Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Web / System / Web / Services / Protocols / ServerProtocol.cs / 1305376 / ServerProtocol.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services.Protocols { using System; using System.Diagnostics; using System.Collections; using System.IO; using System.Reflection; using System.Xml.Serialization; using System.Web.Services.Description; using System.Web.Caching; using System.ComponentModel; using System.Text; using System.Net; using System.Web.Services; using System.Web.Hosting; using System.Threading; using System.Security.Permissions; using System.Web.Services.Diagnostics; [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] public abstract class ServerProtocol { Type type; HttpRequest request; HttpResponse response; HttpContext context; object target; WebMethodAttribute methodAttr; private static Object s_InternalSyncObject; internal static Object InternalSyncObject { get { if (s_InternalSyncObject == null) { Object o = new Object(); Interlocked.CompareExchange(ref s_InternalSyncObject, o, null); } return s_InternalSyncObject; } } internal void SetContext(Type type, HttpContext context, HttpRequest request, HttpResponse response) { this.type = type; this.context = context; this.request = request; this.response = response; Initialize(); } internal virtual void CreateServerInstance() { target = Activator.CreateInstance(ServerType.Type); WebService service = target as WebService; if (service != null) service.SetContext(context); } internal virtual void DisposeServerInstance() { if (target == null) return; IDisposable disposable = target as IDisposable; if (disposable != null) disposable.Dispose(); target = null; } protected internal HttpContext Context { get { return context; } } protected internal HttpRequest Request { get { return request; } } protected internal HttpResponse Response { get { return response; } } internal Type Type { get { return type; } } protected virtual internal object Target { get { return target; } } internal virtual bool WriteException(Exception e, Stream outputStream) { // return true if exception should not be re-thrown to ASP.NET return false; } internal abstract bool Initialize(); internal abstract object[] ReadParameters(); internal abstract void WriteReturns(object[] returns, Stream outputStream); internal abstract LogicalMethodInfo MethodInfo { get;} internal abstract ServerType ServerType { get;} internal abstract bool IsOneWay { get;} internal virtual Exception OnewayInitException { get {return null;}} internal WebMethodAttribute MethodAttribute { get { if (methodAttr == null) methodAttr = MethodInfo.MethodAttribute; return methodAttr; } } internal string GenerateFaultString(Exception e) { return GenerateFaultString(e, false); } internal static void SetHttpResponseStatusCode(HttpResponse httpResponse, int statusCode) { // We skip IIS custom errors for HTTP requests. httpResponse.TrySkipIisCustomErrors = true; httpResponse.StatusCode = statusCode; } // internal string GenerateFaultString(Exception e, bool htmlEscapeMessage) { bool isDevelopmentServer = Context != null && !Context.IsCustomErrorEnabled; if (isDevelopmentServer && !htmlEscapeMessage) { //If the user has specified it's a development server (versus a production server) in ASP.NET config, //then we should just return e.ToString instead of extracting the list of messages. return e.ToString(); } StringBuilder builder = new StringBuilder(); if (isDevelopmentServer) { // we are dumping the ecseption directly to IE, need to encode GenerateFaultString(e, builder); } else { for (Exception inner = e; inner != null; inner = inner.InnerException) { string text = htmlEscapeMessage ? HttpUtility.HtmlEncode(inner.Message) : inner.Message; if (text.Length == 0) text = e.GetType().Name; builder.Append(text); if (inner.InnerException != null) builder.Append(" ---> "); } } return builder.ToString(); } static void GenerateFaultString(Exception