Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Ink / GestureRecognizer.cs / 1305600 / GestureRecognizer.cs
//---------------------------------------------------------------------------- // // File: GestureRecognizer.cs // // Description: // The implementation of GestureRecognizer class // // Features: // // History: // 01/14/2005 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Utility; using MS.Internal.Ink.GestureRecognition; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Runtime.InteropServices; using System; using System.Security; using System.Security.Permissions; using SecurityHelper=MS.Internal.SecurityHelper; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Ink { ////// Performs gesture recognition on a StrokeCollection /// ////// No finalizer is defined because all unmanaged resources are wrapped in SafeHandle's /// NOTE: this class provides the public APIs that call into unmanaged code to perform /// recognition. There are two inputs that are accepted: /// ApplicationGesture[] and StrokeCollection. /// This class verifies the ApplicationGesture[] and StrokeCollection / Stroke are validated. /// public sealed class GestureRecognizer : DependencyObject, IDisposable { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// The default constructor which enables all the application gestures. /// public GestureRecognizer() : this ( new ApplicationGesture[] { ApplicationGesture.AllGestures } ) { } ////// The constructor which take an array of the enabled application gestures. /// /// public GestureRecognizer(IEnumerableenabledApplicationGestures) { _nativeRecognizer = NativeRecognizer.CreateInstance(); if (_nativeRecognizer == null) { //just verify the gestures NativeRecognizer.GetApplicationGestureArrayAndVerify(enabledApplicationGestures); } else { // // we only set this if _nativeRecognizer is non null // (available) because there is no way to use this state // otherwise. // SetEnabledGestures(enabledApplicationGestures); } } #endregion Constructors //-------------------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------------------- #region Public Methods /// /// Set the enabled gestures /// /// ////// We wrap the System.Runtime.InteropServices.COMException with a more specific one /// /// Do wrap specific exceptions in a more descriptive exception when appropriate. /// public Int32 GetInt(Int32[] array, Int32 index){ /// try{ /// return array[index]; /// }catch(IndexOutOfRangeException e){ /// throw new ArgumentOutOfRangeException( /// Parameter index is out of range.); /// } /// } /// /// public void SetEnabledGestures(IEnumerableapplicationGestures) { VerifyAccess(); VerifyDisposed(); VerifyRecognizerAvailable(); //don't verfify the gestures, NativeRecognizer.SetEnabledGestures does it // since it is the TAS boundary // // we don't wrap the COM exceptions generated from the Recognizer // with our own exception // ApplicationGesture[] enabledGestures = _nativeRecognizer.SetEnabledGestures(applicationGestures); //only update the state when SetEnabledGestures succeeds (since it verifies the array) _enabledGestures = enabledGestures; } /// /// Get the enabled gestures /// ///public ReadOnlyCollection GetEnabledGestures() { VerifyAccess(); VerifyDisposed(); VerifyRecognizerAvailable(); //can be null if the call to SetEnabledGestures failed if (_enabledGestures == null) { _enabledGestures = new ApplicationGesture[] { }; } return new ReadOnlyCollection (_enabledGestures); } /// /// Performs gesture recognition on the StrokeCollection if a gesture recognizer /// is present and installed on the system. If not, this method throws an InvalidOperationException. /// To determine if this method will throw an exception, only call this method if /// the RecognizerAvailable property returns true. /// /// /// The StrokeCollection to perform gesture recognition on ////// Callers must have UnmanagedCode permission to call this API. ////// Critical: Calls SecurityCritical method RecognizeImpl /// /// PublicOK: We demand UnmanagedCode before proceeding and /// UnmanagedCode is the only permission we assert. /// [SecurityCritical] public ReadOnlyCollectionRecognize(StrokeCollection strokes) { // // due to possible exploits in the Tablet PC Gesture recognizer's Recognize method, // we demand unmanaged code. // SecurityHelper.DemandUnmanagedCode(); return RecognizeImpl(strokes); } /// /// Performs gesture recognition on the StrokeCollection if a gesture recognizer /// is present and installed on the system. If not, this method throws an InvalidOperationException. /// To determine if this method will throw an exception, only call this method if /// the RecognizerAvailable property returns true. /// /// The StrokeCollection to perform gesture recognition on ////// /// Critical: Calls a SecurityCritical method RecognizeImpl. /// /// FriendAccess is allowed so the critical method InkCanvas.RaiseGestureOrStrokeCollected /// can use this method /// // Built into Core, also used by Framework. [SecurityCritical] internal ReadOnlyCollectionCriticalRecognize(StrokeCollection strokes) { return RecognizeImpl(strokes); } /// /// Performs