Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / MetaForeignKeyColumn.cs / 1305376 / MetaForeignKeyColumn.cs
using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Security.Permissions; using System.Web.DynamicData.ModelProviders; using System.Linq; using System.Web.UI; namespace System.Web.DynamicData { ////// A special column representing many-1 relationships /// public class MetaForeignKeyColumn : MetaColumn, IMetaForeignKeyColumn { // Maps a foreign key name to the name that should be used in a Linq expression for filtering // i.e. the foreignkey name might be surfaced through a custom type descriptor e.g. CategoryID but we might really want to use // Category.CategoryId in the expression private Dictionary_foreignKeyFilterMapping; public MetaForeignKeyColumn(MetaTable table, ColumnProvider entityMember) : base(table, entityMember) { } /// /// Perform initialization logic for this column /// internal protected override void Initialize() { base.Initialize(); ParentTable = Model.GetTable(Provider.Association.ToTable.Name, Table.DataContextType); CreateForeignKeyFilterMapping(ForeignKeyNames, ParentTable.PrimaryKeyNames, (foreignKey) => Table.EntityType.GetProperty(foreignKey) != null); } internal void CreateForeignKeyFilterMapping(IListforeignKeyNames, IList primaryKeyNames, Func propertyExists) { // HACK: Some tests don't mock foreign key names, but this should never be the case at runtime if (foreignKeyNames == null) { return; } int pKIndex = 0; foreach (string fkName in foreignKeyNames) { if (!propertyExists(fkName)) { if (_foreignKeyFilterMapping == null) { _foreignKeyFilterMapping = new Dictionary (); } _foreignKeyFilterMapping[fkName] = Name + "." + primaryKeyNames[pKIndex]; } pKIndex++; } } /// /// The parent table of the relationship (e.g. Categories in Products->Categories) /// public MetaTable ParentTable { get; // internal for unit testing internal set; } ////// Returns true if this foriegn key column is part of the primary key of its table /// e.g. Order and Product are PKs in the Order_Details table /// public bool IsPrimaryKeyInThisTable { get { return Provider.Association.IsPrimaryKeyInThisTable; } } ////// This is used when saving the value of a foreign key, e.g. when selected from a drop down. /// public void ExtractForeignKey(IDictionary dictionary, string value) { if (String.IsNullOrEmpty(value)) { // If the value is null, set all the FKs to null foreach (string fkName in ForeignKeyNames) { dictionary[fkName] = null; } } else { string[] fkValues = Misc.ParseCommaSeparatedString(value); Debug.Assert(fkValues.Length == ForeignKeyNames.Count); for (int i = 0; i < fkValues.Length; i++) { dictionary[ForeignKeyNames[i]] = fkValues[i]; } } } ////// Return the value of all the foreign keys components for the passed in row /// public IList
Link Menu
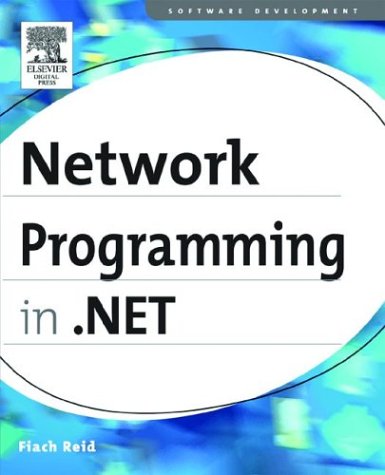
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataSourceControlBuilder.cs
- UpdatePanelTriggerCollection.cs
- DataTableExtensions.cs
- MeshGeometry3D.cs
- LayoutDump.cs
- ControlAdapter.cs
- EventData.cs
- SqlDataSourceCustomCommandPanel.cs
- WindowsBrush.cs
- WebDisplayNameAttribute.cs
- FamilyMapCollection.cs
- VisualBasicHelper.cs
- CategoryAttribute.cs
- ResourceDescriptionAttribute.cs
- ChildTable.cs
- RemoveStoryboard.cs
- KeyConverter.cs
- AmbientValueAttribute.cs
- CircleHotSpot.cs
- CustomErrorCollection.cs
- SqlXml.cs
- UInt64Storage.cs
- ParameterReplacerVisitor.cs
- ScrollEventArgs.cs
- EraserBehavior.cs
- ScriptManager.cs
- SchemaNamespaceManager.cs
- ToolboxCategory.cs
- MsmqIntegrationSecurityMode.cs
- MarginsConverter.cs
- DetailsViewUpdateEventArgs.cs
- glyphs.cs
- InternalCompensate.cs
- UInt32.cs
- XmlSchemaRedefine.cs
- PopupRoot.cs
- GlyphsSerializer.cs
- SiteMapNodeItem.cs
- DataGridViewCellCancelEventArgs.cs
- Activation.cs
- ObjectListTitleAttribute.cs
- DetailsViewPagerRow.cs
- SpellerHighlightLayer.cs
- InfoCardSymmetricCrypto.cs
- CodeAssignStatement.cs
- SQLBytes.cs
- BindUriHelper.cs
- TableItemProviderWrapper.cs
- OdbcInfoMessageEvent.cs
- ValuePattern.cs
- OdbcConnectionStringbuilder.cs
- CustomErrorsSectionWrapper.cs
- RoleManagerModule.cs
- Control.cs
- HtmlMeta.cs
- BigInt.cs
- FrameAutomationPeer.cs
- GridViewUpdatedEventArgs.cs
- ConfigXmlElement.cs
- DependencyPropertyAttribute.cs
- RTLAwareMessageBox.cs
- MemberInfoSerializationHolder.cs
- MethodImplAttribute.cs
- EncodingDataItem.cs
- SignatureGenerator.cs
- QilVisitor.cs
- Substitution.cs
- DeferrableContentConverter.cs
- BuildProviderUtils.cs
- PropertyHelper.cs
- StylusPointProperties.cs
- Misc.cs
- PrintDocument.cs
- OracleBFile.cs
- TypeResolvingOptionsAttribute.cs
- LostFocusEventManager.cs
- FindCompletedEventArgs.cs
- SchemaElement.cs
- SecurityPolicySection.cs
- Bezier.cs
- ObjectAnimationBase.cs
- BindingWorker.cs
- LambdaCompiler.Expressions.cs
- PackageStore.cs
- HtmlLink.cs
- CopyNamespacesAction.cs
- ValuePattern.cs
- ArrayEditor.cs
- CompilerResults.cs
- XmlUtilWriter.cs
- TextRangeBase.cs
- CipherData.cs
- VisualCollection.cs
- MimeMultiPart.cs
- SafeArrayTypeMismatchException.cs
- InertiaTranslationBehavior.cs
- SignedInfo.cs
- CqlBlock.cs
- RectValueSerializer.cs
- AutoSizeToolBoxItem.cs