Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / NetworkInformation / NetworkInterface.cs / 1 / NetworkInterface.cs
using System; namespace System.Net.NetworkInformation { public abstract class NetworkInterface { /// Returns objects that describe the network interfaces on the local computer. public static NetworkInterface[] GetAllNetworkInterfaces(){ (new NetworkInformationPermission(NetworkInformationAccess.Read)).Demand(); return SystemNetworkInterface.GetNetworkInterfaces(); } public static bool GetIsNetworkAvailable(){ return SystemNetworkInterface.InternalGetIsNetworkAvailable(); } public static int LoopbackInterfaceIndex{ get{ return SystemNetworkInterface.InternalLoopbackInterfaceIndex; } } public abstract string Id{get;} /// Gets the name of the network interface. public abstract string Name{get;} /// Gets the description of the network interface public abstract string Description{get;} /// Gets the IP properties for this network interface. public abstract IPInterfaceProperties GetIPProperties(); /// Provides Internet Protocol (IP) statistical data for thisnetwork interface. public abstract IPv4InterfaceStatistics GetIPv4Statistics(); /// Gets the current operational state of the network connection. public abstract OperationalStatus OperationalStatus{get;} /// Gets the speed of the interface in bits per second as reported by the interface. public abstract long Speed{get;} /// Gets a bool value that indicates whether the network interface is set to only receive data packets. public abstract bool IsReceiveOnly{get;} /// Gets a bool value that indicates whether this network interface is enabled to receive multicast packets. public abstract bool SupportsMulticast{get;} /// Gets the physical address of this network interface /// deonb. This is okay if you don't support this in Whidbey. This actually belongs in the NetworkAdapter derived class public abstract PhysicalAddress GetPhysicalAddress(); /// Gets the interface type. public abstract NetworkInterfaceType NetworkInterfaceType{get;} public abstract bool Supports(NetworkInterfaceComponent networkInterfaceComponent); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Net.NetworkInformation { public abstract class NetworkInterface { /// Returns objects that describe the network interfaces on the local computer. public static NetworkInterface[] GetAllNetworkInterfaces(){ (new NetworkInformationPermission(NetworkInformationAccess.Read)).Demand(); return SystemNetworkInterface.GetNetworkInterfaces(); } public static bool GetIsNetworkAvailable(){ return SystemNetworkInterface.InternalGetIsNetworkAvailable(); } public static int LoopbackInterfaceIndex{ get{ return SystemNetworkInterface.InternalLoopbackInterfaceIndex; } } public abstract string Id{get;} /// Gets the name of the network interface. public abstract string Name{get;} /// Gets the description of the network interface public abstract string Description{get;} /// Gets the IP properties for this network interface. public abstract IPInterfaceProperties GetIPProperties(); /// Provides Internet Protocol (IP) statistical data for thisnetwork interface. public abstract IPv4InterfaceStatistics GetIPv4Statistics(); /// Gets the current operational state of the network connection. public abstract OperationalStatus OperationalStatus{get;} /// Gets the speed of the interface in bits per second as reported by