e, StringBuilder builder) { builder.Append(e.GetType().FullName); if (e.Message != null && e.Message.Length > 0) { builder.Append(": "); builder.Append(HttpUtility.HtmlEncode(e.Message)); } if (e.InnerException != null) { builder.Append(" ---> "); GenerateFaultString(e.InnerException, builder); builder.Append(Environment.NewLine); builder.Append(" "); builder.Append(Res.GetString(Res.StackTraceEnd)); } if (e.StackTrace != null) { builder.Append(Environment.NewLine); builder.Append(e.StackTrace); } } internal void WriteOneWayResponse() { context.Response.ContentType = null; Response.StatusCode = (int) HttpStatusCode.Accepted; } string CreateKey(Type protocolType, Type serverType) { // // we want to use the hostname to cache since for documentation, WSDL // contains the cache hostname, but we definitely don't want to cache the query string! // string protocolTypeName = protocolType.FullName; string serverTypeName = serverType.FullName; string typeHandleString = serverType.TypeHandle.Value.ToString(); string url = Request.Url.GetLeftPart(UriPartial.Path); int length = protocolTypeName.Length + url.Length + serverTypeName.Length + typeHandleString.Length; StringBuilder sb = new StringBuilder(length); sb.Append(protocolTypeName); sb.Append(url); sb.Append(serverTypeName); sb.Append(typeHandleString); return sb.ToString(); } protected void AddToCache(Type protocolType, Type serverType, object value) { HttpRuntime.Cache.Insert(CreateKey(protocolType, serverType), value, null, Cache.NoAbsoluteExpiration, Cache.NoSlidingExpiration, CacheItemPriority.NotRemovable, null); } protected object GetFromCache(Type protocolType, Type serverType) { return HttpRuntime.Cache.Get(CreateKey(protocolType, serverType)); } } [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] public abstract class ServerProtocolFactory { internal ServerProtocol Create(Type type, HttpContext context, HttpRequest request, HttpResponse response, out bool abortProcessing) { ServerProtocol serverProtocol = null; abortProcessing = false; serverProtocol = CreateIfRequestCompatible(request); try { if (serverProtocol!=null) serverProtocol.SetContext(type, context, request, response); return serverProtocol; } catch (Exception e) { abortProcessing = true; if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (Tracing.On) Tracing.ExceptionCatch(TraceEventType.Warning, this, "Create", e); if (serverProtocol != null) { // give the protocol a shot at handling the error in a custom way if (!serverProtocol.WriteException(e, serverProtocol.Response.OutputStream)) throw new InvalidOperationException(Res.GetString(Res.UnableToHandleRequest0), e); } return null; } } protected abstract ServerProtocol CreateIfRequestCompatible(HttpRequest request); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services.Protocols { using System; using System.Diagnostics; using System.Collections; using System.IO; using System.Reflection; using System.Xml.Serialization; using System.Web.Services.Description; using System.Web.Caching; using System.ComponentModel; using System.Text; using System.Net; using System.Web.Services; using System.Web.Hosting; using System.Threading; using System.Security.Permissions; using System.Web.Services.Diagnostics; [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] public abstract class ServerProtocol { Type type; HttpRequest request; HttpResponse response; HttpContext context; object target; WebMethodAttribute methodAttr; private static Object s_InternalSyncObject; internal static Object InternalSyncObject { get { if (s_InternalSyncObject == null) { Object o = new Object(); Interlocked.CompareExchange(ref s_InternalSyncObject, o, null); } return s_InternalSyncObject; } } internal void SetContext(Type type, HttpContext context, HttpRequest request, HttpResponse response) { this.type = type; this.context = context; this.request = request; this.response = response; Initialize(); } internal virtual void