Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DLinq / Dlinq / CompiledQuery.cs / 1 / CompiledQuery.cs
using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Data; using System.Data.Common; using System.Linq.Expressions; using System.Globalization; using System.IO; using System.Linq; using System.Reflection; using System.Text; using System.Xml; using System.Transactions; using System.Data.Linq.Provider; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public sealed class CompiledQuery { LambdaExpression query; ICompiledQuery compiled; private CompiledQuery(LambdaExpression query) { this.query = query; } public LambdaExpression Expression { get { return this.query; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static FuncCompile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } private static bool UseExpressionCompile(LambdaExpression query) { return typeof(ITable).IsAssignableFrom(query.Body.Type); } private TResult Invoke (TArg0 arg0) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0}); } private TResult Invoke (TArg0 arg0, TArg1 arg1) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3}); } private object ExecuteQuery(DataContext context, object[] args) { if (context == null) { throw Error.ArgumentNull("context"); } if (this.compiled == null) { lock (this) { if (this.compiled == null) { this.compiled = context.Provider.Compile(this.query); } } } return this.compiled.Execute(context.Provider, args).ReturnValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Data; using System.Data.Common; using System.Linq.Expressions; using System.Globalization; using System.IO; using System.Linq; using System.Reflection; using System.Text; using System.Xml; using System.Transactions; using System.Data.Linq.Provider; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public sealed class CompiledQuery { LambdaExpression query; ICompiledQuery compiled; private CompiledQuery(LambdaExpression query) { this.query = query; } public LambdaExpression Expression { get { return this.query; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } private static bool UseExpressionCompile(LambdaExpression query) { return typeof(ITable).IsAssignableFrom(query.Body.Type); } private TResult Invoke (TArg0 arg0) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0}); } private TResult Invoke (TArg0 arg0, TArg1 arg1) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3}); } private object ExecuteQuery(DataContext context, object[] args) { if (context == null) { throw Error.ArgumentNull("context"); } if (this.compiled == null) { lock (this) { if (this.compiled == null) { this.compiled = context.Provider.Compile(this.query); } } } return this.compiled.Execute(context.Provider, args).ReturnValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
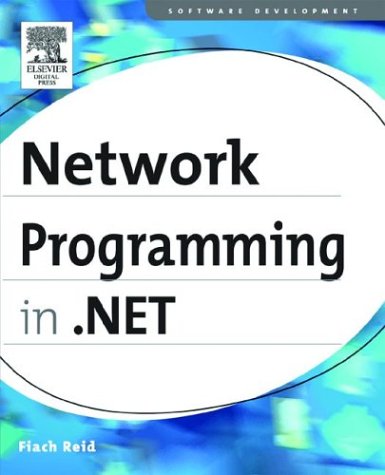
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InfoCardProofToken.cs
- InkSerializer.cs
- TimeZone.cs
- NameTable.cs
- BezierSegment.cs
- VirtualizingStackPanel.cs
- InvokeHandlers.cs
- HierarchicalDataBoundControl.cs
- FontFamilyValueSerializer.cs
- QueryExpr.cs
- GlyphInfoList.cs
- FormsAuthenticationTicket.cs
- ClientApiGenerator.cs
- ObjectToIdCache.cs
- EntityViewContainer.cs
- XmlSchema.cs
- UncommonField.cs
- SafeFileMapViewHandle.cs
- SessionStateSection.cs
- DataServiceRequest.cs
- DetailsView.cs
- FixedSOMContainer.cs
- FloaterParaClient.cs
- UpWmlMobileTextWriter.cs
- IIS7WorkerRequest.cs
- VectorAnimationBase.cs
- RootNamespaceAttribute.cs
- LexicalChunk.cs
- TextRunCache.cs
- TextEffect.cs
- ContextStaticAttribute.cs
- regiisutil.cs
- MatrixAnimationUsingPath.cs
- SR.Designer.cs
- SubpageParaClient.cs
- ModelItemDictionaryImpl.cs
- PreviewPageInfo.cs
- RoutedEvent.cs
- WorkerRequest.cs
- WebOperationContext.cs
- WebFaultClientMessageInspector.cs
- DataFieldConverter.cs
- TypeConverterAttribute.cs
- MaterialGroup.cs
- TextProperties.cs
- SmiContext.cs
- AppSettingsExpressionBuilder.cs
- RoleService.cs
- TextTreeText.cs
- SslStream.cs
- _DisconnectOverlappedAsyncResult.cs
- LinkedList.cs
- ParseChildrenAsPropertiesAttribute.cs
- TransformFinalBlockRequest.cs
- NonParentingControl.cs
- TreeNodeEventArgs.cs
- QilList.cs
- DbReferenceCollection.cs
- ComEventsMethod.cs
- TableRowGroup.cs
- BmpBitmapEncoder.cs
- DbException.cs
- Domain.cs
- ISAPIWorkerRequest.cs
- EditorOptionAttribute.cs
- QilScopedVisitor.cs
- AutomationPatternInfo.cs
- ContainerParaClient.cs
- SecurityUniqueId.cs
- EntityContainerRelationshipSet.cs
- GridViewColumnHeaderAutomationPeer.cs
- WebPartExportVerb.cs
- HostProtectionPermission.cs
- Help.cs
- TypeReference.cs
- UpdateRecord.cs
- CapabilitiesPattern.cs
- TextureBrush.cs
- DataControlLinkButton.cs
- TrackingParticipant.cs
- HierarchicalDataSourceControl.cs
- DataGridItemEventArgs.cs
- NetworkAddressChange.cs
- DSASignatureFormatter.cs
- SoundPlayer.cs
- InkSerializer.cs
- SoapSchemaMember.cs
- EventPropertyMap.cs
- SafeArrayTypeMismatchException.cs
- XmlSchemaAll.cs
- DebugView.cs
- MinMaxParagraphWidth.cs
- DocumentationServerProtocol.cs
- StorageFunctionMapping.cs
- _RegBlobWebProxyDataBuilder.cs
- EditorServiceContext.cs
- AssemblyAttributes.cs
- WrappedIUnknown.cs
- UntypedNullExpression.cs
- StopRoutingHandler.cs