Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / OuterProxyWrapper.cs / 1 / OuterProxyWrapper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Reflection; using System.Collections.Generic; using System.Threading; using System.ServiceModel.Channels; internal class ProxySupportWrapper { internal delegate int DelegateDllGetClassObject([In, MarshalAs(UnmanagedType.LPStruct)] Guid clsid, [In, MarshalAs(UnmanagedType.LPStruct)] Guid iid, ref IClassFactory ppv); const string fileName = @"ServiceMonikerSupport.dll"; const string functionName = @"DllGetClassObject"; static readonly Guid ClsidProxyInstanceProvider = new Guid("(BF0514FB-6912-4659-AD69-B727E5B7ADD4)"); SafeLibraryHandle monikerSupportLibrary; DelegateDllGetClassObject getCODelegate; internal ProxySupportWrapper() { monikerSupportLibrary = null; getCODelegate = null; } ~ProxySupportWrapper() { if (null != monikerSupportLibrary) { monikerSupportLibrary.Close(); monikerSupportLibrary = null; } } internal IProxyProvider GetProxyProvider() { if (null == monikerSupportLibrary) { lock (this) { if (null == monikerSupportLibrary) { getCODelegate = null; using (RegistryHandle regKey = RegistryHandle.GetCorrectBitnessHKLMSubkey((IntPtr.Size == 8), ServiceModelInstallStrings.WcfRegistryKey)) { string file = regKey.GetStringValue(ServiceModelInstallStrings.RuntimeInstallPathName).TrimEnd('\0') + "\\" + fileName; #pragma warning suppress 56523 // monikerSupportLibrary = UnsafeNativeMethods.LoadLibrary(file); if (monikerSupportLibrary.IsInvalid) { monikerSupportLibrary.SetHandleAsInvalid(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ServiceMonikerSupportLoadFailed(file)); } } } } } if (null == getCODelegate) { lock (this) { if (null == getCODelegate) { try { IntPtr procaddr = UnsafeNativeMethods.GetProcAddress(monikerSupportLibrary, functionName); getCODelegate = (DelegateDllGetClassObject)Marshal.GetDelegateForFunctionPointer(procaddr, typeof(DelegateDllGetClassObject)); } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ComPlusProxyProviderException(SR.GetString(SR.FailedProxyProviderCreation), e)); } } } } IClassFactory cf = null; IProxyProvider proxyProvider = null; try { getCODelegate(ClsidProxyInstanceProvider, typeof(IClassFactory).GUID, ref cf); proxyProvider = cf.CreateInstance(null, typeof(IProxyProvider).GUID) as IProxyProvider; Thread.MemoryBarrier(); } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ComPlusProxyProviderException(SR.GetString(SR.FailedProxyProviderCreation), e)); } finally { if (null != cf) { Marshal.ReleaseComObject(cf); cf = null; } } return proxyProvider; } } internal static class OuterProxyWrapper { static ProxySupportWrapper proxySupport = new ProxySupportWrapper(); public static IntPtr CreateOuterProxyInstance (IProxyManager proxyManager, ref Guid riid) { IntPtr pOuter = IntPtr.Zero; IProxyProvider proxyProvider = proxySupport.GetProxyProvider(); if (proxyProvider == null) { DiagnosticUtility.DebugAssert("Proxy Provider cannot be NULL"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(true); } Guid riid2 = riid; int hr = proxyProvider.CreateOuterProxyInstance ( proxyManager, ref riid2, out pOuter); Marshal.ReleaseComObject(proxyProvider); if (hr != HR.S_OK) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new