CreateServerInstance() { target = Activator.CreateInstance(ServerType.Type); WebService service = target as WebService; if (service != null) service.SetContext(context); } internal virtual void DisposeServerInstance() { if (target == null) return; IDisposable disposable = target as IDisposable; if (disposable != null) disposable.Dispose(); target = null; } protected internal HttpContext Context { get { return context; } } protected internal HttpRequest Request { get { return request; } } protected internal HttpResponse Response { get { return response; } } internal Type Type { get { return type; } } protected virtual internal object Target { get { return target; } } internal virtual bool WriteException(Exception e, Stream outputStream) { // return true if exception should not be re-thrown to ASP.NET return false; } internal abstract bool Initialize(); internal abstract object[] ReadParameters(); internal abstract void WriteReturns(object[] returns, Stream outputStream); internal abstract LogicalMethodInfo MethodInfo { get;} internal abstract ServerType ServerType { get;} internal abstract bool IsOneWay { get;} internal virtual Exception OnewayInitException { get {return null;}} internal WebMethodAttribute MethodAttribute { get { if (methodAttr == null) methodAttr = MethodInfo.MethodAttribute; return methodAttr; } } internal string GenerateFaultString(Exception e) { return GenerateFaultString(e, false); } internal static void SetHttpResponseStatusCode(HttpResponse httpResponse, int statusCode) { // We skip IIS custom errors for HTTP requests. httpResponse.TrySkipIisCustomErrors = true; httpResponse.StatusCode = statusCode; } // internal string GenerateFaultString(Exception e, bool htmlEscapeMessage) { bool isDevelopmentServer = Context != null && !Context.IsCustomErrorEnabled; if (isDevelopmentServer && !htmlEscapeMessage) { //If the user has specified it's a development server (versus a production server) in ASP.NET config, //then we should just return e.ToString instead of extracting the list of messages. return e.ToString(); } StringBuilder builder = new StringBuilder(); if (isDevelopmentServer) { // we are dumping the ecseption directly to IE, need to encode GenerateFaultString(e, builder); } else { for (Exception inner = e; inner != null; inner = inner.InnerException) { string text = htmlEscapeMessage ? HttpUtility.HtmlEncode(inner.Message) : inner.Message; if (text.Length == 0) text = e.GetType().Name; builder.Append(text); if (inner.InnerException != null) builder.Append(" ---> "); } } return builder.ToString(); } static void GenerateFaultString(Exception e, StringBuilder builder) { builder.Append(e.GetType().FullName); if (e.Message != null && e.Message.Length > 0) { builder.Append(": "); builder.Append(HttpUtility.HtmlEncode(e.Message)); } if (e.InnerException != null) { builder.Append(" ---> "); GenerateFaultString(e.InnerException, builder); builder.Append(Environment.NewLine); builder.Append(" "); builder.Append(Res.GetString(Res.StackTraceEnd)); } if (e.StackTrace != null) { builder.Append(Environment.NewLine); builder.Append(e.StackTrace); } } internal void WriteOneWayResponse() { context.Response.ContentType = null; Response.StatusCode = (int) HttpStatusCode.Accepted; } string CreateKey(Type protocolType, Type serverType) { // // we want to use the hostname to cache since for documentation, WSDL // contains the cache hostname, but we definitely don't want to cache the query string! // string protocolTypeName = protocolType.FullName; string serverTypeName = serverType.FullName; string typeHandleString = serverType.TypeHandle.Value.ToString(); string url = Request.Url.GetLeftPart(UriPartial.Path); int length = protocolTypeName.Length + url.Length + serverTypeName.Length + typeHandleString.Length; StringBuilder sb = new StringBuilder(length); sb.Append(protocolTypeName); sb.Append(url); sb.Append(serverTypeName); sb.Append(typeHandleString); return sb.ToString(); } protected