Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / internal / materialization / recordstatefactory.cs / 1305376 / recordstatefactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Linq; using System.Linq.Expressions; namespace System.Data.Common.Internal.Materialization { ////// An immutable class used to generate new RecordStates, which are used /// at runtime to produce value-layer (aka DataReader) results. /// /// Contains static information collected by the Translator visitor. The /// expressions produced by the Translator are compiled. The RecordStates /// will refer to this object for all static information. /// /// This class is cached in the query cache as part of the CoordinatorFactory. /// internal class RecordStateFactory { #region state ////// Indicates which state slot in the Shaper.State is expected to hold the /// value for this record state. Each unique record shape has it's own state /// slot. /// internal readonly int StateSlotNumber; ////// How many column values we have to reserve space for in this record. /// internal readonly int ColumnCount; ////// The DataRecordInfo we must return for this record. If the record represents /// an entity, this will be used to construct a unique EntityRecordInfo with the /// EntityKey and EntitySet for the entity. /// internal readonly DataRecordInfo DataRecordInfo; ////// A function that will gather the data for the row and store it on the record state. /// internal readonly FuncGatherData; /// /// Collection of nested records for this record, such as a complex type that is /// part of an entity. This does not include records that are part of a nested /// collection, however. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionNestedRecordStateFactories; /// /// The name for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionColumnNames; /// /// The type usage information for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionTypeUsages; /// /// Tracks which columns might need special handling (nested readers/records) /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionIsColumnNested; /// /// Tracks whether there are ANY columns that need special handling. /// internal readonly bool HasNestedColumns; ////// A helper class to make the translation from name->ordinal. /// internal readonly FieldNameLookup FieldNameLookup; ////// Description of this RecordStateFactory, used for debugging only; while this /// is not needed in retail code, it is pretty important because it's the only /// description we'll have once we compile the Expressions; debugging a problem /// with retail bits would be pretty hard without this. /// private readonly string Description; #endregion #region constructor public RecordStateFactory(int stateSlotNumber, int columnCount, RecordStateFactory[] nestedRecordStateFactories, DataRecordInfo dataRecordInfo, Expression gatherData, string[] propertyNames, TypeUsage[] typeUsages) { this.StateSlotNumber = stateSlotNumber; this.ColumnCount = columnCount; this.NestedRecordStateFactories = new System.Collections.ObjectModel.ReadOnlyCollection(nestedRecordStateFactories); this.DataRecordInfo = dataRecordInfo; this.GatherData = Translator.Compile (gatherData); this.Description = gatherData.ToString(); this.ColumnNames = new System.Collections.ObjectModel.ReadOnlyCollection (propertyNames); this.TypeUsages = new System.Collections.ObjectModel.ReadOnlyCollection (typeUsages); this.FieldNameLookup = new FieldNameLookup(this.ColumnNames, -1); // pre-compute the nested objects from typeUsage, for performance bool[] isColumnNested = new bool[columnCount]; for (int ordinal = 0; ordinal < columnCount; ordinal++) { switch (typeUsages[ordinal].EdmType.BuiltInTypeKind) { case BuiltInTypeKind.EntityType: case BuiltInTypeKind.ComplexType: case BuiltInTypeKind.RowType: case BuiltInTypeKind.CollectionType: isColumnNested[ordinal] = true; this.HasNestedColumns = true; break; default: isColumnNested[ordinal] = false; break; } } this.IsColumnNested = new System.Collections.ObjectModel.ReadOnlyCollection (isColumnNested); } #endregion #region "public" surface area /// /// It's GO time, create the record state. /// internal RecordState Create(CoordinatorFactory coordinatorFactory) { return new RecordState(this, coordinatorFactory); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Linq; using System.Linq.Expressions; namespace System.Data.Common.Internal.Materialization { ////// An immutable class used to generate new RecordStates, which are used /// at runtime to produce value-layer (aka DataReader) results. /// /// Contains static information collected by the Translator visitor. The /// expressions produced by the Translator are compiled. The RecordStates /// will refer to this object for all static information. /// /// This class is cached in the query cache as part of the CoordinatorFactory. /// internal class RecordStateFactory { #region state ////// Indicates which state slot in the Shaper.State is expected to hold the /// value for this record state. Each unique record shape has it's own state /// slot. /// internal readonly int StateSlotNumber; ////// How many column values we have to reserve space for in this record. /// internal readonly int ColumnCount; ////// The DataRecordInfo we must return for this record. If the record represents /// an entity, this will be used to construct a unique EntityRecordInfo with the /// EntityKey and EntitySet for the entity. /// internal readonly DataRecordInfo DataRecordInfo; ////// A function that will gather the data for the row and store it on the record state. /// internal readonly FuncGatherData; /// /// Collection of nested records for this record, such as a complex type that is /// part of an entity. This does not include records that are part of a nested /// collection, however. