventArgs.cs
- LambdaCompiler.Generated.cs
- XmlStrings.cs
- __Filters.cs
- AttributeUsageAttribute.cs
- BaseValidator.cs
- AuthenticatingEventArgs.cs
- FtpCachePolicyElement.cs
- CodeTypeParameter.cs
- BrowserDefinition.cs
- KnowledgeBase.cs
- GlyphTypeface.cs
- GeometryConverter.cs
- XsdBuilder.cs
- PropertyStore.cs
- DrawItemEvent.cs
- SecurityUtils.cs
- QueryConverter.cs
- RolePrincipal.cs
- AudienceUriMode.cs
- wgx_exports.cs
- TransformGroup.cs
- BindingFormattingDialog.cs
- TextEndOfParagraph.cs
- ToolboxItemCollection.cs
- ThreadAttributes.cs
- AdPostCacheSubstitution.cs
- ExceptionValidationRule.cs
- FaultPropagationRecord.cs
- GradientStop.cs
- xdrvalidator.cs
- recordstatefactory.cs
- SamlNameIdentifierClaimResource.cs
- MatrixAnimationUsingPath.cs
- CompositionTarget.cs
- SystemIPAddressInformation.cs
- OleDbFactory.cs
- ElementHostAutomationPeer.cs
- FontDriver.cs
- CommonDialog.cs
- PropertySet.cs
- XPathBuilder.cs
- RectangleGeometry.cs
- _NestedMultipleAsyncResult.cs
- DataReaderContainer.cs
- ResourcePool.cs
- ImageMapEventArgs.cs
- BooleanFunctions.cs
- CurrencyWrapper.cs
- BinaryReader.cs
- SystemKeyConverter.cs
- SslStreamSecurityElement.cs
- ContentElementAutomationPeer.cs
- Composition.cs
- EventDescriptor.cs
- DBSqlParserColumn.cs
- ProviderUtil.cs
- GenericEnumerator.cs
- WebPartVerbsEventArgs.cs
- StringResourceManager.cs
- securitycriticaldataClass.cs
- FeatureAttribute.cs
- RoutedUICommand.cs
- SecurityTokenProvider.cs
- DataGridViewLinkCell.cs
- OleServicesContext.cs
- SizeAnimationClockResource.cs
- UriTemplateTrieLocation.cs
- ColorAnimationUsingKeyFrames.cs
- TextDecoration.cs
- UIElementPropertyUndoUnit.cs
- KerberosSecurityTokenParameters.cs
- StateWorkerRequest.cs
- IImplicitResourceProvider.cs