void AddToCache(Type protocolType, Type serverType, object value) { HttpRuntime.Cache.Insert(CreateKey(protocolType, serverType), value, null, Cache.NoAbsoluteExpiration, Cache.NoSlidingExpiration, CacheItemPriority.NotRemovable, null); } protected object GetFromCache(Type protocolType, Type serverType) { return HttpRuntime.Cache.Get(CreateKey(protocolType, serverType)); } } [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] public abstract class ServerProtocolFactory { internal ServerProtocol Create(Type type, HttpContext context, HttpRequest request, HttpResponse response, out bool abortProcessing) { ServerProtocol serverProtocol = null; abortProcessing = false; serverProtocol = CreateIfRequestCompatible(request); try { if (serverProtocol!=null) serverProtocol.SetContext(type, context, request, response); return serverProtocol; } catch (Exception e) { abortProcessing = true; if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (Tracing.On) Tracing.ExceptionCatch(TraceEventType.Warning, this, "Create", e); if (serverProtocol != null) { // give the protocol a shot at handling the error in a custom way if (!serverProtocol.WriteException(e, serverProtocol.Response.OutputStream)) throw new InvalidOperationException(Res.GetString(Res.UnableToHandleRequest0), e); } return null; } } protected abstract ServerProtocol CreateIfRequestCompatible(HttpRequest request); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
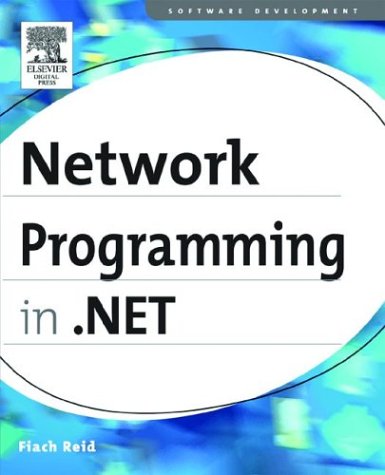
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostingPreferredMapPath.cs
- IndexingContentUnit.cs
- DataControlFieldCell.cs
- CompressStream.cs
- SemanticAnalyzer.cs
- CodeSubDirectoriesCollection.cs
- TagPrefixInfo.cs
- SecurityKeyUsage.cs
- AssemblyResourceLoader.cs
- Interlocked.cs
- AmbientLight.cs
- EncryptedXml.cs
- ManagedIStream.cs
- sqlnorm.cs
- SmtpReplyReaderFactory.cs
- DesigntimeLicenseContextSerializer.cs
- Error.cs
- CollectionConverter.cs
- ColumnMap.cs
- QueryAsyncResult.cs
- XmlSerializerSection.cs
- ChooseAction.cs
- ErrorEventArgs.cs
- ClientScriptManager.cs
- ClientSettings.cs
- AsnEncodedData.cs
- FrameworkRichTextComposition.cs
- _SecureChannel.cs
- ViewCellRelation.cs
- SQLInt64Storage.cs
- DataControlHelper.cs
- HtmlInputPassword.cs
- TypedTableBaseExtensions.cs
- XmlSerializerSection.cs
- UrlParameterWriter.cs
- XDeferredAxisSource.cs
- DataGridRowEventArgs.cs
- UnaryNode.cs
- OuterProxyWrapper.cs
- SqlBooleanMismatchVisitor.cs
- MissingFieldException.cs
- SiteMapProvider.cs
- CodeGen.cs
- Track.cs
- ElapsedEventArgs.cs
- HostingEnvironmentException.cs
- CompoundFileDeflateTransform.cs
- AnnotationMap.cs
- MdiWindowListItemConverter.cs
- DbgUtil.cs
- precedingsibling.cs
- FixedFindEngine.cs
- X509Certificate2Collection.cs
- MappingMetadataHelper.cs
- ElementNotEnabledException.cs
- DataGridViewTopRowAccessibleObject.cs
- Enlistment.cs
- ProgressBarAutomationPeer.cs
- GetChildSubtree.cs
- EventsTab.cs
- PositiveTimeSpanValidator.cs
- SecurityManager.cs
- TimeoutException.cs
- ImageMapEventArgs.cs
- HttpCookiesSection.cs
- ClientRuntimeConfig.cs
- ResXResourceWriter.cs
- ImageMetadata.cs
- COM2AboutBoxPropertyDescriptor.cs
- CompensateDesigner.cs
- Lease.cs
- TextHidden.cs
- WindowsSysHeader.cs
- JsonEncodingStreamWrapper.cs
- DesignOnlyAttribute.cs
- QueryableDataSourceView.cs
- ReflectPropertyDescriptor.cs
- DbModificationCommandTree.cs
- sqlstateclientmanager.cs
- BufferedGraphicsContext.cs
- RuleSettingsCollection.cs
- FixedSOMTableRow.cs
- PersonalizationState.cs
- RawAppCommandInputReport.cs
- IUnknownConstantAttribute.cs
- UITypeEditor.cs
- Model3DGroup.cs
- ScrollItemProviderWrapper.cs
- WebScriptEnablingBehavior.cs
- LicenseException.cs
- _LoggingObject.cs
- ScriptingJsonSerializationSection.cs
- SystemFonts.cs
- PropertyKey.cs
- FileLevelControlBuilderAttribute.cs
- Visual3DCollection.cs
- JsonStringDataContract.cs
- MemoryMappedView.cs
- GradientStop.cs
- PageAdapter.cs