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionNestedRecordStateFactories; /// /// The name for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionColumnNames; /// /// The type usage information for each column. /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionTypeUsages; /// /// Tracks which columns might need special handling (nested readers/records) /// internal readonly System.Collections.ObjectModel.ReadOnlyCollectionIsColumnNested; /// /// Tracks whether there are ANY columns that need special handling. /// internal readonly bool HasNestedColumns; ////// A helper class to make the translation from name->ordinal. /// internal readonly FieldNameLookup FieldNameLookup; ////// Description of this RecordStateFactory, used for debugging only; while this /// is not needed in retail code, it is pretty important because it's the only /// description we'll have once we compile the Expressions; debugging a problem /// with retail bits would be pretty hard without this. /// private readonly string Description; #endregion #region constructor public RecordStateFactory(int stateSlotNumber, int columnCount, RecordStateFactory[] nestedRecordStateFactories, DataRecordInfo dataRecordInfo, Expression gatherData, string[] propertyNames, TypeUsage[] typeUsages) { this.StateSlotNumber = stateSlotNumber; this.ColumnCount = columnCount; this.NestedRecordStateFactories = new System.Collections.ObjectModel.ReadOnlyCollection(nestedRecordStateFactories); this.DataRecordInfo = dataRecordInfo; this.GatherData = Translator.Compile (gatherData); this.Description = gatherData.ToString(); this.ColumnNames = new System.Collections.ObjectModel.ReadOnlyCollection (propertyNames); this.TypeUsages = new System.Collections.ObjectModel.ReadOnlyCollection (typeUsages); this.FieldNameLookup = new FieldNameLookup(this.ColumnNames, -1); // pre-compute the nested objects from typeUsage, for performance bool[] isColumnNested = new bool[columnCount]; for (int ordinal = 0; ordinal < columnCount; ordinal++) { switch (typeUsages[ordinal].EdmType.BuiltInTypeKind) { case BuiltInTypeKind.EntityType: case BuiltInTypeKind.ComplexType: case BuiltInTypeKind.RowType: case BuiltInTypeKind.CollectionType: isColumnNested[ordinal] = true; this.HasNestedColumns = true; break; default: isColumnNested[ordinal] = false; break; } } this.IsColumnNested = new System.Collections.ObjectModel.ReadOnlyCollection (isColumnNested); } #endregion #region "public" surface area /// /// It's GO time, create the record state. /// internal RecordState Create(CoordinatorFactory coordinatorFactory) { return new RecordState(this, coordinatorFactory); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
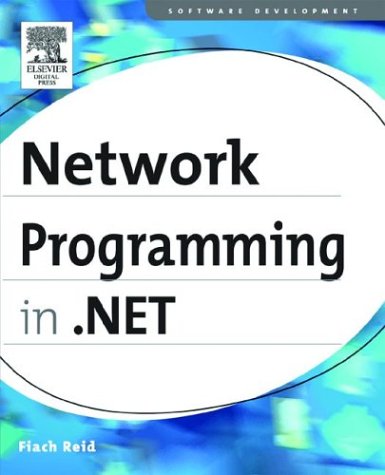
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InertiaExpansionBehavior.cs
- ICspAsymmetricAlgorithm.cs
- TableAdapterManagerNameHandler.cs
- ListControlConvertEventArgs.cs
- XmlDomTextWriter.cs
- DataBoundControlAdapter.cs
- ClickablePoint.cs
- PageParser.cs
- CLSCompliantAttribute.cs
- TypeExtension.cs
- DependencyObjectType.cs
- DesignTableCollection.cs
- TreeIterators.cs
- PageRanges.cs
- WebPermission.cs
- ProvidePropertyAttribute.cs
- SurrogateChar.cs
- DesignerForm.cs
- EntityDataSourceDesigner.cs
- ReadOnlyDataSource.cs
- ObjectListComponentEditor.cs
- safesecurityhelperavalon.cs
- OrderedDictionaryStateHelper.cs
- DoubleLinkListEnumerator.cs
- MD5.cs
- RootCodeDomSerializer.cs
- DataGridViewSortCompareEventArgs.cs
- ListViewGroup.cs
- UrlRoutingModule.cs
- OutputScopeManager.cs
- ToolZoneDesigner.cs
- _TLSstream.cs
- EventDescriptor.cs
- XomlSerializationHelpers.cs
- _HelperAsyncResults.cs
- JumpTask.cs
- AsyncOperationManager.cs
- EntryIndex.cs
- XmlILTrace.cs
- streamingZipPartStream.cs
- TypeConverterHelper.cs
- CharacterShapingProperties.cs
- ToolStripScrollButton.cs
- RTLAwareMessageBox.cs
- KeyboardDevice.cs
- InputReportEventArgs.cs
- EnumerationRangeValidationUtil.cs
- ListMarkerLine.cs
- Misc.cs
- UrlPath.cs
- LayoutManager.cs
- InputBinding.cs
- PrintPreviewGraphics.cs
- SqlCaseSimplifier.cs
- TableColumnCollectionInternal.cs
- XamlClipboardData.cs
- NumericExpr.cs
- TransactionFlowOption.cs
- HttpListenerResponse.cs
- TypeDescriptionProviderAttribute.cs
- GlyphTypeface.cs
- DBSqlParserColumn.cs
- DataTemplateSelector.cs
- XPathNavigatorReader.cs
- FunctionParameter.cs
- XmlDataSource.cs
- ListItemViewAttribute.cs
- AddressUtility.cs
- OdbcConnectionFactory.cs
- userdatakeys.cs
- Cursor.cs
- Rect3D.cs
- AppDomainShutdownMonitor.cs
- DataGridViewCellStateChangedEventArgs.cs
- HttpClientChannel.cs
- ReflectionTypeLoadException.cs
- PropagatorResult.cs
- ExceptionHandlers.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- ProcessHostMapPath.cs
- XmlSchemaChoice.cs
- PropertySet.cs
- DataSourceUtil.cs
- DateTimeOffsetConverter.cs
- ProcessHostServerConfig.cs
- QualificationDataAttribute.cs
- AttachInfo.cs
- UniqueSet.cs
- DataGridItem.cs
- RuleDefinitions.cs
- _AutoWebProxyScriptWrapper.cs
- UserMapPath.cs
- ProxyHwnd.cs
- BezierSegment.cs
- EventNotify.cs
- DatagridviewDisplayedBandsData.cs
- DesignerActionUIService.cs
- ToolboxItemCollection.cs
- OperationAbortedException.cs
- TypeGenericEnumerableViewSchema.cs