the interface. public abstract long Speed{get;} /// Gets a bool value that indicates whether the network interface is set to only receive data packets. public abstract bool IsReceiveOnly{get;} /// Gets a bool value that indicates whether this network interface is enabled to receive multicast packets. public abstract bool SupportsMulticast{get;} /// Gets the physical address of this network interface /// deonb. This is okay if you don't support this in Whidbey. This actually belongs in the NetworkAdapter derived class public abstract PhysicalAddress GetPhysicalAddress(); /// Gets the interface type. public abstract NetworkInterfaceType NetworkInterfaceType{get;} public abstract bool Supports(NetworkInterfaceComponent networkInterfaceComponent); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
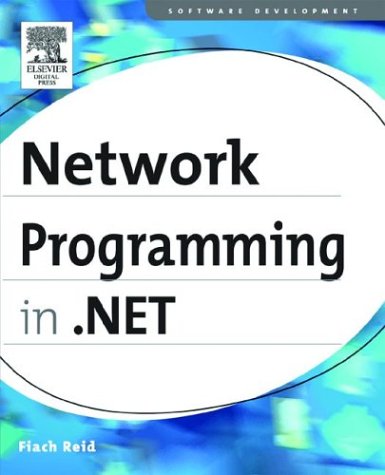
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestTimeoutManager.cs
- DataGridViewRowStateChangedEventArgs.cs
- XmlWhitespace.cs
- XmlDataLoader.cs
- SiteMapHierarchicalDataSourceView.cs
- ListViewUpdateEventArgs.cs
- TextParagraphProperties.cs
- EdmRelationshipRoleAttribute.cs
- ButtonField.cs
- HTMLTextWriter.cs
- FunctionQuery.cs
- Memoizer.cs
- ConstraintEnumerator.cs
- ContextProperty.cs
- DecoderFallback.cs
- ConnectionPoint.cs
- wgx_commands.cs
- ShaderEffect.cs
- MdbDataFileEditor.cs
- CssTextWriter.cs
- XmlDigitalSignatureProcessor.cs
- CodeStatementCollection.cs
- PolicyLevel.cs
- HorizontalAlignConverter.cs
- LinqDataSourceUpdateEventArgs.cs
- GridViewCancelEditEventArgs.cs
- ExeContext.cs
- DataGridCellClipboardEventArgs.cs
- JapaneseCalendar.cs
- PeerCollaboration.cs
- MILUtilities.cs
- SoapIgnoreAttribute.cs
- InternalCache.cs
- ContextMenuService.cs
- CoreChannel.cs
- Hyperlink.cs
- UIntPtr.cs
- XmlMapping.cs
- TextElementCollectionHelper.cs
- HtmlInputButton.cs
- XamlBrushSerializer.cs
- RelationshipWrapper.cs
- FormViewUpdateEventArgs.cs
- InstanceOwnerQueryResult.cs
- AttributeCollection.cs
- Int64AnimationUsingKeyFrames.cs
- NonSerializedAttribute.cs
- EntityDataSourceContextDisposingEventArgs.cs
- CodePageUtils.cs
- ToolStripItemDataObject.cs
- NameTable.cs
- RequestQueryParser.cs
- PriorityRange.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- LassoSelectionBehavior.cs
- DependencyPropertyConverter.cs
- ReadOnlyNameValueCollection.cs
- Proxy.cs
- MethodCallExpression.cs
- HtmlTextBoxAdapter.cs
- ProfileManager.cs
- List.cs
- SmtpTransport.cs
- FormattedText.cs
- altserialization.cs
- SqlFunctionAttribute.cs
- SelectorItemAutomationPeer.cs
- ClassImporter.cs
- BrowserCapabilitiesFactory.cs
- Transform3DGroup.cs
- TrackingRecord.cs
- BinaryMethodMessage.cs
- OperandQuery.cs
- HttpWebRequest.cs
- DeclarativeCatalogPart.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- SByteStorage.cs
- PropagatorResult.cs
- OperationParameterInfo.cs
- MembershipValidatePasswordEventArgs.cs
- SingletonInstanceContextProvider.cs
- ProfessionalColorTable.cs
- GridViewColumnCollectionChangedEventArgs.cs
- XamlSerializationHelper.cs
- BamlLocalizabilityResolver.cs
- QueryStringParameter.cs
- OracleString.cs
- GridToolTip.cs
- QueryStringParameter.cs
- SID.cs
- RichTextBox.cs
- TextFormatter.cs
- QueryContinueDragEventArgs.cs
- FontFamilyConverter.cs
- RenderData.cs
- SqlBulkCopy.cs
- BasePropertyDescriptor.cs
- StreamWithDictionary.cs
- KeyConstraint.cs
- ToolConsole.cs