Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / MS / Internal / IO / Packaging / CompoundFile / StreamWithDictionary.cs / 1 / StreamWithDictionary.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The object for wrapping a data stream and its associated context // information dictionary. // // History: // 07/05/2002: [....]: Initial implementation. // 05/20/2003: [....]: Ported to WCP tree. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.IO; namespace MS.Internal.IO.Packaging.CompoundFile { internal class StreamWithDictionary : Stream, IDictionary { Stream baseStream; IDictionary baseDictionary; private bool _disposed; // keep track of if we are disposed internal StreamWithDictionary( Stream wrappedStream, IDictionary wrappedDictionary ) { baseStream = wrappedStream; baseDictionary = wrappedDictionary; } /*************************************************************************/ // Stream members public override bool CanRead { get{ return !_disposed && baseStream.CanRead; }} public override bool CanSeek { get { return !_disposed && baseStream.CanSeek; } } public override bool CanWrite { get { return !_disposed && baseStream.CanWrite; } } public override long Length { get { CheckDisposed(); return baseStream.Length; } } public override long Position { get { CheckDisposed(); return baseStream.Position; } set { CheckDisposed(); baseStream.Position = value; } } public override void Flush() { CheckDisposed(); baseStream.Flush(); } public override long Seek( long offset, SeekOrigin origin ) { CheckDisposed(); return baseStream.Seek(offset, origin); } public override void SetLength( long newLength ) { CheckDisposed(); baseStream.SetLength(newLength); } public override int Read( byte[] buffer, int offset, int count ) { CheckDisposed(); return baseStream.Read(buffer, offset, count); } public override void Write( byte[] buffer, int offset, int count ) { CheckDisposed(); baseStream.Write(buffer, offset, count); } //----------------------------------------------------- // // Protected Methods // //----------------------------------------------------- ////// Dispose(bool) /// /// protected override void Dispose(bool disposing) { try { if (disposing && !_disposed) { _disposed = true; baseStream.Close(); } } finally { base.Dispose(disposing); } } /*************************************************************************/ // IDictionary members bool IDictionary.Contains( object key ) { CheckDisposed(); return baseDictionary.Contains(key); } void IDictionary.Add( object key, object val ) { CheckDisposed(); baseDictionary.Add(key, val); } void IDictionary.Clear() { CheckDisposed(); baseDictionary.Clear(); } IDictionaryEnumerator IDictionary.GetEnumerator() { CheckDisposed(); // IDictionary.GetEnumerator vs. IEnumerable.GetEnumerator? return ((IDictionary)baseDictionary).GetEnumerator(); } void IDictionary.Remove( object key ) { CheckDisposed(); baseDictionary.Remove(key); } object IDictionary.this[ object index ] { get { CheckDisposed(); return baseDictionary[index]; } set { CheckDisposed(); baseDictionary[index] = value; } } ICollection IDictionary.Keys { get { CheckDisposed(); return baseDictionary.Keys; } } ICollection IDictionary.Values { get { CheckDisposed(); return baseDictionary.Values; } } bool IDictionary.IsReadOnly { get { CheckDisposed(); return baseDictionary.IsReadOnly; } } bool IDictionary.IsFixedSize { get { CheckDisposed(); return baseDictionary.IsFixedSize; } } /*************************************************************************/ // ICollection