gesture recognition on the StrokeCollection if a gesture recognizer /// is present and installed on the system. /// /// The StrokeCollection to perform gesture recognition on ////// /// Critical: Calls SecurityCritical method NativeRecognizer.Recognize /// [SecurityCritical] private ReadOnlyCollectionRecognizeImpl(StrokeCollection strokes) { if (strokes == null) { throw new ArgumentNullException("strokes"); // Null is not allowed as the argument value } if (strokes.Count > 2) { throw new ArgumentException(SR.Get(SRID.StrokeCollectionCountTooBig), "strokes"); } VerifyAccess(); VerifyDisposed(); VerifyRecognizerAvailable(); return new ReadOnlyCollection (_nativeRecognizer.Recognize(strokes)); } #endregion Public Methods //-------------------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------------------- #region Public Properties /// /// Indicates if a GestureRecognizer is present and installed on the system. /// public bool IsRecognizerAvailable { get { VerifyAccess(); VerifyDisposed(); if (_nativeRecognizer == null) { return false; } return true; } } #endregion Public Properties //------------------------------------------------------------------------------- // // IDisposable // //-------------------------------------------------------------------------------- #region IDisposable ////// Dispose this GestureRecognizer /// public void Dispose() { VerifyAccess(); // A simple pattern of the dispose implementation. // There is no finalizer since the SafeHandle in the NativeRecognizer will release the context properly. if ( _disposed ) { return; } // NTRAID-WINDOWSBUG#1102945-WAYNEZEN, // Since the constructor might create a null _nativeRecognizer, // here we have to make sure we do have some thing to dispose. // Otherwise just no-op. if ( _nativeRecognizer != null ) { _nativeRecognizer.Dispose(); _nativeRecognizer = null; } _disposed = true; } #endregion IDisposable //------------------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------------------- #region Private Methods //verify that there is a recognizer available, throw if not private void VerifyRecognizerAvailable() { if (_nativeRecognizer == null) { throw new InvalidOperationException(SR.Get(SRID.GestureRecognizerNotAvailable)); } } // Verify whether this object has been disposed. private void VerifyDisposed() { if ( _disposed ) { throw new ObjectDisposedException("GestureRecognizer"); } } #endregion Private Methods //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private ApplicationGesture[] _enabledGestures; private NativeRecognizer _nativeRecognizer; private bool _disposed; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
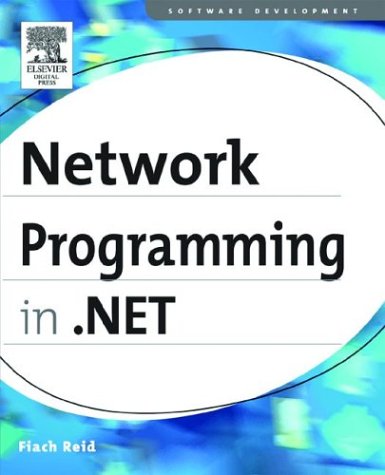
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FrameworkContextData.cs
- ManipulationDelta.cs
- TransactionTraceIdentifier.cs
- DataGridViewCheckBoxCell.cs
- TimeSpanSecondsConverter.cs
- IntegerFacetDescriptionElement.cs
- Model3DGroup.cs
- iisPickupDirectory.cs
- PolyQuadraticBezierSegment.cs
- ProviderCollection.cs
- Parsers.cs
- SerializationObjectManager.cs
- AdCreatedEventArgs.cs
- MarginCollapsingState.cs
- HtmlTableRowCollection.cs
- CreateUserWizard.cs
- PartialArray.cs
- VisualBrush.cs
- ComplexPropertyEntry.cs
- FormViewCommandEventArgs.cs
- StrongNamePublicKeyBlob.cs
- PrincipalPermission.cs
- SerializerDescriptor.cs
- PropertyGridView.cs
- SafeRightsManagementHandle.cs
- TreeSet.cs
- BufferAllocator.cs
- PageStatePersister.cs
- SupportedAddressingMode.cs
- WebPartConnectionsDisconnectVerb.cs
- WebPartTransformerAttribute.cs
- TableMethodGenerator.cs
- DockAndAnchorLayout.cs
- OleDbConnectionInternal.cs
- PrefixQName.cs
- ImageFormatConverter.cs
- QilInvoke.cs
- dsa.cs
- WindowsRichEdit.cs
- TableRowCollection.cs
- NumberAction.cs
- UserControlAutomationPeer.cs
- RecipientInfo.cs
- LayoutTableCell.cs
- PasswordPropertyTextAttribute.cs
- ExceptionCollection.cs
- ComponentEditorForm.cs
- SecurityToken.cs
- PriorityBindingExpression.cs
- BinaryExpressionHelper.cs
- SiteMapPath.cs
- HttpChannelBindingToken.cs
- ProfileServiceManager.cs
- PropertyEmitterBase.cs
- ExtentKey.cs
- SettingsBindableAttribute.cs
- FilterEventArgs.cs
- WebPartsSection.cs
- SqlWriter.cs
- RequestNavigateEventArgs.cs
- DataGridCommandEventArgs.cs
- ExternalCalls.cs
- OdbcRowUpdatingEvent.cs
- XPathConvert.cs
- PagesSection.cs
- DecoderFallback.cs
- ButtonStandardAdapter.cs
- ProjectedWrapper.cs
- Control.cs
- ProtectedProviderSettings.cs
- Processor.cs
- FileUtil.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- XmlSchemaException.cs
- Clock.cs
- ForeignKeyConstraint.cs
- SoapServerProtocol.cs
- BehaviorEditorPart.cs
- WindowsIdentity.cs
- PersonalizationEntry.cs
- CacheEntry.cs
- Parser.cs
- StateChangeEvent.cs
- XmlSerializerVersionAttribute.cs
- MetaForeignKeyColumn.cs
- TreeNodeCollectionEditor.cs
- ASCIIEncoding.cs
- AccessDataSource.cs
- SubclassTypeValidator.cs
- TreeViewBindingsEditorForm.cs
- GuidTagList.cs
- XmlSchemaAnnotated.cs
- InvalidOperationException.cs
- RayHitTestParameters.cs
- MexHttpsBindingElement.cs
- RoleService.cs
- PlanCompiler.cs
- Msec.cs
- LogRecordSequence.cs
- ObjectStateEntry.cs