COMException (SR.GetString (SR.FailedProxyProviderCreation), hr)); return pOuter; } public static IntPtr CreateDispatchProxy (IntPtr pOuter, IPseudoDispatch proxy) { IntPtr pInner = IntPtr.Zero; IProxyProvider proxyProvider = proxySupport.GetProxyProvider(); if (proxyProvider == null) { DiagnosticUtility.DebugAssert("Proxy Provider cannot be NULL"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(true); } int hr = proxyProvider.CreateDispatchProxyInstance ( pOuter, proxy, out pInner); Marshal.ReleaseComObject(proxyProvider); if (hr != HR.S_OK) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new COMException (SR.GetString (SR.FailedProxyProviderCreation), hr)); return pInner; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
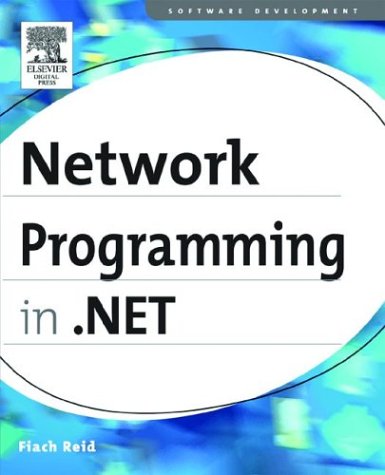
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTrigger.cs
- DataGridViewRowHeaderCell.cs
- DynamicValueConverter.cs
- Console.cs
- ElapsedEventArgs.cs
- BindingMAnagerBase.cs
- InputReportEventArgs.cs
- Pair.cs
- InterleavedZipPartStream.cs
- ReflectPropertyDescriptor.cs
- WmlFormAdapter.cs
- SchemaRegistration.cs
- EditingCoordinator.cs
- DataPagerFieldCollection.cs
- TreeNodeCollectionEditorDialog.cs
- ImportContext.cs
- LineSegment.cs
- CounterCreationDataCollection.cs
- DoubleCollectionConverter.cs
- AppDomainManager.cs
- httpapplicationstate.cs
- DataControlReferenceCollection.cs
- PingOptions.cs
- DeploymentSection.cs
- indexingfiltermarshaler.cs
- ImageDrawing.cs
- BaseDataBoundControlDesigner.cs
- ResourceSet.cs
- XamlInt32CollectionSerializer.cs
- UnsafeNativeMethods.cs
- TextSelectionProcessor.cs
- WebSysDescriptionAttribute.cs
- EncoderExceptionFallback.cs
- DBSqlParserTableCollection.cs
- BitmapCacheBrush.cs
- TableCellAutomationPeer.cs
- TimeSpanValidatorAttribute.cs
- ObjectAnimationUsingKeyFrames.cs
- Update.cs
- FormViewUpdatedEventArgs.cs
- ByteBufferPool.cs
- OdbcDataAdapter.cs
- SiteMapDataSourceView.cs
- ChineseLunisolarCalendar.cs
- followingquery.cs
- XmlBinaryReader.cs
- DataGridItem.cs
- DesignerDataTableBase.cs
- coordinator.cs
- UrlPath.cs
- QueryReaderSettings.cs
- ProcessHostServerConfig.cs
- CodeDomLoader.cs
- DataKey.cs
- ResizeGrip.cs
- TrackingLocation.cs
- QuotedPrintableStream.cs
- VariableDesigner.xaml.cs
- IOException.cs
- QuaternionRotation3D.cs
- FilteredXmlReader.cs
- DataMisalignedException.cs
- XmlILAnnotation.cs
- ObjectCloneHelper.cs
- UrlMappingsModule.cs
- IndependentAnimationStorage.cs
- IntSecurity.cs
- WhereQueryOperator.cs
- ServiceBehaviorAttribute.cs
- XmlHierarchyData.cs
- FontWeight.cs
- TileModeValidation.cs
- GestureRecognizer.cs
- ListQueryResults.cs
- PropertyEntry.cs
- TextRangeAdaptor.cs
- GroupQuery.cs
- DetailsViewCommandEventArgs.cs
- ScriptingSectionGroup.cs
- securitycriticaldataClass.cs
- WebMessageFormatHelper.cs
- InfoCardBaseException.cs
- ThrowHelper.cs
- ExpressionTextBox.xaml.cs
- Propagator.Evaluator.cs
- GroupItemAutomationPeer.cs
- ZipPackagePart.cs
- PublishLicense.cs
- IdentityModelDictionary.cs
- COM2ExtendedTypeConverter.cs
- ConstNode.cs
- SamlAuthorizationDecisionClaimResource.cs
- RelationshipEndMember.cs
- SmtpException.cs
- UIElement3D.cs
- ChannelFactory.cs
- XmlParserContext.cs
- ColumnHeader.cs
- ExclusiveCanonicalizationTransform.cs
- EditorZoneBase.cs