methods void ICollection.CopyTo( Array array, int index ) { CheckDisposed(); ((ICollection)baseDictionary).CopyTo(array, index); } int ICollection.Count { get { CheckDisposed(); return ((ICollection)baseDictionary).Count; } } object ICollection.SyncRoot { get { CheckDisposed(); return ((ICollection)baseDictionary).SyncRoot; } } bool ICollection.IsSynchronized { get { CheckDisposed(); return ((ICollection)baseDictionary).IsSynchronized; } } /*************************************************************************/ // IEnumerable method IEnumerator IEnumerable.GetEnumerator() { CheckDisposed(); return ((IEnumerable)baseDictionary).GetEnumerator(); } ////// Disposed - were we disposed? Offer this to DataSpaceManager so it can do smart flushing /// ///internal bool Disposed { get { return _disposed; } } //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- private void CheckDisposed() { if (_disposed) throw new ObjectDisposedException("Stream"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
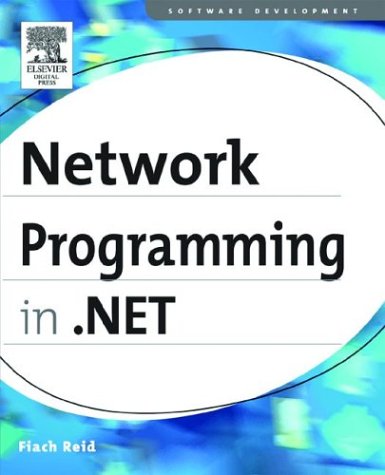
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LicenseException.cs
- MetadataProperty.cs
- ConfigurationLoaderException.cs
- ToolStripDesignerAvailabilityAttribute.cs
- MenuCommand.cs
- ServiceMetadataPublishingElement.cs
- XPathMessageFilter.cs
- DataGridTemplateColumn.cs
- TreeViewCancelEvent.cs
- ConfigXmlText.cs
- xsdvalidator.cs
- SspiHelper.cs
- PrivacyNoticeBindingElement.cs
- WindowsSlider.cs
- ConsoleKeyInfo.cs
- MiniConstructorInfo.cs
- FlowDocumentFormatter.cs
- HttpHandlerActionCollection.cs
- MouseGesture.cs
- Context.cs
- QueryComponents.cs
- ItemList.cs
- Visitors.cs
- XmlElementCollection.cs
- DataBindingHandlerAttribute.cs
- TextTreeUndo.cs
- DrawingCollection.cs
- PhoneCall.cs
- ContainerParaClient.cs
- ScrollData.cs
- PolyBezierSegment.cs
- UriExt.cs
- TypeExtensionSerializer.cs
- TextBoxAutoCompleteSourceConverter.cs
- PropertyPathConverter.cs
- BaseCollection.cs
- XmlEntityReference.cs
- LicenseProviderAttribute.cs
- XmlQueryCardinality.cs
- Int32Collection.cs
- ContainsRowNumberChecker.cs
- SingleKeyFrameCollection.cs
- SqlMetaData.cs
- SchemaImporterExtension.cs
- SecurityUtils.cs
- AstNode.cs
- XmlSchemaAttributeGroupRef.cs
- CompilationUnit.cs
- _TLSstream.cs
- WebPartManager.cs
- Transform.cs
- DataGridRowDetailsEventArgs.cs
- PropertyDescriptor.cs
- CorrelationManager.cs
- RadialGradientBrush.cs
- HMACRIPEMD160.cs
- XmlHierarchicalDataSourceView.cs
- FlowDocumentPage.cs
- SimpleApplicationHost.cs
- EventDescriptorCollection.cs
- UDPClient.cs
- TextContainerHelper.cs
- Transform.cs
- ImageMapEventArgs.cs
- EventSourceCreationData.cs
- MsmqBindingMonitor.cs
- HttpInputStream.cs
- WmlPageAdapter.cs
- GridItemCollection.cs
- DataTablePropertyDescriptor.cs
- SourceFileInfo.cs
- ListViewDeletedEventArgs.cs
- EventLogPermissionEntryCollection.cs
- Rect3DValueSerializer.cs
- UIPropertyMetadata.cs
- ContentPlaceHolder.cs
- HtmlWindow.cs
- TextBlock.cs
- ScriptReference.cs
- DataGridHeadersVisibilityToVisibilityConverter.cs
- Mutex.cs
- XPathChildIterator.cs
- listitem.cs
- CommandHelpers.cs
- KeyValueSerializer.cs
- ExecutedRoutedEventArgs.cs
- PeerTransportBindingElement.cs
- ChangesetResponse.cs
- FixedSOMElement.cs
- WindowsFormsHost.cs
- DataGridViewSortCompareEventArgs.cs
- Delegate.cs
- ValidationSummary.cs
- Effect.cs
- MouseCaptureWithinProperty.cs
- DataGridDetailsPresenter.cs
- InputBindingCollection.cs
- FlowPanelDesigner.cs
- ConnectionOrientedTransportChannelFactory.cs
- NativeActivityTransactionContext.cs