Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / IO / FileLoadException.cs / 1 / FileLoadException.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FileLoadException ** ** ** Purpose: Exception for failure to load a file that was successfully found. ** ** ===========================================================*/ using System; using System.Globalization; using System.Runtime.Serialization; using System.Runtime.CompilerServices; using System.Security.Permissions; using SecurityException = System.Security.SecurityException; namespace System.IO { [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class FileLoadException : IOException { private String _fileName; // the name of the file we could not load. private String _fusionLog; // fusion log (when applicable) public FileLoadException() : base(Environment.GetResourceString("IO.FileLoad")) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message) : base(message) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message, String fileName) : base(message) { SetErrorCode(__HResults.COR_E_FILELOAD); _fileName = fileName; } public FileLoadException(String message, String fileName, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_FILELOAD); _fileName = fileName; } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) _message = FormatFileLoadExceptionMessage(_fileName, HResult); } public String FileName { get { return _fileName; } } public override String ToString() { String s = GetType().FullName + ": " + Message; if (_fileName != null && _fileName.Length != 0) s += Environment.NewLine + String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("IO.FileName_Name"), _fileName); if (InnerException != null) s = s + " ---> " + InnerException.ToString(); if (StackTrace != null) s += Environment.NewLine + StackTrace; try { if(FusionLog!=null) { if (s==null) s=" "; s+=Environment.NewLine; s+=Environment.NewLine; s+=FusionLog; } } catch(SecurityException) { } return s; } protected FileLoadException(SerializationInfo info, StreamingContext context) : base (info, context) { // Base class constructor will check info != null. _fileName = info.GetString("FileLoad_FileName"); try { _fusionLog = info.GetString("FileLoad_FusionLog"); } catch { _fusionLog = null; } } private FileLoadException(String fileName, String fusionLog,int hResult) : base(null) { SetErrorCode(hResult); _fileName = fileName; _fusionLog=fusionLog; SetMessageField(); } public String FusionLog { [SecurityPermissionAttribute( SecurityAction.Demand, Flags = SecurityPermissionFlag.ControlEvidence | SecurityPermissionFlag.ControlPolicy)] get { return _fusionLog; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { // Serialize data for our base classes. base will verify info != null. base.GetObjectData(info, context); // Serialize data for this class info.AddValue("FileLoad_FileName", _fileName, typeof(String)); try { info.AddValue("FileLoad_FusionLog", FusionLog, typeof(String)); } catch (SecurityException) { } } internal static String FormatFileLoadExceptionMessage(String fileName, int hResult) { return String.Format(CultureInfo.CurrentCulture, GetFileLoadExceptionMessage(hResult), fileName, GetMessageForHR(hResult)); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetFileLoadExceptionMessage(int hResult); [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetMessageForHR(int hresult); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FileLoadException ** ** ** Purpose: Exception for failure to load a file that was successfully found. ** ** ===========================================================*/ using System; using System.Globalization; using System.Runtime.Serialization; using System.Runtime.CompilerServices; using System.Security.Permissions; using SecurityException = System.Security.SecurityException; namespace System.IO { [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class FileLoadException : IOException { private String _fileName; // the name of the file we could not load. private String _fusionLog; // fusion log (when applicable) public FileLoadException() : base(Environment.GetResourceString("IO.FileLoad")) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message) : base(message) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_FILELOAD); } public FileLoadException(String message, String fileName) : base(message) { SetErrorCode(__HResults.COR_E_FILELOAD); _fileName = fileName; } public FileLoadException(String message, String fileName, Exception inner) : base(message, inner) { SetErrorCode(__HResults.COR_E_FILELOAD); _fileName = fileName; } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) _message = FormatFileLoadExceptionMessage(_fileName, HResult); } public String FileName { get { return _fileName; } } public override String ToString() { String s = GetType().FullName + ": " + Message; if (_fileName != null && _fileName.Length != 0) s += Environment.NewLine + String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("IO.FileName_Name"), _fileName); if (InnerException != null) s = s + " ---> " + InnerException.ToString(); if (StackTrace != null) s += Environment.NewLine + StackTrace; try { if(FusionLog!=null) { if (s==null) s=" "; s+=Environment.NewLine; s+=Environment.NewLine; s+=FusionLog; } } catch(SecurityException) { } return s; } protected FileLoadException(SerializationInfo info, StreamingContext context) : base (info, context) { // Base class constructor will check info != null. _fileName = info.GetString("FileLoad_FileName"); try { _fusionLog = info.GetString("FileLoad_FusionLog"); } catch { _fusionLog = null; } } private FileLoadException(String fileName, String fusionLog,int hResult) : base(null) { SetErrorCode(hResult); _fileName = fileName; _fusionLog=fusionLog; SetMessageField(); } public String FusionLog { [SecurityPermissionAttribute( SecurityAction.Demand, Flags = SecurityPermissionFlag.ControlEvidence | SecurityPermissionFlag.ControlPolicy)] get { return _fusionLog; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { // Serialize data for our base classes. base will verify info != null. base.GetObjectData(info, context); // Serialize data for this class info.AddValue("FileLoad_FileName", _fileName, typeof(String)); try { info.AddValue("FileLoad_FusionLog", FusionLog, typeof(String)); } catch (SecurityException) { } } internal static String FormatFileLoadExceptionMessage(String fileName, int hResult) { return String.Format(CultureInfo.CurrentCulture, GetFileLoadExceptionMessage(hResult), fileName, GetMessageForHR(hResult)); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetFileLoadExceptionMessage(int hResult); [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern String GetMessageForHR(int hresult); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
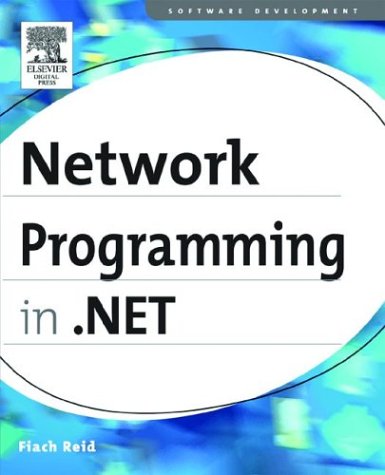
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryNode.cs
- ScopeCollection.cs
- linebase.cs
- PagePropertiesChangingEventArgs.cs
- EventsTab.cs
- BufferedGraphicsContext.cs
- CommentAction.cs
- ViewDesigner.cs
- LoginDesigner.cs
- HtmlInputReset.cs
- EmptyEnumerator.cs
- DbMetaDataFactory.cs
- TraceHandlerErrorFormatter.cs
- ContextBase.cs
- RelationshipEndCollection.cs
- validationstate.cs
- IPPacketInformation.cs
- InputManager.cs
- ParseHttpDate.cs
- CombinedHttpChannel.cs
- SqlDataRecord.cs
- InputElement.cs
- FontClient.cs
- XmlDataLoader.cs
- AppliedDeviceFiltersEditor.cs
- ApplicationServiceManager.cs
- DataListItemEventArgs.cs
- ChangeNode.cs
- ProviderBase.cs
- ResourceContainer.cs
- HandlerFactoryWrapper.cs
- ToolStripSplitButton.cs
- WebDescriptionAttribute.cs
- Underline.cs
- CompiledELinqQueryState.cs
- MeasurementDCInfo.cs
- EmitterCache.cs
- InvalidOperationException.cs
- RtfControlWordInfo.cs
- RSAPKCS1KeyExchangeFormatter.cs
- Control.cs
- DictionarySectionHandler.cs
- MasterPageParser.cs
- C14NUtil.cs
- DbConnectionClosed.cs
- ApplicationServiceHelper.cs
- NetworkInterface.cs
- ConfigurationValues.cs
- ButtonField.cs
- X509Chain.cs
- SystemInfo.cs
- DurableInstancingOptions.cs
- Rotation3DAnimationBase.cs
- safemediahandle.cs
- SplineQuaternionKeyFrame.cs
- FileDialogCustomPlace.cs
- GregorianCalendar.cs
- PrefixHandle.cs
- DictionaryChange.cs
- RewritingSimplifier.cs
- Switch.cs
- HashSet.cs
- EventLogStatus.cs
- ByteAnimation.cs
- Timeline.cs
- datacache.cs
- DynamicValidatorEventArgs.cs
- EditBehavior.cs
- NamespaceEmitter.cs
- Bits.cs
- WindowsGraphicsCacheManager.cs
- DisplayMemberTemplateSelector.cs
- BindingListCollectionView.cs
- VectorCollection.cs
- RuntimeConfig.cs
- SlipBehavior.cs
- MonthCalendar.cs
- SelectionPatternIdentifiers.cs
- AddInPipelineAttributes.cs
- HtmlToClrEventProxy.cs
- TextEndOfLine.cs
- AccessDataSourceDesigner.cs
- ProtectedProviderSettings.cs
- QuaternionRotation3D.cs
- DropDownButton.cs
- AmbientEnvironment.cs
- ToolStripScrollButton.cs
- NavigationProperty.cs
- GroupLabel.cs
- WebGetAttribute.cs
- PageSetupDialog.cs
- AlternateViewCollection.cs
- HighlightComponent.cs
- BindingList.cs
- PlaceHolder.cs
- DashStyles.cs
- RijndaelCryptoServiceProvider.cs
- ByeOperation11AsyncResult.cs
- RightNameExpirationInfoPair.cs
- UnsafeNativeMethods.cs